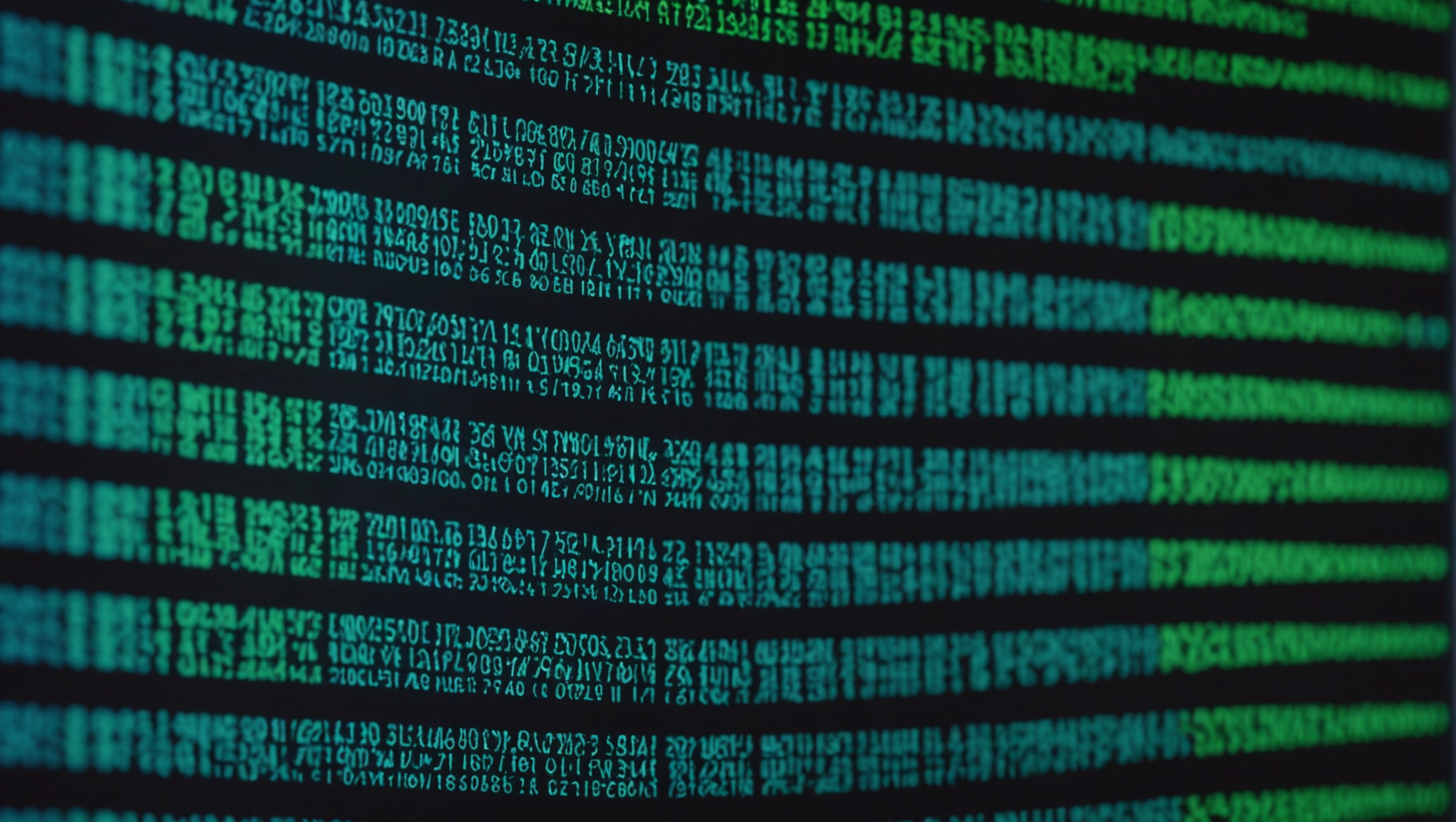
SQL Tricks for Data Conversion
Understanding SQL data types is fundamental for effective database management and data manipulation. SQL supports various data types, so that you can define the kind of data that can be stored in a column. The choice of data type significantly affects the performance and accuracy of your SQL queries and operations.
Here’s a breakdown of the primary SQL data types:
- Numeric Types:
- A standard integer data type.
- Used for floating-point numbers, providing approximate numeric values.
- Fixed-point type where
p
is the precision ands
is the scale.
- String Types:
- Fixed-length string of length
n
. - Variable-length string with a maximum length of
n
. - Used for large text or binary data.
- Fixed-length string of length
- Date and Time Types:
- Stores date values.
- Stores time values.
- Combines both date and time into a single value.
- Boolean Types:
- Represents true/false values.
When defining a data type for a column, it is critical to choose one that accurately represents the nature of the data to be stored. For instance, using VARCHAR
for names and DECIMAL
for monetary values ensures efficient data storage and retrieval.
Here’s a simple example of how to create a table with various data types:
CREATE TABLE Users ( UserID INT PRIMARY KEY, UserName VARCHAR(50), Email VARCHAR(100), Age INT, Balance DECIMAL(10, 2), CreatedAt DATETIME DEFAULT CURRENT_TIMESTAMP );
In this example, the Users
table is designed to hold various types of information about users, showcasing the application of different data types in a practical context. Optimizing query performance and ensuring data integrity are crucial reasons to be mindful of data types when designing your database schema.
Converting Between Numeric and String Types
Converting between numeric and string types in SQL is a common operation, essential for scenarios where data types do not match. Often, numeric data needs to be transformed into a string format for reporting or concatenation operations, while strings may need to be converted into numeric types for calculations or comparisons.
SQL provides several functions to facilitate these conversions, namely CAST and CONVERT. The CAST function is used to convert an expression from one data type to another, while CONVERT offers additional formatting options and is specific to certain SQL dialects, such as SQL Server.
Here’s how you can use these functions for converting between numeric and string types:
To convert a numeric value to a string, you can use CAST like this:
SELECT CAST(12345 AS VARCHAR(10)) AS StringValue;
This query will return the numeric value 12345 as a string. It’s important to specify the length of the VARCHAR to avoid truncation.
Similarly, the CONVERT function can be employed as follows:
SELECT CONVERT(VARCHAR(10), 12345) AS StringValue;
In both cases, you will get 12345 represented as a string.
On the other hand, converting a string to a numeric type can be equally simpler. For example:
SELECT CAST('123.45' AS DECIMAL(10, 2)) AS NumericValue;
This will convert the string ‘123.45’ to a numeric type with two decimal places, effectively allowing for mathematical operations to be performed on it.
Using CONVERT for the same operation would look like this:
SELECT CONVERT(DECIMAL(10, 2), '123.45') AS NumericValue;
It’s essential to handle potential conversion errors, particularly when converting strings to numeric types. If the string contains non-numeric characters, the conversion will fail. Implementing error handling and validation checks can prevent runtime exceptions, ensuring that your SQL queries execute smoothly.
For example, you could use a CASE statement to ensure only valid numeric strings are converted:
SELECT CASE WHEN ISNUMERIC(YourStringColumn) = 1 THEN CAST(YourStringColumn AS DECIMAL(10, 2)) ELSE NULL END AS ConvertedValue FROM YourTable;
This query checks if the string is numeric before attempting the conversion, returning NULL if it is not. This approach guards against conversion errors and maintains data integrity.
In summary, the ability to convert between numeric and string types is a powerful feature in SQL that enhances data manipulation capabilities. Understanding how to effectively utilize CAST and CONVERT functions will empower you to handle diverse data scenarios with ease.
Handling Date and Time Formats
Working with dates and times in SQL can often feel like navigating a labyrinth of formats and representations. Each database management system may have its own nuances when it comes to handling date and time data types. However, regardless of the system, there are some fundamental principles that can help you manage date and time formats efficiently.
SQL provides various data types for storing date and time information, including DATE, TIME, and DATETIME. Each of these data types serves a specific purpose, and understanding their differences is key to effective data management.
The DATE type stores just the date (year, month, day), while TIME captures only the time (hours, minutes, seconds). On the other hand, DATETIME combines both date and time into a single timestamp, making it versatile for many applications.
When inserting dates or times into your SQL tables, it’s essential to use the correct format. The standard format for SQL dates is 'YYYY-MM-DD'
, and for times, it’s 'HH:MM:SS'
. Here’s an example of how to insert a record with a date and time:
INSERT INTO Events (EventID, EventDate, EventTime) VALUES (1, '2023-10-01', '15:30:00');
Retrieving and formatting date and time data is another area where SQL shines. Functions like DATE_FORMAT in MySQL or FORMAT in SQL Server allow you to convert dates into human-readable formats. For example:
SELECT DATE_FORMAT(EventDate, '%W, %M %d, %Y') AS FormattedDate FROM Events;
This query will convert the EventDate into a more readable string format, such as “Sunday, October 01, 2023”. This capability especially important for reporting and display purposes.
When dealing with time zones, SQL offers functions like AT TIME ZONE in SQL Server, which allows you to convert a datetime value to a specified time zone. For instance:
SELECT EventDate AT TIME ZONE 'UTC' AS UTCDateTime FROM Events;
This command converts the EventDate to UTC time, ensuring that time zone differences are accounted for.
Another common operation is extracting specific components from date and time values. SQL provides functions such as YEAR, MONTH, and DAY to retrieve these components easily. For example:
SELECT YEAR(EventDate) AS EventYear, MONTH(EventDate) AS EventMonth, DAY(EventDate) AS EventDay FROM Events;
This query extracts and displays the year, month, and day from the EventDate field, which will allow you to analyze data across specific timeframes.
However, challenges can arise when working with date and time formats, particularly with differing regional settings or legacy data that may be stored in non-standard formats. In such cases, using functions like STR_TO_DATE in MySQL can help convert strings to date formats:
SELECT STR_TO_DATE('01-10-2023', '%d-%m-%Y') AS ConvertedDate;
This command converts a string formatted as ‘DD-MM-YYYY’ into a date value. Understanding and using these conversion functions is key to maintaining data integrity when dealing with various date formats.
Handling date and time formats in SQL requires a solid understanding of the various data types available and the functions that facilitate conversion and formatting. By mastering these elements, you can ensure accurate data representation and retrieval in your SQL databases.
Using CAST and CONVERT Functions
In SQL, the CAST and CONVERT functions are essential tools for converting data types, providing flexibility in managing data transformations. While both functions serve the purpose of changing one data type to another, they have distinct characteristics that cater to different scenarios. Understanding how to use these functions effectively can enhance your data manipulation capabilities.
The CAST function is standardized and widely supported across different SQL database systems. It takes two arguments: the expression you want to convert and the target data type. Here’s a basic syntax:
CAST(expression AS target_data_type)
For example, if you want to convert a numeric value to a string, you can use:
SELECT CAST(12345 AS VARCHAR(10)) AS StringValue;
This query returns the numeric value 12345 as a string. It’s crucial to specify the length for the target VARCHAR to prevent truncation issues.
The CONVERT function, on the other hand, is often specific to certain SQL dialects like SQL Server, offering additional formatting options, particularly for date and time conversions. Here’s the basic syntax of CONVERT:
CONVERT(target_data_type, expression[, style])
To convert a string to a date with a specific format, you might use:
SELECT CONVERT(DATETIME, '2023-10-01 15:30:00', 120) AS EventDate;
In this case, the third argument specifies the style of the date format, allowing for greater control over how the conversion is executed.
Both functions can also handle conversions from strings to numeric types, but developers must take care to handle potential errors that may arise from invalid data. For instance, converting a string that contains non-numeric characters will lead to conversion failures. To mitigate this, you can use validation functions in combination with CAST or CONVERT.
For example, using a CASE statement with ISNUMERIC helps ensure only valid strings are converted:
SELECT CASE WHEN ISNUMERIC(YourStringColumn) = 1 THEN CAST(YourStringColumn AS DECIMAL(10, 2)) ELSE NULL END AS ConvertedValue FROM YourTable;
This approach safeguards against errors, returning NULL for any non-numeric strings while successfully converting valid ones.
When dealing with date and time data, the CONVERT function shines, particularly in SQL Server. For example, if you have a datetime field and want to extract only the date portion, you might use:
SELECT CONVERT(DATE, YourDateTimeColumn) AS JustDate FROM YourTable;
This command provides a simpler way to isolate the date from a datetime value, which can be particularly useful for grouping and filtering operations.
In addition to error handling, understanding the performance implications of data type conversions is vital. Frequent or unnecessary conversions can lead to performance bottlenecks in your SQL queries. Therefore, it’s advisable to design your database schema with optimal data types from the outset, minimizing the need for extensive conversions later in your data processing workflows.
Using the CAST and CONVERT functions properly can greatly enhance your SQL proficiency, allowing you to handle diverse data types with confidence and precision. Mastering these functions will empower you to tackle data conversion challenges effectively, ensuring seamless data management in your SQL environments.
Best Practices for Data Conversion
When it comes to data conversion in SQL, adopting best practices ensures that your processes are efficient, error-free, and maintain integrity in your database operations. Here are several strategies to consider:
1. Choose the Right Data Types: Start by defining your database schema with the most appropriate data types from the outset. This reduces the need for conversions later on. For instance, if a column is intended to hold whole numbers, use INT
instead of FLOAT
or DECIMAL
. This not only improves performance but also minimizes potential conversion errors.
2. Validate Data Before Conversion: Always validate your data before performing conversions. Implement checks to ensure that the data you intend to convert is in the correct format and compatible with the target data type. You can use the TRY_CAST
or TRY_CONVERT
functions in SQL Server to safely attempt a conversion without throwing an error if it fails:
SELECT TRY_CAST(YourStringColumn AS INT) AS SafeIntValue FROM YourTable;
This approach will return NULL
for any row that fails the conversion, protecting your queries from abrupt failures.
3. Leverage Built-in Functions: Utilize SQL’s built-in functions for conversions whenever possible. These functions are optimized for performance and are less prone to errors than custom conversion logic. For example, the FORMAT
function can be used to convert date and time types into a specific format:
SELECT FORMAT(YourDateColumn, 'yyyy-MM-dd') AS FormattedDate FROM YourTable;
4. Handle Nulls Gracefully: Be mindful of how conversions interact with NULL
values. Converting NULL
should not throw errors, but it’s good practice to explicitly handle NULL
cases in your queries. For instance:
SELECT COALESCE(CAST(YourStringColumn AS DECIMAL(10, 2)), 0) AS NumericValue FROM YourTable;
This query will return 0
for any NULL
values instead of failing the conversion.
5. Optimize Performance: Frequent or unnecessary data type conversions can degrade performance, particularly in large datasets. To mitigate this, analyze your queries to identify any potential bottlenecks caused by conversions and think caching results where feasible. Try to avoid in-query conversions in SELECT statements when possible by storing data in its intended format.
6. Document Your Conversions: Maintain clear documentation of any data type conversions performed in your SQL processes. That is particularly important for complex systems where multiple developers may interact with the database. Well-documented conversion logic helps ensure that everyone working with the database understands the data flow and transformations.
7. Thoroughly Test Your Queries: After implementing conversions, it’s critical to conduct thorough testing. Create test cases that cover edge scenarios, such as unexpected data formats or NULL
values, to validate the robustness of your conversions. Automation can aid in regression testing, ensuring that your data conversion processes remain intact as your database evolves.
By following these best practices for data conversion in SQL, you can enhance the reliability, performance, and maintainability of your database systems, leading to a smoother experience in managing and manipulating your data.