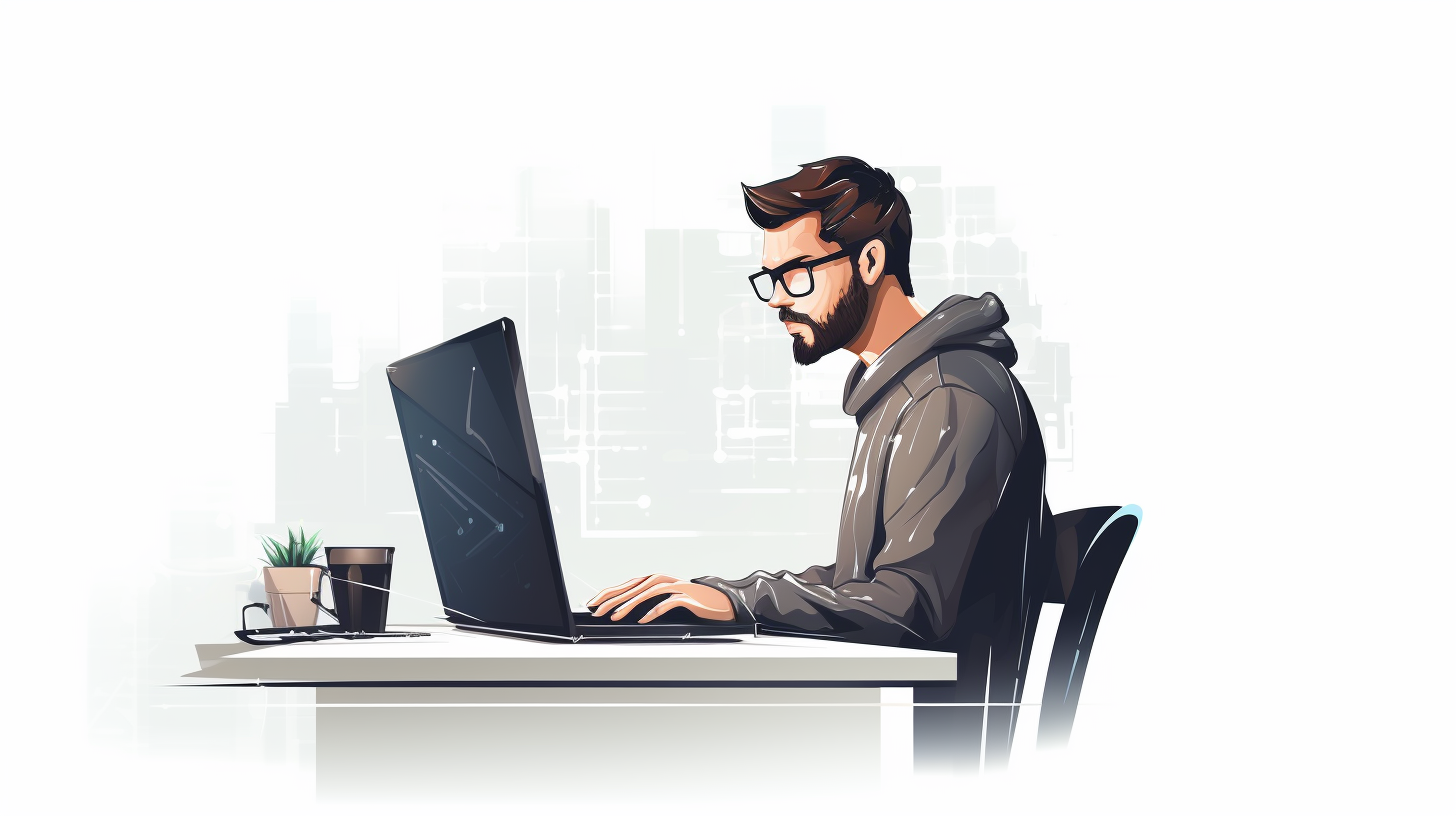
String Manipulation in Python
In Python, strings are one of the most commonly used data types, serving as the foundation for handling text-based data. A string in Python is essentially a sequence of characters, encapsulated within either single quotes ('
) or double quotes ("
). This flexibility allows developers to choose the style that best fits their coding preferences or project needs.
Immutable Nature of Strings
It’s important to note that strings in Python are immutable. This means that once a string is created, it cannot be altered. Any operation that seems to change the string actually results in a new string being created. This property is essential to understand, as it affects both performance and memory usage in your applications.
For example, consider the following code snippet:
original_string = "Hello, World!" modified_string = original_string.replace("World", "Python") print(original_string) # Output: Hello, World! print(modified_string) # Output: Hello, Python!
In the example above, the replace
method does not modify the original_string
; instead, it returns a new string with the specified modifications.
String Representation
Strings in Python are designed to handle various character encodings. The default encoding is UTF-8, which allows for a wide range of characters from different languages and symbol sets. You can also work with raw strings by prefixing them with an r
. That is particularly useful when dealing with regular expressions or file paths, where backslashes are common.
raw_string = r"C:UsersNameDocuments" print(raw_string) # Output: C:UsersNameDocuments
String Concatenation and Repetition
Another key aspect of string manipulation in Python is concatenation and repetition. You can concatenate strings using the +
operator and repeat them using the *
operator. Here’s how it looks:
greeting = "Hello" name = "Alice" # Concatenation full_greeting = greeting + ", " + name + "!" print(full_greeting) # Output: Hello, Alice! # Repetition laugh = "ha" * 3 print(laugh) # Output: hahaha
In the realms of string manipulation, understanding these fundamental characteristics and operations is important for efficient coding in Python. Strings form the backbone of text handling, and mastering their data types and behaviors will vastly enhance your programming capabilities.
Common String Methods and Functions
When working with strings in Python, a plethora of built-in methods are at your disposal, making it easier to manipulate and transform string data. These methods provide a convenient way to perform common operations without the need for complex algorithms. Below, we will explore some of the most useful string methods and functions available in Python.
1. String Length: len()
The len()
function allows you to determine the number of characters in a string, including spaces and punctuation. This is often a useful first step when processing string data.
my_string = "Hello, Python!" length = len(my_string) print(length) # Output: 15
2. Finding Substrings: find() and index()
To locate the position of a substring within a string, you can use the find()
and index()
methods. While find()
returns -1 if the substring is not found, index()
raises a ValueError, making it essential to handle exceptions when using it.
sentence = "The quick brown fox jumps over the lazy dog" position = sentence.find("fox") print(position) # Output: 16 # Using index() position_index = sentence.index("fox") print(position_index) # Output: 16 # Uncommenting the following line will raise a ValueError # position_error = sentence.index("cat")
3. String Slicing
Slicing is a powerful feature in Python that allows you to extract a portion of a string. You can specify a start index and an end index, making it easy to grab substrings.
text = "Hello, World!" substring = text[7:12] # Slicing from index 7 to 11 print(substring) # Output: World
4. Changing Case: upper(), lower(), title()
Python provides several methods for altering the case of strings. The upper()
method converts all characters to uppercase, while lower()
does the opposite. The title()
method capitalizes the first letter of each word.
phrase = "hello, python!" print(phrase.upper()) # Output: HELLO, PYTHON! print(phrase.lower()) # Output: hello, python! print(phrase.title()) # Output: Hello, Python!
5. Stripping Whitespace: strip(), lstrip(), rstrip()
When working with user input or data read from files, it’s common to encounter unwanted whitespace. The strip()
method removes whitespace from both ends of a string, while lstrip()
and rstrip()
remove whitespace from the left and right ends, respectively.
dirty_string = " Hello, Python! " cleaned_string = dirty_string.strip() print(cleaned_string) # Output: Hello, Python!
6. Joining Strings
To combine elements of a list into a single string, the join()
method is extremely useful. It takes an iterable and concatenates its elements, using the string on which join()
was called as the delimiter.
words = ["Hello", "Python", "World"] sentence = " ".join(words) print(sentence) # Output: Hello Python World
7. Replacing Substrings: replace()
The replace()
method is handy for substituting occurrences of a substring with another string. As mentioned earlier, this operation does not modify the original string but returns a new one.
original = "Goodbye, World!" new_string = original.replace("Goodbye", "Hello") print(new_string) # Output: Hello, World!
These string methods and functions provide a robust toolkit for performing string manipulation in Python. By using these capabilities, you can handle text data with precision and efficiency, allowing for cleaner and more maintainable code.
String Formatting Techniques
String formatting techniques in Python play a pivotal role in how we present and manipulate strings, making it easier to construct dynamic text outputs. There are several ways to format strings, each offering unique benefits and varying levels of complexity. Let’s delve deeper into the most common methods of string formatting available in Python.
1. The % Operator
The oldest method of string formatting involves using the % operator. This method is reminiscent of C-style string formatting and allows you to embed values in a string using placeholders. The syntax is straightforward: use %s for strings, %d for integers, %f for floating-point numbers, and so forth.
name = "Alice" age = 30 formatted_string = "Hello, %s. You're %d years old." % (name, age) print(formatted_string) # Output: Hello, Alice. You are 30 years old.
2. str.format() Method
The str.format() method was introduced in Python 2.7 and provides a more powerful way to format strings. It uses curly braces {} as placeholders that can be filled by passing values to the format() method. This method allows for reordering and formatting of values using various format specifiers.
name = "Alice" age = 30 formatted_string = "Hello, {}. You are {} years old.".format(name, age) print(formatted_string) # Output: Hello, Alice. You're 30 years old. # Using positional and keyword arguments formatted_string_with_args = "Hello, {0}. You're {1} years old. {0}, welcome!".format(name, age) print(formatted_string_with_args) # Output: Hello, Alice. You're 30 years old. Alice, welcome!
3. f-Strings (Formatted String Literals)
Introduced in Python 3.6, f-strings have quickly become the preferred method for string formatting due to their readability and efficiency. By prefixing a string with the letter ‘f’, you can directly include variables in the string using curly braces, which significantly simplifies the formatting process.
name = "Alice" age = 30 formatted_string = f"Hello, {name}. You're {age} years old." print(formatted_string) # Output: Hello, Alice. You are 30 years old.
f-strings also support expressions, allowing for inline calculations or transformations.
length = 5 width = 10 area_string = f"The area of the rectangle is {length * width} square units." print(area_string) # Output: The area of the rectangle is 50 square units.
4. Old-Style vs New-Style Formatting
While the % operator represents the old-style formatting, methods such as str.format() and f-strings represent the new style. It’s generally recommended to use the newer methods for their enhanced functionality and readability. However, understanding the older methods can still be useful, especially when working with legacy code.
5. Formatting Numbers
String formatting techniques in Python also allow for sophisticated formatting of numeric values. You can control the number of decimal places and include thousands separators using format specifiers.
pi = 3.14159 formatted_pi = f"{pi:.2f}" # Formatting to 2 decimal places print(formatted_pi) # Output: 3.14 large_number = 1000000 formatted_number = f"{large_number:,}" # Adding a comma as a thousands separator print(formatted_number) # Output: 1,000,000
These string formatting techniques in Python not only facilitate the creation of uncomplicated to manage output but also enhance the clarity of your code. By mastering these methods, you can produce dynamic strings that adapt to the data they represent, making your applications more effective and engaging.
Working with Multi-line Strings
When it comes to handling multi-line strings in Python, there are a few techniques that allow you to maintain readability and structure in your code. Multi-line strings can be useful for representing large blocks of text, such as documentation, SQL queries, or any other long text that spans across several lines.
In Python, you can create multi-line strings using triple quotes, either with three single quotes (”’) or three double quotes (“””). This allows you to include line breaks and indentation without needing to concatenate multiple strings or use escape characters.
multi_line_string = """This is a multi-line string. It spans several lines, and retains the formatting exactly as it's written.""" print(multi_line_string) # Output: # That is a multi-line string. # It spans several lines, # and retains the formatting exactly as it is written.
The beauty of using triple quotes is that the string retains the formatting and line breaks exactly as you type them, which can be particularly beneficial for readability. That’s especially handy when you want to include structured text.
Sometimes, however, you may want to remove the leading whitespace from each line in a multi-line string. The textwrap module provides a convenient way to do this. By using the textwrap.dedent function, you can neatly strip the common leading whitespace from all lines, making the string easier to work with.
import textwrap multi_line_string_with_indentation = """ That is a multi-line string. Notice how the leading whitespace is consistent across all lines. """ dedented_string = textwrap.dedent(multi_line_string_with_indentation) print(dedented_string) # Output: # That's a multi-line string. # Notice how the leading whitespace # is consistent across all lines.
The backslash () is used at the beginning of the string to avoid the initial newline that would otherwise occur. This technique helps maintain clean and professional code while ensuring the output is well-structured.
Another useful feature when working with multi-line strings is the ability to format them dynamically. This can be achieved with the use of f-strings or the str.format() method, so that you can insert variables directly into your string.
name = "Alice" age = 30 formatted_multi_line_string = f""" Hello, {name}. You are {age} years old. Welcome to the world of Python!""" print(formatted_multi_line_string) # Output: # Hello, Alice. # You are 30 years old. # Welcome to the world of Python!
In this example, the f-string allows you to embed the variables directly within the multi-line structure, rendering it effortless to create dynamic content while preserving the readability of your code.
Working with multi-line strings in Python can greatly enhance the clarity of your programs. Whether you are creating long-form texts, stripping unnecessary whitespace, or constructing dynamic messages, these techniques ensure that your string manipulation remains efficient and maintainable.
Performance Considerations in String Manipulation
When it comes to performance considerations in string manipulation in Python, understanding the implications of immutability and the costs associated with various operations is important. Due to strings being immutable, every modification results in the creation of a new string object, which can lead to increased memory usage and slower performance, particularly in scenarios involving heavy string operations.
Memory Overhead
Each time you modify a string, Python allocates new memory for the new string and deallocates the old one after its reference count drops to zero. This behavior can lead to significant memory overhead if you’re repeatedly altering strings in loops or large-scale applications. For example:
original_string = "Hello" for i in range(1000): original_string += " World"
This code snippet repeatedly concatenates ” World” to `original_string`, leading to the creation of many intermediate string objects. Each concatenation results in copying the entire existing string to a new allocation, which becomes increasingly inefficient as the length of `original_string` grows.
Using Join for Efficient Concatenation
A more efficient approach for concatenating multiple strings is to use the `join()` method. This method creates a single new string in one go, minimizing overhead as it allocates memory only once. Here’s an example:
words = ["Hello"] + [" World"] * 1000 result = " ".join(words)
In this case, `join()` collects the strings into a list first, avoiding the repeated allocation and copying incurred in the earlier example. This results in a performance boost, especially when combining a large number of strings.
String Interpolation and Performance
When formatting strings, f-strings (introduced in Python 3.6) not only provide syntactic clarity but also generally offer better performance than the older % formatting or `str.format()` methods. This performance advantage is especially noticeable in cases involving a large number of variables or frequent string formatting operations:
name = "Alice" age = 30 formatted_string = f"Hello, {name}. You're {age} years old."
In performance tests, f-strings typically outperform the alternatives, making them the preferred choice for string formatting in performance-sensitive applications.
Profiling and Optimization
For developers serious about string manipulation performance, profiling your code can provide insight into potential bottlenecks. Tools such as `cProfile` can help identify sections of code that may need optimization. In addition, ponder replacing heavy string operations with more efficient data structures when necessary. For instance, if you need to build a string incrementally based on conditional logic, using a list to gather parts and joining them at the end can offer significant performance gains.
parts = [] for i in range(1000): parts.append("World") result = "Hello " + " ".join(parts)
This method is not only faster but also more memory-efficient than repeated concatenations.
When working with strings in Python, being mindful of the costs associated with immutability, using efficient methods like `join()`, and profiling your code can lead to performance improvements. As with many aspects of programming, understanding the underlying mechanics allows for more informed and optimized coding practices.