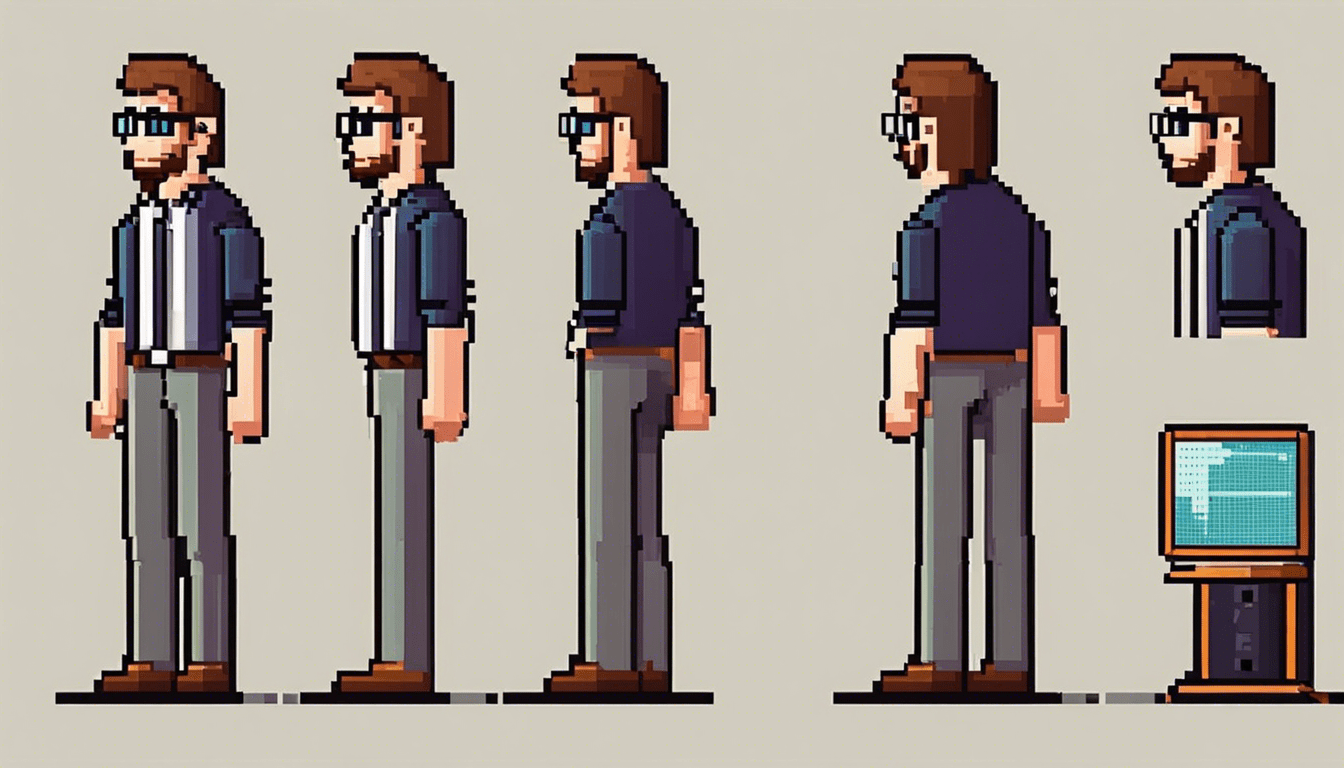
Swift and CoreText
In today’s technology-driven world, text rendering plays an indispensable role in creating interactive user experiences. For those diving into iOS development, dealing with text might be on top of their tutorial list. This article will introduce you to Swift and CoreText, specifically explaining how to deal with text and typography in Swift using the CoreText framework.
Swift is a powerful programming language used in all levels of iOS development. As the successor to Objective-C, it boasts a number of features that make it easier to read and write, simplify memory management and generally speed up the software development process.
On the other hand, CoreText is a font rendering framework in iOS. It provides a flexible system for laying out text and handling fonts. With CoreText, you can perform direct operations on your text in terms of drawing, parsing, and editing. Let’s dive deeper into what it entails!
The prime motive behind CoreText is to give your apps a high-quality typographical disposition. It brings about elaborate typography control to iOS apps and an easy platform to deal with complex scripts (like Arabic or Asian languages).
Time to put your new knowledge into practice!
For example, if we want to display some text in a UILabel and manipulate it using CoreText, our code might look like this:
let label = UILabel() label.text = "Hello World!" let attributedString = NSMutableAttributedString(string: label.text!) let range = NSRange(location: 0, length: attributedString.length) attributedString.addAttribute(.kern, value: 5, range: range) // add kerning attributedString.addAttribute(.foregroundColor, value: UIColor.red, range: range) // change text color label.attributedText = attributedString
This above mentioned Swift code depicts the interaction with CoreText. Here, we create a UILabel, and use an NSMutableAttributedString to handle the actual text. CoreText allows us to add attributes (like kerning and color) to the string, which we then assign back to the label’s ‘attributedText’ property.
One thing you might have noticed is that we’re using a .kern attribute. Kerning refers to the spacing between characters in a piece of text. By adjusting the kerning, we can make our text look more balanced and easier to read.
Next, let’s try to display our text vertically:
let label = UILabel() let attributedString = NSMutableAttributedString(string: "Hello World!") let range = NSRange(location: 0, length: attributedString.length) attributedString.addAttribute(.verticalGlyphForm, value: true, range: range) // vertical text label.attributedText = attributedString
Here we’ve used the .verticalGlyphForm attribute, which causes the text in the specified range to be laid out vertically. Please note that vertical laying doesn’t work with all fonts.
CoreText provides a broad array of other text attributes including ones for controlling font size and face, underline style and color, strikethrough style and color, shadow, and text effect among others.
To conclude, CoreText is a powerful tool for controlling the appearance of text in your apps. It provides detailed control over the layout and presentation of text, which can be invaluable for creating more polished user interfaces. Combined with Swift, it allows for simplified manipulation of text attributes. From applying different font styles to changing text directions, Swift with CoreText delivers an impressive control over typography.