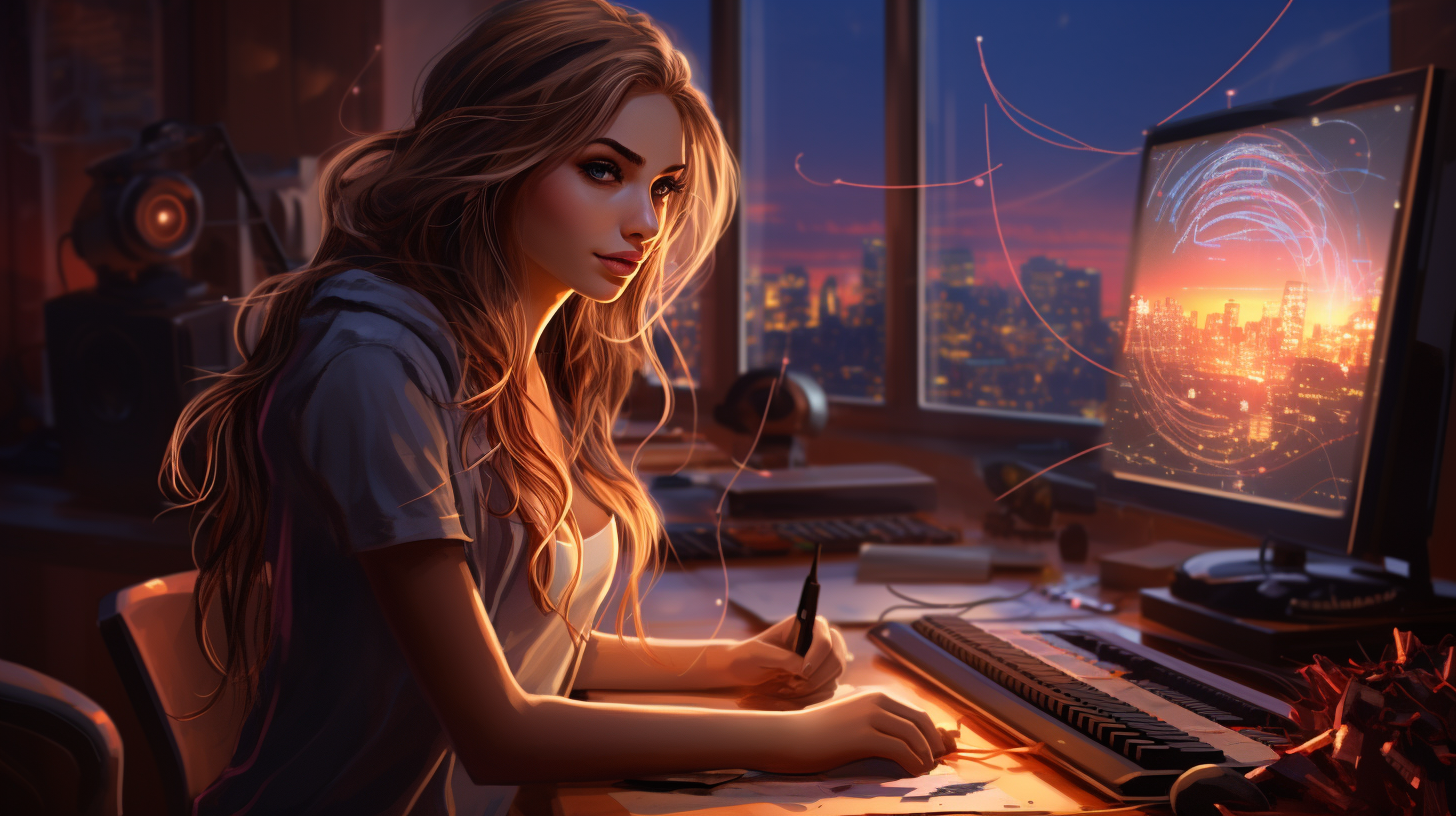
SQL Server Replication Techniques
SQL Server Replication is a powerful feature that allows for the distribution of data across multiple databases, ensuring that changes made in one database are reflected in others. It enables various scenarios, such as data integration, load balancing, and high availability. By replicating data, SQL Server helps maintain consistency across different systems while providing flexibility in application design.
Replication is built on a publish/subscribe model, where a publisher sends data changes to subscribers. This mechanism helps in creating a distributed system, allowing applications to read from local databases while keeping data synchronized with a central server. SQL Server supports several replication types, catering to different use cases and requirements.
Understanding the fundamentals of SQL Server Replication is essential for database administrators and developers alike. The main components involved in SQL Server Replication include:
- The source database that makes data available for replication.
- The target database that receives data from the publisher.
- A server that acts as a mediator between the publisher and subscriber, managing the flow of data and tracking changes.
SQL Server Replication can be configured to work in various environments, whether on-premises or in cloud scenarios. It can be utilized for:
- Distributing data for reporting purposes.
- Synchronizing data between different geographic locations.
- Providing high availability for critical applications.
The replication process involves creating publications and subscriptions. A publication is a collection of database objects that are made available for replication, while a subscription is a request for the data contained in a publication. Here is a simplified example of how to create a publication:
EXEC sp_addpublication @publication = 'SamplePublication', @status = 'active', @description = 'Sample publication for replication', @sync_method = 'native', @force_invalidate_snapshot = 1;
After setting up a publication, you can add a subscriber to receive the replicated data:
EXEC sp_addsubscription @publication = 'SamplePublication', @subscriber = 'SubscriberServer', @destination_db = 'SubscriberDatabase', @sync_type = 'native';
Monitoring the status of replication is important for ensuring that data is being propagated correctly. SQL Server provides several tools and DMVs (Dynamic Management Views) to help track replication health and performance. Understanding the current state of replication can prevent issues that may arise from data discrepancies.
Types of SQL Server Replication
SQL Server supports several types of replication to accommodate different needs and scenarios. Each type is designed with specific use cases in mind, allowing administrators to select the most suitable method based on their requirements for data distribution, consistency, and performance. The three primary types of SQL Server replication are Snapshot Replication, Transactional Replication, and Merge Replication.
Snapshot Replication is the simplest form of replication. In this method, a complete copy of the data is taken at a specific point in time and sent to subscribers. This approach is ideal when the changes to the data are infrequent or when the dataset is relatively small. The entire snapshot is refreshed at regular intervals, which can be scheduled according to the needs of the organization. Here’s how you can set up Snapshot Replication:
EXEC sp_addpublication @publication = 'SnapshotPublication', @status = 'active', @description = 'Snapshot replication for infrequent changes', @snapshot_folder = 'C:Snapshots', @sync_method = 'native';
Next, you need to add a subscription to this publication:
EXEC sp_addsubscription @publication = 'SnapshotPublication', @subscriber = 'SubscriberServer', @destination_db = 'SubscriberDatabase', @sync_type = 'snapshot';
Transactional Replication, on the other hand, is suited for scenarios where data changes frequently and near real-time data consistency is required. This method replicates individual data changes (inserts, updates, and deletes) as they occur. It offers higher performance than Snapshot Replication because it minimizes the amount of data transferred at any given time. To set up Transactional Replication, you can execute the following:
EXEC sp_addpublication @publication = 'TransactionalPublication', @status = 'active', @description = 'Transactional replication for high-frequency updates', @replication_type = 'transactional';
Then, add a subscriber:
EXEC sp_addsubscription @publication = 'TransactionalPublication', @subscriber = 'SubscriberServer', @destination_db = 'SubscriberDatabase', @sync_type = 'transactional';
Merge Replication is a more complex type that allows changes to be made at both the publisher and subscriber databases. This is particularly useful in scenarios where subscribers may not always be connected to the publisher (e.g., mobile applications). Merge Replication combines the changes made at both ends and resolves any conflicts that may arise. To set up Merge Replication, you would use:
EXEC sp_addpublication @publication = 'MergePublication', @status = 'active', @description = 'Merge replication for bi-directional updates', @replication_type = 'merge';
Followed by adding a subscriber:
EXEC sp_addsubscription @publication = 'MergePublication', @subscriber = 'SubscriberServer', @destination_db = 'SubscriberDatabase', @sync_type = 'merge';
Each replication type serves its purpose, and the choice of which to implement should be based on the specific requirements of the application environment, the frequency of data updates, and the need for data consistency. Understanding these replication types is fundamental for effectively managing a SQL Server environment and ensuring that data is appropriately synchronized across different databases.
Configuring SQL Server Replication
Configuring SQL Server Replication requires a systematic approach to ensure that the publication, subscription, and distributor components work seamlessly together. The steps below provide a roadmap to set up replication effectively.
First, ensure that the necessary SQL Server services are running. You need the SQL Server Agent and the SQL Server Replication Monitor to be active. The following commands can help you verify the status:
EXEC sp_server_info; EXEC sp_helpserver;
Next, you need to define the distributor. The distributor can be configured either on the same server as the publisher or on a separate server. Here’s how you can configure the distributor on the publisher:
EXEC sp_adddistributor @distributor = 'DistributorServer', @password = 'your_password';
After establishing the distributor, the next step is to configure the publication. The following SQL code snippet demonstrates how to create a publication with a specified database:
EXEC sp_addpublication @publication = 'MyPublication', @database = 'MyDatabase', @status = 'active', @description = 'Example publication for replication', @sync_method = 'native', @allow_push = 'true', @allow_pull = 'true', @allow_anonymous = 'false';
Once the publication is created, you need to define the articles that will be included in this publication. Articles refer to the specific database objects, such as tables and stored procedures, that you want to replicate:
EXEC sp_addarticle @publication = 'MyPublication', @article = 'MyTable', @source_object = 'MyTable', @type = 'logbased';
Now, now, let’s create the subscription. This is where you specify which server will receive the replicated data. The subscriber can either be on the same server as the publisher or on a different server altogether:
EXEC sp_addsubscription @publication = 'MyPublication', @subscriber = 'SubscriberServer', @destination_db = 'SubscriberDatabase', @sync_type = 'push';
If you want to set up a pull subscription, the subscriber initiates the data transfer. The configuration would look slightly different, involving the distribution agent, which will periodically check for changes:
EXEC sp_addsubscription @publication = 'MyPublication', @subscriber = 'SubscriberServer', @destination_db = 'SubscriberDatabase', @sync_type = 'pull';
After creating the publication and subscriptions, ensure that you initialize the subscriptions. Initialization especially important for the subscriber to receive the initial snapshot of the publication:
EXEC sp_start_job @job_name = 'distribution';
It’s also essential to monitor the replication health once configured. You can query the status of the replication using the following command:
SELECT * FROM distribution.dbo.MSdistribution_history;
This will provide you with insights into the last synchronization status, any errors encountered, and the overall health of the replication process. Regular monitoring is vital to maintain data integrity and performance.
Monitoring and Troubleshooting Replication
Monitoring SQL Server Replication involves a careful balance of observation and analysis to ensure that data flows smoothly between publisher and subscriber. Without diligent monitoring, issues such as lag, failed transactions, or data discrepancies can arise, undermining the integrity of your distributed systems. SQL Server provides several tools and techniques to facilitate this oversight.
One of the primary methods for monitoring replication status is through the use of Replication Monitor. This graphical tool allows you to visualize the health of your replication setup, providing details on the synchronization status of each publication and subscription. You can access it through SQL Server Management Studio (SSMS) by navigating to the Replication folder and selecting “Launch Replication Monitor.” From here, you can monitor the following:
- The time it takes for changes to propagate from the publisher to the subscribers.
- Whether the data has been delivered to the subscriber successfully.
- Any issues that have occurred during the replication process.
In addition to the graphical interface, SQL Server also provides a suite of Dynamic Management Views (DMVs) that allow for programmatic access to replication metrics. For instance, the following query can be used to gather information about the status of replication agents:
SELECT ra.name AS agent_name, ra.status AS agent_status, ra.last_action AS last_action, ra.last_action_time AS last_action_time, ra.error_id AS error_id, ra.error_description AS error_description FROM distribution.dbo.MSagents ra WHERE ra.type IN (1, 2); -- 1 = Snapshot, 2 = Transactional
This query filters the agents to show only those related to Snapshot and Transactional Replication, enabling you to quickly assess their performance. Monitoring the various statuses and errors reported by these agents very important for preemptively identifying and resolving issues.
Another critical aspect of monitoring replication is checking the health of the distribution database. The distribution database contains metadata and history about the replication process. You can use the following query to examine the health and size of the distribution database:
SELECT db.name, mf.physical_name, mf.size / 128 AS size_in_MB, mf.max_size, mf.state_desc FROM sys.master_files mf INNER JOIN sys.databases db ON db.database_id = mf.database_id WHERE db.name = 'distribution';
This command provides information about the distribution database’s physical files, their sizes, and their current state. Keeping an eye on the size is especially important; as the distribution database grows, you may need to manage it to avoid performance degradation.
When troubleshooting replication issues, it’s vital to leverage the error logs and history tables provided by SQL Server. The MSdistribution_history
table contains records of the last synchronization attempts and any errors that occurred during the process. For example, you can execute the following command to view the history of delivery attempts:
SELECT *, ROW_NUMBER() OVER (ORDER BY time) AS row_num FROM distribution.dbo.MSdistribution_history WHERE publication_id = 'YourPublicationID' ORDER BY time DESC;
By querying this table, you can pinpoint when issues occurred and assess whether they were transient or indicative of deeper systemic problems.
Lastly, setting up alerts is an essential part of monitoring replication health. SQL Server Agent can be configured to send notifications when certain thresholds are met, such as increased latency or agent failures. This proactive approach allows you to react swiftly to potential issues, minimizing downtime and data inconsistencies.
Effective monitoring and troubleshooting of SQL Server replication require a combination of built-in tools, querying DMVs, examining distribution health, and establishing alerting mechanisms. By employing these strategies, database administrators can maintain a robust and reliable replication environment, ensuring that data integrity is upheld across all systems.