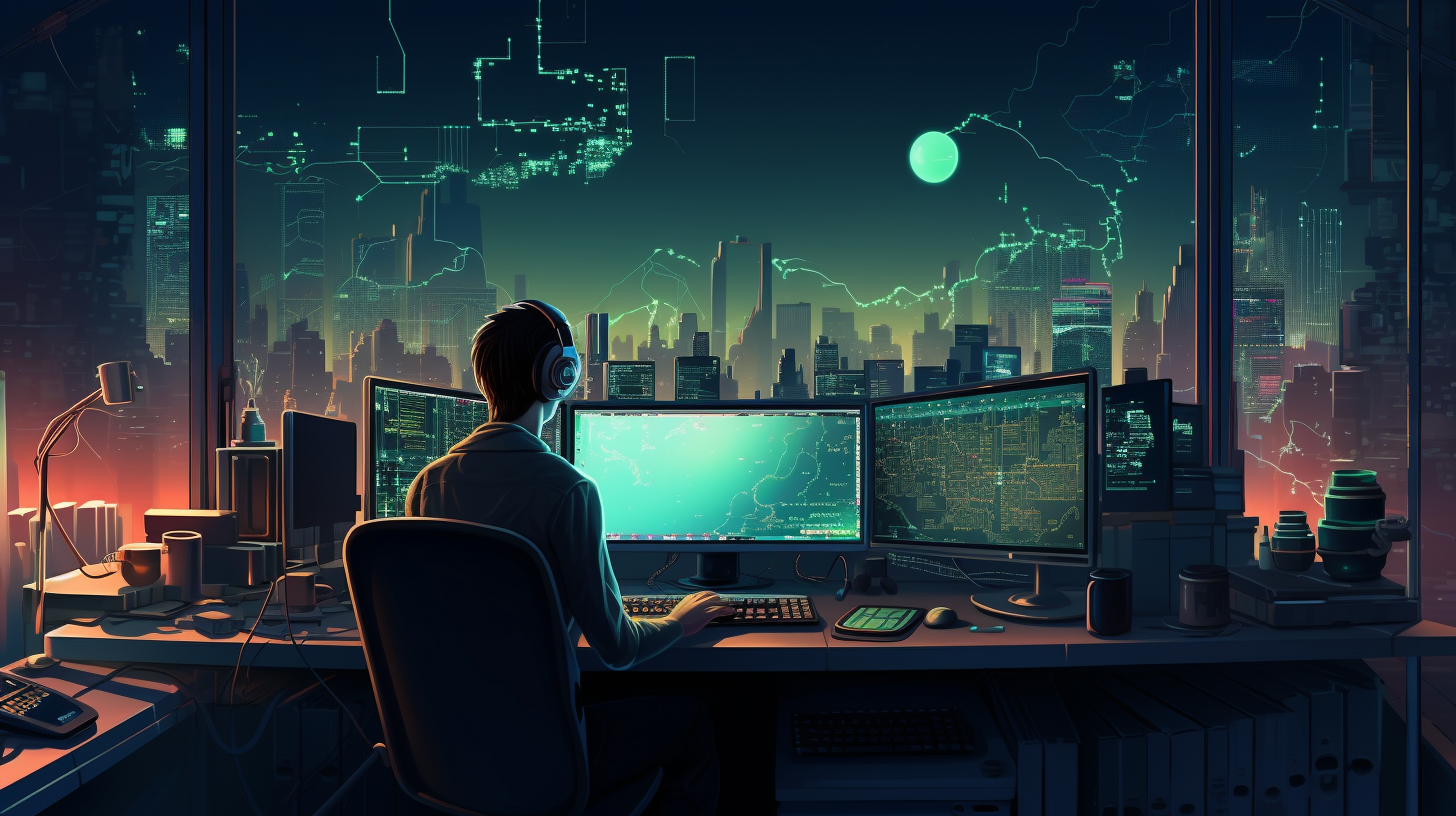
Swift and Combine Framework
The Combine framework, introduced by Apple in Swift, represents a declarative approach to handling asynchronous events and offers a powerful way to manage data streams. At its core, Combine is designed to simplify the complex task of reacting to changes in data over time, making it an invaluable tool for Swift developers.
At its foundation, Combine revolves around the idea of publishers and subscribers. Publishers emit a series of values over time, while subscribers listen to those emissions and respond accordingly. This creates a reactive programming model that allows for more graceful handling of asynchronous data flows.
To demonstrate how Combine works, consider the following example where we create a simple publisher that emits a sequence of integers:
import Combine let publisher = NumbersPublisher() // Custom publisher that emits integers let cancellable = publisher .sink(receiveCompletion: { completion in switch completion { case .finished: print("Finished receiving values.") case .failure(let error): print("Received error: (error)") } }, receiveValue: { value in print("Received value: (value)") })
In this example, we define a custom NumbersPublisher
that emits integers. We then create a subscriber that responds to emitted values while also handling completion events, whether successful or erroneous.
Combine embraces the idea of functional programming, allowing developers to compose and manipulate data streams with a variety of operators. This makes it possible to transform the data as it flows from publishers to subscribers, enhancing the readability and maintainability of your code.
In addition to its core components, Combine integrates seamlessly with other parts of the Swift ecosystem, including SwiftUI, making it particularly useful for building modern iOS applications. The interplay between Combine and SwiftUI allows for automatic updates to the UI in response to changes in data models, creating a more dynamic and responsive user experience.
Understanding the basics of the Combine framework is essential for using its full potential in your applications, so that you can write cleaner, more efficient code that elegantly handles asynchronous events.
Key Components of Combine: Publishers and Subscribers
To delve deeper into the key components of Combine, it is crucial to understand the roles that publishers and subscribers play in this framework. Publishers are the core entities that generate values over time, while subscribers respond to those values and perform actions based on the received data. This relationship creates a powerful pattern where data flows in a unidirectional manner, facilitating clear and predictable interactions.
Publishers can be thought of as the source of data streams. There are various types of built-in publishers in Combine, such as Just
, Future
, and PassthroughSubject
. Each serves a different purpose, but they all conform to the Publisher protocol, which defines how values and completion events are emitted. For example, the Just
publisher emits a single value and then completes:
import Combine let justPublisher = Just("Hello, Combine!") let cancellable = justPublisher .sink(receiveCompletion: { completion in switch completion { case .finished: print("Just publisher finished.") case .failure(let error): print("Just publisher received error: (error)") } }, receiveValue: { value in print("Just publisher emitted value: (value)") })
In this example, the Just
publisher emits a single string value. The subscriber receives this value and handles the completion event appropriately.
On the other hand, subscribers are the consumers of the data emitted by publishers. They are defined by the Subscriber protocol and require a subscription to start receiving values. Subscribers have methods to handle both the incoming values and the completion events. The most common subscriber in Combine is the sink
method, which allows you to specify how to react to values and completion. Here’s an example using a PassthroughSubject
, which can emit multiple values over time:
import Combine let subject = PassthroughSubject() let cancellable = subject .sink(receiveCompletion: { completion in print("Subject completed with: (completion)") }, receiveValue: { value in print("Subject received value: (value)") }) subject.send(1) subject.send(2) subject.send(completion: .finished)
In this case, the PassthroughSubject
serves as both a publisher and a subscriber, allowing for dynamic emissions of values. Each time we call send
, the subscriber reacts to the new data. When we call send(completion: .finished)
, it indicates that no more values will be sent, and the subscriber can react accordingly.
By understanding how publishers and subscribers interact in Combine, developers can create robust, reactive systems that respond to events in real-time. This architecture leads to cleaner code and improved application responsiveness. Through the combination of these two components, Combine enables the creation of sophisticated data pipelines that can handle complex workflows efficiently.
Handling Asynchronous Events with Combine
Handling asynchronous events with Combine involves using its powerful publisher-subscriber architecture to respond to real-time data changes effectively. The beauty of Combine lies in its ability to seamlessly handle asynchronous operations, allowing developers to write concise, readable code that reacts to data flows as they happen.
Asynchronous events can come from various sources, such as network responses, user interface interactions, or any other time-based operations. To illustrate this, let’s ponder a common scenario where we fetch data from a network API and handle the results asynchronously. With Combine, we can create a publisher that represents our network request, allowing us to subscribe to its output and manage the response efficiently.
import Combine import Foundation struct APIResponse: Decodable { let id: Int let name: String } class APIClient { func fetchData(from url: URL) -> AnyPublisher { return URLSession.shared.dataTaskPublisher(for: url) .map { $0.data } .decode(type: APIResponse.self, decoder: JSONDecoder()) .eraseToAnyPublisher() } } let url = URL(string: "https://api.example.com/data")! let apiClient = APIClient() let cancellable = apiClient.fetchData(from: url) .sink(receiveCompletion: { completion in switch completion { case .finished: print("Finished fetching data.") case .failure(let error): print("Failed to fetch data with error: (error)") } }, receiveValue: { response in print("Received response: (response)") })
In this example, we define an APIClient class that features a method to fetch data from a given URL. The method returns an AnyPublisher
that emits an APIResponse
or an Error
. By using dataTaskPublisher
from URLSession
, we create a publisher that handles the network request. The map
operator transforms the output to just the data part of the response, while decode
converts the JSON data into our response model.
Once we have our publisher, we subscribe to it using sink
. That’s where we handle the completion and the received value. The receiveCompletion
closure lets us manage both successful and error states. If the request completes successfully, we print a message indicating completion; in the case of an error, we handle it gracefully.
Combine also allows us to chain multiple operations together, rendering it effortless to handle complex asynchronous workflows. For instance, let’s say we want to perform some additional processing on the data received from our network request:
let cancellable = apiClient.fetchData(from: url) .map { $0.name.uppercased() } // Transforming the name to upper case .sink(receiveCompletion: { completion in switch completion { case .finished: print("Finished processing data.") case .failure(let error): print("Failed to process data with error: (error)") } }, receiveValue: { transformedValue in print("Transformed value: (transformedValue)") })
In this extended example, we take the received APIResponse
and use the map
operator to transform the name
property to upper case. This illustrates how Combine enables a clean flow of data transformation, maintaining a clear and simple handling of asynchronous events.
By embracing the Combine framework, developers can manage asynchronous events with confidence, enabling smooth and responsive applications. The reactive approach not only simplifies error handling but also enriches the development experience by allowing for a declarative style, ultimately leading to code that is easier to understand and maintain.
Combining Multiple Publishers: Operators and Transformations
Combining multiple publishers in Combine is a powerful feature that allows developers to orchestrate complex data flows by merging or transforming streams of values from different sources. The framework offers a variety of operators that facilitate the combination of publishers, allowing you to create sophisticated pipelines that respond to data in real-time.
One of the most simpler ways to combine publishers is through the merge operator. This operator takes multiple publishers and merges their output into a single stream. Here’s how you can use it:
import Combine let publisher1 = PassthroughSubject() let publisher2 = PassthroughSubject() let mergedPublisher = publisher1.merge(with: publisher2) let cancellable = mergedPublisher .sink(receiveValue: { value in print("Received merged value: (value)") }) publisher1.send(1) publisher2.send(2) publisher1.send(3) publisher2.send(completion: .finished)
In this example, two PassthroughSubject
instances are created, representing different sources of integer values. By using the merge
operator, we combine their emissions into a single stream. When either publisher sends a value, the subscriber receives it, demonstrating how seamlessly Combine can handle multiple data sources.
Another powerful operator is combineLatest, which allows you to combine the latest emitted values from multiple publishers. That’s particularly useful when you want to respond to changes in multiple sources of data at the same time. Here’s an example:
import Combine let nameSubject = PassthroughSubject() let ageSubject = PassthroughSubject() let combinedPublisher = nameSubject.combineLatest(ageSubject) let cancellable = combinedPublisher .sink(receiveValue: { name, age in print("Received combined value: Name - (name), Age - (age)") }) nameSubject.send("Alice") ageSubject.send(30) nameSubject.send("Bob") ageSubject.send(25)
In this scenario, the combineLatest
operator combines the latest values from both the nameSubject
and the ageSubject
. Whenever either publisher emits a new value, the subscriber receives the latest values from both, allowing for real-time updates based on multiple inputs.
Transformations are equally important when combining publishers. The map operator allows you to modify the emitted values before they reach the subscriber. For instance:
import Combine let numbersPublisher = [1, 2, 3, 4, 5].publisher let transformedPublisher = numbersPublisher .map { $0 * 2 } // Transforming each emitted value let cancellable = transformedPublisher .sink(receiveValue: { value in print("Transformed value: (value)") })
Here, we create a simple publisher from an array and use the map
operator to double each emitted value. This transformation occurs seamlessly, allowing the subscriber to receive and react to the modified values.
Combine also provides operators like flatMap, which is used to flatten nested publishers. That’s particularly useful when you have a publisher that emits other publishers. For example:
import Combine func fetchData(for id: Int) -> AnyPublisher { Just("Data for ID: (id)") .delay(for: .seconds(1), scheduler: RunLoop.main) // Simulating a delay .eraseToAnyPublisher() } let idsPublisher = [1, 2, 3].publisher let combinedDataPublisher = idsPublisher .flatMap { id in fetchData(for: id) } let cancellable = combinedDataPublisher .sink(receiveValue: { data in print("Received data: (data)") })
In this example, the flatMap
operator allows us to take each emitted ID and call a function that returns a publisher. This effectively flattens the structure, and the subscriber receives the results of the asynchronous fetch calls in a linear stream.
Through these operators and transformations, Combine empowers developers to create intricate data pipelines that are both elegant and efficient. The declarative syntax not only enhances code readability but also aligns with Swift’s type-safe nature, ensuring that data handling is predictable and reliable. By thoughtfully combining multiple publishers, you can manage complex asynchronous workflows with grace and precision.
Best Practices for Integrating Combine into Swift Applications
Integrating Combine into Swift applications requires a thoughtful approach to ensure that the reactive programming model enhances the overall architecture and maintainability of the codebase. Here are some best practices to consider when using Combine in your projects.
1. Keep Your Subscribers Organized
Managing subscriptions is critical when using Combine, especially to avoid memory leaks. Assign any cancellable subscriptions to a property within your view model or controller. This ensures that they are properly held in memory while needed and released when no longer in use.
class MyViewModel { private var cancellables = Set() // Store cancellables here func fetchData() { let publisher = Just("Hello, Combine!") publisher .sink(receiveValue: { value in print(value) }) .store(in: &cancellables) // Store the cancellable } }
2. Use Operators Wisely
Combine offers a plethora of operators to manipulate data streams effectively. However, using too many operators can lead to complex chains that are hard to read and maintain. Aim for a balance between functionality and clarity. Think breaking down complex transformations into smaller, reusable methods or computed properties to enhance readability.
func transformData(_ input: AnyPublisher) -> AnyPublisher { return input .map { $0.uppercased() } .eraseToAnyPublisher() }
3. Leverage Combine with SwiftUI
When working with SwiftUI, Combine becomes even more powerful. Use the @Published property wrapper in your view models to bind properties directly to SwiftUI views. This allows for automatic updates in the UI when the data changes, aligning the reactive nature of Combine with the declarative syntax of SwiftUI.
class UserViewModel: ObservableObject { @Published var userName: String = "" func updateUserName(to newName: String) { userName = newName } }
4. Handle Errors Gracefully
Errors are an intrinsic part of asynchronous workflows. Combine provides various ways to handle errors elegantly. Use the catch operator to provide fallback values or the retry operator to attempt a subscription again upon failure. This not only ensures robustness but also improves the user experience by managing unexpected situations gracefully.
let publisher = URLSession.shared.dataTaskPublisher(for: url) .map(.data) .decode(type: APIResponse.self, decoder: JSONDecoder()) .catch { error in Just(APIResponse(id: 0, name: "Fallback Name")) // Provide a fallback } .eraseToAnyPublisher()
5. Test Your Combine Code
Unit testing Combine publishers can be tricky, but it is essential for ensuring your reactive code behaves as expected. Use the TestScheduler to trigger and verify publisher emissions in a controlled manner. This can help you ensure that your Combine logic is robust and reliable.
func testPublisher() { let scheduler = TestScheduler(initialClock: 0) let publisher = scheduler.createColdObservable([.next(100, "Test"), .completed(200)]) let observer = scheduler.createObserver(String.self) publisher.subscribe(observer).disposed(by: disposeBag) scheduler.start() XCTAssertEqual(observer.events, [.next(100, "Test"), .completed(200)]) }
By following these best practices, you can effectively integrate Combine into your Swift applications, enabling a more reactive, maintainable, and robust codebase. The key lies in understanding the framework’s capabilities and ensuring that the usage aligns with the principles of clean and efficient coding. Combine’s power comes from its ability to simplify complex data flows while maintaining clarity and performance.