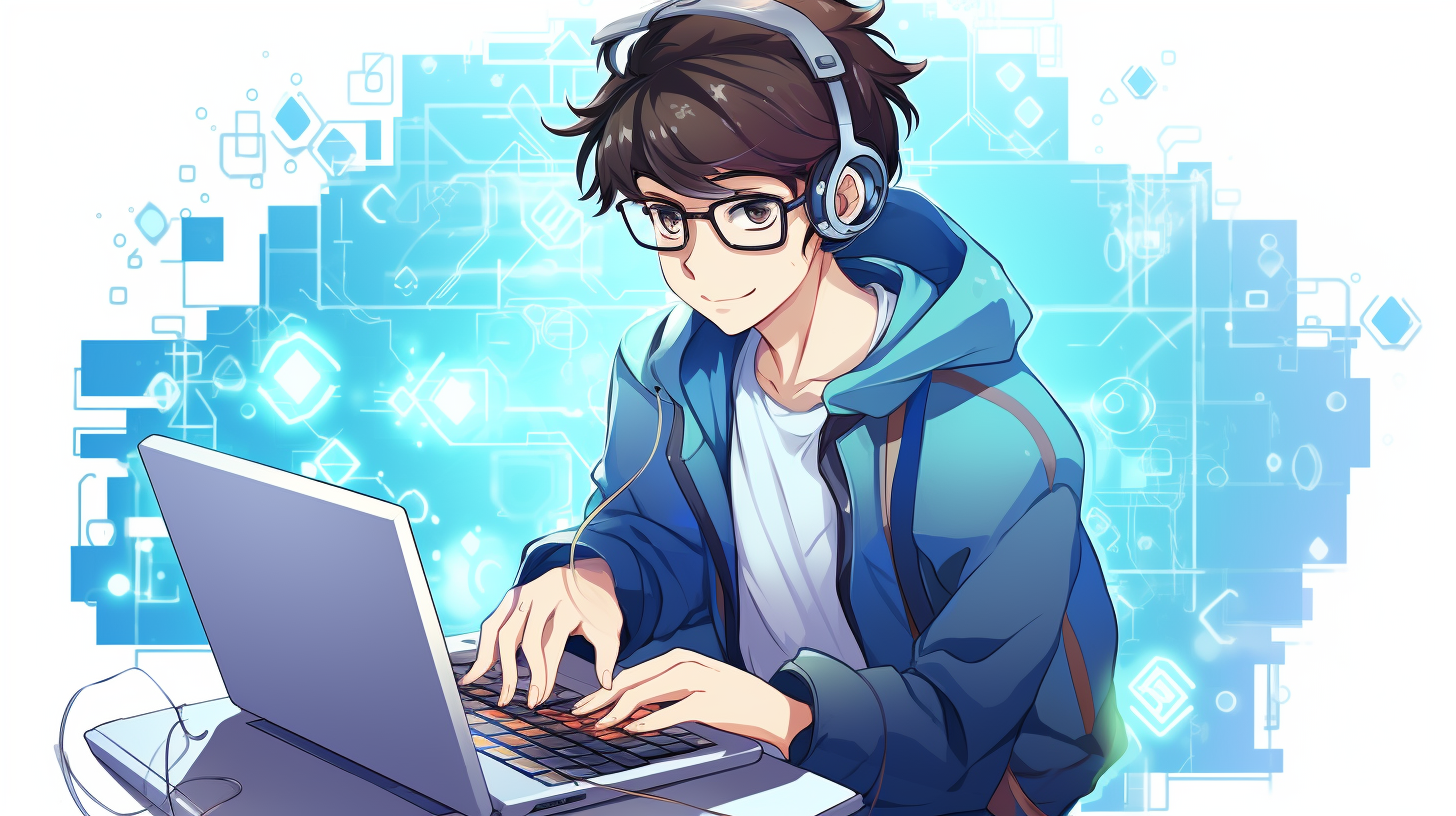
Swift and CoreSpotlight
Core Spotlight is a powerful framework provided by Apple that allows developers to index and search content in their applications, making it discoverable via the system’s search functionality. This means that users can find relevant content from your app directly from the iOS or macOS search interface, enhancing the overall user experience. Understanding how Core Spotlight operates within Swift is important for using its capabilities effectively.
At its core, Core Spotlight revolves around the idea of searchable items. These are the objects that you want to make discoverable. Each item can be indexed with a set of attributes that describe the content and make it searchable. This includes metadata such as titles, descriptions, and unique identifiers. The indexing process transforms your app’s content into a format suitable for search integration, allowing it to be queried seamlessly.
To work with Core Spotlight effectively in Swift, you need to be familiar with several key components:
- This class represents a single item that can be indexed and searched. It encapsulates the necessary data that will be used by the search system.
- This is the interface through which you interact with the Core Spotlight index. It provides methods to add, update, and delete searchable items from the index.
- This protocol allows you to handle events related to the indexing process.
When implementing Core Spotlight in your Swift application, you will primarily deal with the CSSearchableItem
and CSSearchableIndex
classes. Let’s look at a basic example of how to create and index a searchable item:
import CoreSpotlight let searchableItemAttributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) searchableItemAttributeSet.title = "Sample Item" searchableItemAttributeSet.contentDescription = "This is a description of the sample item." searchableItemAttributeSet.thumbnailData = UIImage(named: "thumbnail")?.pngData() let searchableItem = CSSearchableItem(uniqueIdentifier: "com.example.sampleItem", domainIdentifier: "SampleDomain", attributeSet: searchableItemAttributeSet) let searchableIndex = CSSearchableIndex.default() searchableIndex.indexSearchableItems([searchableItem]) { error in if let error = error { print("Error indexing item: (error.localizedDescription)") } else { print("Successfully indexed item.") } }
In this example, we first create an instance of CSSearchableItemAttributeSet
, where we define the properties of the item, including the title and description. We then create a CSSearchableItem
, providing it with a unique identifier and the attribute set. Finally, we use CSSearchableIndex
to index our item and handle any potential errors during the process.
With a solid understanding of how Core Spotlight works within Swift, you can begin to integrate it into your applications, enabling users to find your content conveniently through system-wide searches. The flexibility and power of Core Spotlight can significantly enhance your app’s usability and engagement.
Setting Up Core Spotlight in Your Project
To set up Core Spotlight in your Swift project, you need to ensure that you have the appropriate framework included in your project settings. The following steps will guide you through the process of integrating Core Spotlight smoothly.
First, open your Xcode project and navigate to the project settings. Under the “General” tab, locate the “Frameworks, Libraries, and Embedded Content” section. Here, you will need to add the CoreSpotlight framework. Click on the “+” button, search for “CoreSpotlight,” and add it to your project.
Once the framework is included, you also need to ensure that your app has the appropriate permissions to access the user’s data when creating searchable items. That’s particularly important if your app’s content is user-generated or linked to sensitive information. You will typically define these permissions in your app’s Info.plist file. Add a key for NSPhotoLibraryUsageDescription if your app accesses the photo library, or any other relevant keys based on the data types your app will index.
// Example of setting up permissions in Info.plist NSPhotoLibraryUsageDescription Your app needs access to the photo library to index items.
Next, you should check that your app’s deployment target is set to iOS 9.0 or later, as Core Spotlight was introduced in this version. This can be done in the “General” tab of your project settings under “Deployment Info.”
After ensuring that everything is set up correctly, you can start using Core Spotlight in your Swift application. You may also ponder creating a dedicated class to encapsulate your Core Spotlight logic. This will help maintain clean code and allow for better organization of your indexing operations.
import CoreSpotlight class SearchIndexManager { let searchableIndex = CSSearchableIndex.default() func indexItem(withIdentifier identifier: String, title: String, description: String, thumbnail: UIImage?) { let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = title attributeSet.contentDescription = description if let thumbnailData = thumbnail?.pngData() { attributeSet.thumbnailData = thumbnailData } let item = CSSearchableItem(uniqueIdentifier: identifier, domainIdentifier: "com.example.domain", attributeSet: attributeSet) searchableIndex.indexSearchableItems([item]) { error in if let error = error { print("Error indexing item: (error.localizedDescription)") } else { print("Successfully indexed item with identifier: (identifier)") } } } }
In this example, we created a SearchIndexManager class, which abstracts the indexing process. The indexItem method takes care of creating and indexing a searchable item while handling the Core Spotlight logic internally. This encapsulation allows you to call this method wherever necessary in your application without cluttering your view controllers.
With your project set up correctly for Core Spotlight, you are now ready to move on to creating and indexing searchable items. That is where the real power of Core Spotlight comes into play, enabling your users to discover content seamlessly.
Creating and Indexing Searchable Items
Creating and indexing searchable items in Core Spotlight is a simpler yet fundamental process that allows your application to expose its content to system-wide search functionality. Each searchable item you create is associated with a unique identifier and a set of attributes that describe the content, such as its title, description, and thumbnail image. Let’s dive deeper into how to create these items effectively.
To begin the indexing process, you need to create an instance of CSSearchableItemAttributeSet
, which holds the information about the item you want to index. The properties of this attribute set include metadata that will be displayed in search results, significantly affecting how users interact with your content.
import CoreSpotlight import MobileCoreServices let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = "My First Searchable Item" attributeSet.contentDescription = "This item represents an essential piece of content in my application." attributeSet.thumbnailData = UIImage(named: "thumbnail")?.pngData() let searchableItem = CSSearchableItem(uniqueIdentifier: "com.example.myFirstItem", domainIdentifier: "MyAppDomain", attributeSet: attributeSet)
In this snippet, we first create an instance of CSSearchableItemAttributeSet
and populate it with relevant data. The key here is to ensure that the content description is clear and the title is compelling, as these will be the first points of interaction for users searching for items in your app.
Once you have prepared the attribute set and the searchable item, the next step is to index this item. That’s done through the CSSearchableIndex
class, which manages the items in your application’s search index. The indexing process can be asynchronous, so you should handle any potential errors and confirm successful indexing appropriately.
let searchableIndex = CSSearchableIndex.default() searchableIndex.indexSearchableItems([searchableItem]) { error in if let error = error { print("Indexing error: (error.localizedDescription)") } else { print("Item indexed successfully: (searchableItem.uniqueIdentifier)") } }
This code snippet demonstrates how to index your searchable item using the indexSearchableItems
method. It’s important to note that indexing may fail for various reasons, such as lack of permissions or incorrect attribute settings, so robust error handling is essential.
In addition to indexing single items, you can also batch index multiple items simultaneously, which can improve performance when you have a large set of searchable content. Simply create an array of CSSearchableItem
instances and pass it to the indexSearchableItems
method.
func indexMultipleItems() { var itemsToIndex: [CSSearchableItem] = [] for i in 1...5 { let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = "Item (i)" attributeSet.contentDescription = "Description for item (i)." attributeSet.thumbnailData = UIImage(named: "thumbnail(i)")?.pngData() let item = CSSearchableItem(uniqueIdentifier: "com.example.item(i)", domainIdentifier: "MyAppDomain", attributeSet: attributeSet) itemsToIndex.append(item) } searchableIndex.indexSearchableItems(itemsToIndex) { error in if let error = error { print("Batch indexing error: (error.localizedDescription)") } else { print("All items indexed successfully.") } } }
This example showcases how to create multiple searchable items and index them in a single operation. By managing your indexing strategy efficiently, you can enhance the user experience, making it easier for users to find what they are looking for.
As you continue to implement Core Spotlight in your Swift applications, remember that creating and indexing searchable items is just the beginning. The way you structure and manage your index will play a significant role in how effectively users can discover your content. Properly crafted and indexed items set the stage for a seamless search experience that can substantially increase user engagement with your app.
Configuring Searchable Attributes
When dealing with Core Spotlight, configuring searchable attributes is a critical step that directly influences how your content is presented in the search results. The attributes you assign to your CSSearchableItemAttributeSet not only provide essential metadata but also enhance the discoverability of your items. They’re essentially the breadcrumbs that guide users towards the information they’re seeking.
The CSSearchableItemAttributeSet class allows you to define a variety of attributes that characterize your searchable items. Some of the most important attributes include:
- This is the display name of the item and especially important for capturing the user’s attention during a search.
- A brief overview that provides context about the item. It should be informative yet concise, giving users a good reason to select the item from the search results.
- A visual representation of the item. Including an appealing thumbnail can significantly improve the chances of user engagement.
- An array of keywords that can help refine the search. These keywords are not visible to users but are used internally to match user queries.
To effectively configure these attributes, you need to consider critically about the nature of the content you are indexing. Each attribute should represent the item accurately and appeal to the user’s search intent. Let’s explore how to configure these attributes in a practical example.
import CoreSpotlight import MobileCoreServices let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = "Swift Programming Guide" attributeSet.contentDescription = "A comprehensive guide to mastering Swift programming." attributeSet.thumbnailData = UIImage(named: "swift_thumbnail")?.pngData() attributeSet.keywords = ["Swift", "Programming", "iOS", "Development"] let searchableItem = CSSearchableItem(uniqueIdentifier: "com.example.swiftGuide", domainIdentifier: "Documentation", attributeSet: attributeSet) let searchableIndex = CSSearchableIndex.default() searchableIndex.indexSearchableItems([searchableItem]) { error in if let error = error { print("Error indexing the item: (error.localizedDescription)") } else { print("Successfully indexed the item with unique identifier: (searchableItem.uniqueIdentifier)") } }
In this snippet, we have constructed a CSSearchableItemAttributeSet with a title, description, thumbnail, and keywords. The title succinctly conveys what the item is about, while the content description offers users a glimpse into its significance. Adding a thumbnail enhances the visual allure, making it more likely to attract user attention. Incorporating keywords helps in associating the item with relevant search queries, making it easier for users to discover it.
It’s important to keep in mind that the attributes should be tailored to the target audience and the context in which they will be searching. A well-configured attribute set can lead to better search results and a more satisfying user experience. As you continue to refine your searchable items, consider how each attribute can enhance the visibility and relevance of your content.
Furthermore, keep in mind that the content you provide should be accurate and up to date. Regularly updating your indexed items to reflect changes in the content will ensure that users receive the most relevant information during their searches, maintaining the credibility of your app and keeping users engaged.
Handling Search Results and User Interaction
Handling search results and user interaction in Core Spotlight is pivotal in creating a seamless user experience. When users initiate a search that corresponds to your indexed items, it’s essential to respond appropriately and guide them through a meaningful interaction with your content. This involves not only presenting search results effectively but also defining how users will engage with the items they find.
First, let’s explore how to manage search results. Core Spotlight provides a way to respond to user selections through the CSSearchableIndexDelegate
protocol. By implementing its method searchableIndex(_:reindexingItemsWithIdentifiers:)
, you can react when a user selects a search result. This allows your application to either open the relevant content directly or present additional context based on the user’s selection.
import CoreSpotlight class SearchResultsHandler: NSObject, CSSearchableIndexDelegate { func searchableIndex(_ searchableIndex: CSSearchableIndex, reindexingItemsWithIdentifiers identifiers: [String]) { for identifier in identifiers { // Here you can handle reindexing logic print("Reindexing item with identifier: (identifier)") // Additional logic to fetch updated data for the item } } }
In this example, the SearchResultsHandler
class confirms when a user selects an item from the results. You can expand this method to include logic to fetch updated content and to reindex items if their underlying data has changed. This keeps your search results fresh and accurate, ensuring users receive the best experience possible.
Next, when a user taps on a search result, it is crucial that they’re navigated to the appropriate section of your app. You can achieve this by implementing the openSearchableItem(_:)
method, which you can set up in your app’s delegate or view controller.
func openSearchableItem(_ item: CSSearchableItem) { // Assuming you have a way to identify the view or content to present switch item.uniqueIdentifier { case "com.example.sampleItem": // Navigate to the specific view for the sample item presentSampleItemView() case "com.example.anotherItem": presentAnotherItemView() default: print("Item not recognized.") } }
Within the openSearchableItem
function, you route users to the appropriate content based on the unique identifier of the selected item. This not only enhances user experience but also allows for a more contextual interaction with your app’s content.
Another significant aspect of handling search results is allowing users to filter or refine their searches. This can be achieved through the use of additional search parameters or conditions that will help users locate specific content efficiently. You may ponder implementing a search bar or suggestions based on user input to narrow down results dynamically.
As you progress in managing search results and user interactions, keep in mind that the ultimate goal is to create a fluid experience that feels integrated into the system’s search ecosystem. This involves not only careful management of indexing but also a thoughtful approach to how users engage with the search results. By prioritizing relevant content and ensuring users can easily access it, you foster a user experience that enhances engagement with your application.
Best Practices for Core Spotlight Integration
Integrating Core Spotlight effectively into your Swift applications involves adhering to certain best practices that enhance performance, improve user experience, and ensure the reliability of your search indexing. The principles outlined below serve as a guide for making the most of Core Spotlight’s features while maintaining peak performance within your app.
1. Indexing Only Relevant Content
Before you index content, evaluate its relevance to user search queries. Avoid indexing every piece of content in your app; instead, focus on items that users are likely to search for. This keeps your index streamlined, reducing overhead and improving search performance. For instance, if your app has a large library of articles, think indexing only the ones that have been recently updated or those that have high user engagement.
2. Regular Updates and Maintenance
Maintain the integrity of your searchable items by regularly updating the index. If content changes (e.g., an article is edited or removed), ensure that the corresponding searchable items are updated or deleted from the index. This prevents users from encountering stale results and enhances their search experience. Implement a routine that checks for changes and updates the index accordingly.
func updateItem(withIdentifier identifier: String, newTitle: String, newDescription: String) { let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = newTitle attributeSet.contentDescription = newDescription let item = CSSearchableItem(uniqueIdentifier: identifier, domainIdentifier: "MyAppDomain", attributeSet: attributeSet) let searchableIndex = CSSearchableIndex.default() searchableIndex.indexSearchableItems([item]) { error in if let error = error { print("Error updating item: (error.localizedDescription)") } else { print("Successfully updated item with identifier: (identifier)") } } }
3. Optimize Thumbnail Usage
Thumbnails can significantly enhance the visual allure of search results, but they also impact performance and memory usage. Ensure that thumbnails are appropriately sized; overburdening the index with large images can slow down searches. Use image formats that balance quality and size, and ponder generating thumbnails dynamically if the content is image-heavy.
4. Test Search Performance
Regularly test the performance of your search indexing and retrieval processes. Use Xcode’s profiling tools to analyze how well your app performs during searches and how quickly items are retrieved. This will help identify bottlenecks and areas for improvement. Adjust your indexing strategy based on performance metrics, focusing on reducing latency and improving user experience.
5. User-Centric Keywords
When configuring your searchable items, consider from the user’s perspective. Choose keywords that align with how users are likely to search for content. Avoid overly technical terms unless your target audience is familiar with them. By selecting user-centric keywords, you can improve the chances of users finding the content they seek.
let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = "Learn Swift Programming" attributeSet.contentDescription = "A beginner's guide to Swift programming." attributeSet.keywords = ["Swift", "Programming", "iOS Development", "Tutorial"]
6. Provide Contextual Information
When users interact with searchable items, providing them with context can enrich their experience. Implementing detailed descriptions and additional metadata can help users make informed decisions about which search results to select. Always aim to present the most valuable information upfront to guide user choices.
7. Monitor User Engagement
After deploying your app with Core Spotlight integration, monitor how users engage with the search functionality. Utilize analytics to track which items are being searched for and how often they’re accessed. This data can inform future indexing decisions and content strategy.
By adhering to these best practices, developers can leverage Core Spotlight to create an engaging and efficient search experience that resonates with users, ultimately fostering greater interaction and satisfaction within their applications.