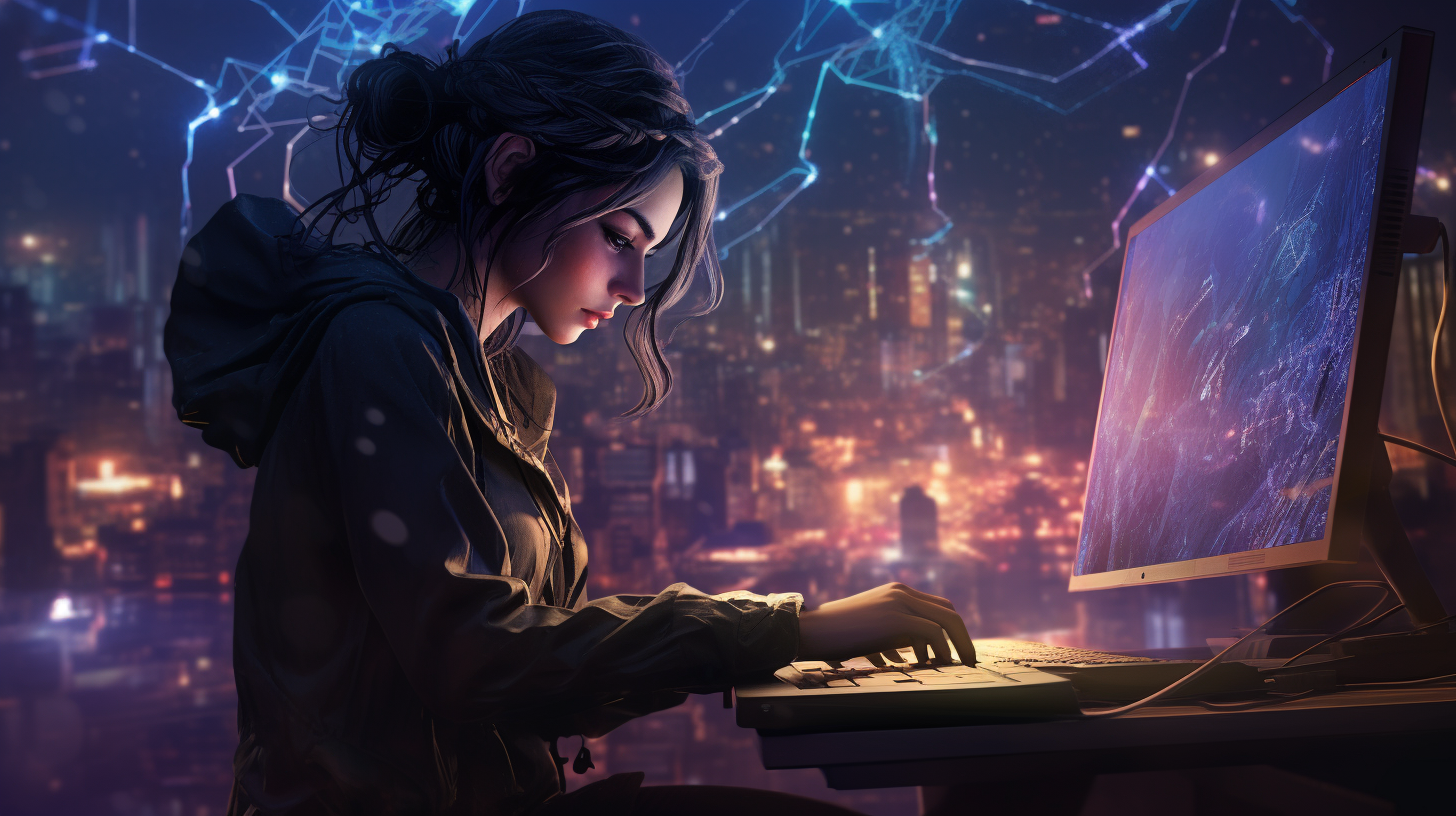
Swift and GameKit
The GameKit framework is a powerful toolset provided by Apple that allows developers to integrate various gaming features into their iOS applications seamlessly. At its core, GameKit facilitates social gaming by enabling features such as leaderboards, achievements, and matchmaking. With GameKit, developers can create engaging multi-player experiences that enhance player interaction and overall enjoyment.
To get started with GameKit, it is crucial to have a firm grasp of its key components. Primarily, the framework consists of:
- This is the online service that connects gamers from around the world, allowing them to compete against each other, compare scores, and track achievements.
- It simplifies the process of finding players for real-time multiplayer games, managing player connections, and ensuring a smooth gaming experience.
- GameKit provides a structured way to implement achievements that reward players for their in-game accomplishments, enhancing motivation and engagement.
- This feature allows players to see how they stack up against others, fostering a competitive spirit among friends and the gaming community.
When developing with GameKit, understanding how to leverage these components will significantly improve the quality of your game. Each element integrates directly with other aspects of iOS development, such as notifications and in-app purchases, creating a richer user experience.
Here’s a simple example of how to authenticate a player with Game Center:
import GameKit func authenticatePlayer() { GKLocalPlayer.local.authenticateHandler = { viewController, error in if let vc = viewController { // Present the Game Center login view controller // Assuming you are in a UIViewController self.present(vc, animated: true, completion: nil) } else if GKLocalPlayer.local.isAuthenticated { // Player is authenticated print("Player authenticated: (GKLocalPlayer.local.displayName)") } else if let error = error { print("Error during Game Center authentication: (error.localizedDescription)") } } }
With this foundation, developers can begin to explore deeper functionalities, such as integrating real-time multiplayer features or managing complex game states. The more you understand GameKit, the more effectively you can create immersive and engaging gaming experiences.
Setting Up Your Swift Project for GameKit
Setting up your Swift project to use GameKit involves several key steps that ensure you’re ready to harness the full power of the framework. Here’s a concise guide to get you started.
1. Enable Game Center in Your App ID:
First, navigate to your Apple Developer account and ensure your App ID has Game Center enabled. This step is essential as Game Center functionality is tied to your App ID, and without it, you won’t be able to use its features.
2. Configure Your Xcode Project:
Open your Xcode project and select your target. Under the “Signing & Capabilities” tab, add the Game Center capability. This automatically configures necessary entitlements in your project.
3. Import the GameKit Framework:
In order to use GameKit features, you need to import the framework into your Swift files. Here’s how you do that:
import GameKit
4. Setting Up the Game Environment:
Initializing the GameKit environment is an important step. Begin by ensuring the local player is authenticated before you attempt to access any Game Center features. Here’s a simple setup:
func setupGameKit() { GKLocalPlayer.local.authenticateHandler = { viewController, error in if let vc = viewController { // Present the Game Center login view controller DispatchQueue.main.async { self.present(vc, animated: true, completion: nil) } } else if GKLocalPlayer.local.isAuthenticated { print("Player authenticated: (GKLocalPlayer.local.displayName)") } else if let error = error { print("Game Center authentication error: (error.localizedDescription)") } } }
5. Testing Your Game with Game Center:
To test Game Center features, you need to set up a sandbox account. This allows you to verify that your integration works correctly without affecting production data. You can create a sandbox account in your Apple Developer account and use it for testing.
6. Use Xcode’s Game Center Testing Features:
Xcode provides built-in tools for testing Game Center functions. You can simulate different game states and user scenarios right from the IDE. This helps in ensuring that your game behaves as expected in various conditions.
By following these steps, you establish a solid foundation for integrating GameKit into your Swift project. As you advance to implementing Game Center features, remember that each component you introduce builds on this base, enabling you to create a rich and engaging gaming experience.
Implementing Game Center Features
Implementing Game Center features in your Swift application involves using the capabilities of GameKit to improve your game’s social experience. Game Center allows players to track their progress, compare scores, and engage in friendly competition through achievements and leaderboards. To effectively implement these features, follow best practices to ensure a smooth integration.
First, let’s dive into how to create and manage achievements. Achievements are a fantastic way to motivate players by rewarding them for completing specific tasks or reaching certain milestones in your game. Here’s how to define and report achievements:
func reportAchievement(identifier: String, percentComplete: Double) { let achievement = GKAchievement(identifier: identifier) achievement.percentComplete = percentComplete GKAchievement.report([achievement]) { error in if let error = error { print("Error reporting achievement: (error.localizedDescription)") } else { print("Achievement (identifier) reported successfully.") } } }
This function creates a new achievement instance and sets its completion percentage. After reporting it, you can verify whether the operation succeeded or failed by checking for errors.
Next, to display achievements to players, you can utilize the Game Center UI. To present the achievements view, use the following code:
func showAchievements() { let gcViewController = GKGameCenterViewController() gcViewController.gameCenterDelegate = self gcViewController.viewState = .achievements self.present(gcViewController, animated: true, completion: nil) } extension YourViewController: GKGameCenterControllerDelegate { func gameCenterViewControllerDidFinish(_ viewController: GKGameCenterViewController) { dismiss(animated: true, completion: nil) } }
Here, we create a `GKGameCenterViewController`, set its delegate, and present it to the user. Don’t forget to conform to the `GKGameCenterControllerDelegate` protocol to handle dismissal of the view controller.
Next, let’s implement leaderboards. Leaderboards provide a competitive edge by allowing players to compare their scores. To report a score to a leaderboard, you can use the following function:
func reportScore(score: Int64, forLeaderboardID leaderboardID: String) { let scoreReporter = GKScore(leaderboardIdentifier: leaderboardID) scoreReporter.value = score GKScore.report([scoreReporter]) { error in if let error = error { print("Error reporting score: (error.localizedDescription)") } else { print("Score (score) reported to leaderboard (leaderboardID) successfully.") } } }
With this function, you can report the player’s score to the specified leaderboard. The completion handler allows you to confirm the success or failure of the operation, helping you manage user feedback effectively.
To present the leaderboard interface to the players, you can use a similar approach to achievements:
func showLeaderboard(leaderboardID: String) { let gcViewController = GKGameCenterViewController() gcViewController.gameCenterDelegate = self gcViewController.viewState = .leaderboards gcViewController.leaderboardIdentifier = leaderboardID self.present(gcViewController, animated: true, completion: nil) }
By following these steps, you can successfully implement Game Center features in your Swift application, providing players with a rich, engaging environment that encourages competition and achievement. The key to a good Game Center implementation lies in seamlessly integrating these features into your gameplay, enhancing the overall user experience.
Integrating Real-Time Multiplayer
Integrating real-time multiplayer features using GameKit in your Swift application opens up a new realm of interactive gameplay, allowing players to engage with each other seamlessly. Real-time multiplayer is ideal for games that require immediate feedback and fast-paced action, such as racing games or battle arenas. To set up real-time multiplayer, you’ll need to grasp the core components of the GameKit framework that facilitate player matchmaking, communication, and session management.
The process begins with matchmaking. GameKit provides a matchmaking API that allows players to find and connect with others based on specific criteria. Here’s how to initiate a matchmaking session:
import GameKit var match: GKMatch? func startMatchmaking() { let request = GKMatchRequest() request.minPlayers = 2 request.maxPlayers = 4 request.playerGroup = 0 // Optional: specify a player group GKLocalPlayer.local.authenticateHandler = { viewController, error in if GKLocalPlayer.local.isAuthenticated { GKMatchmaker.shared().findMatch(for: request) { match, error in if let error = error { print("Error finding match: (error.localizedDescription)") return } guard let match = match else { return } self.match = match self.match?.delegate = self // Set delegate to handle match events print("Match found with (match.expectedPlayerCount) players waiting") } } else if let error = error { print("Authentication error: (error.localizedDescription)") } } }
In this code, we create a `GKMatchRequest`, specifying the minimum and maximum number of players. The matchmaker then searches for a suitable match, and upon finding one, we set the match’s delegate to handle updates and events.
Once players are connected, it’s crucial to manage communication effectively. You can send data between players using the `sendData` method. This method ensures that your game can relay real-time information, such as player movements or game state updates. Here’s a basic example of how to send data:
func sendData(data: Data) { do { try match?.send(data, toAllPlayers: .reliable) print("Data sent to all players") } catch { print("Error sending data: (error.localizedDescription)") } }
In this example, we attempt to send data to all connected players. The use of `.reliable` ensures that the data is delivered, although it may introduce latency, which is acceptable for most game states.
Receiving data is as important as sending it. You’ll need to implement the `GKMatchDelegate` protocol, specifically the `match(_:didReceive:fromRemotePlayer:)` method, to respond to incoming data:
extension YourViewController: GKMatchDelegate { func match(_ match: GKMatch, didReceive data: Data, fromRemotePlayer player: GKPlayer) { // Handle incoming data print("Received data from (player.displayName)") // Process the data (e.g., update player position) } }
In this extension, you can handle any received data, updating game state or player positions as necessary. This allows your game to remain synchronized across all connected players.
Finally, managing the match lifecycle is critical. You should handle player disconnections, match end events, and errors gracefully. Implementing the `match(_:player:didChange:)` method will help you track player connections:
func match(_ match: GKMatch, player: GKPlayer, didChange state: GKPlayerConnectionState) { switch state { case .stateConnected: print("(player.displayName) connected") case .stateDisconnected: print("(player.displayName) disconnected") // Handle player disconnection (e.g., notify remaining players, end match) default: break } }
With these components in place, you’ll have a solid foundation for real-time multiplayer gaming using GameKit in Swift. By focusing on effective matchmaking, reliable communication, and proper management of the match lifecycle, you can create a compelling multiplayer experience that keeps players engaged and coming back for more.
Using GameKit for Achievements and Leaderboards
Using GameKit to implement achievements and leaderboards in your Swift game can significantly enhance player engagement and retention. These features allow players to track their accomplishments and compare their performance with others, fostering a competitive atmosphere that drives continued play. Below, we’ll delve into how to effectively use GameKit for these features, including examples of how to manage achievements and leaderboards within your application.
Achievements
Achievements motivate players by rewarding them for reaching specific milestones or completing particular tasks within your game. To define and report achievements, you must first create them in App Store Connect, where you specify an identifier for each achievement. Once created, you can implement the following Swift function to report an achievement when a player reaches a certain milestone:
func reportAchievement(identifier: String, percentComplete: Double) { let achievement = GKAchievement(identifier: identifier) achievement.percentComplete = percentComplete GKAchievement.report([achievement]) { error in if let error = error { print("Error reporting achievement: (error.localizedDescription)") } else { print("Achievement (identifier) reported successfully.") } } }
In this function, we create a new achievement instance using its identifier and set the completion percentage. The `report` method sends the achievement data to Game Center, allowing players to see their progress. Always ensure that you handle potential errors gracefully, as this will improve the user experience.
Displaying Achievements
To display the achievements view to players, you can use the following code snippet that presents the Game Center interface:
func showAchievements() { let gcViewController = GKGameCenterViewController() gcViewController.gameCenterDelegate = self gcViewController.viewState = .achievements self.present(gcViewController, animated: true, completion: nil) } extension YourViewController: GKGameCenterControllerDelegate { func gameCenterViewControllerDidFinish(_ viewController: GKGameCenterViewController) { dismiss(animated: true, completion: nil) } }
In this code, we initialize a `GKGameCenterViewController`, define its view state to show achievements, and present it to the user. Implementing the `GKGameCenterControllerDelegate` allows for smooth dismissal of the view controller when the player is done.
Leaderboards
Leaderboards are essential for enhancing competitive gameplay, enabling players to see how they rank against others. Similar to achievements, you must first create leaderboards in App Store Connect. Once set up, use the following function to report a player’s score:
func reportScore(score: Int64, forLeaderboardID leaderboardID: String) { let scoreReporter = GKScore(leaderboardIdentifier: leaderboardID) scoreReporter.value = score GKScore.report([scoreReporter]) { error in if let error = error { print("Error reporting score: (error.localizedDescription)") } else { print("Score (score) reported to leaderboard (leaderboardID) successfully.") } } }
This function creates a new score instance, assigns the score value, and reports it to the specified leaderboard. Similar to achievements, ensure that you handle any errors that may arise during this process.
Displaying Leaderboards
To present the leaderboard interface, you can use a code structure similar to that of achievements:
func showLeaderboard(leaderboardID: String) { let gcViewController = GKGameCenterViewController() gcViewController.gameCenterDelegate = self gcViewController.viewState = .leaderboards gcViewController.leaderboardIdentifier = leaderboardID self.present(gcViewController, animated: true, completion: nil) }
Here we set the `viewState` to display leaderboards and specify the particular leaderboard identifier. This presents a unified view for players to gauge their performance against others.
By effectively implementing achievements and leaderboards using GameKit, you not only enhance player motivation but also create a vibrant gaming community where competition thrives. The seamless integration of these features will ultimately lead to a more rewarding experience for players, increasing their investment in your game.
Best Practices for Game Development with Swift and GameKit
When developing games with Swift and the GameKit framework, adhering to best practices is vital for creating a polished, enjoyable user experience. These practices not only enhance the game’s functionality but also streamline the development process, making it easier to manage complexities as they arise. Here’s a detailed look at some essential best practices to follow when using GameKit in your game development.
1. Authentication Management
Player authentication is the gateway to using Game Center features. Always ensure that you check the player’s authentication status before attempting to access any Game Center functionalities. Using the `GKLocalPlayer`’s `authenticateHandler` is important for managing this process. Think implementing a robust error-handling strategy to address various authentication scenarios:
func authenticatePlayer() { GKLocalPlayer.local.authenticateHandler = { viewController, error in if let vc = viewController { // Present the Game Center login view controller DispatchQueue.main.async { self.present(vc, animated: true, completion: nil) } } else if GKLocalPlayer.local.isAuthenticated { print("Player authenticated: (GKLocalPlayer.local.displayName)") } else if let error = error { print("Authentication error: (error.localizedDescription)") } } }
By managing player authentication proactively, you can prevent unnecessary errors when attempting to call GameKit services.
2. Efficient Data Handling
When dealing with real-time multiplayer games, optimizing data handling becomes critical. Use compact data structures to minimize bandwidth and ensure timely communication. The `sendData` method should be used judiciously. Prefer using `.unreliable` for non-critical data to enhance performance:
func sendData(data: Data) { do { try match?.send(data, toAllPlayers: .unreliable) print("Non-critical data sent") } catch { print("Error sending data: (error.localizedDescription)") } }
Remember that while `.reliable` guarantees delivery, it may introduce latency, which is often unacceptable in fast-paced gameplay.
3. Utilize Delegates for Match Handling
Implement the `GKMatchDelegate` protocol thoroughly to handle match events effectively. This includes player connection states and incoming data. By compartmentalizing your handling of these events, you can keep your game logic clean and responsive:
extension YourViewController: GKMatchDelegate { func match(_ match: GKMatch, player: GKPlayer, didChange state: GKPlayerConnectionState) { switch state { case .stateConnected: print("(player.displayName) connected") case .stateDisconnected: print("(player.displayName) disconnected") // Handle disconnection appropriately default: break } } }
This approach allows your game to dynamically respond to player actions and maintain a coherent game state.
4. Regularly Update and Report Achievements
To keep players engaged, regularly update and report achievements. Create a system that checks for achievement progress after significant game events. Use a dedicated function to handle reporting, ensuring that you handle errors gracefully to maintain a seamless experience:
func reportAchievement(identifier: String, percentComplete: Double) { let achievement = GKAchievement(identifier: identifier) achievement.percentComplete = percentComplete GKAchievement.report([achievement]) { error in if let error = error { print("Error reporting achievement: (error.localizedDescription)") } else { print("Achievement (identifier) reported successfully.") } } }
This structure allows you to keep track of player progress and gives timely feedback, enhancing motivation to continue playing.
5. Implement Testing and Sandbox Environments
Before you release your game, extensively test all GameKit features in a sandbox environment. Apple provides a sandbox account for Game Center, which allows you to simulate real user interactions without risking your live environment. Test achievements, leaderboards, matchmaking, and multiplayer functionality to ensure everything works as intended:
Using Xcode’s Game Center testing tools is highly recommended. They allow you to simulate various user scenarios and game states, ensuring your implementation is robust and error-free.
6. Keep the User Experience in Mind
Finally, always prioritize the user experience. Ensure that Game Center integrations do not interrupt gameplay unnecessarily. Present Game Center UI elements only when they enhance the gaming experience, such as after a match concludes or when a player achieves a noteworthy milestone. This keeps players engaged and minimizes disruptions:
func showLeaderboard(leaderboardID: String) { let gcViewController = GKGameCenterViewController() gcViewController.gameCenterDelegate = self gcViewController.viewState = .leaderboards gcViewController.leaderboardIdentifier = leaderboardID self.present(gcViewController, animated: true, completion: nil) }
By embedding these best practices into your game development process, you not only enhance the functionality of your application but also create a more enjoyable experience for players. The combination of technical proficiency and user-centric design will ultimately lead to greater player retention and satisfaction.