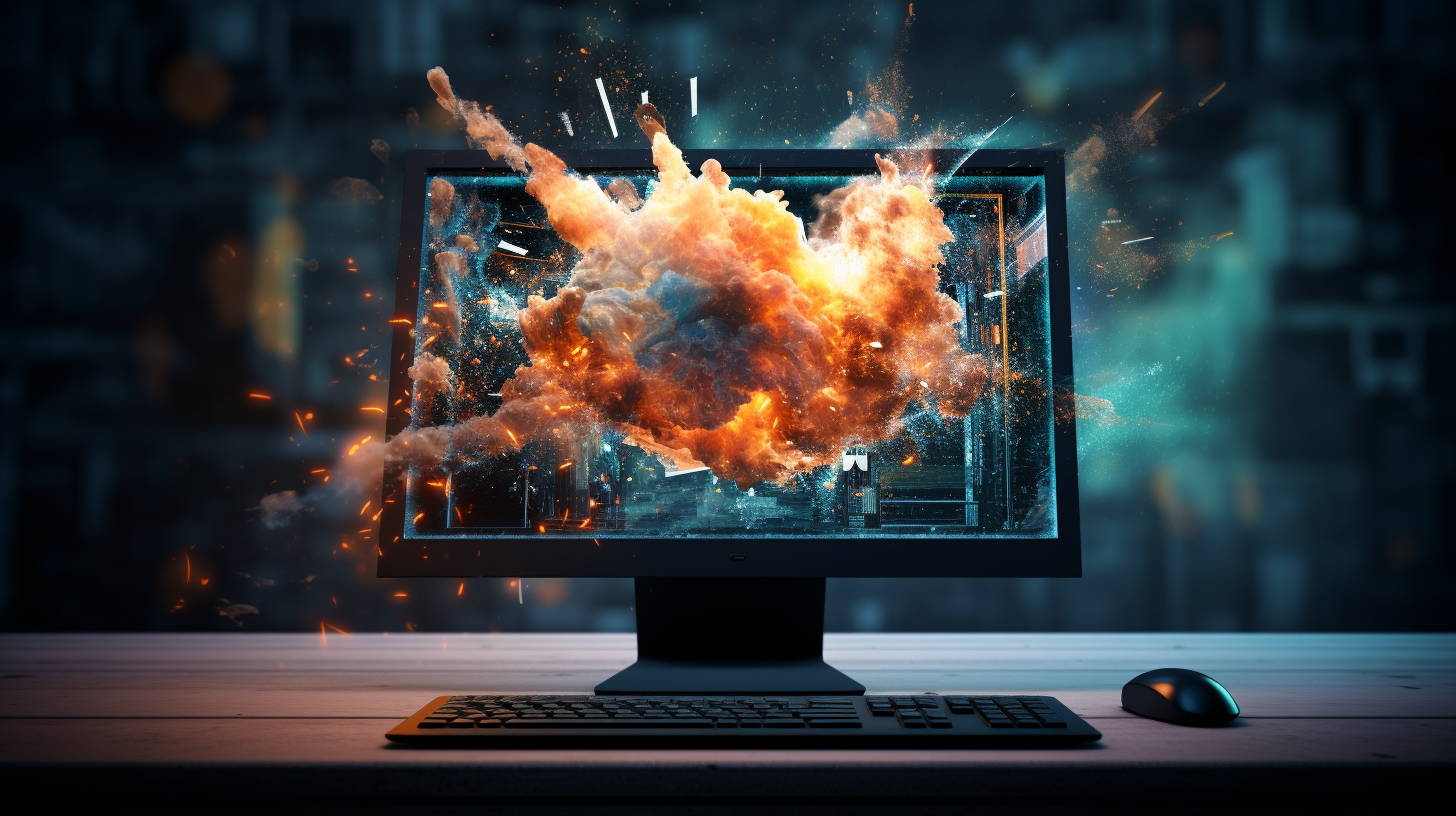
Swift and CoreAudio
The CoreAudio framework is the backbone of audio processing in macOS and iOS, providing a comprehensive suite of low-level APIs for managing audio data. At its core, CoreAudio is designed to offer high performance and low latency, making it ideal for real-time audio applications. Understanding CoreAudio is essential for any Swift developer looking to create sophisticated audio applications.
CoreAudio consists of several components, such as Audio Units, Audio File Services, and Audio Queues. Each component serves a specific purpose in the audio processing pipeline, allowing developers to manipulate audio data, apply effects, and manage audio playback.
One of the fundamental building blocks of CoreAudio is the Audio Buffer. Audio Buffers are used to store audio data that is being processed. They come in various formats, such as interleaved and non-interleaved, and can handle multiple channels. Understanding how to work with these buffers is important for effective audio manipulation.
CoreAudio also provides a powerful mechanism for handling different audio formats through Audio Format Descriptions. Each audio format includes information about sample rate, bit depth, channel count, and encoding type. This allows developers to work seamlessly with different audio sources and ensure compatibility across various platforms.
To interact with audio streams, CoreAudio employs Audio Sessions. These sessions manage audio behavior for an application, such as playback and recording, and help to coordinate audio with other system resources. Swift developers can leverage the AVFoundation framework to interface with CoreAudio, making it easier to manage audio sessions while providing higher-level abstractions.
For instance, when working with audio in Swift, you might define an audio format like this:
let audioFormat = AudioStreamBasicDescription( mSampleRate: 44100.0, mFormatID: kAudioFormatLinearPCM, mFormatFlags: kAudioFormatFlagIsPacked | kAudioFormatFlagIsSignedInteger, mBytesPerPacket: 4, mFramesPerPacket: 1, mBytesPerFrame: 4, mChannelsPerFrame: 2, mBitsPerChannel: 16, mReserved: 0 )
This structure defines a linear PCM audio format with 44.1 kHz sample rate, stereo channels, and 16-bit depth. The parameters specified allow CoreAudio to correctly interpret the audio data being processed.
Understanding the CoreAudio framework is not just about knowing the API; it’s about grasping the underlying concepts that drive audio behavior in your applications. Whether you’re handling simple sound playback or building complex audio processing systems, a solid foundation in CoreAudio will serve you well in your development journey.
Setting Up Swift for CoreAudio Development
Setting up Swift for CoreAudio development involves configuring your development environment and understanding how to best utilize the CoreAudio framework along with Swift’s powerful features. To get started, ensure that you have Xcode installed on your macOS system, as this is the primary development environment for Swift applications. The process includes creating a new project, linking the necessary frameworks, and configuring build settings to accommodate audio processing tasks.
Begin by launching Xcode and creating a new project. Choose the “macOS” platform and then select “App” from the templates. After creating your project, navigate to the project settings by clicking on your project name in the navigator pane. Here, you will need to link the CoreAudio framework to your project. This can be done by selecting the “Build Phases” tab and adding the CoreAudio framework under “Link Binary With Libraries.” This step especially important, as it allows you to access CoreAudio APIs directly from your Swift code.
You’ll also want to configure your project’s build settings to ensure optimal performance for audio processing. For instance, set the “Optimization Level” to “Fastest, Smallest [-Os]” in the “Build Settings” tab to enhance runtime efficiency. Additionally, if you are planning to work with real-time audio processing, you should ensure your app is configured to run in a real-time environment, which may involve adjusting the audio session settings.
Once the project is set up, you can begin coding with Swift. It’s important to note that while Swift offers a more uncomplicated to manage syntax compared to Objective-C, CoreAudio is still fundamentally a C-based API. This means that you will often need to bridge between Swift types and CoreAudio types. A common practice is to create helper functions that facilitate this interaction.
An example of a helper function that converts Swift arrays into CoreAudio-compatible buffers might look like this:
func createAudioBuffer(from samples: [Float]) -> UnsafeMutableAudioBufferListPointer { var audioBufferList = AudioBufferList( mNumberBuffers: 1, mBuffers: AudioBuffer( mNumberChannels: 1, mDataByteSize: UInt32(samples.count * MemoryLayout.size), mData: UnsafeMutableRawPointer(mutating: samples) ) ) return UnsafeMutableAudioBufferListPointer(&audioBufferList) }
This function takes an array of `Float` samples and converts it into a format that CoreAudio can use. The `UnsafeMutableAudioBufferListPointer` allows you to work with the raw audio data directly, which is essential for low-level audio processing.
Moreover, make sure to manage memory appropriately, as CoreAudio requires precise control over audio data buffers. Utilize Swift’s automatic reference counting (ARC) judiciously when dealing with audio buffers, especially when passing pointers to CoreAudio functions.
As you dive deeper into CoreAudio, you may also want to explore other frameworks that work seamlessly with it, such as AVFoundation. This higher-level framework can often simplify tasks like audio session management and media playback while still so that you can tap into CoreAudio’s capabilities when necessary. By using both CoreAudio and Swift, you can build powerful, efficient audio applications that push the boundaries of what’s possible in audio processing.
Working with Audio Buffers in Swift
Working with audio buffers in Swift within the CoreAudio framework is critical for managing audio data effectively. Audio buffers are essential for any audio processing task, serving as the temporary storage locations for audio data during playback or recording. Understanding how to create, manipulate, and pass audio buffers between different components of CoreAudio is key to achieving high-performance audio applications.
CoreAudio uses the AudioBuffer
and AudioBufferList
structures to represent audio data. Each AudioBuffer
contains information about the number of channels, the size of the data being handled, and a pointer to the actual audio data. The AudioBufferList
acts as a container for multiple AudioBuffer
instances, so that you can manage audio data for different channels and formats in a single structure.
To effectively work with audio buffers in Swift, you will often need to perform operations like allocating memory for buffers, filling them with audio data, and releasing that memory when done. Below is a Swift function that demonstrates how to create an audio buffer and populate it with audio samples:
func createAudioBuffer(from samples: [Float], channels: Int) -> AudioBufferList { let numSamples = samples.count let bytesPerSample = MemoryLayout.size let dataSize = numSamples * bytesPerSample // Allocate memory for the audio buffer let audioBufferList = AudioBufferList( mNumberBuffers: 1, mBuffers: AudioBuffer( mNumberChannels: UInt32(channels), mDataByteSize: UInt32(dataSize), mData: UnsafeMutableRawPointer(malloc(dataSize)) ) ) // Copy the samples into the audio buffer memcpy(audioBufferList.mBuffers.mData, samples, dataSize) return audioBufferList }
This function takes an array of audio samples and the number of channels as parameters. It allocates memory for the audio buffer and copies the sample data into the buffer. The use of malloc
is important here, as it allows for dynamic memory allocation, which is necessary when dealing with varying sizes of audio data.
After processing the audio data, it’s vital to release the allocated memory to avoid memory leaks. Below is an example of how to properly free the memory associated with the audio buffer:
func freeAudioBuffer(_ audioBufferList: AudioBufferList) { free(audioBufferList.mBuffers.mData) }
In practice, handling audio buffers often involves passing them to and from various CoreAudio functions. For instance, when working with an AudioUnit
for playback or processing, you will frequently need to fill the audio buffer with input data before calling a function to process or play it. Here’s a simple example of how to use an audio buffer with an AudioUnit
:
func renderAudio(using audioUnit: AudioUnit, audioBufferList: AudioBufferList) { var ioActionFlags = AudioUnitRenderActionFlags() var inNumberFrames: UInt32 = 512 let status = AudioUnitRender(audioUnit, &ioActionFlags, nil, 0, inNumberFrames, &audioBufferList) if status != noErr { print("Error rendering audio: (status)") } }
This function demonstrates rendering audio through an AudioUnit
. It specifies the number of frames to process and calls AudioUnitRender
to fill the provided AudioBufferList
with audio data. Proper error handling is essential to ensure the audio processing pipeline runs smoothly.
Overall, mastering audio buffers in Swift is an integral part of CoreAudio development. By creating efficient buffer management routines and understanding how to manipulate audio data, you can unlock the full potential of audio processing in your applications. As you build your audio applications, always pay attention to performance and memory management, ensuring that you provide a seamless audio experience for your users.
Handling Audio Streams and Formats
In the sphere of audio programming, understanding how to handle audio streams and formats is paramount for ensuring compatibility and performance across different audio sources. CoreAudio provides a robust framework for managing audio streams, allowing developers to manipulate various audio formats seamlessly. This involves not only recognizing the different audio data types but also effectively routing these streams through your application.
Audio streams are essentially sequences of audio data, which can be captured from various sources like microphone input, music files, or synthesized audio. Each stream comes with its own audio format, defined by parameters such as sample rate, bit depth, and channel configuration. Swift developers can leverage this feature by creating and configuring audio formats using the AudioStreamBasicDescription
structure, which lays the groundwork for any audio operation.
To work with audio streams effectively, you need to understand the idea of audio format conversion. Given that audio sources can vary in format, converting between formats is a common requirement. Below is a Swift function that demonstrates how to convert an audio format from one type to another:
func convertAudioFormat(sourceFormat: AudioStreamBasicDescription, targetFormat: AudioStreamBasicDescription) -> AudioStreamBasicDescription? { // Check if the conversion is feasible based on the formats guard sourceFormat.mChannelsPerFrame == targetFormat.mChannelsPerFrame else { print("Channel count mismatch.") return nil } // Adjust sample rate if different if sourceFormat.mSampleRate != targetFormat.mSampleRate { print("Sample rate adjustment required.") // Implement conversion logic based on your requirements } // Return the target format for further processing return targetFormat }
This function checks for compatibility between the source and target audio formats, specifically ensuring that the channel count matches. If they differ, you can implement specific conversion logic for the sample rates or other parameters as needed. That is essential for maintaining audio quality and ensuring that playback or processing occurs without issues.
Moreover, when dealing with audio streams, it’s common to use audio sessions to manage audio behavior across the app. Swift developers can utilize the AVFoundation framework to streamline this process. For instance, configuring an AVAudioSession
allows you to set the category for your audio session, which determines how your app interacts with audio playback and recording. Here is how you might configure an audio session in Swift:
import AVFoundation func setupAudioSession() { let session = AVAudioSession.sharedInstance() do { try session.setCategory(.playAndRecord, mode: .default, options: [.defaultToSpeaker]) try session.setActive(true) print("Audio session configured successfully.") } catch { print("Failed to configure audio session: (error)") } }
This setup configures the audio session for both playback and recording, so that you can manage audio streams effectively. By setting the audio session category and mode, you can dictate how your application will interact with other audio on the device, which is vital for maintaining a smooth user experience.
In addition to audio session management, handling input and output streams especially important for any audio application. Here’s how you can capture audio input from the microphone and process it using CoreAudio:
func startAudioInput() { let audioEngine = AVAudioEngine() let inputNode = audioEngine.inputNode let format = inputNode.outputFormat(forBus: 0) inputNode.installTap(onBus: 0, bufferSize: 1024, format: format) { (buffer, time) in // Process audio buffer print("Received audio buffer: (buffer)") } do { try audioEngine.start() print("Audio input started successfully.") } catch { print("Failed to start audio input: (error)") } }
In this example, the AVAudioEngine
is initialized, and an input node is set up to capture audio. The tap installed on the input node allows you to receive audio data in the form of buffers, which you can then process as needed. This flexibility is one of the key strengths of using CoreAudio in conjunction with Swift.
Ultimately, handling audio streams and formats encompasses a wide range of techniques, from format conversion to session management. The ability to seamlessly work with various audio data types ensures that your applications can adapt to different audio sources and environments. By mastering these concepts, you can create sophisticated audio applications that deliver high performance and an exceptional user experience.
Advanced CoreAudio Techniques with Swift
Advanced CoreAudio techniques in Swift delve into the intricate functionalities of audio processing, offering developers a myriad of opportunities to customize and optimize their audio applications. One of the most powerful aspects of CoreAudio is its support for real-time audio processing through Audio Units. These units can be used for effects processing, mixing, synthesis, and much more. To effectively utilize Audio Units, you must understand how to set them up, configure their parameters, and manage their audio data flow.
When working with Audio Units, the first step is to create an instance of the desired unit type. For example, if you want to create a reverb effect unit, you can do so as follows:
let audioUnitDescription = AudioComponentDescription( componentType: kAudioUnitType_Effect, componentSubType: kAudioUnitSubType_Reverb, componentManufacturer: kAudioUnitManufacturer_Apple, componentFlags: 0, componentFlagsMask: 0 ) let audioComponent = AudioComponentFindNext(nil, &audioUnitDescription) var audioUnit: AudioUnit? AudioComponentInstanceNew(audioComponent!, &audioUnit)
This code snippet creates a new Audio Unit instance for the reverb effect. Once the Audio Unit is instantiated, you can configure its parameters. Each Audio Unit has a set of properties that you can modify to achieve the desired audio effect. For instance, to set the reverb level, you can use the following code:
let reverbAmount: Float = 0.5 // Range from 0.0 to 1.0 let parameterAddress: AudioUnitParameterID = kReverbParam_DecayTime AudioUnitSetParameter(audioUnit!, parameterAddress, kAudioUnitScope_Global, 0, reverbAmount, 0)
After configuring the Audio Unit parameters, you need to manage the audio data that flows through the unit. This involves creating an audio buffer that you can use when rendering audio. You can connect the output of one unit to the input of another, enabling complex signal chains that process audio in real time. Here’s an example of how to set up an audio processing graph that connects an audio source to the reverb unit:
// Create a new Audio Graph var processingGraph: AUGraph? AUGraphInitialize(processingGraph!) // Assume you have already added nodes for the audio source and effect let audioSourceNode = ... // Your audio source node let reverbNode = ... // Your reverb effect node AUGraphConnectNodeInput(processingGraph!, audioSourceNode, 0, reverbNode, 0) AUGraphConnectNodeInput(processingGraph!, reverbNode, 0, outputNode, 0) // Start the graph AUGraphStart(processingGraph!)
Memory management is also a critical component of advanced CoreAudio techniques. CoreAudio operates at a low level, which means that you need to handle memory allocation and deallocation carefully. When you allocate buffers, ensure that you free them correctly to avoid memory leaks:
func freeAudioBuffers(bufferList: UnsafeMutableAudioBufferListPointer) { for i in 0..<bufferList.pointee.mNumberBuffers { let buffer = bufferList[i] if let dataPointer = buffer.mData { free(dataPointer) } } }
Moreover, for performance optimization, you can take advantage of callbacks and audio processing modes. By using a render callback, you can fill your audio buffer dynamically during the rendering process. That is particularly useful for synths or real-time audio effects where the audio data changes continuously:
func renderCallback(inRefCon: UnsafeMutableRawPointer?, ioActionFlags: UnsafeMutablePointer, inTimeStamp: UnsafePointer, inBusNumber: UInt32, inNumberFrames: UInt32, ioData: UnsafeMutablePointer?) -> OSStatus { // Fill the buffer with audio data let audioBuffer = ioData!.pointee.mBuffers.mData!.assumingMemoryBound(to: Float.self) for frame in 0..<Int(inNumberFrames) { audioBuffer[frame] = generateAudioSample(frame: frame) } return noErr }
This render callback allows you to generate audio samples on-the-fly, making your applications responsive and flexible. Remember to always check the return values of CoreAudio functions, as they can provide insights into issues that may arise during audio processing.
Using the advanced techniques provided by CoreAudio in Swift can significantly enhance the audio experience in your applications. From managing Audio Units and handling memory to performance optimization via callbacks, these practices will empower you to create rich, immersive audio environments that push the boundaries of audio processing capabilities.