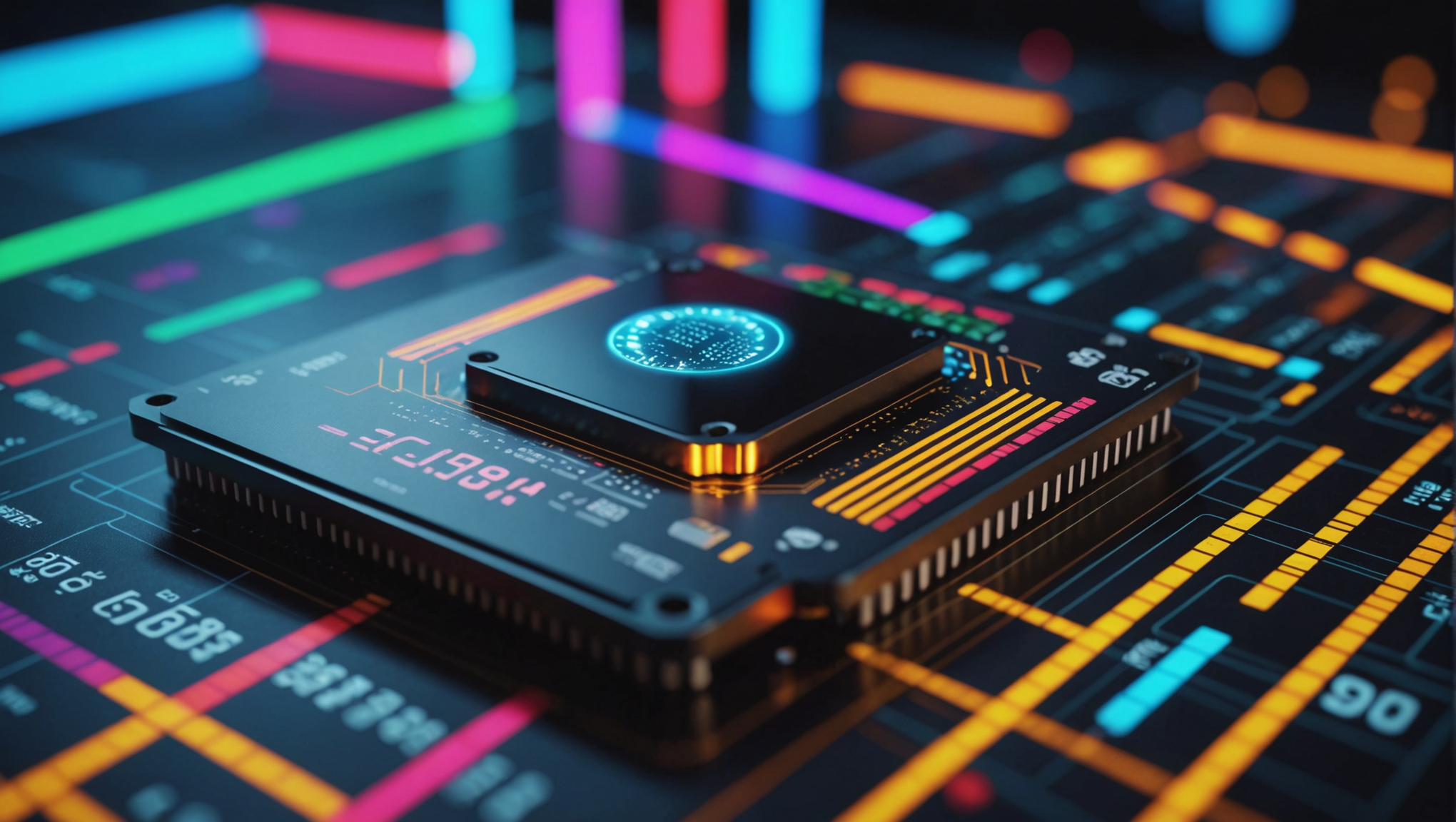
SQL Server Performance Monitoring
Monitoring SQL Server performance effectively requires a solid understanding of key metrics that can indicate the health and efficiency of the database system. Here are some of the most crucial metrics to keep an eye on:
- High CPU utilization can indicate inefficient queries or insufficient server resources. Monitoring the CPU usage over time helps in identifying peaks and potential bottlenecks.
- SQL Server uses memory to cache data and execution plans. It’s important to monitor memory usage to ensure that there is enough available memory for optimal performance. Look out for Page Life Expectancy to measure how long a page stays in the buffer cache.
- The ratio of reads to writes can indicate how well the storage subsystem performs. Monitoring the Disk Queue Length can reveal delays in I/O operations.
- SQL Server tracks waits on various resources. Analyzing wait statistics can help identify bottlenecks and contention issues. Common waits include PAGEIOLATCH and LOCK waits.
- Tracking the execution time of queries is essential. Use the SQL Server Profiler or Extended Events to identify slow-running queries and their execution plans.
- Monitoring the size and usage of the transaction log can help avoid potential issues related to transactions being unable to commit due to insufficient log space.
Here are some SQL queries that can help you monitor these key metrics:
-- Query to monitor CPU usage SELECT TOP 10 recordID, CPU, duration, total_elapsed_time FROM sys.dm_exec_requests WHERE session_id > 50 ORDER BY CPU DESC; -- Query to check memory usage SELECT (physical_memory_in_use_kb / 1024) AS Memory_Used_MB, (large_page_kb / 1024) AS Large_Page_MB, (virtual_memory_committed_kb / 1024) AS Virtual_Memory_MB FROM sys.dm_os_process_memory; -- Query to monitor wait statistics SELECT wait_type, wait_time_ms / 1000.0 AS Wait_Time_S, waiting_tasks_count AS Wait_Count, wait_time_ms / waiting_tasks_count AS Avg_Wait_Time_S FROM sys.dm_os_wait_stats ORDER BY wait_time_ms DESC;
Tools and Techniques for Monitoring
When it comes to SQL Server performance monitoring, using the right tools and techniques is essential for effectively tracking the performance metrics identified earlier. SQL Server provides a multitude of built-in tools, as well as external solutions, that can help administrators monitor, troubleshoot, and optimize the database environment. Here are some important tools and techniques to consider:
- SSMS is a powerful integrated environment for managing SQL Server infrastructure. It offers various graphical tools and wizards to monitor server health, performance, and activity. Using Activity Monitor within SSMS provides real-time information on processes, resource usage, and performance statistics.
- DMVs are system views that provide information about server state and performance. They’re instrumental for querying performance-related information, such as active sessions and resource usage. The earlier provided queries utilize DMVs to extract key metrics.
- This graphical tool allows administrators to capture and analyze events occurring in the SQL Server instance. By defining a trace and monitoring specific events, such as SQL query execution and performance statistics, administrators can identify slow-running queries and optimize them.
- A lightweight performance monitoring system that enables users to collect data about SQL Server events. It is more flexible and powerful than SQL Server Profiler, allowing for detailed tracking of events without significant overhead on server performance.
- This Windows tool can be used to monitor SQL Server running on Windows. By tracking various performance counters such as CPU, memory, and disk I/O, it provides a comprehensive view of the server’s performance over time.
-
High CPU Usage:
High CPU usage can stem from inefficient queries, poorly indexed tables, or high levels of parallelism. Common solutions include:
- Optimizing queries by examining execution plans to identify bottlenecks.
- Creating or adjusting indexes on frequently queried columns to speed up data retrieval.
- Reducing parallel processing by adjusting the max degree of parallelism setting.
Here’s an example query to identify long-running queries that may be causing high CPU usage:
SELECT TOP 10 total_worker_time / 1000 AS CPU_Time_MS, execution_count, total_elapsed_time / 1000 AS Total_Elapsed_Time_MS, SUBSTRING(qt.text, (qs.statement_start_offset/2)+1, ((CASE qs.statement_end_offset WHEN -1 THEN DATALENGTH(qt.text) ELSE qs.statement_end_offset END - qs.statement_start_offset)/2) + 1) AS Query_Text FROM sys.dm_exec_query_stats AS qs CROSS APPLY sys.dm_exec_sql_text(qs.sql_handle) AS qt ORDER BY CPU_Time_MS DESC;
-
Memory Pressure:
Memory pressure can lead to slower response times if SQL Server is unable to cache data effectively. Solutions include:
- Increasing the SQL Server’s maximum memory allocation to ensure it has sufficient resources.
- Reviewing and optimizing queries and their associated execution plans to ensure they use memory efficiently.
- Checking for memory leaks or issues in other applications running on the same server.
-
Slow Query Performance:
Slow-running queries can significantly affect overall performance. Here’s how to address it:
- Use the SQL Server Profiler or Extended Events to capture execution details and identify slow queries.
- Analyze execution plans to determine if indexes or optimizations are needed.
- Implement query hints or re-write queries for better performance.
Here’s a query to analyze the performance of queries executed in the last day:
SELECT TOP 10 qs.total_elapsed_time / qs.execution_count AS Avg_Elapsed_Time, qt.text AS Query_Text FROM sys.dm_exec_query_stats AS qs CROSS APPLY sys.dm_exec_sql_text(qs.sql_handle) AS qt WHERE qs.creation_time >= DATEADD(DAY, -1, GETDATE()) ORDER BY Avg_Elapsed_Time DESC;
- Continuously monitor key performance metrics and review collected data to identify trends. Set up alerts for significant deviations from the established performance baselines to facilitate quick intervention. Utilize tools like SQL Server Management Studio and Dynamic Management Views (DMVs) for real-time insights.
- Proper index management is vital for performance. Regularly review and optimize indexes to ensure they are aligned with query patterns. Ponder implementing scheduled tasks for index rebuilding and reorganizing to reduce fragmentation. Here’s an SQL command to rebuild an index:
Common Performance Issues and Solutions
When dealing with SQL Server performance issues, it’s important to identify the common problems that can arise and the corresponding solutions to mitigate them. Key performance challenges include high CPU usage, memory pressure, slow query performance, and I/O bottlenecks. Below are some common issues along with their solutions:
Best Practices for Ongoing Performance Optimization
To ensure ongoing performance optimization in SQL Server, it especially important to implement best practices that align with the needs of your specific environment. Here are several strategies that can help maintain and enhance your SQL Server performance:
ALTER INDEX [IndexName] ON [TableName] REBUILD;
SELECT TOP 10 qs.total_elapsed_time / qs.execution_count AS Avg_Elapsed_Time, qt.text AS Query_Text FROM sys.dm_exec_query_stats AS qs CROSS APPLY sys.dm_exec_sql_text(qs.sql_handle) AS qt ORDER BY Avg_Elapsed_Time DESC;
UPDATE STATISTICS [TableName];