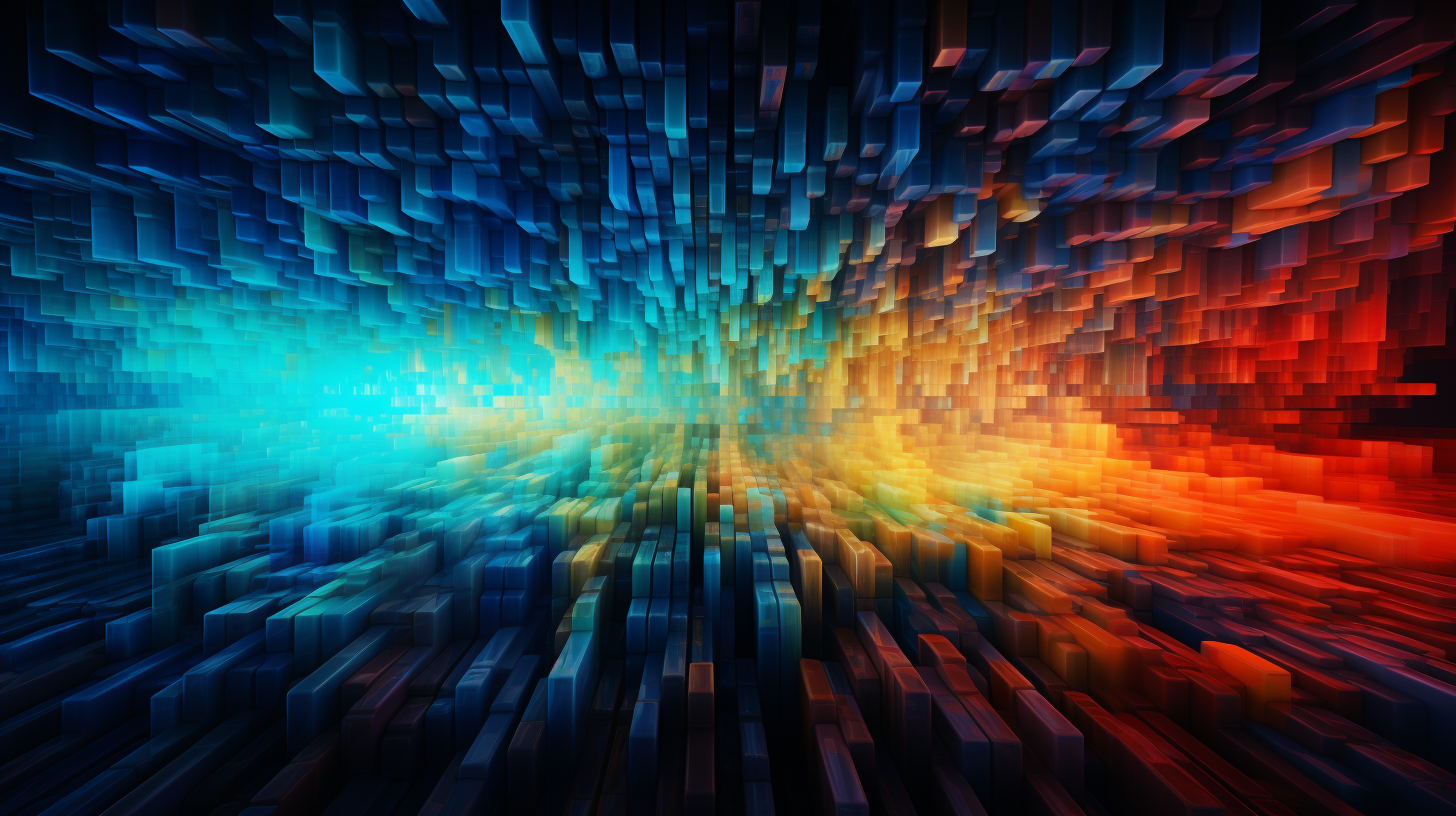
SQL Techniques for Quick Data Retrieval
Indexing is an important technique in SQL that dramatically enhances data retrieval speed. By creating indexes on your tables, you provide the database engine with a structured way to quickly find rows that match certain criteria. However, crafting the right indexing strategy requires an understanding of how indexes work and when they’re most effective.
There are several types of indexes available in SQL databases:
- An index on a single column to improve the performance of queries filtering on that column.
- Indexes that cover multiple columns, useful for queries that filter or sort based on more than one column.
- Ensure that values in the indexed column are unique, enforcing data integrity in addition to speeding up retrieval.
- Designed for querying large text fields, enabling efficient searching of words within text.
To illustrate the effectiveness of indexing, consider the following example. Here is how you might create a single-column index on a ‘customers’ table:
CREATE INDEX idx_customer_name ON customers(name);
Now, if you run a query that selects customers by name, the database can utilize the index to speed up the search:
SELECT * FROM customers WHERE name = 'Mitch Carter';
For queries that involve multiple columns, a composite index is advantageous. For example, in a sales database, if you frequently query sales records by both ‘customer_id’ and ‘order_date’, you might define a composite index as follows:
CREATE INDEX idx_customer_order ON sales(customer_id, order_date);
With this index in place, the following query will execute significantly faster:
SELECT * FROM sales WHERE customer_id = 123 AND order_date >= '2023-01-01';
However, it’s essential to strike a balance between the number of indexes and the performance of write operations. Each index requires additional storage space and incurs a cost during INSERT, UPDATE, and DELETE operations because the index must also be updated. Therefore, you should carefully evaluate which columns are most frequently used in WHERE clauses, ORDER BY clauses, and JOIN conditions to decide where to place your indexes.
Moreover, you can monitor the performance of your queries and adjust your indexing strategy accordingly. SQL Server, for instance, provides dynamic management views to help analyze index usage. Here’s an example to retrieve the usage statistics of indexes:
SELECT * FROM sys.dm_db_index_usage_stats WHERE database_id = DB_ID('YourDatabaseName');
By using effective indexing strategies, you can help ensure that your SQL queries perform at their best, enabling quick data retrieval and an overall enhanced user experience.
Using Joins for Enhanced Performance
When it comes to optimizing SQL queries, using joins effectively can significantly enhance performance, especially when dealing with multiple tables. Joins allow you to combine rows from two or more tables based on a related column, making it easier to retrieve the necessary data without extensive subqueries or multiple queries.
There are several types of joins in SQL, each serving a distinct purpose:
- Returns records that have matching values in both tables.
- Returns all records from the left table and the matched records from the right table. If there is no match, NULL values are returned for columns from the right table.
- Similar to the Left Join, but returns all records from the right table and the matched records from the left table.
- Returns records when there is a match in either left or right table records.
- Returns the Cartesian product of both tables, meaning it combines every row of the first table with every row of the second table.
To demonstrate the power of joins, consider a simple database with two tables: employees
and departments
. The employees
table might contain employee details, and the departments
table would include department information. If you want to retrieve a list of employees along with their respective department names, an inner join would be appropriate:
SELECT e.name, d.department_name FROM employees e INNER JOIN departments d ON e.department_id = d.id;
This query efficiently combines the two tables based on the department ID, returning only those employees who belong to a department. Joins can become complex, but SQL’s optimizer generally excels at handling them if designed properly.
However, when working with left joins, you might want to include employees who do not belong to any department. This can be accomplished as follows:
SELECT e.name, d.department_name FROM employees e LEFT JOIN departments d ON e.department_id = d.id;
This query will return all employees, showing NULL for the department name where there is no matching department, thus preserving all employee records in the result set.
In addition to these techniques, performance can also be enhanced by ensuring that the columns used in join conditions are indexed. This makes the retrieval of records efficient as the database engine can quickly locate the relevant rows. For example, if you have an index on employees.department_id
and departments.id
, your joins can execute considerably faster:
CREATE INDEX idx_employee_department ON employees(department_id); CREATE INDEX idx_department_id ON departments(id);
Moreover, when working with large datasets, ponder the impact of join types on performance. Inner joins are generally faster than outer joins due to their simpler nature of only returning matched records. In contrast, outer joins can require more processing as they need to account for NULL values and unmatched records.
Understanding how to effectively utilize joins not only simplifies SQL queries but also enhances performance, making your data retrieval efforts more efficient and responsive. As with any SQL operation, continuous monitoring and optimization based on actual query performance are key to maintaining high efficiency in your database operations.
Optimizing Query Structure
Optimizing the structure of your SQL queries is pivotal for achieving maximum efficiency, particularly when dealing with complex datasets. A well-crafted query can make a significant difference in execution time, and understanding how to structure your SQL commands effectively can be the difference between a sluggish application and one that responds in the blink of an eye.
First and foremost, it’s crucial to minimize the number of rows processed by your queries. This can often be accomplished through selective filtering and proper use of WHERE clauses. For example, rather than retrieving all records from a table and letting the application handle filtering, the database should do this work:
SELECT * FROM orders WHERE order_date >= '2023-01-01';
In this example, only relevant records from the ‘orders’ table are retrieved, allowing the database to use any available indexes effectively. Avoid using SELECT * when you only need specific columns, as this not only increases the amount of data processed but also burdens the network with unnecessary data transfer.
Another common optimization tactic is to leverage aggregate functions wisely. When performing calculations on large data sets, make sure to use GROUP BY clauses judiciously:
SELECT customer_id, COUNT(*) AS order_count FROM orders GROUP BY customer_id;
This query efficiently groups the order data by customer, significantly reducing the volume of data returned compared to processing each order individually. However, ensure that any columns used in GROUP BY are indexed to further enhance performance.
Moreover, think rewriting queries for efficiency. Instead of using subqueries that can be performance-heavy, aim for joins or Common Table Expressions (CTEs) which may be more efficient. For instance, instead of:
SELECT * FROM customers WHERE id IN (SELECT customer_id FROM orders WHERE order_date >= '2023-01-01');
You can rewrite it using a join:
SELECT DISTINCT c.* FROM customers c INNER JOIN orders o ON c.id = o.customer_id WHERE o.order_date >= '2023-01-01';
This approach can often yield better performance as the database can optimize the join operation more effectively than it can handle a subquery.
Furthermore, ensure that your queries avoid unnecessary complexity. Break down intricate queries into simpler components where possible, and avoid using functions on indexed columns in WHERE clauses, as this can negate the benefit of indexing:
SELECT * FROM employees WHERE YEAR(hire_date) = 2020;
Instead, use a more simpler approach:
SELECT * FROM employees WHERE hire_date >= '2020-01-01' AND hire_date < '2021-01-01';
This minor adjustment can allow the database engine to leverage any indexes on the ‘hire_date’ column effectively.
Lastly, think the execution plan of your queries. Most SQL databases provide a way to analyze how the database engine processes your SQL commands. By checking the execution plan, you can identify potential bottlenecks and optimize your queries accordingly. For example, in SQL Server, you might use:
SET STATISTICS IO ON; SET STATISTICS TIME ON;
By following these guidelines for query structure optimization, you can ensure that your SQL commands are performing at their best, allowing for rapid data retrieval that keeps your applications responsive and your users satisfied.
Using SQL Functions for Speed
Using SQL functions can significantly improve the speed and efficiency of your data retrieval operations. SQL functions allow you to perform calculations, manipulate data, and return results in a streamlined manner, which can lead to more efficient queries and faster performance. Understanding how to use these functions effectively is essential for any SQL practitioner aiming to enhance query performance.
One of the primary benefits of SQL functions is their ability to process data directly within the database engine. This reduces the amount of data that needs to be sent across the network, as only the necessary results are returned. For example, if you need to calculate the total sales amount for each customer, a SQL function can handle the aggregation without transferring all individual sales records:
SELECT customer_id, SUM(sale_amount) AS total_sales FROM sales GROUP BY customer_id;
In this query, the database performs the summation and grouping operations internally, resulting in a more efficient data retrieval process.
Another powerful feature of SQL functions is their ability to transform data directly in the query. Functions such as UPPER, LOWER, and CONCAT can be used to manipulate string values, which can save time when formatting data for reporting or presentation:
SELECT customer_id, UPPER(customer_name) AS formatted_name FROM customers;
In this case, the UPPER function converts the customer names to uppercase before they’re sent to the application layer, creating a cleaner output directly from the database.
Additionally, using built-in SQL functions for date manipulation can simplify queries that involve time-based data. For instance, if you want to retrieve orders placed in the last month, you can use the DATEADD function to calculate the relevant date range:
SELECT * FROM orders WHERE order_date >= DATEADD(MONTH, -1, GETDATE());
This approach allows the database to handle the date calculation efficiently, reducing complexity and potential errors in the application logic.
Furthermore, user-defined functions (UDFs) can be created to encapsulate frequently used calculations or transformations. By defining a function to calculate discounts, for example, you can simplify your queries and ensure consistent application of the logic:
CREATE FUNCTION CalculateDiscount(@price DECIMAL(10, 2)) RETURNS DECIMAL(10, 2) AS BEGIN RETURN @price * 0.90; -- Apply a 10% discount END;
Once the function is defined, it can be used in your queries like this:
SELECT product_id, CalculateDiscount(price) AS discounted_price FROM products;
Using functions in this manner not only enhances query readability but also improves maintainability, as any changes to the discount logic need only be made in one place instead of across multiple queries.
However, it’s important to use SQL functions judiciously. Functions that perform operations on indexed columns in the WHERE clause can hinder performance by preventing the database from using indexes effectively. For example, avoid:
SELECT * FROM employees WHERE YEAR(hire_date) = 2020;
Instead, use a range condition that allows for index utilization:
SELECT * FROM employees WHERE hire_date >= '2020-01-01' AND hire_date < '2021-01-01';
Effectively using SQL functions can lead to significant performance improvements in data retrieval. By using built-in and user-defined functions, you can streamline your queries, reduce data transmission, and maintain efficient processing within the database engine. Ultimately, this approach can enhance application responsiveness and user satisfaction, making your SQL operations not just functional, but also highly efficient.
Caching and Temporary Tables for Efficiency
Caching and temporary tables are powerful tools for enhancing SQL query performance, particularly when dealing with large datasets or frequently accessed data. By minimizing the need to repeatedly access the disk for data retrieval, these techniques can significantly reduce response times and improve overall efficiency.
Caching refers to the process of storing frequently accessed data in memory, allowing the database to retrieve it quickly without hitting the disk every time a query is executed. Most modern SQL databases have built-in caching mechanisms that retain the results of previously executed queries. This can drastically reduce the time taken to fetch repeat results. For instance, if your application frequently queries customer details, caching those results means that subsequent requests for the same data can be served from memory:
SELECT * FROM customers WHERE customer_id = 123;
Once this query is executed, the database engine retains the result in cache. Thus, any future requests for the same customer data can be served from the cache, eliminating the need for disk access.
However, caching is not without its challenges. Stale data can be a concern, especially in environments where data is frequently updated. It’s crucial to implement cache invalidation strategies that ensure the cached data remains accurate and up-to-date. For example, when a customer record is updated, the corresponding cache entry should be invalidated to prevent serving outdated information:
UPDATE customers SET name = 'Jane Doe' WHERE customer_id = 123; -- Invalidate cache entry for this customer.
Temporary tables are another effective way to improve SQL performance, especially when dealing with complex queries or intermediate data results. Temporary tables allow you to store intermediate results that can be reused throughout the session. This is particularly useful when you need to perform multiple operations on the same dataset without recalculating or re-fetching it repeatedly.
For example, suppose you need to analyze sales data over several years. Instead of repeatedly querying the sales table for each year’s data, you can create a temporary table to store the relevant records:
CREATE TEMPORARY TABLE temp_sales AS SELECT * FROM sales WHERE order_date >= '2022-01-01';
Now, you can perform multiple operations on the `temp_sales` table without needing to access the `sales` table multiple times, thus improving performance. For instance, you can quickly calculate total sales for the year:
SELECT SUM(sale_amount) AS total_sales FROM temp_sales;
In addition to performance improvements, using temporary tables can also simplify complex queries. Instead of nesting subqueries, you can break down the logic into manageable parts by using temporary tables. This not only enhances readability but can also aid in debugging:
CREATE TEMPORARY TABLE temp_customer_orders AS SELECT customer_id, COUNT(*) AS order_count FROM orders GROUP BY customer_id;
After creating the temporary table, you can join it with other tables to retrieve additional insights, such as customer details:
SELECT c.name, t.order_count FROM customers c JOIN temp_customer_orders t ON c.id = t.customer_id;
While temporary tables and caching can offer significant performance advantages, it’s important to use them judiciously. Over-reliance on these techniques can lead to increased memory usage and complexity in managing data states. Regularly monitor the performance of your SQL queries and adjust your caching and temporary table strategies as necessary to ensure optimal efficiency.