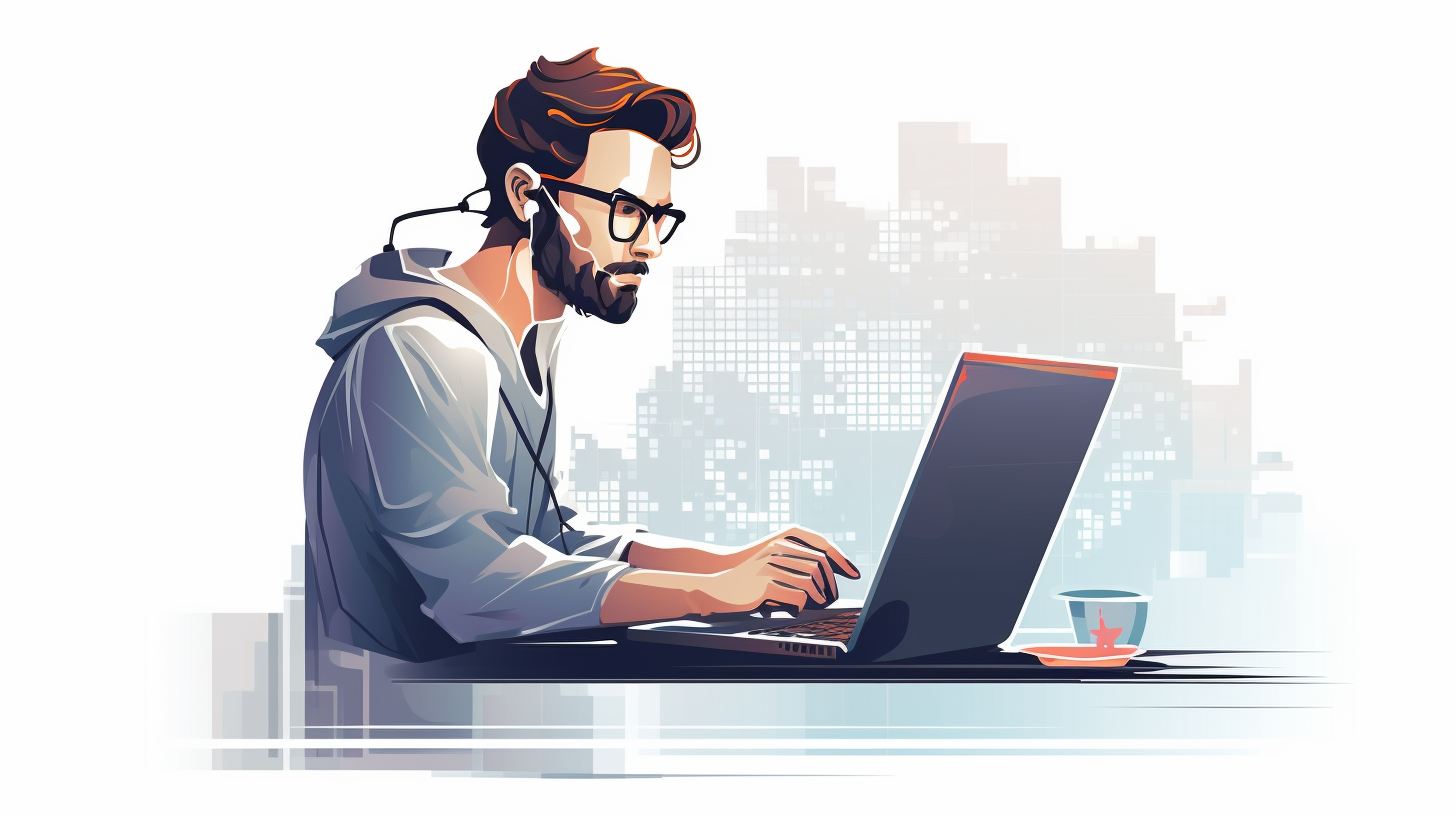
Swift and CoreImage
The Core Image framework is a powerful image processing and analysis API available in iOS and macOS. It was designed to provide high-performance image processing capabilities, using the GPU to accelerate image manipulation tasks. At its core, Core Image is built around the idea of filters that can be applied to images, which allows developers to create rich visual effects, perform image enhancements, and analyze image data with ease.
Core Image operates on the principle of a processing pipeline. Each filter represents a step in this pipeline that takes an input image, applies some transformation, and produces an output image. This modular approach not only simplifies the process of image manipulation but also enables the reuse of filters across different applications.
One of the striking features of Core Image is its ability to handle a wide variety of image formats, including JPEG, PNG, TIFF, and more. This flexibility makes it an ideal choice for applications that require robust image processing capabilities. Furthermore, Core Image supports both 2D and 3D image processing, allowing for a wide range of creative and analytical applications.
As you begin to explore Core Image, you will encounter a rich library of built-in filters that can be used to perform tasks such as blurring, sharpening, color adjustment, and even complex effects like compositing and distortion. Each of these filters can be fine-tuned with various parameters, giving developers a high degree of control over the final output.
let inputImage = CIImage(image: UIImage(named: "example.jpg")!) let filter = CIFilter(name: "CISepiaTone") filter?.setValue(inputImage, forKey: kCIInputImageKey) filter?.setValue(0.8, forKey: kCIInputIntensityKey) if let outputImage = filter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let processedImage = UIImage(cgImage: cgImage) // Use processedImage for your image display or further processing } }
To effectively utilize Core Image, it’s essential to understand the types of images and data structures involved. The primary class used is CIImage
, which represents an image in a format that Core Image can manipulate. You’ll also interact with the CIFilter
class, which provides a simpler interface for applying various image effects.
By using Core Image’s capabilities, developers can enhance user experiences in applications ranging from photo editing to augmented reality. The combination of GPU acceleration and a comprehensive set of image processing functions positions Core Image as a fundamental tool for any Swift developer working with images.
Setting Up Core Image in Swift
Setting up Core Image in Swift is a simpler process, but it requires a clear understanding of how to create and manipulate instances of the necessary classes. First, ensure that you import the Core Image framework in your Swift file. This will allow you to access the various classes and methods that Power the image processing capabilities of Core Image.
import CoreImage
The heart of working with Core Image revolves around the CIImage and CIFilter classes. You begin by creating an instance of CIImage from an existing image. This can be done using an image asset in your app or by loading an image from a URL. Below is an example of how to create a CIImage from a UIImage:
guard let inputImage = CIImage(image: UIImage(named: "example.jpg")!) else { fatalError("Unable to create CIImage from image") }
Once you have your CIImage instance, you can proceed to apply filters. The CIFilter class is responsible for applying various effects. When you create a filter, you need to specify its type using a string identifier, which corresponds to the filter you wish to apply. For example, to create a sepia tone effect, you would do the following:
let filter = CIFilter(name: "CISepiaTone")
After creating the filter, it’s essential to set the input image and any other parameters the filter requires. For the sepia tone filter, you can adjust the intensity level:
filter?.setValue(inputImage, forKey: kCIInputImageKey) filter?.setValue(0.8, forKey: kCIInputIntensityKey)
With the input image and parameters set, you can now retrieve the output image from the filter. This output image is also a CIImage, which can be processed further or converted to a CGImage for display:
if let outputImage = filter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let processedImage = UIImage(cgImage: cgImage) // Use processedImage for your image display or further processing } }
Lastly, the CIContext class is vital for rendering the final image. It acts as a bridge between the CIImage and the graphics output, using the GPU for efficient rendering. Creating a context is typically a one-time operation that can be reused throughout your app to optimize performance.
By following these steps, you can effectively set up and start using Core Image in your Swift projects, paving the way for powerful image processing and manipulation capabilities.
Working with Image Filters
The real power of Core Image lies in its extensive library of filters, each designed to achieve a specific visual effect or transformation. When working with image filters, it's essential first to grasp how to interact with the CIFilter class, which serves as the gateway to applying various effects. To use a filter effectively, you can create an instance of CIFilter by specifying its type as a string. For example, if you want to apply a Gaussian blur to an image, you would do the following: let blurFilter = CIFilter(name: "CIGaussianBlur") blurFilter?.setValue(inputImage, forKey: kCIInputImageKey) blurFilter?.setValue(10.0, forKey: kCIInputRadiusKey) In this example, we create a filter named "CIGaussianBlur" and set the input image. The `kCIInputRadiusKey` parameter determines the intensity of the blur effect. After configuring the filter, you retrieve the output image as usual: if let outputImage = blurFilter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let processedImage = UIImage(cgImage: cgImage) // Use processedImage for your image display or further processing } } Core Image provides a rich assortment of filters, each identifiable by a unique name. You can explore them by querying the available filters programmatically. This allows you to dynamically apply effects based on user input or predefined criteria. For instance, to list all available filters, you can use the following code snippet: let availableFilters = CIFilter.filterNames(inCategory: kCICategoryBuiltIn) for filterName in availableFilters { print(filterName) } This loop outputs all built-in filters, giving you an overview of what visual transformations you can apply. Moreover, many filters offer adjustable parameters, so that you can fine-tune effects to suit your needs. For example, the "CIColorControls" filter lets you adjust brightness, contrast, and saturation: let colorControlsFilter = CIFilter(name: "CIColorControls") colorControlsFilter?.setValue(inputImage, forKey: kCIInputImageKey) colorControlsFilter?.setValue(1.5, forKey: kCIInputBrightnessKey) colorControlsFilter?.setValue(1.5, forKey: kCIInputContrastKey) colorControlsFilter?.setValue(1.0, forKey: kCIInputSaturationKey) As you can see, by manipulating parameters, you can achieve a wide variety of effects, all within a streamlined workflow. Core Image also supports more complex filtering operations, such as compositing multiple images together. For example, the "CISourceOverCompositing" filter can blend two images, creating interesting overlays: let backgroundImage = CIImage(image: UIImage(named: "background.jpg")!) let overlayImage = CIImage(image: UIImage(named: "overlay.png")!) let compositingFilter = CIFilter(name: "CISourceOverCompositing") compositingFilter?.setValue(overlayImage, forKey: kCIInputImageKey) compositingFilter?.setValue(backgroundImage, forKey: kCIInputBackgroundImageKey) if let outputImage = compositingFilter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let finalImage = UIImage(cgImage: cgImage) // Use finalImage for your image display or further processing } } This example illustrates how simple it's to combine images, which can lead to a plethora of creative possibilities in your applications. With each filter, the key is to experiment with different combinations and settings to achieve your desired visual effect. The modular nature of filters in Core Image makes it easy to layer effects, providing a powerful toolkit for image manipulation that can be both fun and productive. As you continue to delve into Core Image, remember that the only limit is your imagination, and the vast array of filters at your disposal is just waiting to be explored.
Enhancing Images with Core Image
Enhancing images with Core Image allows developers to incorporate sophisticated visual effects and optimizations into their applications conveniently. By using the built-in filters, you can manipulate images to achieve everything from basic corrections to artistic transformations. The following examples will illustrate some common enhancement techniques you can apply using Core Image.
One of the simplest and most effective enhancements is adjusting the brightness and contrast of an image. By using the CIColorControls
filter, you can create a more vibrant or subdued look based on your needs. Here’s how to implement it:
let inputImage = CIImage(image: UIImage(named: "example.jpg")!) let colorControlsFilter = CIFilter(name: "CIColorControls") colorControlsFilter?.setValue(inputImage, forKey: kCIInputImageKey) colorControlsFilter?.setValue(1.0, forKey: kCIInputBrightnessKey) // Adjust brightness colorControlsFilter?.setValue(1.2, forKey: kCIInputContrastKey) // Adjust contrast colorControlsFilter?.setValue(1.0, forKey: kCIInputSaturationKey) // Adjust saturation if let outputImage = colorControlsFilter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let enhancedImage = UIImage(cgImage: cgImage) // Use enhancedImage for your image display or further processing } }
Another powerful enhancement technique is sharpening an image, which can help to bring out details. The CILanczosScaleTransform
filter is useful for sharpness adjustments. Here’s an example of how to use it:
let sharpenFilter = CIFilter(name: "CILanczosScaleTransform") sharpenFilter?.setValue(inputImage, forKey: kCIInputImageKey) sharpenFilter?.setValue(1.0, forKey: kCIInputScaleKey) // Scale factor sharpenFilter?.setValue(0.0, forKey: kCIInputAspectRatioKey) // Keep original aspect ratio if let outputImage = sharpenFilter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let sharpenedImage = UIImage(cgImage: cgImage) // Use sharpenedImage for your image display or further processing } }
Color adjustments can also be made to evoke different moods or styles in images. The CIHueAdjust
filter allows for hue shifting, providing a way to change the overall color tone of an image:
let hueAdjustFilter = CIFilter(name: "CIHueAdjust") hueAdjustFilter?.setValue(inputImage, forKey: kCIInputImageKey) hueAdjustFilter?.setValue(Float.pi / 4, forKey: kCIInputAngleKey) // Rotate hue by 45 degrees if let outputImage = hueAdjustFilter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let hueAdjustedImage = UIImage(cgImage: cgImage) // Use hueAdjustedImage for your image display or further processing } }
For a more artistic touch, you can apply styles like vintage effects using the CISepiaTone
filter combined with additional adjustments. This can create a warm, nostalgic feel:
let sepiaFilter = CIFilter(name: "CISepiaTone") sepiaFilter?.setValue(inputImage, forKey: kCIInputImageKey) sepiaFilter?.setValue(0.7, forKey: kCIInputIntensityKey) // Adjust sepia tone intensity if let outputImage = sepiaFilter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let vintageImage = UIImage(cgImage: cgImage) // Use vintageImage for your image display or further processing } }
Each filter can be customized with various parameters, opening up a world of creative possibilities. By combining multiple filters, you can produce unique effects that enhance the aesthetics of your images further. For example, layering a blur effect followed by a sharpen filter can yield interesting results:
let blurFilter = CIFilter(name: "CIGaussianBlur") blurFilter?.setValue(inputImage, forKey: kCIInputImageKey) blurFilter?.setValue(5.0, forKey: kCIInputRadiusKey) if let blurredImage = blurFilter?.outputImage { let sharpenedBlurFilter = CIFilter(name: "CILanczosScaleTransform") sharpenedBlurFilter?.setValue(blurredImage, forKey: kCIInputImageKey) sharpenedBlurFilter?.setValue(1.0, forKey: kCIInputScaleKey) sharpenedBlurFilter?.setValue(0.0, forKey: kCIInputAspectRatioKey) if let finalOutputImage = sharpenedBlurFilter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(finalOutputImage, from: finalOutputImage.extent) { let combinedEffectImage = UIImage(cgImage: cgImage) // Use combinedEffectImage for your image display or further processing } } }
With Core Image, the possibilities for enhancing images are vast and powerful. By experimenting with different filters and their parameters, you can create stunning visual effects directly within your Swift applications, resulting in engaging and captivating user experiences.
Performance Optimization Techniques
When working with Core Image, performance optimization is key to ensuring that image processing tasks are executed efficiently, especially when dealing with large images or complex filter chains. The performance of your Core Image operations can greatly affect the responsiveness of your applications, particularly on mobile devices where resources are limited. Here are several techniques to enhance performance when using Core Image in Swift.
Utilize CIContext Wisely
Creating a CIContext
can be an expensive operation, as it sets up the necessary GPU resources for rendering images. To optimize performance, ponder keeping a single instance of CIContext
throughout your application. This allows you to reuse the context for multiple image processing tasks without incurring the overhead of creating a new context each time.
let sharedContext = CIContext()
Use sharedContext
whenever you need to render an output image, reducing the overhead significantly:
if let cgImage = sharedContext.createCGImage(outputImage, from: outputImage.extent) { let processedImage = UIImage(cgImage: cgImage) // Use processedImage for your image display or further processing }
Optimize Image Size
Processing high-resolution images can be resource-intensive. Before applying filters, ponder resizing images to a more manageable size. Resizing not only speeds up processing time but also reduces memory usage. You can create a smaller version of your image using CIFilter
or by scaling down the CIImage
directly.
let scaleFilter = CIFilter(name: "CILanczosScaleTransform") scaleFilter?.setValue(inputImage, forKey: kCIInputImageKey) scaleFilter?.setValue(0.5, forKey: kCIInputScaleKey) // Resize to 50% scaleFilter?.setValue(1.0, forKey: kCIInputAspectRatioKey) // Maintain aspect ratio if let resizedImage = scaleFilter?.outputImage { // Proceed with processing the smaller image }
Batch Processing
When applying filters to multiple images, batch processing can be an effective approach. Instead of processing images one by one, you can prepare multiple CIImage
instances and apply the same filter settings across them. This minimizes the overhead of setting up the filter multiple times and can lead to better performance.
let images: [CIImage] = [image1, image2, image3] let filter = CIFilter(name: "CISepiaTone") filter?.setValue(0.7, forKey: kCIInputIntensityKey) for image in images { filter?.setValue(image, forKey: kCIInputImageKey) if let outputImage = filter?.outputImage { if let cgImage = sharedContext.createCGImage(outputImage, from: outputImage.extent) { let processedImage = UIImage(cgImage: cgImage) // Store or display processedImage } } }
Use Metal for Complex Processing
If your application demands highly complex image processing, consider using Metal alongside Core Image. Metal is designed for high-performance graphics and compute operations, and it can provide a significant performance boost when processing images. Core Image can integrate with Metal, which will allow you to create custom filters using Metal shaders. That’s particularly advantageous for applications that require real-time image manipulation, such as video processing or games.
Asynchronous Processing
For applications that require image processing on the main thread, ponder offloading intensive tasks to a background queue. This keeps the user interface responsive while filters are applied. Use Grand Central Dispatch to manage this:
DispatchQueue.global(qos: .userInitiated).async { if let outputImage = filter?.outputImage { let cgImage = sharedContext.createCGImage(outputImage, from: outputImage.extent) DispatchQueue.main.async { let processedImage = UIImage(cgImage: cgImage) // Update UI with processedImage } } }
By implementing these performance optimization techniques, you can greatly enhance the efficiency and responsiveness of your image processing tasks in Core Image. It not only improves user experience but also allows for more complex and visually appealing applications.
Real-World Applications of Core Image in Swift
Real-world applications of Core Image in Swift span across various domains, making it an essential framework for developers who want to enrich their applications with advanced image processing capabilities. From photo editing software to augmented reality experiences, Core Image provides the tools necessary to manipulate and enhance images dynamically. In this section, we’ll explore how Core Image can be effectively utilized in different scenarios.
One compelling application of Core Image is in photo editing apps. By integrating filters and image enhancements, developers can create rich user experiences that allow users to modify their photos with just a few taps. For instance, users can apply a series of filters like “CISepiaTone” or “CIPhotoEffectProcess” to achieve specific looks. The setup is simpler, as shown in the following code snippet:
let inputImage = CIImage(image: UIImage(named: "photo.jpg")!) let sepiaFilter = CIFilter(name: "CISepiaTone") sepiaFilter?.setValue(inputImage, forKey: kCIInputImageKey) sepiaFilter?.setValue(0.9, forKey: kCIInputIntensityKey) if let outputImage = sepiaFilter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let processedImage = UIImage(cgImage: cgImage) // Display processedImage in the app } }
In the sphere of social media, Core Image can enhance user-generated content. By allowing users to apply filters and effects to their images before sharing, applications can encourage engagement and creativity. Integrating features such as real-time previews of filters can significantly improve user interaction. The following example demonstrates how to create a live filter effect as the user takes a photo:
let cameraOutputImage: CIImage = ... // Get current camera frame as CIImage let liveFilter = CIFilter(name: "CIColorInvert") liveFilter?.setValue(cameraOutputImage, forKey: kCIInputImageKey) if let outputImage = liveFilter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let filteredPreview = UIImage(cgImage: cgImage) // Update UI with filteredPreview } }
Augmented reality applications also benefit from Core Image. By applying filters to the camera feed or detected images, developers can create engaging AR experiences that blend the real and digital worlds seamlessly. For example, detecting faces and applying virtual makeup or effects in real-time can be accomplished using Core Image along with ARKit:
let faceDetectionFilter = CIFilter(name: "CIDetectorFace") faceDetectionFilter?.setValue(cameraOutputImage, forKey: kCIInputImageKey) // Process the detected faces and apply effects if let detectedFaces = faceDetectionFilter?.outputImage { // Apply makeup or effects to detected faces }
Another interesting application is in the sphere of medical imaging. Core Image can be used to process and analyze images, such as X-rays or MRIs. By applying filters to enhance contrast or highlight specific features, developers can assist healthcare professionals in making more informed decisions:
let medicalImage = CIImage(image: UIImage(named: "xray.jpg")!) let contrastFilter = CIFilter(name: "CIContrastEnhance") contrastFilter?.setValue(medicalImage, forKey: kCIInputImageKey) if let outputImage = contrastFilter?.outputImage { let context = CIContext() if let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let enhancedMedicalImage = UIImage(cgImage: cgImage) // Use enhancedMedicalImage for diagnosis } }
Additionally, in the field of gaming, Core Image can be used to create dynamic textures and effects on-the-fly. This can enhance visual fidelity and responsiveness in games. For example, applying post-processing effects like bloom or motion blur can significantly elevate the player’s experience.
Overall, the versatility of Core Image makes it suitable for a wide array of applications in today’s digital landscape. By using its powerful features, Swift developers can create engaging, visually stunning applications that enhance user interaction across various platforms.