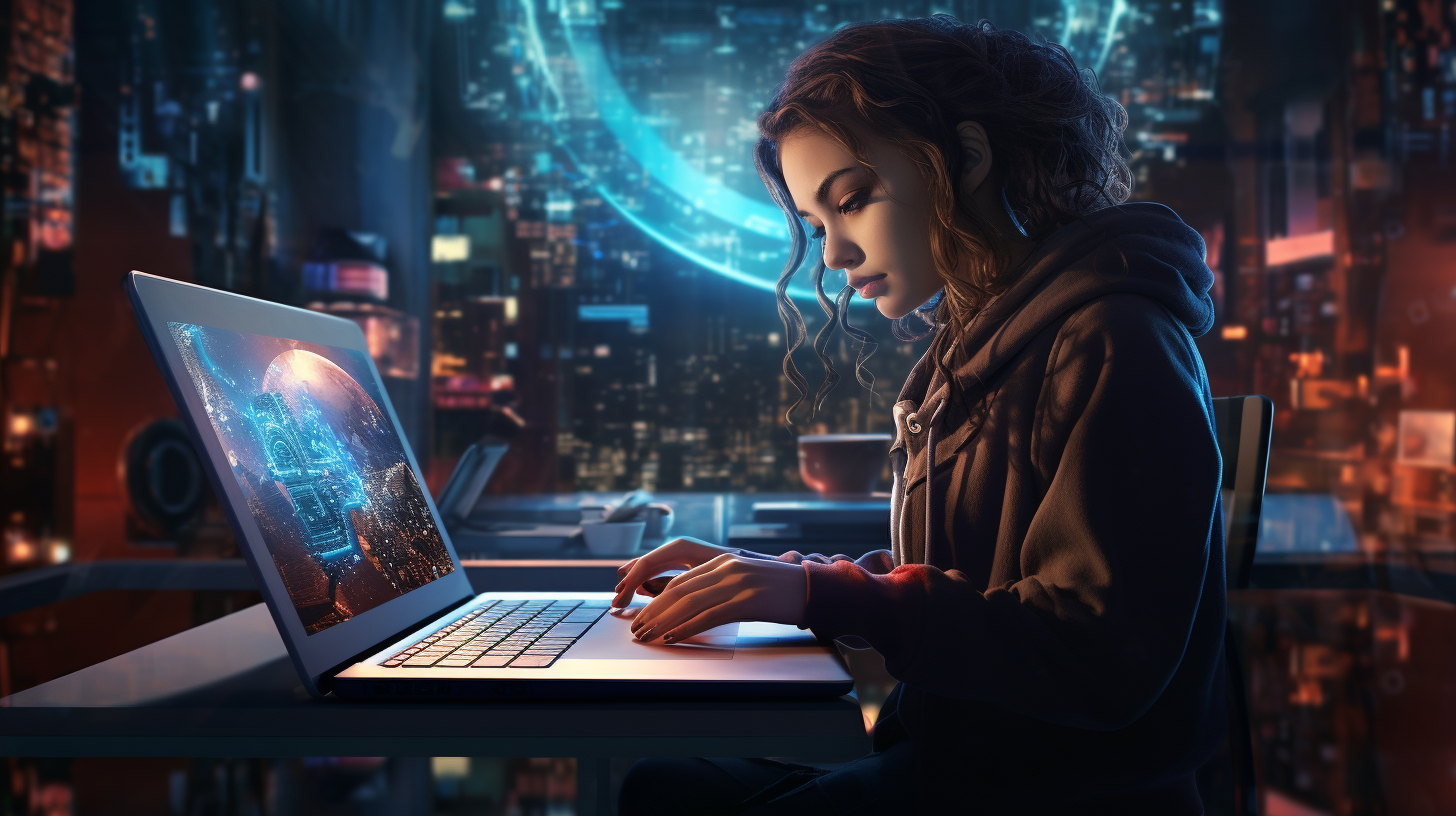
Swift and Data Persistence
In the context of Swift programming, the idea of data persistence is paramount. It enables applications to save their state and retain information across app launches, ensuring a seamless experience for users. When we ponder about data persistence, we are essentially considering the various methods available to store data, retrieve it, and manage it effectively. Swift offers several options, each catering to different needs based on complexity, performance, and data structure.
At its core, data persistence is about maintaining the integrity of information even when the application is not actively running. This involves the use of various storage mechanisms, such as files, databases, and user preferences, each of which serves specific use cases. Understanding the strengths and weaknesses of these methods very important for any Swift developer aiming to create robust applications.
One of the simplest forms of data persistence in Swift is through UserDefaults, which allows developers to store small pieces of data in a key-value format. This is suitable for settings, preferences, and other lightweight data that doesn’t require complex storage solutions.
For more structured data, especially when dealing with complex models, Core Data is a powerful framework provided by Apple. Core Data allows developers to manage an object graph and persist data in a more manageable way, offering a high level of performance and efficiency. The ability to leverage features like data validation, model relationships, and faulting makes it an attractive choice for many applications.
For those who prefer a lightweight database approach, using SQLite directly is also a viable option. SQLite gives developers fine-grained control over their data schema and is incredibly fast, making it suitable for applications that require high-performance data operations. However, it does come with an increased complexity compared to higher-level frameworks like Core Data.
When considering file system access, developers can read and write data to files directly. This method allows for a flexible approach to data storage, where files can be structured in various formats such as JSON or XML. Direct file access is particularly useful for applications that need to handle larger datasets or require specific file formats.
Regardless of the method chosen, it’s essential to consider factors such as data retrieval speed, ease of implementation, and the type of data being managed. Swift developers must weigh these factors carefully to select the most appropriate data persistence strategy that aligns with the application’s goals.
Here’s an example of how you might use UserDefaults for storing a simple user preference:
let userDefaults = UserDefaults.standard userDefaults.set("JohnDoe", forKey: "username")
In this case, we save a username under the key “username”. Retrieving this value is just as straightforward:
if let username = userDefaults.string(forKey: "username") { print("Username: (username)") }
As we delve deeper into the world of data persistence, keep in mind that the choice of method will depend largely on the specific requirements and constraints of your application. Each method comes with its own set of trade-offs, and understanding these will empower you to make informed decisions on how to manage data in your Swift applications.
Core Data Framework Overview
Core Data is a comprehensive framework that provides an object graph management and persistence solution for Swift applications. It abstracts the complexities of data storage, allowing developers to focus more on their application logic rather than the intricacies of data management. Core Data supports a variety of use cases, from managing a simple list of items to complex data models with multiple relationships.
At its core, Core Data operates on the concept of managed objects. These objects represent data entities in your application and are defined by a data model, which outlines the structure of your data, relationships, and attributes. You can ponder of managed objects as the building blocks of your data layer, encapsulating both the data and the behaviors associated with that data.
One of the key components of Core Data is the NSManagedObjectContext, which serves as a workspace for your managed objects. This context is responsible for tracking changes to objects and coordinating the flow of data between your application and the underlying persistent store. You typically create and manipulate managed objects within a managed object context, and when you’re ready to save these changes, you commit them to the persistent store.
Creating a simple Core Data stack involves setting up an NSPersistentContainer, which encapsulates the Core Data stack and simplifies the initialization process. Here’s an example of how to set up a basic Core Data stack:
import UIKit import CoreData class AppDelegate: UIResponder, UIApplicationDelegate { var window: UIWindow? lazy var persistentContainer: NSPersistentContainer = { let container = NSPersistentContainer(name: "ModelName") container.loadPersistentStores(completionHandler: { (storeDescription, error) in if let error = error as NSError? { fatalError("Unresolved error (error), (error.userInfo)") } }) return container }() func saveContext () { let context = persistentContainer.viewContext if context.hasChanges { do { try context.save() } catch { let nserror = error as NSError fatalError("Unresolved error (nserror), (nserror.userInfo)") } } } }
In this code, we create an instance of NSPersistentContainer and load the persistent stores. The saveContext function handles saving changes to the context, ensuring data integrity.
Defining your data model is the next step. You can do this using the Core Data Model Editor in Xcode, where you can create entities, define their attributes, and set up relationships. Once your model is ready, you can create instances of your managed objects:
let context = persistentContainer.viewContext let newEntity = NSEntityDescription.insertNewObject(forEntityName: "EntityName", into: context) newEntity.setValue("Some Value", forKey: "attributeName") do { try context.save() } catch { print("Failed saving") }
This snippet demonstrates how to create a new managed object of a specific entity, set its attributes, and save it to the context. Core Data handles the background processes required to persist this data to disk.
One of the strengths of Core Data is its support for complex relationships between entities. You can easily establish one-to-one, one-to-many, or many-to-many relationships, allowing for a rich data model that reflects the real-world entities you’re trying to represent. Core Data manages these relationships efficiently, handling loading and caching of data as needed.
Moreover, Core Data provides powerful features such as data validation, lazy loading of related data (known as faulting), and undo/redo capabilities. These features enable developers to create robust, high-performance applications that can handle complex data interactions with ease.
Core Data is a versatile and powerful framework for managing data persistence in Swift applications. By using its capabilities, developers can create sophisticated data models that are both performant and maintainable, allowing them to focus on delivering a seamless user experience.
UserDefaults for Simple Data Storage
UserDefaults is an essential component of the Swift ecosystem, providing a simpler mechanism for storing simple data types. It excels in scenarios where the data to be persisted is minimal, such as user preferences or settings. Since UserDefaults is designed to handle small amounts of data, it is not suitable for large datasets or complex data structures; however, its ease of use makes it a popular choice for many applications.
When working with UserDefaults, the data is stored in a persistent dictionary and can be accessed using keys. The supported data types include Strings, Numbers, Booleans, Data, and arrays/dictionaries of these types. This flexibility allows developers to quickly save user preferences or other lightweight data without the overhead of more complex data storage solutions.
To demonstrate the functionality of UserDefaults, think the following example. This snippet highlights how to store and retrieve a user’s preferred theme setting:
let userDefaults = UserDefaults.standard // Saving the user's preferred theme userDefaults.set("Dark", forKey: "themePreference") // Retrieving the user's preferred theme if let themePreference = userDefaults.string(forKey: "themePreference") { print("Preferred Theme: (themePreference)") }
In this example, we first save a string value representing the user’s preferred theme under the key themePreference. When retrieving the value, we use the same key to fetch the stored data.
Another common use case for UserDefaults involves storing user settings such as notifications preference. Below is an illustrative example:
let notificationsEnabled = true userDefaults.set(notificationsEnabled, forKey: "notificationsEnabled") if userDefaults.bool(forKey: "notificationsEnabled") { print("Notifications are enabled.") } else { print("Notifications are disabled.") }
In the code above, we store a Boolean value indicating whether notifications are enabled. Retrieving this setting using the bool(forKey:) method allows us to easily check the user’s preference and respond appropriately in the application.
It’s important to note that UserDefaults is not designed for high-performance data storage. Operations on UserDefaults should be limited to small pieces of data, as excessive use can lead to performance issues. Additionally, when saving large datasets, such as an extensive list of items, consider alternative storage mechanisms like Core Data or direct file access.
When managing data in UserDefaults, it is also wise to implement a fail-safe mechanism. For example, when retrieving values, always provide a fallback to ensure your application behaves correctly even in the absence of stored values:
let defaultTheme = "Light" let currentTheme = userDefaults.string(forKey: "themePreference") ?? defaultTheme print("Current Theme: (currentTheme)")
In this case, if the themePreference key does not exist, the application will fall back to a default value, ensuring a smooth user experience.
UserDefaults serves as a powerful yet simple tool for managing lightweight data persistence in Swift applications. Its usability, combined with the capability to automatically handle data across app launches, makes it an invaluable resource for developers seeking to improve user experience with minimal effort.
File System Access and Management
File system access in Swift provides developers with the ability to directly handle files and their contents, offering a flexible approach to data storage and retrieval. This method is particularly beneficial for applications that need to manage large datasets or work with specific file formats such as JSON, XML, or plain text. By interacting with the file system, developers can create, read, write, and delete files as needed, allowing for a customized data persistence strategy tailored to the application’s requirements.
Swift provides several APIs to manage file system tasks, primarily within the FileManager
class. This class acts as the primary interface for interacting with the file system, allowing developers to perform various operations like file creation, deletion, and path management. When working with files, it’s crucial to understand the common directories available in the app’s sandbox environment, such as the Documents directory, Library directory, and Cache directory, each serving different purposes.
To illustrate file system access, let’s consider how to write and read a simple text file in the Documents directory. First, we’ll create a function to write data to a file:
func writeToFile(fileName: String, content: String) { // Get the path to the Documents directory let fileManager = FileManager.default if let documentsDirectory = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first { let fileURL = documentsDirectory.appendingPathComponent(fileName) // Write the content to the file do { try content.write(to: fileURL, atomically: true, encoding: .utf8) print("File written successfully to (fileURL.path)") } catch { print("Error writing file: (error.localizedDescription)") } } }
In the function above, we first obtain the URL of the Documents directory using FileManager
. We then append the desired file name to this path. The writeToFile
function attempts to write the provided content to the file, handling errors gracefully if the operation fails.
Next, let’s create a function to read the content of the file we just created:
func readFromFile(fileName: String) -> String? { let fileManager = FileManager.default if let documentsDirectory = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first { let fileURL = documentsDirectory.appendingPathComponent(fileName) // Read the content of the file do { let content = try String(contentsOf: fileURL, encoding: .utf8) return content } catch { print("Error reading file: (error.localizedDescription)") } } return nil }
This readFromFile
function again gets the path of the Documents directory and attempts to read the file’s content. If successful, it returns the content as a string; otherwise, it logs an error message.
Now, let’s see how we can use these functions together to write and then read a file:
let fileName = "example.txt" let contentToWrite = "Hello, Swift file system!" // Writing to the file writeToFile(fileName: fileName, content: contentToWrite) // Reading from the file if let readContent = readFromFile(fileName: fileName) { print("Read from file: (readContent)") }
In this example, we first write a message to a file named example.txt
and then immediately read from it. The console will display the confirmation of the file write operation followed by the content retrieved from the file.
When handling files, it is essential to think proper error management and input validation. Additionally, be mindful of the app’s sandbox environment, as trying to access files outside of the allowed directories will result in failures. By using the file system effectively, developers can create powerful data-driven applications that manage large amounts of information efficiently.
For applications that require more advanced file handling, such as reading and writing structured data formats or handling large files, think using frameworks like Codable for JSON, or exploring libraries that facilitate XML and other formats. Direct file access can be a powerful tool in your data persistence toolbox, complementing higher-level frameworks like Core Data or UserDefaults when needed.
Using SQLite with Swift
SQLite is an embedded database engine that provides a lightweight, relational database management system. Its integration into Swift applications is simpler, offering developers the ability to perform complex queries and maintain data integrity without the overhead of a server-based database. This makes SQLite an excellent choice for mobile applications where performance and resource management are critical. To utilize SQLite in a Swift application, you typically use the SQLite.swift library, which provides a type-safe, Swift-friendly API to interact with the SQLite database. First, you'll need to install the SQLite.swift library via Swift Package Manager or CocoaPods. Once that’s done, you can start incorporating SQLite into your application. Let's start with the basics of creating a new SQLite database and defining a table. The following example illustrates how to set up a database and create a simple table for storing user information: ```swift import SQLite // Define the database and table let db: Connection let usersTable = Table("users") let id = Expression("id") let name = Expression("name") let age = Expression("age") do { // Create a connection to the database db = try Connection("path/to/db.sqlite3") // Create the users table try db.run(usersTable.create { t in t.column(id, primaryKey: true) t.column(name) t.column(age) }) print("Table created successfully") } catch { print("Error creating database or table: (error.localizedDescription)") } ``` In this code, we first import the SQLite framework and define a connection to our SQLite database file. We then create a `Table` object for the users table and define its columns using `Expression`. After establishing a database connection, we execute a command to create the table, handling any potential errors. After we have our database and table set up, we can insert data into the table. The following example demonstrates how to insert a new user into the users table: ```swift let insert = usersTable.insert(name <- "Alice", age <- 30) do { try db.run(insert) print("Inserted user successfully") } catch { print("Insertion failed: (error.localizedDescription)") } ``` Here, we create an insert query for the users table, assigning values to the name and age columns. We then execute this insert operation, again handling any errors that may arise. Retrieving data from the SQLite database is just as simpler. The following code snippet shows how to query the users table and print out each user's information: ```swift do { for user in try db.prepare(usersTable) { print("User ID: (user[id]), Name: (user[name]), Age: (user[age])") } } catch { print("Query failed: (error.localizedDescription)") } ``` In this example, we use a `for` loop to iterate over the results of our query on the users table. Each user’s ID, name, and age are accessed through the corresponding expressions. Finally, to ensure data integrity and maintain performance, it’s essential to implement proper error handling and manage transactions when performing multiple operations. Here's how you can do that: ```swift do { try db.transaction { let insert1 = usersTable.insert(name <- "Bob", age <- 25) let insert2 = usersTable.insert(name <- "Charlie", age <- 35) try db.run(insert1) try db.run(insert2) } print("Users inserted in transaction successfully") } catch { print("Transaction failed: (error.localizedDescription)") } ``` In this case, we perform multiple insert operations within a single transaction. If any operation fails, the entire transaction is rolled back, ensuring the database remains consistent. Using SQLite in Swift allows for efficient and flexible data persistence while giving developers the power to implement complex queries and data structures. The SQLite.swift library simplifies interactions with the database, making it an excellent choice for applications that require robust data management without the overhead of more complex frameworks. Whether you’re managing user data, application settings, or any other form of structured data, SQLite provides a strong foundation for achieving high performance and reliability in your Swift applications.
Related Posts
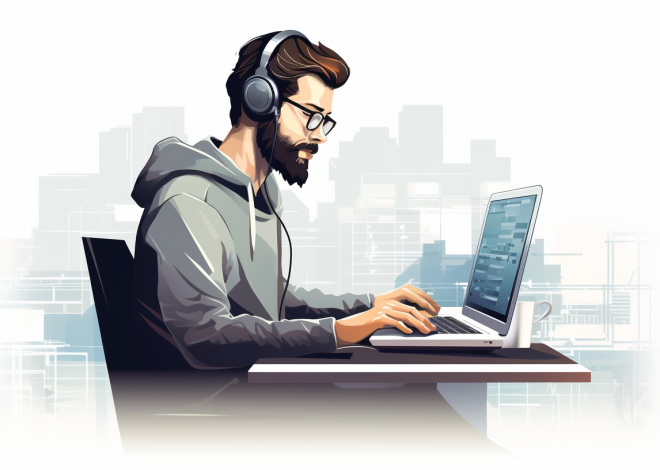
Java Spring Framework: An Introduction
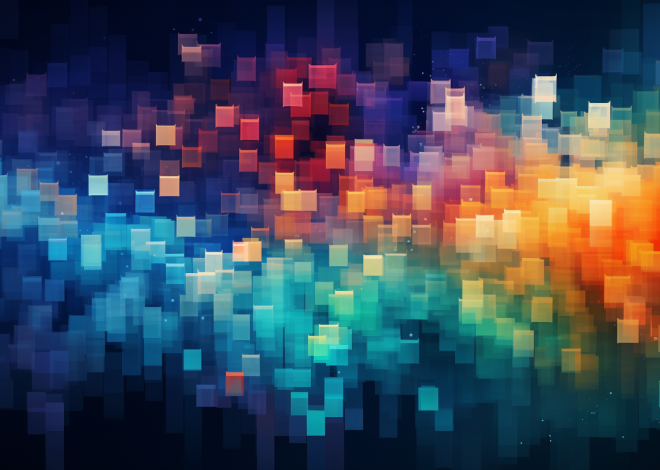
SQL and NoSQL: Working Together
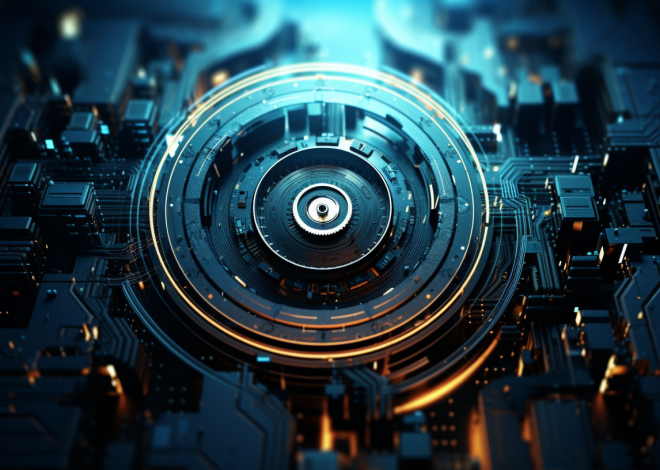