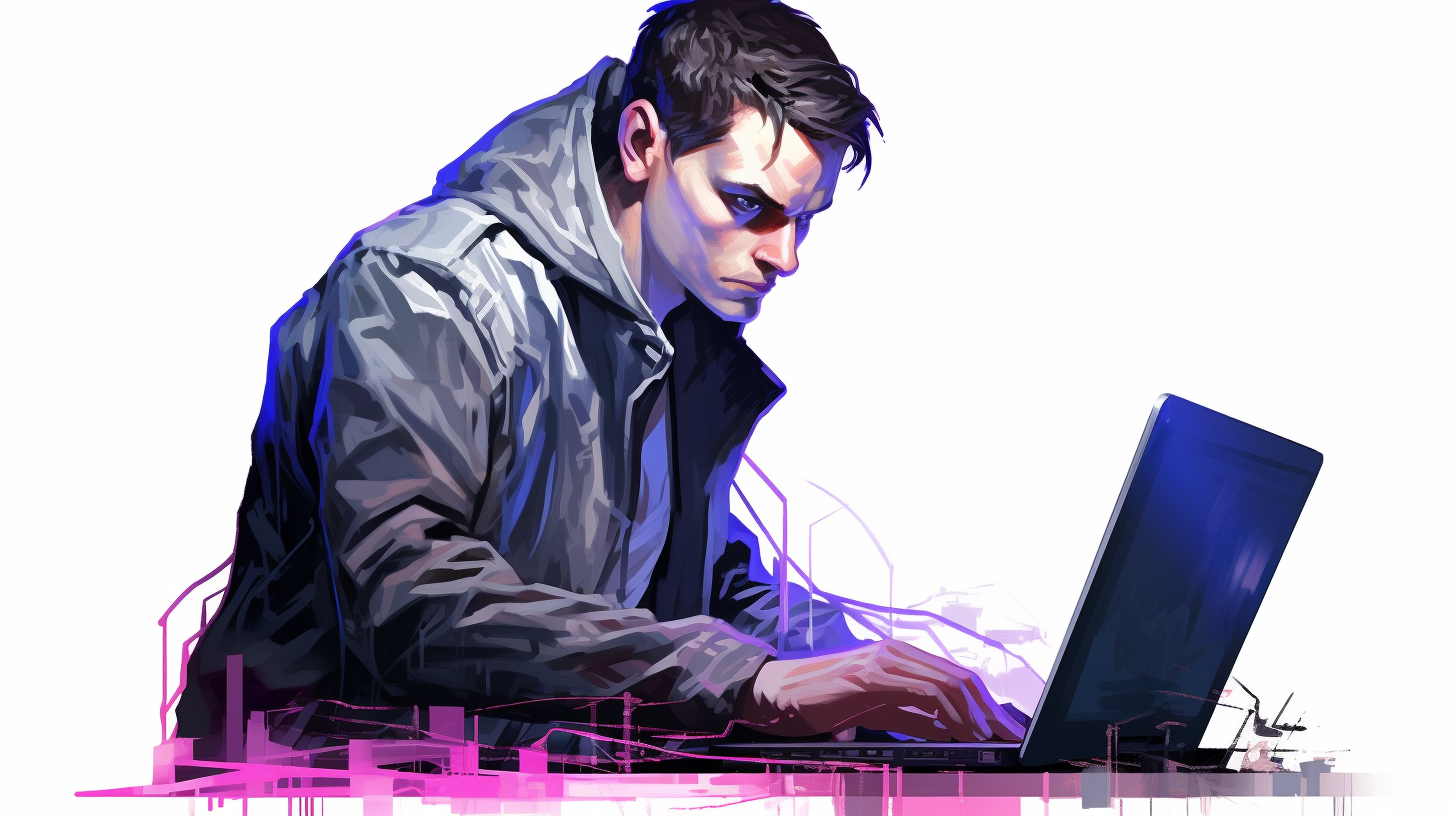
SQL Tricks for Data Anonymization
Data masking is a vital technique in the context of data anonymization, particularly when dealing with sensitive information. It involves altering data so that it is no longer identifiable while still preserving its usability for various applications. Here are some effective methods employed in data masking:
- This method involves creating a copy of the database with masked data. The original data is kept secure while users can work with the masked version.
- Unlike SDM, DDM alters data on-the-fly based on user roles, ensuring that sensitive information is hidden from unauthorized users in real time.
- This approach replaces sensitive data with unique identification symbols (tokens) that retain essential information without compromising security. The mapping between original data and tokens is securely stored in a separate database.
Implementing data masking can significantly enhance privacy without sacrificing the analytical capabilities of the data. Below is an example of how to implement static data masking using SQL:
UPDATE users SET email = CONCAT('user', id, '@example.com'), phone_number = '000-000-0000' WHERE role = 'employee';
In this SQL command, the users’ email addresses are modified to reflect a non-identifiable format, maintaining data anonymity while still allowing for user-related queries.
Another common approach is dynamic data masking. Here’s a simple SQL example to illustrate this:
SELECT user_id, username, CASE WHEN user_role = 'admin' THEN email ELSE '***@example.com' END AS email FROM users;
In the SQL query above, the email address is only displayed in full for users with an ‘admin’ role. All other users see a masked version, ensuring sensitive information is protected based on user permissions.
As organizations increasingly recognize the need for data privacy, using these effective techniques for data masking becomes paramount. By applying the right strategy, businesses can safeguard sensitive information while retaining the analytical utility of their data.
Using Hash Functions for Secure Anonymization
Hash functions play an important role in ensuring secure anonymization of data by transforming sensitive information into a fixed-size string of characters, which is typically represented in a hexadecimal format. This transformation is irreversible, meaning that the original data cannot be reconstructed from the hashed value. By using hash functions, organizations can effectively anonymize data while still allowing for its use in various applications, such as data analysis or reporting.
One of the most widely used hash functions is SHA-256, which produces a 256-bit hash value. This method is exceptionally strong in terms of security, making it suitable for protecting sensitive data like personal identification numbers (PINs), social security numbers, or credit card information. Below is an example of how to implement a hash function in SQL to anonymize user passwords:
UPDATE users SET password_hash = SHA2(password, 256) WHERE password IS NOT NULL;
In this SQL command, the passwords of users are hashed using the SHA2 function, which applies the SHA-256 algorithm. The original password is no longer stored in the database, thus enhancing security and ensuring that even if the database is compromised, the actual passwords cannot be retrieved.
Moreover, hash functions can be utilized to anonymize personally identifiable information (PII) such as email addresses. Here is an example where email addresses are hashed for privacy:
UPDATE users SET email_hash = SHA2(email, 256) WHERE email IS NOT NULL;
This SQL statement takes each user’s email address and generates a hash, which is then stored in a separate column, `email_hash`, in the database. This way, the actual email addresses remain protected and cannot be easily reverse-engineered.
While hashing provides a significant level of security, it’s essential to remember that hash functions can be vulnerable to attacks, especially if the data being hashed is predictable or common. To mitigate this risk, a technique known as salting can be implemented. Salting involves adding a unique random string to each piece of data before hashing it. This prevents attackers from using precomputed hash tables (rainbow tables) to crack the hashes.
Here’s how you can appropriately implement salting along with hashing in an SQL context:
UPDATE users SET password_hash = SHA2(CONCAT(salt, password), 256) WHERE password IS NOT NULL;
In this example, the `salt` column contains a unique random string for each user. By concatenating the salt with the password before hashing, we significantly increase the complexity of the hashed output, making it much harder for an attacker to predict or reverse-engineer the original password.
By using hash functions effectively, organizations can secure sensitive data and maintain compliance with data protection regulations while still enabling analytical capabilities. However, it especially important to regularly review and update security practices to counter evolving threats and vulnerabilities in the database landscape.
Dynamic Data Anonymization Strategies
Dynamic data anonymization takes a more nuanced approach to data protection compared to static methods. It allows organizations to implement data masking in real-time, catering to various user roles and their respective access rights. This means that sensitive information is concealed based on the context of the user’s request, making it an effective tool for enhancing data security without sacrificing usability.
One of the key advantages of dynamic data anonymization is its ability to provide different views of data depending on the user’s authorization level. This real-time capability not only safeguards sensitive information but also improves compliance with data protection regulations by ensuring that only authorized personnel can access certain data. Below is an illustration of how to implement dynamic data masking using SQL:
SELECT user_id, username, CASE WHEN user_role = 'manager' THEN email ELSE '[email protected]' END AS email FROM users;
In the example above, users in the ‘manager’ role are permitted to view the complete email addresses, while all other users receive a masked version. This allows businesses to control who sees sensitive information, thereby minimizing the risk of data breaches.
Furthermore, dynamic data masking can be complemented by using row-level security features offered by modern database systems. This provides even more granular control over data access. Here’s how you can implement row-level security alongside dynamic data masking:
CREATE SECURITY POLICY user_access_policy ADD FILTER PREDICATE user_access_fn(user_id) ON dbo.users WITH (STATE = ON);
In this example, a security policy is created that applies a filter to the `users` table based on the output of the `user_access_fn` function, which determines user access rights. By tying this policy to your dynamic masking strategy, you can ensure that data visibility is tightly controlled and aligned with business rules.
Another effective strategy is to use a combination of dynamic SQL and user-defined functions to handle complex business logic for data access. Here’s a SQL snippet to illustrate how a user-defined function can be applied:
CREATE FUNCTION dbo.get_email (@user_role VARCHAR(50), @email VARCHAR(255)) RETURNS VARCHAR(255) AS BEGIN RETURN CASE WHEN @user_role = 'admin' THEN @email ELSE '***@example.com' END; END;
With this function in place, you can call it within your select statements, allowing for a modular approach to data masking:
SELECT user_id, username, dbo.get_email(user_role, email) AS email FROM users;
This modular approach not only simplifies maintenance but also enhances readability and allows for easier updates to the masking logic as business requirements evolve.
It very important to remember that scaling dynamic data masking strategies requires careful planning and implementation. Organizations must continually assess their roles and permissions structure to ensure that it aligns with security policies and compliance mandates. By using dynamic data anonymization effectively, businesses can enhance their data security posture while still enabling meaningful data analysis and reporting.
Best Practices for Maintaining Data Integrity
Maintaining data integrity while implementing anonymization techniques is paramount for organizations that prioritize both security and usability. Data integrity ensures that the information retained remains accurate, consistent, and trustworthy throughout its lifecycle, even after undergoing various anonymization processes. Here are some best practices to maintain data integrity during data anonymization:
1. Establish Clear Data Governance Policies
Before implementing data anonymization strategies, organizations should develop comprehensive data governance policies that outline how data will be managed, accessed, and protected. This includes determining which data needs to be anonymized, establishing criteria for anonymization methods, and delineating user roles and access permissions. Clear documentation of these policies aids in maintaining data integrity.
2. Use Consistent Anonymization Techniques
Inconsistent application of anonymization techniques can lead to discrepancies in data quality. It is essential to use standardized methods across the organization for similar data types. For instance, if hashing is used to anonymize personal identifiable information (PII), all instances of that PII across the database should follow the same hashing algorithm and strategy. This consistency helps ensure that data remains reliable and valid.
3. Implement Audit Trails
Establishing audit trails is important for tracking changes made to data, including anonymization processes. By maintaining logs of modifications, organizations can review who accessed or altered data and when these changes occurred. SQL triggers can be utilized to log these activities. Here is an example of creating a simple audit trail for changes made to the `users` table:
CREATE TRIGGER users_audit_trigger AFTER UPDATE ON users FOR EACH ROW BEGIN INSERT INTO users_audit (user_id, old_email, new_email, change_time) VALUES (OLD.user_id, OLD.email, NEW.email, NOW()); END;
This trigger captures the old and new email addresses for each update, along with the timestamp, enhancing transparency and accountability in data management.
4. Regularly Validate Anonymized Data
It is important to regularly validate the anonymized data to ensure that it meets the intended criteria for usability and anonymity. Organizations can set up automated tests that run periodically to check data quality and the effectiveness of anonymization methods. This practice helps to identify any issues early in the process, allowing for prompt remediation.
5. Employ Data Reconciliation Techniques
Data reconciliation techniques can help ensure that the anonymized dataset still reflects the original dataset in terms of structure and relationships. This very important for maintaining analytical capabilities. One effective way to implement reconciliation is through checksum comparisons of original and anonymized datasets. Here’s an example:
SELECT MD5(CONCAT_WS(',', user_id, username, email)) AS original_checksum, MD5(CONCAT_WS(',', user_id, username, email_hash)) AS anonymized_checksum FROM users;
By comparing checksums, organizations can determine if the anonymized data has maintained its structural integrity, which is essential for accurate analytics.
6. Regular Training and Awareness
Human error is often a significant factor in data integrity issues. Regular training sessions for employees on data handling best practices, anonymization techniques, and security protocols can greatly contribute to maintaining data integrity. This reinforces the importance of data protection measures and encourages adherence to established policies.
By implementing these best practices, organizations can enhance their data integrity while pursuing effective data anonymization strategies. This ensures that while sensitive information is protected, the usability and reliability of the data are not compromised, allowing businesses to benefit from both security and operational efficiency.