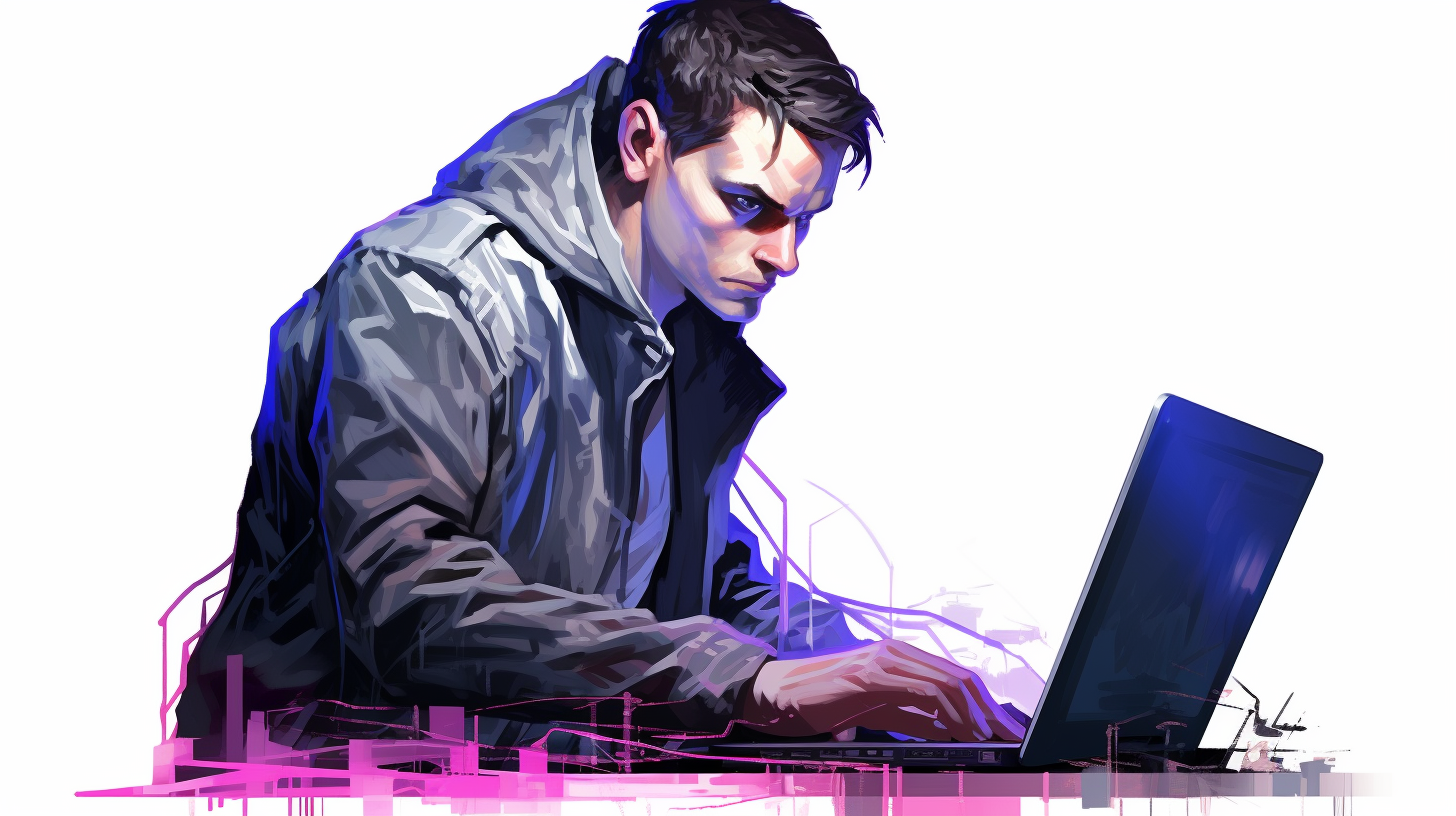
Swift and Location Services
The Core Location framework is a powerful tool provided by Apple that enables developers to access the geographical location and orientation of a device. By using this framework, apps can provide context-aware features, enhancing user experience based on their location. Understanding how Core Location operates is essential for effective implementation.
At its core, the Core Location framework provides a simple interface to access location data, but it also offers rich capabilities for more advanced use cases. Core Location operates using a combination of device GPS, Wi-Fi, and cellular data to determine the device’s location. This multi-faceted approach allows for greater accuracy and responsiveness even in challenging environments.
To begin using Core Location, developers must import the framework into their Swift project:
import CoreLocation
Next, creating an instance of the CLLocationManager
class is essential as this object is responsible for configuring the location services:
let locationManager = CLLocationManager()
The CLLocationManager
provides a variety of methods and properties to manage location updates. For instance, to start receiving location updates, you would typically call:
locationManager.startUpdatingLocation()
However, before this can happen, there are a few critical concepts to understand:
Accuracy: You can specify the desired accuracy of the location services. High accuracy can drain battery life quicker, so it’s important to strike a balance:
locationManager.desiredAccuracy = kCLLocationAccuracyBest
Location Services Authorization: To access location data, the app must have user permission. This is accomplished through a series of request methods, which we will delve into in the subsequent sections.
Location Updates: The framework provides methods to monitor location changes, which can be done continuously or based on significant location changes. This flexibility allows apps to optimize performance based on their specific needs.
With the fundamental concepts in place, you can start building location-aware applications that enhance interactivity and engagement for users. The Core Location framework is not just about tracking a device’s coordinates; it’s about providing context and relevance based on where the user is and what they’re doing.
Setting Up Location Permissions
Before you can begin to utilize the Core Location framework’s capabilities, it is imperative to set up location permissions correctly. That is a critical step that directly influences the user experience and the functionality of your application. Apple has implemented a robust permission model to ensure that users maintain control over their location data. As such, developers must explicitly request access to location services, and the app must handle the responses appropriately.
To request location permissions, you first need to declare your intent in the app’s Info.plist
file. There are two primary keys that can be used for location services:
- This key is required if your app requests location data only while it is in the foreground.
- Use this key when your app needs to access location data in the background as well.
The value for each key should be a string that describes to the user why your app needs access to their location. This transparency is important for user trust.
Once the appropriate keys are added to the Info.plist, you can request location permissions in your code. Begin by checking the current authorization status using the CLLocationManager.authorizationStatus()
method:
let authorizationStatus = CLLocationManager.authorizationStatus()
Based on this status, you can prompt the user for permission if access has not yet been granted. Here’s how to request permission when the app is in use:
if authorizationStatus == .notDetermined { locationManager.requestWhenInUseAuthorization() }
If your application requires continuous location tracking, ponder requesting always authorization:
if authorizationStatus == .notDetermined { locationManager.requestAlwaysAuthorization() }
After requesting permission, it especially important to handle the response in your CLLocationManagerDelegate
method. This will inform you whether the user granted or denied permission. Implement the delegate method:
func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) { switch status { case .authorizedWhenInUse: // Start updating location locationManager.startUpdatingLocation() case .authorizedAlways: // Start updating location locationManager.startUpdatingLocation() case .denied, .restricted: // Handle the case when permission is denied print("Location access denied.") case .notDetermined: break // Permission has not been requested yet @unknown default: break // Handle any future cases } }
By managing location permissions responsibly and respecting user privacy, you set a solid foundation for your app’s location-based features. The user’s comfort and consent are paramount, and the way you handle permissions can significantly affect how they perceive your application. By carefully architecting this part of your app, you will not only gain access to valuable location data but also build trust with your users, enhancing their overall experience.
Implementing Location Tracking
Once you have set up the necessary location permissions, the next step is to implement location tracking effectively. This involves not only starting to receive location updates but also managing those updates to suit your app’s specific needs. The CLLocationManager provides several ways to monitor location changes, allowing for a flexible integration into your app’s functionality.
To initiate continuous location tracking, you would typically begin by configuring your CLLocationManager instance to start updating the user’s location as soon as you have the required permissions. The most basic setup for receiving location updates can be achieved using the following code:
locationManager.delegate = self locationManager.startUpdatingLocation()
In this snippet, you set the delegate of your location manager to the current class, allowing it to receive location updates. The location manager will then invoke delegate methods to notify you about location updates, which can be processed as needed.
Implementing the delegate method very important to handle the incoming location data. The method locationManager(_:didUpdateLocations:)
is your go-to point for receiving the latest location updates:
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) { guard let location = locations.last else { return } // Use the location data print("Updated location: (location.coordinate.latitude), (location.coordinate.longitude)") }
In this method, you can access the most recent location from the locations
array. Typically, you will want to work with the last location, as it represents the most current data. You can leverage this information to update your app’s UI or perform any necessary actions based on the user’s location.
For scenarios where you only need to track significant changes in location, you can optimize performance by using the startMonitoringSignificantLocationChanges()
method. This method conserves battery life, as it does not require constant location updates:
locationManager.startMonitoringSignificantLocationChanges()
This approach is particularly useful for applications that do not require real-time location updates but still need to respond to user movement, such as a fitness app that tracks runs or walks.
It is also important to handle the stop of location updates appropriately. If your application no longer needs location data, you should call:
locationManager.stopUpdatingLocation()
By pausing location updates, you help conserve the device’s battery life, which is an important consideration for user experience.
To summarize, implementing location tracking with Core Location involves configuring your location manager, properly handling updates in the delegate methods, and optimizing location updates based on your app’s requirements. By following these steps, you can create a responsive and efficient location-aware application that enhances user engagement through contextual awareness.
Handling Location Updates
Handling location updates effectively very important for creating a responsive and easy to use application. In the Core Location framework, the CLLocationManager is at the heart of managing location updates, providing a robust interface for accessing location data. This section will delve into how to properly handle these updates to ensure that your app responds gracefully to changes in user location.
Once you have initiated location tracking by calling startUpdatingLocation()
, the CLLocationManager begins to deliver location updates to its delegate. It is essential to implement the locationManager(_:didUpdateLocations:)
delegate method, as that is where you’ll receive the latest location data:
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) { guard let location = locations.last else { return } let latitude = location.coordinate.latitude let longitude = location.coordinate.longitude print("Updated location: (latitude), (longitude)") // You can also perform actions based on location here. }
In this method, you access the most recent location from the locations
array. This allows you to perform various actions, such as updating the UI or triggering other functionalities based on the user’s current position. For instance, you might update a map view or fetch nearby points of interest, depending on your application’s needs.
Moreover, the Core Location framework provides the flexibility to adjust the frequency of these updates. You can modify the distanceFilter
property of your location manager to control how often the method is called. By setting a value greater than zero, you can specify the minimum distance (in meters) that the device must move before an update is sent. That is particularly useful to conserve battery life:
locationManager.distanceFilter = 50 // Update only when the device moves by 50 meters
Another effective way to manage location updates is to utilize startMonitoringSignificantLocationChanges()
. This method optimizes battery usage by only providing updates when significant changes in location occur, which is ideal for applications like fitness trackers where constant updates are unnecessary:
locationManager.startMonitoringSignificantLocationChanges()
When the user no longer requires location updates, be sure to stop them to conserve resources and battery life. This can be done using the stopUpdatingLocation()
method:
locationManager.stopUpdatingLocation()
Handling errors in location updates is also an essential aspect of your implementation. You should implement the locationManager(_:didFailWithError:)
method to respond to any issues that might arise, such as denied permissions or location services being disabled:
func locationManager(_ manager: CLLocationManager, didFailWithError error: Error) { print("Failed to find user's location: (error.localizedDescription)") }
By managing location updates thoughtfully and addressing potential errors, you can create a seamless user experience that leverages the power of location awareness in your application. Remember, the goal is to provide relevant context to the user without draining their device’s resources unnecessarily. Balancing real-time data needs with efficiency is key to an effective implementation.
Geofencing and Region Monitoring
Geofencing is a powerful feature in the Core Location framework that allows developers to monitor specific geographical regions and trigger actions when a device enters or exits those regions. This capability is particularly useful for applications that require context-aware notifications or actions based on user movement within defined areas, such as sending alerts when a user approaches a store or tracking when they leave a certain location.
To implement geofencing in your application, you first need to define the regions you want to monitor. This is done using the CLCircularRegion
class, which represents a circular geographic region. You must specify the center and radius of the region, as well as how to handle the monitoring behavior with respect to entering and exiting the region.
let center = CLLocationCoordinate2D(latitude: 37.33233141, longitude: -122.0312186) let region = CLCircularRegion(center: center, radius: 100, identifier: "MyRegion") region.notifyOnEntry = true region.notifyOnExit = true
In this example, we create a circular region with a center point defined by a latitude and longitude, a radius of 100 meters, and a unique identifier “MyRegion”. We also specify that we want to receive notifications when the user enters or exits this region.
Once you have defined your geofence, you must add it to the location manager using the startMonitoring(for:)
method. This starts the monitoring process, allowing your application to react when the user enters or exits the specified region:
locationManager.startMonitoring(for: region)
To handle region monitoring events, you need to implement the delegate methods provided by the CLLocationManagerDelegate
. Specifically, you will use locationManager(_:didEnterRegion:)
and locationManager(_:didExitRegion:)
to respond to entry and exit events:
func locationManager(_ manager: CLLocationManager, didEnterRegion region: CLRegion) { print("Entered region: (region.identifier)") // Perform an action, like sending a local notification } func locationManager(_ manager: CLLocationManager, didExitRegion region: CLRegion) { print("Exited region: (region.identifier)") // Perform an action, such as updating the UI }
In these methods, you can execute any logic necessary when the user enters or exits the defined geofence. For instance, triggering local notifications or updating the app’s UI can enhance user engagement based on their current location relative to the geofenced area.
It is important to note that geofencing is subject to certain limitations and behaviors. For instance, if the app is terminated or does not have permission to access location services in the background, region monitoring will continue, but entry and exit events may not be processed until the app is relaunched. To ensure that your app functions smoothly, always verify that necessary permissions are granted and handle any potential errors that may occur during monitoring.
In addition to monitoring single regions, you can also monitor multiple regions simultaneously by adding additional CLCircularRegion
instances to the location manager. However, keep in mind the system’s limitations on the total number of regions that can be monitored at the same time. As of current guidelines, this limit is 20 regions.
Geofencing can significantly enhance your application’s utility by providing users with timely, location-based information and notifications. By tapping into the Core Location framework’s geofencing capabilities, you can create a more engaging and responsive experience that resonates deeply with users and meets their contextual needs.
Best Practices for Location Services
When using location services within your application, adhering to best practices is essential for optimizing both user experience and resource management. Understanding how to leverage the capabilities of the Core Location framework effectively not only enhances your application’s functionality but also respects user privacy and device resources.
One of the foremost considerations is the frequency and accuracy of location updates. While high accuracy can be beneficial for applications requiring precise location data, such as navigation apps, it can also lead to increased battery drainage. It’s vital to balance the need for accuracy with the impact on battery life. For instance, use the desiredAccuracy property to set appropriate levels based on your application’s context:
locationManager.desiredAccuracy = kCLLocationAccuracyNearestTenMeters
This setting is suitable for applications that need a fair level of accuracy without the heavy resource load of the best accuracy settings. When high precision is not necessary, consider opting for a lower accuracy level to conserve power.
Additionally, it’s crucial to implement an effective distance filter. The distanceFilter property allows you to specify the minimum distance that the device must move before an update is received. Setting this can significantly reduce the number of location updates, thereby conserving battery and improving overall app performance:
locationManager.distanceFilter = 100 // Updates only when the device moves by 100 meters
Another best practice involves managing location updates intelligently. Use startUpdatingLocation() judiciously, and ensure you stop updates when they’re no longer needed. This can be particularly important in scenarios where the user enters a background state or when the app is temporarily not in use:
locationManager.stopUpdatingLocation()
Moreover, consider the user’s perspective and respect their privacy. Always clearly communicate why your app needs location access and provide a compelling reason. This transparency can be reflected in the strings provided for NSLocationWhenInUseUsageDescription and NSLocationAlwaysUsageDescription in your Info.plist. A well-phrased explanation can significantly impact user trust and their willingness to grant permissions.
Handling location updates effectively also requires robust error management. Implement the locationManager(_:didFailWithError:) method to gracefully handle any errors that may occur, such as the user denying location access or the device being unable to determine its location:
func locationManager(_ manager: CLLocationManager, didFailWithError error: Error) { print("Failed to find user's location: (error.localizedDescription)") }
Furthermore, ponder the implications of background location updates. If your app needs to track locations while in the background, ensure that you have configured the appropriate background modes in your app’s capabilities. However, clearly outline to users why that’s necessary, as it can significantly affect their device’s battery life.
Finally, test your application thoroughly in various scenarios to ensure that location services behave as expected under different conditions—both in terms of accuracy and responsiveness. This testing will help you identify potential issues and ensure a smooth user experience.