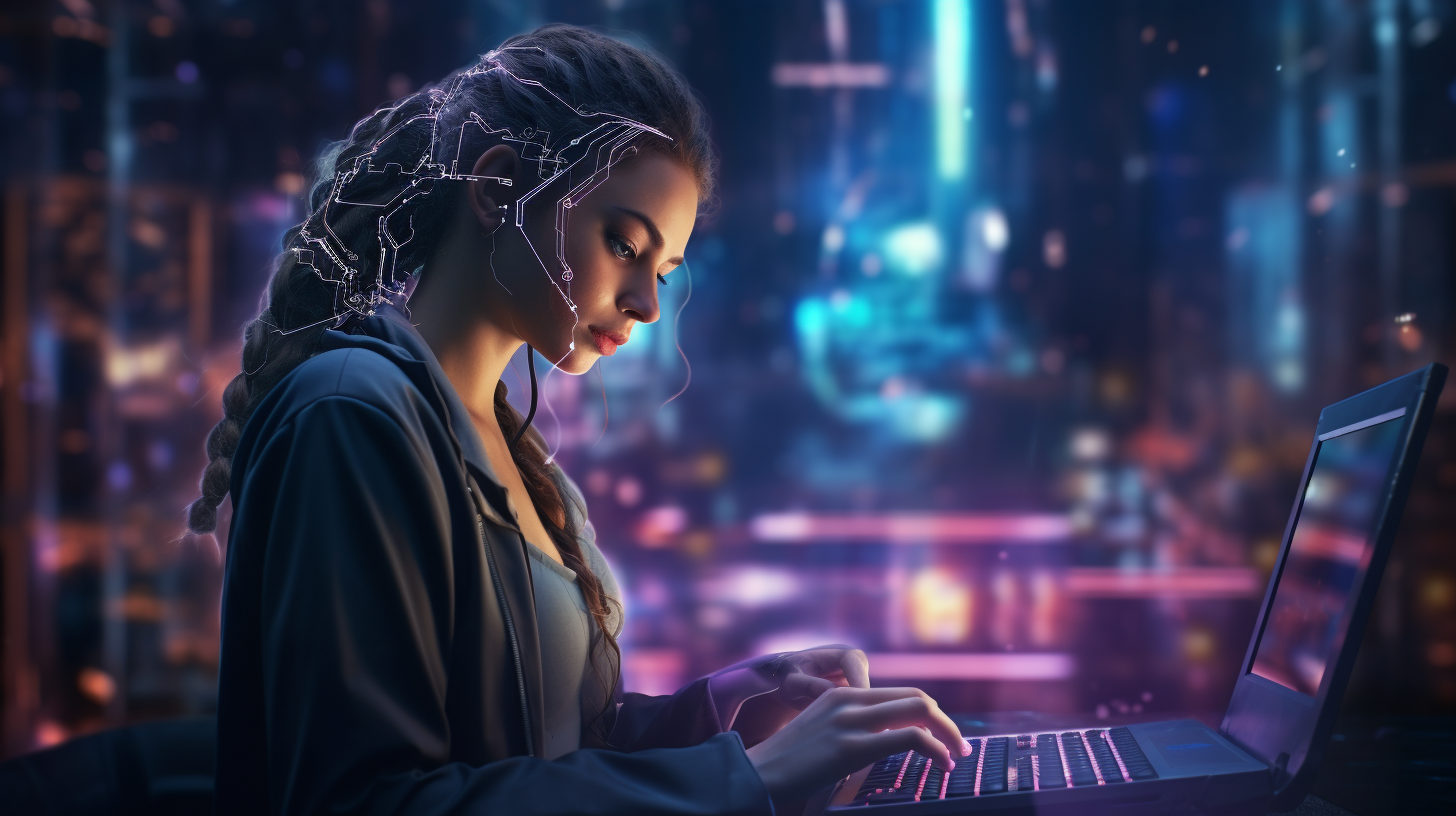
Swift and CallKit
The CallKit framework is an essential component for developers looking to integrate VoIP (Voice over Internet Protocol) services into their iOS applications. At its core, CallKit provides a consistent interface for users to manage and interact with incoming and outgoing calls, similar to the native phone experience. This seamless integration enhances user experience by using system-level features such as call blocking, identification, and interaction with other call-based apps.
CallKit operates using two primary concepts: the provider and the call. The provider is responsible for handling the call-related logic, while the call represents the state and details of a single call. This architecture allows you to create rich call experiences without needing to reinvent the wheel. Moreover, CallKit manages the audio session lifecycle, enabling audio routing, and optimizes for background execution to ensure calls remain active even when your app is not in the foreground.
One of the standout features of CallKit is its ability to present incoming calls using the native call interface. This means that when a call comes in, users will see a familiar interface that resembles the native phone app, complete with options to answer or decline the call. This level of integration not only improves usability but also builds trust with users, as they can rely on a consistent experience across different applications.
Furthermore, CallKit supports both audio and video calls, giving developers the flexibility to build a variety of communication applications. With the underlying support for the VoIP background mode, your application can receive calls even when it’s not actively running, enhancing the reliability of real-time communication.
Implementing CallKit requires a solid understanding of its components and structure. Developers must manage call actions, handle updates in call state, and communicate these changes to the system. The framework also provides capabilities for reporting call updates to users and the system, thereby ensuring the information reflected in the UI is accurate and timely.
To illustrate the setup of a basic CallKit provider, consider the following Swift code snippet that initializes a call provider:
import CallKit class CallManager: NSObject, CXProviderDelegate { private let provider: CXProvider override init() { let providerConfiguration = CXProviderConfiguration(localizedName: "My VoIP App") providerConfiguration.supportsVideo = true providerConfiguration.maximumCallGroups = 1 providerConfiguration.maximumCallsPerCallGroup = 1 self.provider = CXProvider(configuration: providerConfiguration) super.init() self.provider.setDelegate(self, queue: nil) } func provider(_ provider: CXProvider, perform action: CXStartCallAction) { // Handle the start call action action.fulfill() } func provider(_ provider: CXProvider, perform action: CXEndCallAction) { // Handle the end call action action.fulfill() } }
In this example, a CallManager
class is created, which conforms to the CXProviderDelegate
protocol. The provider is configured with essential properties such as support for video and limitations on call groups. By implementing the delegate methods, you can handle call start and end actions, ensuring that your app responds appropriately to user interactions.
Understanding the intricacies of CallKit allows developers to build powerful communication applications that take advantage of iOS’s built-in capabilities, providing a rich and engaging user experience. As you delve deeper into the framework, you’ll discover its potential to simplify call management while maintaining a high level of performance and reliability.
Setting Up CallKit in Your Swift Application
Setting up CallKit in your Swift application is an important step towards creating a seamless VoIP experience. To begin, ensure that your project has the appropriate entitlements and permissions configured in your app’s Info.plist file. You’ll need to declare the use of audio and potentially video capabilities, which are essential for any VoIP application.
In addition to the basic setup in your Info.plist, you need to initialize a CXProvider, which acts as an intermediary between your application logic and the system’s call interface. This provider is responsible for managing the lifecycle of calls and reporting updates to the system. Below is an example of how to set up a basic CXProvider:
import CallKit class CallManager: NSObject, CXProviderDelegate { private let provider: CXProvider override init() { let providerConfiguration = CXProviderConfiguration(localizedName: "My VoIP App") providerConfiguration.supportsVideo = true providerConfiguration.maximumCallGroups = 1 providerConfiguration.maximumCallsPerCallGroup = 1 providerConfiguration.supportedHandleTypes = [.phoneNumber] self.provider = CXProvider(configuration: providerConfiguration) super.init() self.provider.setDelegate(self, queue: nil) } func provider(_ provider: CXProvider, perform action: CXStartCallAction) { // Handle the start call action action.fulfill() } func provider(_ provider: CXProvider, perform action: CXEndCallAction) { // Handle the end call action action.fulfill() } }
This code sets up a simple call manager that prepares the provider configuration, indicating support for video and specifying call limitations. The delegate methods handle the actions for starting and ending calls, fulfilling these actions to indicate they have been processed.
Next, you need to manage the call actions using the CXCallController, which allows you to request call actions, such as starting or ending a call. Here’s how to integrate it into your application:
class CallManager: NSObject, CXProviderDelegate { private let provider: CXProvider private let callController: CXCallController override init() { let providerConfiguration = CXProviderConfiguration(localizedName: "My VoIP App") // ... (same configuration as before) self.provider = CXProvider(configuration: providerConfiguration) self.callController = CXCallController() // Initializing the call controller super.init() self.provider.setDelegate(self, queue: nil) } func startCall(uuid: UUID, handle: CXHandle) { let startCallAction = CXStartCallAction(call: uuid, handle: handle) let transaction = CXTransaction(action: startCallAction) callController.request(transaction) { error in if let error = error { print("Error requesting transaction: (error)") } else { self.provider.reportOutgoingCall(with: uuid, startedAt: Date()) } } } func endCall(uuid: UUID) { let endCallAction = CXEndCallAction(call: uuid) let transaction = CXTransaction(action: endCallAction) callController.request(transaction) { error in if let error = error { print("Error requesting transaction: (error)") } else { self.provider.reportCall(with: uuid, endedAt: Date(), reason: .remoteEnded) } } } }
In the `startCall` function, we create a new `CXStartCallAction`, encapsulate it in a `CXTransaction`, and then request that transaction through the `callController`. Upon success, we report the outgoing call to the provider. Similarly, the `endCall` function initiates the ending of a call and reports the end event to the system.
Once you have the provider and call controller set up, you can begin handling incoming calls, managing call states, and updating the user interface accordingly. This setup provides a solid foundation for building a fully functional VoIP application using CallKit, which will allow you to focus on delivering a high-quality user experience.
Implementing Call Handling in CallKit
Implementing call handling in CallKit is a critical component that ensures your VoIP application can interact effectively with the system’s telephony features. When users initiate or receive calls, the application must seamlessly manage the call states and respond to user actions. The core of this interaction revolves around the use of the CXProvider and CXCallController, which work together to facilitate call operations.
To manage calls, you first need to set up your CXProvider delegate methods to handle various call actions. These delegate methods, such as perform action
, are invoked by the system in response to user interactions, like starting or ending a call. Here’s how you can structure these delegate methods:
class CallManager: NSObject, CXProviderDelegate { // ... previous properties and initialization func provider(_ provider: CXProvider, perform action: CXStartCallAction) { // Start the call on the VoIP service startVoIPCall(action.callUUID) action.fulfill() } func provider(_ provider: CXProvider, perform action: CXEndCallAction) { // End the call on the VoIP service endVoIPCall(action.callUUID) action.fulfill() } }
In the above code, when a call starts, you initiate the call on your VoIP service and fulfill the action to inform CallKit that the action has been processed. Similarly, when a call ends, you handle the termination of the call.
For a complete implementation, you’ll want to manage the active calls. This can be achieved using a data structure to keep track of the calls currently in progress. A common approach is to use a dictionary or an array to store the UUIDs of active calls:
private var ongoingCalls: [UUID: CXCall] = [:] func startVoIPCall(_ uuid: UUID) { // Create and manage the VoIP call logic here let call = CXCall(uuid: uuid) ongoingCalls[uuid] = call // Additional logic to connect to the VoIP server can be added here } func endVoIPCall(_ uuid: UUID) { // Logic to end the VoIP call ongoingCalls.removeValue(forKey: uuid) // Additional logic to disconnect from the VoIP server can be added here }
Implementing call handling also involves notifying the system about call updates. CallKit provides methods to report incoming, outgoing, and ended calls, enabling you to keep the system UI in sync with your app’s state. For instance, when a call is incoming, you can report it as follows:
func reportIncomingCall(uuid: UUID, handle: String) { let callUpdate = CXCallUpdate() callUpdate.remoteHandle = CXHandle(type: .phoneNumber, value: handle) callUpdate.hasVideo = false provider.reportNewIncomingCall(with: uuid, update: callUpdate) { error in if let error = error { print("Failed to report incoming call: (error)") } else { print("Incoming call reported successfully.") } } }
This function creates a CXCallUpdate
instance to describe the incoming call and then reports it to the provider. The system will display the call interface to the user, allowing them to answer or decline the call.
Moreover, handling call states such as connected, disconnected, or on hold especially important for providing the user with accurate and timely information. You can update the UI and call states using the appropriate methods provided by CallKit:
func reportCallConnected(uuid: UUID) { // Update call state as connected provider.reportCall(with: uuid, connectedAt: Date()) } func reportCallEnded(uuid: UUID) { // Update call state as ended provider.reportCall(with: uuid, endedAt: Date(), reason: .remoteEnded) }
Implementing call handling in CallKit involves creating a robust structure to manage calls, responding to user actions through delegate methods, and keeping the system informed of call states. This allows your application to provide a cohesive and interactive experience that mirrors the native iOS calling capabilities, ultimately enhancing user satisfaction and engagement.
Integrating VoIP Services with CallKit
Integrating VoIP services with CallKit is where the magic happens, enabling developers to leverage iOS’s powerful telephony features for their applications. When integrating a VoIP solution, it’s critical to ensure that your app can communicate effectively with the CallKit framework. This involves creating a seamless bridge between your VoIP backend and the CallKit provider to handle call setups, updates, and terminations.
First and foremost, you’ll want to establish a connection to your VoIP service. This typically includes initializing the service and ensuring that your app can send and receive call signals. Once your service is operational, you can begin to implement logic that bridges CallKit with your VoIP backend.
class VoIPService { func startCall(to contact: String) { // Logic to start a call with the VoIP backend print("Initiating call to (contact) on VoIP service") } func endCall() { // Logic to end the call with the VoIP backend print("Ending the call on VoIP service") } }
In your CallManager class, you can now utilize this VoIPService to manage call lifecycle events. For instance, when a user initiates a call through your app, you should invoke the startCall method of your VoIP service within the appropriate delegate method:
func provider(_ provider: CXProvider, perform action: CXStartCallAction) { let contactHandle = action.handle.value // Assuming the handle is a phone number voipService.startCall(to: contactHandle) action.fulfill() }
This approach encapsulates the call initiation logic within the VoIPService class, allowing your CallManager to focus solely on CallKit interactions. It also makes the code more modular, which is beneficial for maintenance and scalability.
When receiving an incoming call, you similarly need to ensure that your VoIP service is capable of handling call signaling. This typically involves listening for call events from your VoIP backend and then reporting these to CallKit appropriately. For example, when a call comes in, your VoIP service should notify the CallManager:
func incomingCallReceived(from contact: String) { let uuid = UUID() // Generate a unique UUID for the incoming call reportIncomingCall(uuid: uuid, handle: contact) }
In this scenario, the incomingCallReceived method would be invoked based on an event from your VoIP service, triggering the reporting of the incoming call to CallKit:
func reportIncomingCall(uuid: UUID, handle: String) { let callUpdate = CXCallUpdate() callUpdate.remoteHandle = CXHandle(type: .phoneNumber, value: handle) callUpdate.hasVideo = false provider.reportNewIncomingCall(with: uuid, update: callUpdate) { error in if let error = error { print("Failed to report incoming call: (error)") } else { print("Incoming call reported successfully.") } } }
It’s also essential to keep your VoIP service in sync with the CallKit state. For instance, when a call is answered or terminated, you should ensure that your VoIP service reflects this state change. To do this, you can call the endCall method of your VoIP service from within the appropriate delegate methods:
func provider(_ provider: CXProvider, perform action: CXEndCallAction) { voipService.endCall() action.fulfill() }
This integration allows your VoIP application to respond to user actions and system events efficiently. The connection between CallKit and your VoIP service should be robust, allowing for real-time call management that can adapt to network conditions and user interactions.
Integrating VoIP services with CallKit requires careful consideration of the call lifecycle and signaling. By modularizing your VoIP logic into a dedicated service and using CallKit’s capabilities effectively, you can deliver a high-quality user experience that feels native to iOS. This not only enhances the reliability of your communication application but also fosters user trust through a consistent and seamless interface.
User Interface Design for CallKit
User Interface Design for CallKit is a pivotal aspect that contributes significantly to the overall user experience of your VoIP application. Given that CallKit presents calls using the native iOS phone interface, it is crucial to align your app’s UI with user expectations while ensuring that it complements the functionalities provided by CallKit. A well-designed interface will not only make your application more intuitive but will also enhance user engagement and satisfaction.
When designing your user interface for a CallKit-enabled application, you should consider the following elements:
1. Call Screens: The call screen should provide essential information about the ongoing call, including the caller’s name or number, call duration, and call control buttons (mute, hold, end call). Using system-provided UI components can ensure consistency with the native phone app. For instance, you can create a call view controller that displays this information clearly:
import UIKit class CallViewController: UIViewController { var callerName: String? var callDurationLabel: UILabel! override func viewDidLoad() { super.viewDidLoad() setupUI() } private func setupUI() { let nameLabel = UILabel() nameLabel.text = callerName ?? "Unknown" nameLabel.font = UIFont.boldSystemFont(ofSize: 24) callDurationLabel = UILabel() callDurationLabel.text = "00:00" // Duration will be updated as the call goes on let endCallButton = UIButton(type: .system) endCallButton.setTitle("End Call", for: .normal) endCallButton.addTarget(self, action: #selector(endCall), for: .touchUpInside) // Layout code for adding subviews (nameLabel, callDurationLabel, endCallButton) will go here. } @objc private func endCall() { // Implement end call logic } }
2. Incoming Call Notifications: When an incoming call is received, your app should be able to present a notification to the user. CallKit handles this aspect, but you can customize the notification sound or display a custom message along with the standard incoming call interface. Make sure to report incoming calls with accurate details, ensuring the user has relevant information about who is calling:
func reportIncomingCall(uuid: UUID, handle: String) { let callUpdate = CXCallUpdate() callUpdate.remoteHandle = CXHandle(type: .phoneNumber, value: handle) callUpdate.hasVideo = false // Set to true if the call supports video provider.reportNewIncomingCall(with: uuid, update: callUpdate) { error in if let error = error { print("Failed to report incoming call: (error)") } else { print("Incoming call reported successfully.") } } }
3. Call State Updates: Your interface should reflect changes in call state (connected, on hold, ended) in real-time. This can be achieved by listening to CallKit delegate callbacks and updating the UI accordingly. You might want to show a different interface when the call is on hold or when it has ended:
func provider(_ provider: CXProvider, didActivate audioSession: AVAudioSession) { // Update UI to reflect that the call is active updateCallUI(isActive: true) } func provider(_ provider: CXProvider, perform action: CXEndCallAction) { // Update UI to reflect that the call has ended updateCallUI(isActive: false) action.fulfill() } private func updateCallUI(isActive: Bool) { // Logic to show/hide UI elements based on call state callDurationLabel.isHidden = !isActive }
4. Accessibility: Ensure that your UI is accessible to all users. Implement voiceover support and make sure the buttons are large enough to be easily tapped. Providing a good experience for users with disabilities should be a priority in your design.
5. System Integration: Since CallKit uses the native call interface, avoid trying to replicate it entirely in your app. Instead, focus on enhancing the user experience for VoIP calls while adhering to iOS design guidelines. Use system colors and fonts to maintain a consistent look and feel with the rest of iOS.
Designing a user interface for CallKit requires careful consideration of the elements and user interactions involved in making and receiving calls. By focusing on clarity, responsiveness, and integration with native iOS features, you can create a VoIP application that feels like a natural extension of the iPhone’s calling capabilities, ultimately leading to greater user satisfaction and engagement.
Testing and Debugging CallKit Applications
Testing and debugging CallKit applications can be an intricate process, as it involves not only the calls themselves but also the underlying VoIP service interaction and the overall flow of call states in your application. It’s crucial to ensure that your app behaves correctly under various scenarios, such as incoming calls, outgoing calls, call interruptions, and network changes. Below are some strategies to effectively test and debug CallKit applications.
1. Use Unit Tests: Begin by writing unit tests for individual components of your CallKit integration. This includes testing methods that handle call actions, like starting and ending calls. For example, you can mock the CXProvider and CXCallController interactions to verify that your call management logic functions as expected.
func testStartCall() { let callManager = CallManager() let uuid = UUID() // Simulate starting a call callManager.startCall(uuid: uuid, handle: CXHandle(type: .phoneNumber, value: "1234567890")) // Assert that the call is started and reported correctly XCTAssertTrue(callManager.isCallActive(uuid), "Call should be active after starting.") }
2. Logging: Incorporate detailed logging throughout your CallKit implementation. This will help you trace the flow of call states and the corresponding actions taken by the application. Think using a logging framework or simply print statements to track call state transitions.
func provider(_ provider: CXProvider, perform action: CXStartCallAction) { print("Starting call with UUID: (action.callUUID)") // Additional logic... action.fulfill() }
3. Use Xcode’s Debugger: Take advantage of Xcode’s debugging tools to set breakpoints and monitor the state of your application as calls are initiated and terminated. You can inspect the state of your ongoing calls and ensure that updates are reflected accurately in the UI.
4. Simulate Incoming Calls: To test how your app handles incoming calls, you can create a mock VoIP service that triggers incoming call events. This allows you to see if the CallKit integration responds as expected when a call is received, including the display of the native call interface.
func simulateIncomingCall() { let uuid = UUID() reportIncomingCall(uuid: uuid, handle: "9876543210") // Verify that the incoming call is reported correctly }
5. Test in Different Scenarios: Ensure to test your application in various scenarios, such as network interruptions, background state, and when the app is terminated. CallKit’s behavior can change based on the state of your app, so it’s essential to validate that your app handles transitions smoothly.
6. Test on Physical Devices: Always conduct tests on real devices rather than simulators, as some CallKit features, particularly those involving VoIP, may not function correctly in the simulator environment. Testing on actual hardware will yield more accurate results and better emulate how users will experience your app.
// Sample code to test handling of network changes func simulateNetworkChange() { // Logic to simulate network interruption // Verify that the app handles the interruption correctly print("Simulating network change...") }
By applying these testing and debugging strategies, you can ensure that your CallKit application operates reliably and provides a smooth user experience. The complexities of managing call states and interactions can be mitigated through thorough testing, which will allow you to identify issues early in the development cycle and enhance the quality of your VoIP application.