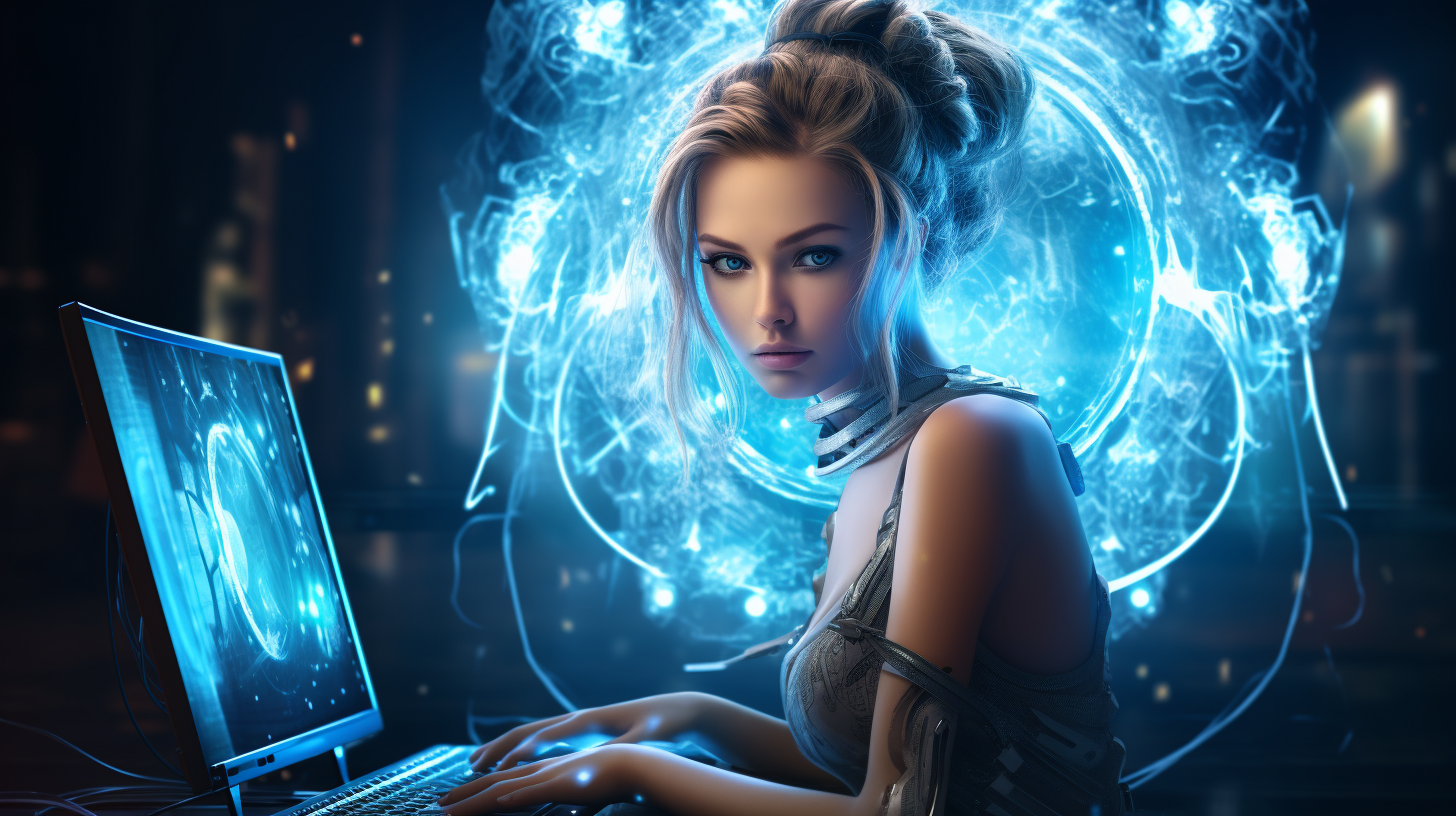
Swift and CryptoKit
CryptoKit is a powerful framework introduced by Apple that enables developers to implement cryptography in their Swift applications with ease and efficiency. It provides a range of cryptographic functionalities, including hashing, encryption, and key management, all designed to leverage the underlying hardware security capabilities of Apple devices.
At its core, CryptoKit abstracts the complexity of cryptographic operations, allowing developers to focus on building secure applications without needing to become cryptography experts. The framework is built on modern cryptographic algorithms and adheres to industry standards, making it a reliable choice for handling sensitive data.
One of the standout features of CryptoKit is its integration with Swift’s type safety and language constructs. This ensures that cryptographic operations are not only simple but also safe from common pitfalls that can lead to vulnerabilities. For instance, the use of strong typing prevents many errors that can occur when dealing with raw byte manipulation.
CryptoKit operates around a few key components: Hashers, Symmetric Key Encryption, Asymmetric Key Encryption, and Key Agreement. Each component is designed to perform specific cryptographic tasks while maintaining a consistent API that seamlessly integrates with Swift’s idioms.
For example, when hashing data, you wouldn’t need to worry about the internal workings of the algorithm; instead, you can focus on the data itself. Here’s how you can create a SHA256 hash of some data:
import CryptoKit let inputData = "Hello, CryptoKit!".data(using: .utf8)! let sha256Hash = SHA256.hash(data: inputData) print("SHA256 Hash: (sha256Hash)')
This simple approach illustrates how CryptoKit allows developers to perform complex operations with just a few lines of code, freeing them from the intricacies of cryptographic implementation.
Additionally, CryptoKit’s design encourages the use of secure coding practices. By providing mechanisms for securely generating cryptographic keys and allowing for secure storage, it helps ensure that sensitive data remains protected against various attack vectors.
CryptoKit is an essential framework for Swift developers looking to implement cryptography in their applications. With its intuitive API, robust functionality, and emphasis on security, it lays a solid foundation for building secure applications that can withstand the challenges posed by today’s digital landscape.
Getting Started with CryptoKit
To get started with CryptoKit, the first step is to ensure that your project has access to the framework. You can easily do this by importing CryptoKit at the beginning of your Swift file. This allows you to utilize all the cryptographic functionalities that CryptoKit offers.
Once you have imported the framework, you can begin to explore its capabilities by creating instances of the various cryptographic components provided by CryptoKit. A common use case is generating a symmetric key for encryption. Here’s how you can generate a secure symmetric key:
import CryptoKit let symmetricKey = SymmetricKey(size: .bits256) print("Generated Symmetric Key: (symmetricKey)")
This code snippet demonstrates the creation of a symmetric key with a size of 256 bits, which is a commonly recommended key length for strong encryption. The generated key is securely stored in memory, and you can use it for any symmetric encryption operations you need to perform.
In addition to generating keys, CryptoKit allows you to perform key management tasks. This includes securely storing keys in the Keychain, which is essential for maintaining the confidentiality and integrity of the keys used in your application. Here’s an example of how you might store a symmetric key securely:
import Security func storeKeyInKeychain(key: SymmetricKey, keyIdentifier: String) { let keyData = key.withUnsafeBytes { Data($0) } let query: [String: Any] = [ kSecClass as String: kSecClassKey, kSecAttrKeyClass as String: kSecAttrKeyClassSymmetric, kSecAttrIsSensitive as String: true, kSecAttrAccessible as String: kSecAttrAccessibleWhenUnlocked, kSecValueData as String: keyData, kSecAttrAccount as String: keyIdentifier ] let status = SecItemAdd(query as CFDictionary, nil) if status == errSecSuccess { print("Key stored successfully.") } else { print("Failed to store key: (status)") } }
In this example, the `storeKeyInKeychain` function takes a symmetric key and a unique identifier as parameters. It converts the key into a data representation and then creates a query dictionary that specifies how and where to store the key in the Keychain. By calling `SecItemAdd`, the key is securely saved, ensuring that it can be retrieved later without exposing it in plaintext.
Another fundamental aspect of using CryptoKit is understanding the various types of cryptographic operations you can perform. For instance, hashing is a simpler operation used for data integrity checks. Hashing transforms input data into a fixed-size string, making it easy to verify whether the data has changed.
To compute a hash using CryptoKit, you can utilize the `SHA256` algorithm as follows:
let inputData = "Swift is awesome!".data(using: .utf8)! let hash = SHA256.hash(data: inputData) print("Hash Value: (hash)")
This example shows how easy it’s to compute a hash of a string input. The resulting hash can be used to verify data integrity, as even the slightest change in the input will produce an entirely different hash value.
As you start using CryptoKit, you’ll find that its design philosophy emphasizes simplicity while ensuring that cryptographic operations are performed correctly and securely. This allows developers to build applications that are not only functional but also resilient against common vulnerabilities associated with cryptography.
Common Cryptographic Operations
Within the scope of cryptographic operations, CryptoKit offers a suite of tools designed to simplify and enhance the security of data handling in Swift applications. While hashing is a foundational operation, there are several other cryptographic techniques that CryptoKit provides, enabling robust security measures for sensitive information.
One major category of operations is symmetric encryption, which allows data to be encrypted and subsequently decrypted using the same key. CryptoKit provides the `AES.GCM` algorithm for authenticated encryption, ensuring data confidentiality and integrity. Here’s how you can encrypt and then decrypt data using symmetric encryption:
import CryptoKit // Generate a symmetric key let symmetricKey = SymmetricKey(size: .bits256) // The plaintext data to be encrypted let plaintext = "This is a secret message.".data(using: .utf8)! // Encrypting the data do { let sealedBox = try AES.GCM.seal(plaintext, using: symmetricKey) print("Encrypted Data: (sealedBox.ciphertext)") // Decrypting the data let decryptedData = try AES.GCM.open(sealedBox, using: symmetricKey) let decryptedMessage = String(data: decryptedData, encoding: .utf8) print("Decrypted Message: (decryptedMessage ?? "Decryption failed")") } catch { print("Encryption/Decryption error: (error)") }
This code snippet illustrates the process of encrypting a plaintext message and then decrypting it back to its original form. It first generates a symmetric key and encrypts the data using the `AES.GCM.seal` method. The resulting `sealedBox` contains the ciphertext, which you can safely transmit or store. Decrypting the ciphertext is equally simpler with `AES.GCM.open`, provided the same symmetric key is used.
In addition to symmetric encryption, CryptoKit supports asymmetric encryption, using public and private key pairs. This is critical for scenarios requiring secure key exchange or digital signatures. For instance, generating a key pair with `Curve25519` for use in secure communications is simple:
import CryptoKit // Generating a key pair let privateKey = Curve25519.KeyAgreement.PrivateKey() let publicKey = privateKey.publicKey print("Private Key: (privateKey)") print("Public Key: (publicKey)")
With asymmetric encryption, the public key can be shared openly, while the private key remains confidential. In practice, you can use this setup to establish a shared secret between parties, allowing for secure communication without the need to share secret keys directly.
Moreover, CryptoKit facilitates key agreement protocols, which are essential for securely sharing keys over an insecure channel. The `KeyAgreement` operations can be succinctly performed as follows:
import CryptoKit // Party A's private and public keys let partyAPrivateKey = Curve25519.KeyAgreement.PrivateKey() let partyAPublicKey = partyAPrivateKey.publicKey // Party B generates its key pair let partyBPrivateKey = Curve25519.KeyAgreement.PrivateKey() let partyBPublicKey = partyBPrivateKey.publicKey // Establishing a shared secret let sharedSecretA = try! partyAPrivateKey.sharedSecret(from: partyBPublicKey) let sharedSecretB = try! partyBPrivateKey.sharedSecret(from: partyAPublicKey) print("Shared Secret A: (sharedSecretA)") print("Shared Secret B: (sharedSecretB)")
In this example, each party generates its private and public keys. Using each other’s public keys, they can compute a shared secret, which can then be used for symmetric encryption. This method ensures that even if the public keys are intercepted, the shared secret remains secure, as only the private keys can derive it.
Through these cryptographic operations, CryptoKit empowers developers to implement advanced security features within their applications. By abstracting the complexities of cryptographic algorithms and providing an intuitive API, it enables a wide range of functionalities—from secure messaging to data integrity verification—while maintaining a focus on safety and best practices.
Implementing Hash Functions
import CryptoKit // Implementing hash functions using CryptoKit // Creating a function to hash a message func hashMessage(message: String) -> SHA256.Digest { let data = message.data(using: .utf8)! let hash = SHA256.hash(data: data) return hash } // Example usage let message = "This is a secure message." let hashedValue = hashMessage(message: message) print("Hashed Value: (hashedValue)")
In the code above, we define a function `hashMessage` that takes a string message as input and returns the SHA256 hash of that message. The function converts the input string to `Data` and calculates its hash using CryptoKit’s `SHA256` class. This encapsulation makes reusing the hashing logic simpler and keeps your cryptographic code organized.
When dealing with hash functions, it’s vital to understand the concept of a hash collision, where two different inputs result in the same hash output. While the probability of this happening is extremely low with strong hash functions like SHA256, it’s essential to choose the right hashing algorithm depending on your application’s specific requirements. For most use cases, including digital signatures and data integrity checks, SHA256 is a solid choice.
For more complex scenarios, CryptoKit provides additional hashing options, such as SHA384 and SHA512, which offer larger hash sizes. When using these algorithms, the implementation remains largely similar, as shown below:
func hashMessageSHA384(message: String) -> SHA384.Digest { let data = message.data(using: .utf8)! let hash = SHA384.hash(data: data) return hash } let messageSHA384 = "This is another secure message." let hashedValueSHA384 = hashMessageSHA384(message: messageSHA384) print("Hashed Value (SHA384): (hashedValueSHA384)")
This example demonstrates how to implement and utilize SHA384 with a similar function structure as before. The ease of switching between different hashing algorithms showcases the flexibility of CryptoKit, allowing developers to quickly adapt their hashing strategy based on the security needs of the application.
Furthermore, hashing is not limited to strings; you can hash any kind of data, making it a versatile tool in your cryptographic toolkit. This capability is particularly useful when working with files or binary data, where direct hashing can ensure data integrity without needing to convert the data into a specific format.
As you continue to implement hash functions within your applications, remember to always think the security implications. Hashes should never be used for storing sensitive data like passwords directly; instead, you should use a technique called salting, which adds a unique value to the input before hashing. This practice helps to mitigate attacks such as rainbow table attacks.
// Example of salting and hashing a password func hashPassword(password: String, salt: String) -> SHA256.Digest { let saltedPassword = password + salt return SHA256.hash(data: saltedPassword.data(using: .utf8)!) } let password = "SuperSecretPassword" let salt = "RandomSaltValue" let hashedPassword = hashPassword(password: password, salt: salt) print("Hashed Password: (hashedPassword)")
By combining the password with a unique salt value, we ensure that even if two users have the same password, their hashes will be different. This is an important part of secure password storage practices and underscores the importance of combining multiple security measures to achieve a robust solution.
Implementing hash functions in Swift using CryptoKit is a simpler yet powerful tool for maintaining data integrity and security. With strong hashing algorithms at your disposal, you can enhance the security posture of your applications while keeping your code clean and maintainable.
Encrypting and Decrypting Data
import CryptoKit // Function to encrypt and decrypt data using symmetric encryption func encryptAndDecrypt(plaintext: String) { // Generate a symmetric key let symmetricKey = SymmetricKey(size: .bits256) // The plaintext data to be encrypted guard let dataToEncrypt = plaintext.data(using: .utf8) else { print("Failed to convert plaintext to Data.") return } do { // Encrypting the data let sealedBox = try AES.GCM.seal(dataToEncrypt, using: symmetricKey) print("Encrypted Data: (sealedBox.ciphertext)") // Decrypting the data let decryptedData = try AES.GCM.open(sealedBox, using: symmetricKey) let decryptedMessage = String(data: decryptedData, encoding: .utf8) print("Decrypted Message: (decryptedMessage ?? "Decryption failed")") } catch { print("Encryption/Decryption error: (error)") } } // Example usage let message = "This is a secret message." encryptAndDecrypt(plaintext: message)
In the code above, the function encryptAndDecrypt demonstrates the process of encrypting a given plaintext string and then decrypting it back to its original form. It begins by generating a symmetric key of 256 bits, which especially important for ensuring the security of the encryption process.
The plaintext string is converted into a Data
object, and then the AES.GCM.seal method is invoked to encrypt this data. The resulting sealedBox contains the ciphertext, which is safe for storage or transmission. Importantly, this method also ensures the integrity of the data, as any modification made to the ciphertext would render the decryption invalid.
When it comes to decryption, the process is just as simpler. The AES.GCM.open method is used to decrypt the sealedBox back into plaintext. The decrypted data is then converted back into a string for easy readability. This highlights the simplicity of CryptoKit, as you can perform complex operations with minimal code.
In scenarios where you require asymmetric encryption, you can utilize public and private key pairs for secure communications. For example, generating key pairs using Curve25519 for secure key exchanges can be achieved as follows:
import CryptoKit // Generate a key pair for asymmetric encryption let privateKey = Curve25519.KeyAgreement.PrivateKey() let publicKey = privateKey.publicKey print("Private Key: (privateKey)") print("Public Key: (publicKey)")
In this snippet, we create a new private key and obtain its corresponding public key. The public key can be shared openly, while the private key remains confidential. This especially important for establishing secure communications without the need to share secret keys directly.
When implementing encryption and decryption in your applications, it’s essential to think the security of your keys. CryptoKit facilitates the use of the Keychain for secure key storage, ensuring that your symmetric and asymmetric keys are protected from unauthorized access.
As you dive deeper into cryptographic operations with CryptoKit, remember that the principles of confidentiality, integrity, and availability are paramount. By using these robust features, you can build applications that not only meet functional requirements but also adhere to high standards of security.
Best Practices for Security in Swift Applications
When developing Swift applications that incorporate cryptographic functionality using CryptoKit, adhering to best security practices is imperative. Even with a robust framework like CryptoKit, the way you implement cryptographic operations can significantly impact your application’s security. Here are some key practices to ensure that your Swift applications remain secure.
1. Secure Key Management
Handling cryptographic keys securely is one of the most crucial aspects of developing secure applications. Always use the Keychain to store sensitive keys. The Keychain provides a secure way to store and retrieve sensitive information, ensuring that your keys are protected against unauthorized access. Here’s a simple demonstration of how to store and retrieve a symmetric key in the Keychain:
import Security import CryptoKit func storeKeyInKeychain(key: SymmetricKey, keyIdentifier: String) { let keyData = key.withUnsafeBytes { Data($0) } let query: [String: Any] = [ kSecClass as String: kSecClassKey, kSecAttrKeyClass as String: kSecAttrKeyClassSymmetric, kSecAttrIsSensitive as String: true, kSecAttrAccessible as String: kSecAttrAccessibleWhenUnlocked, kSecValueData as String: keyData, kSecAttrAccount as String: keyIdentifier ] let status = SecItemAdd(query as CFDictionary, nil) if status == errSecSuccess { print("Key stored successfully.") } else { print("Failed to store key: (status)") } } func retrieveKeyFromKeychain(keyIdentifier: String) -> SymmetricKey? { let query: [String: Any] = [ kSecClass as String: kSecClassKey, kSecAttrAccount as String: keyIdentifier, kSecReturnData as String: kCFBooleanTrue!, kSecMatchLimit as String: kSecMatchLimitOne ] var item: CFTypeRef? let status = SecItemCopyMatching(query as CFDictionary, &item) if status == errSecSuccess, let keyData = item as? Data { return SymmetricKey(data: keyData) } else { print("Failed to retrieve key: (status)") return nil } }
This code snippet demonstrates how to securely store and retrieve a symmetric key from the Keychain, helping prevent exposure of sensitive keys during runtime.
2. Use Strong Cryptographic Algorithms
CryptoKit offers a variety of cryptographic algorithms, but it is essential to choose the right one for your use case. For symmetric encryption, AES is the standard. Always opt for AES with GCM mode, which provides both encryption and integrity verification. For hashing, SHA256 is a solid choice, but ponder using SHA384 or SHA512 for higher security needs. Here’s how you can use AES.GCM:
import CryptoKit func encryptData(plaintext: String, key: SymmetricKey) -> Data? { guard let dataToEncrypt = plaintext.data(using: .utf8) else { return nil } do { let sealedBox = try AES.GCM.seal(dataToEncrypt, using: key) return sealedBox.combined // Combine ciphertext, nonce, and tag } catch { print("Encryption error: (error)") return nil } }
This function encrypts plaintext using the AES.GCM algorithm with a provided symmetric key and returns the combined ciphertext, nonce, and authentication tag.
3. Always Salt Passwords Before Hashing
When dealing with user passwords, always use a salt to defend against rainbow table attacks. The salt should be unique per user and stored alongside the hashed password. Below is an example of hashing a password using SHA256 with salting:
func hashPassword(password: String, salt: String) -> SHA256.Digest { let saltedPassword = password + salt return SHA256.hash(data: saltedPassword.data(using: .utf8)!) }
Incorporating salting into your password hashing routine helps ensure that identical passwords do not yield identical hashes, enhancing security.
4. Regularly Review and Update Dependencies
Dependencies can introduce vulnerabilities, so it’s vital to regularly review and update any third-party libraries or frameworks you utilize in your application. Keep an eye on the official repositories for CryptoKit and other dependencies to ensure that you’re using the latest, most secure versions.
5. Conduct Security Audits and Testing
Perform regular security audits and penetration testing on your applications to identify potential vulnerabilities. Tools such as static analysis tools for Swift can help catch issues early in the development process. Moreover, testing your application under different scenarios can reveal potential weaknesses in your cryptographic implementations.
By following these best practices, you can significantly enhance the security of your Swift applications that utilize CryptoKit. Always prioritize security in your development process, as the stakes are high when it comes to handling sensitive data.