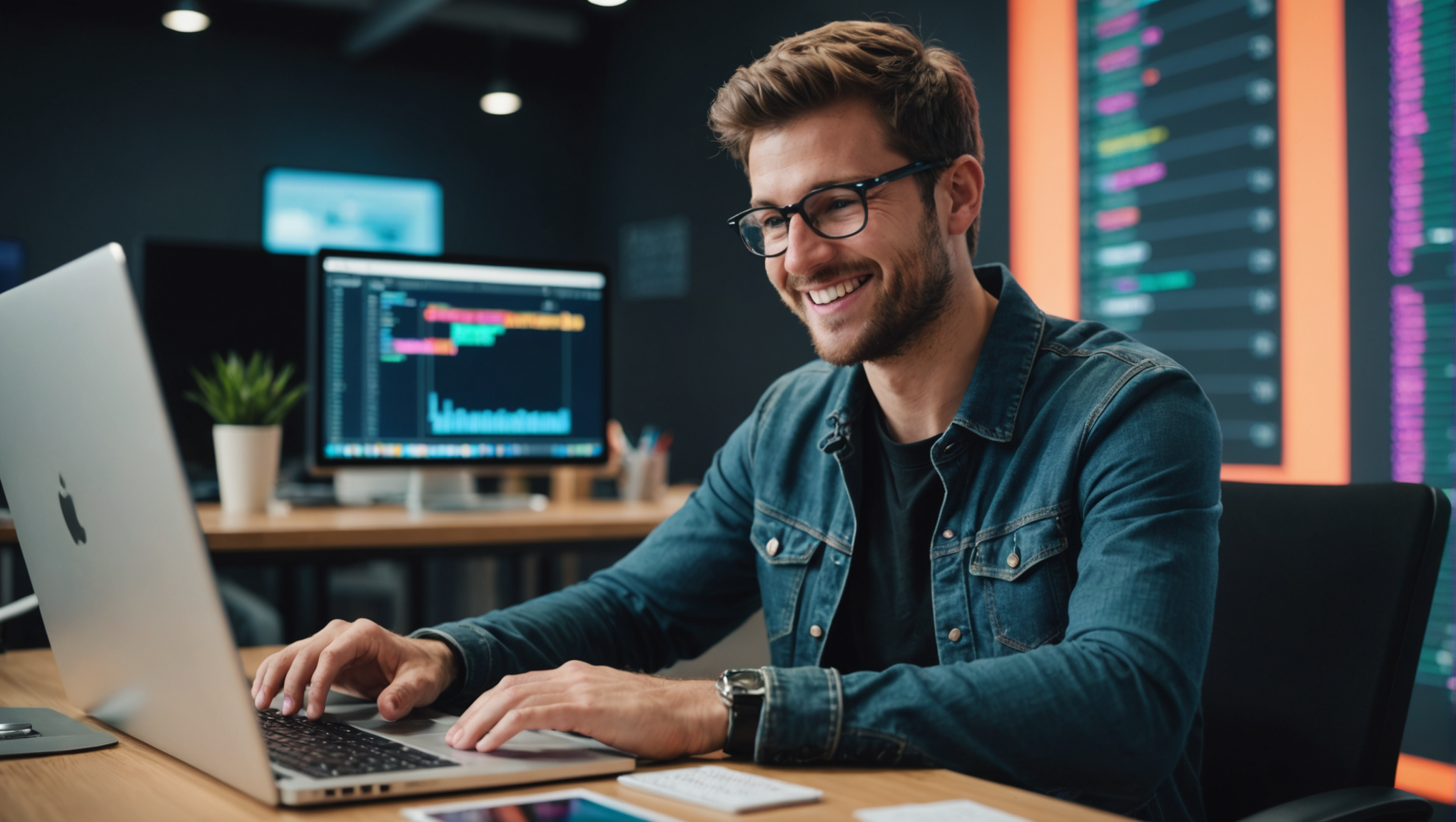
Swift and Continuous Integration
Continuous Integration (CI) fundamentally transforms the development lifecycle for Swift applications, allowing teams to integrate code changes more frequently and reliably. At its core, CI is the practice of automatically testing and merging code changes, ensuring that any new code introduced doesn’t break existing functionality. This process helps developers catch bugs early, improve code quality, and streamline the deployment pipeline.
In the Swift ecosystem, CI processes can be integrated seamlessly with tools like Xcode and various CI services. By using CI, Swift developers can maintain a clean and functional codebase, enabling them to focus on innovation rather than debugging. The CI practice promotes a culture of collaboration, where developers can confidently push their changes, knowing that automated tests will verify the integrity of the application.
The essence of CI involves a few key components:
- Swift’s robust testing framework, XCTest, allows developers to write unit tests and UI tests. By automating these tests in the CI pipeline, developers can ensure that every code change is validated against a suite of tests.
- Each change pushed to the repository triggers a build process. This automation verifies that the application compiles successfully and that the new changes integrate seamlessly with the existing code.
- A critical aspect of CI is the quick feedback loop it creates. Developers receive immediate notifications about the success or failure of builds and tests, enabling them to address issues promptly.
To illustrate the power of CI in Swift development, ponder this simple example of a test case written using XCTest:
import XCTest class MySwiftTests: XCTestCase { func testExample() { let result = addNumbers(2, 3) XCTAssertEqual(result, 5, "The addNumbers function should return the sum of the two numbers") } func addNumbers(_ a: Int, _ b: Int) -> Int { return a + b } }
In this example, the test case checks the functionality of a simple addition function. When integrated into a CI pipeline, each code push would trigger this test, ensuring that the `addNumbers` function behaves as expected. If the test fails, developers are alerted immediately, allowing them to diagnose the issue before it affects the main codebase.
Furthermore, CI isn’t merely about automating tests and builds; it also plays a vital role in code quality. By enforcing coding standards and conducting static analysis as part of the CI process, teams can ensure that the code adheres to best practices, ultimately reducing technical debt over time.
Understanding Continuous Integration in Swift development underscores its importance in creating a robust and maintainable codebase. By integrating CI practices, Swift developers can foster a more collaborative and efficient development environment, where quality is paramount, and innovation can thrive.
Setting Up a CI/CD Pipeline for Swift Projects
Setting up a CI/CD (Continuous Integration/Continuous Deployment) pipeline for Swift projects involves several systematic steps that ensure your application is built, tested, and deployed in an efficient manner. The goal is to automate as much of the process as possible, allowing developers to focus on writing code rather than managing builds and deployments.
The first step in establishing a CI/CD pipeline is to select a CI/CD service that aligns with your project requirements. Popular choices include GitHub Actions, Bitrise, Travis CI, and CircleCI. Each of these tools offers unique features tailored specifically for Swift projects, such as integration with Xcode and support for Swift Package Manager.
Once you have selected a CI tool, the next step is to configure your project repository. The configuration usually involves creating a YAML or similar file that outlines the build steps your CI tool should perform. Here’s an example configuration snippet for GitHub Actions:
name: CI on: push: branches: [ main ] pull_request: branches: [ main ] jobs: build: runs-on: macos-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Setup Xcode uses: maxim-lobanov/setup-xcode@v1 with: xcode-version: '12.5' - name: Install Dependencies run: | xcodebuild -resolvePackageDependencies - name: Build run: | xcodebuild -scheme YourSchemeName -sdk iphonesimulator -destination 'platform=iOS Simulator,name=iPhone 12,OS=latest' build - name: Run Tests run: | xcodebuild test -scheme YourSchemeName -sdk iphonesimulator -destination 'platform=iOS Simulator,name=iPhone 12,OS=latest'
This YAML file specifies that the workflow should run on pushes or pull requests to the main branch. It checks out the code, sets up the specified Xcode version, installs dependencies via Swift Package Manager, builds the project, and finally runs tests against the built application.
Next, you’ll want to ensure your project is configured for testing. Swift’s XCTest framework allows you to write unit and UI tests. For the CI/CD pipeline to be effective, ensure that you have adequate test coverage of your codebase. A well-structured test suite not only validates functionality but can also detect regressions introduced by new code changes.
Incorporating code quality checks is another vital aspect of setting up your CI/CD pipeline. Tools such as SwiftLint can help enforce coding standards by automatically checking your code for stylistic issues. You can integrate SwiftLint in your CI workflow with a simple step added to your configuration file:
- name: Run SwiftLint run: | swift run swiftlint
This step ensures that your code adheres to the defined style guide before it gets merged into the main branch, helping maintain a clean and consistent codebase.
Finally, after successfully building and testing your application, you might want to deploy it automatically. This can be done by adding deployment steps to your CI/CD configuration. For example, you can use fastlane to handle deployment to the App Store or distribute builds to testers. Here’s a quick overview of how you might add a deployment step using fastlane:
- name: Deploy to App Store run: | bundle exec fastlane deploy
With the above setup, every time you push code to your repository or create a pull request, your pipeline will automatically build the application, run tests, check coding standards, and deploy to the App Store if everything passes. This level of automation is powerful, allowing your team to deliver features and fixes rapidly while maintaining high quality.
Popular CI Tools for Swift: A Comparative Overview
When it comes to selecting Continuous Integration (CI) tools for Swift development, there are several options available, each with its own strengths and weaknesses. The choice of tool can significantly impact the efficiency of your development process, the ease of integration with existing workflows, and the overall quality of your codebase. Below, we will explore some popular CI services tailored for Swift projects, providing a comparative overview to help you make an informed decision.
GitHub Actions has rapidly gained popularity due to its seamless integration with GitHub repositories. It allows developers to define workflows directly within their repositories using YAML files. The flexibility of GitHub Actions means you can customize your CI pipelines to suit various development needs, from building and testing to deploying applications. The extensive marketplace also offers pre-built actions for common tasks, rendering it effortless to incorporate functionalities like code linting or deployment. For Swift developers, GitHub Actions is particularly appealing because of its ability to run on macOS environments, which are essential for building and testing iOS applications.
Bitrise is another robust CI/CD tool specifically designed for mobile applications, including those built with Swift. One of its standout features is its intuitive user interface that simplifies the process of creating workflows. Bitrise provides a large selection of pre-defined steps, rendering it effortless to integrate testing, building, and deployment processes without extensive configuration. The platform also boasts a rich ecosystem of integrations with other tools, such as Firebase and Slack, allowing teams to streamline their development workflows further. Bitrise’s focus on mobile CI/CD makes it an excellent choice for Swift developers looking for a dedicated solution.
Travis CI has been a staple in the CI/CD landscape for years and continues to be a popular option among developers. Although it originally gained traction for open-source projects, it has expanded its offerings to support private repositories. Travis CI allows for comprehensive configuration through its .travis.yml file, enabling developers to define build environments, dependencies, and test commands. While Travis CI supports macOS builds, it is essential to think the limitations in terms of resources and concurrency, which could impact larger Swift projects requiring extensive testing.
CircleCI is known for its speed and flexibility. Like Travis CI, it provides extensive configurability through a YAML configuration file. CircleCI’s parallelism and caching capabilities can significantly reduce build times, making it a great choice for projects with substantial testing requirements. Additionally, CircleCI offers a robust set of integrations, allowing teams to connect with other tools in their development stack effortlessly. Swift developers benefit from CircleCI’s ability to run builds in macOS environments, though it may require more manual setup compared to other tools like Bitrise.
As you evaluate these options, ponder the following factors to guide your decision:
- Choose a tool that aligns with your team’s existing knowledge and expertise to minimize the learning curve.
- Assess whether the CI tool can easily integrate with the services and tools your team already uses.
- Look for tools that have a strong community and readily available support, which can be invaluable when troubleshooting issues.
- While some tools offer free tiers, others may incur costs as your needs scale. Evaluate the pricing models to ensure they fit within your budget.
Ultimately, the right CI tool for your Swift projects will depend on your specific requirements, team dynamics, and project scope. To aid in your transition to CI, think starting with a simple setup to get a feel for the workflow before expanding to more complex configurations. This iterative approach can help you understand the nuances of your chosen CI tool and maximize its potential for enhancing your Swift development process.
Best Practices for Testing and Deployment in Swift CI
When implementing Continuous Integration (CI) practices in Swift development, adhering to best practices for testing and deployment is important. These practices not only ensure that the code is reliable but also streamline the entire development lifecycle, allowing teams to deliver high-quality applications efficiently. Below are some foundational best practices to think.
Maintain a Robust Test Suite
A strong test suite is the backbone of any CI process. Swift developers should strive to incorporate both unit and UI tests using the XCTest framework. This ensures that all components of the application are tested thoroughly. Here’s an example of a UI test that checks if a button is correctly displayed:
import XCTest class MyUITests: XCTestCase { func testButtonExists() { let app = XCUIApplication() app.launch() XCTAssertTrue(app.buttons["MyButton"].exists, "The button should be present on the screen") } }
Running this suite with every code push can reveal issues early, reducing the risk of bugs in production.
Emphasize Test Coverage
While writing tests is essential, monitoring test coverage is equally important. Tools like Xcode’s built-in code coverage reports help identify untested areas of the codebase. Aim for high coverage, but remember that quantity does not always equal quality. Focus on critical paths and potentially problematic areas that could introduce bugs.
Utilize Continuous Deployment
Once the CI setup is functioning smoothly, ponder aligning it with Continuous Deployment (CD) practices. Automating deployment processes minimizes manual errors and accelerates release cycles. Fastlane is a popular tool that can be integrated into CI pipelines to automate deployment to the App Store or TestFlight. Here’s an example of a Fastlane lane for deploying an app:
lane :deploy do build_app(scheme: "YourSchemeName") upload_to_app_store end
This simplistic lane builds the application and uploads it to the App Store, making it an essential component of a CI/CD pipeline.
Run Tests on Multiple Environments
To ensure that your application behaves as expected across different devices and operating system versions, it’s beneficial to run tests on various environments. Leverage simulators for testing across multiple iOS versions and device types. CI tools like Bitrise or GitHub Actions allow you to specify different build configurations easily.
Integrate Static Analysis
Static analysis tools, such as SwiftLint and SonarQube, can catch potential issues before they become problems. By including static analysis in your CI pipeline, you can enforce coding standards and identify code smells. Here’s how you can run SwiftLint as part of your CI configuration:
- name: Run SwiftLint run: | swift run swiftlint
This step ensures that all code adheres to defined conventions, reducing the likelihood of bugs and increasing maintainability.
Implement a Rollback Strategy
No matter how rigorous your testing may be, unforeseen issues can arise in production. Establish a rollback strategy that allows for quick recovery from deployment failures. This might involve maintaining a stable version of your app that can be redeployed with minimal downtime, ensuring seamless user experience.
Monitor CI Results
Finally, a consistent feedback loop is vital. Monitoring the results of each CI run helps identify trends over time. If builds are frequently failing, it may be symptomatic of deeper issues in the codebase or testing strategy. Utilize tools such as Slack for notifications to keep the team informed about the build status, enabling rapid responses to issues as they arise.
By implementing these best practices, Swift developers can create a robust CI environment that enhances code quality, reduces bugs, and accelerates deployment cycles. This holistic approach not only benefits individual developers but also fosters a culture of collaboration and continuous improvement within the team.
Troubleshooting Common CI Issues in Swift Development
When it comes to troubleshooting common CI issues in Swift development, several pitfalls can disrupt the smooth flow of your CI/CD pipeline. By understanding and addressing these challenges, developers can maintain a healthy workflow. Here’s a look at some of the most frequent CI issues encountered and how to resolve them.
1. Build Failures
One of the primary challenges in any CI setup is encountering build failures. These can arise from several sources, including dependency issues, missing files, or incorrect build configurations. To diagnose build failures effectively, it’s essential to analyze the CI logs thoroughly. CI tools usually provide detailed logs that pinpoint where the failure occurred, including compiler errors or missing resources.
To mitigate build failures, ensure that:
- All dependencies are correctly defined in your project file and can be resolved in the CI environment.
- Your CI configuration accurately specifies the build settings required for your project.
- You maintain consistent Xcode versions across local and CI environments.
Example CI Build Command
xcodebuild -scheme YourSchemeName -sdk iphonesimulator -destination 'platform=iOS Simulator,name=iPhone 12,OS=latest' build
2. Test Failures
Test failures can often be more challenging to troubleshoot than build issues. They can stem from a variety of causes, including changes in the codebase, environmental differences, or even flaky tests that fail intermittently. To effectively handle test failures:
- Run tests locally before pushing changes to the repository to confirm that they pass.
- Ensure that tests are deterministic and do not rely on external states that may change.
- Utilize tools like XCTest’s `XCTFail` to help identify which tests are failing during the CI process.
Example Test Case
import XCTest class ExampleTests: XCTestCase { func testNonFlakyBehavior() { let result = performAction() XCTAssertEqual(result, expectedValue, "The result should match the expected value.") } }
3. Configuration Mistakes
Incorrect CI configurations are a common source of headaches. These mistakes can occur in the YAML configuration files, such as misconfigured environment variables, incorrect script paths, or missing steps. It’s advisable to have a version of the CI configuration file that works locally to help track down discrepancies between local and CI environments.
To avoid configuration errors:
- Keep the CI configuration in version control to track changes over time.
- Use CI tools’ built-in testing environments to validate configuration changes before committing.
- Leverage comments within your configuration files to document each step clearly.
4. Resource Limitations
Resource limitations can lead to timeouts during builds or tests. CI services often impose limits on the number of concurrent jobs or the amount of time a job can run. To address these issues:
- Optimize your build and testing processes to run faster. This can include only running necessary tests based on the changes made.
- Think using caching strategies to speed up dependency resolution and build times.
5. Environment Inconsistencies
Differences between local and CI environments can manifest in various ways, impacting the build and test processes. Ensure that the CI environment closely mirrors the local development setup. This includes maintaining the same Xcode version, operating system, and installed dependencies. Regularly updating your CI environment can help close gaps in consistency.
To help align environments:
- Utilize Docker containers or virtual machines that define the environment precisely.
- Document environment specifications to ensure uniform setups across teams.
By staying vigilant and proactive in addressing these common CI issues, Swift developers can maintain a robust and efficient CI/CD pipeline. Emphasizing clear communication, thorough testing, and diligent configuration management will pave the way for a more streamlined development process.