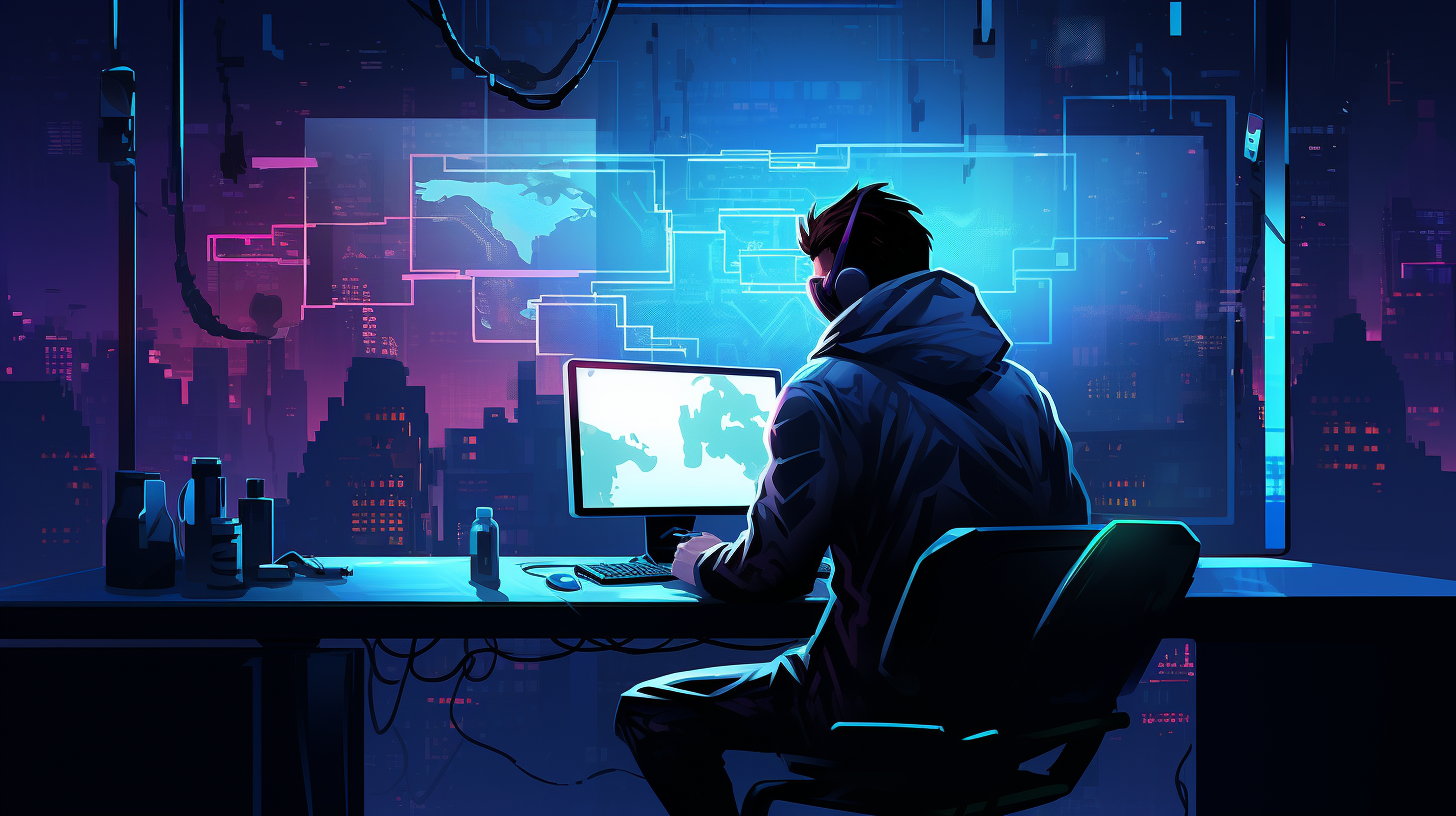
Swift and Machine Learning
Machine learning (ML) has emerged as a powerful paradigm capable of solving complex problems by enabling systems to learn from data. Understanding the foundational concepts of machine learning is essential for effectively using Swift in this domain. At its core, machine learning revolves around the idea of training algorithms to recognize patterns in data, making predictions, or taking actions based on those patterns.
In Swift, the approach to machine learning not only leverages the language’s robust capabilities but also its integration with powerful frameworks like Core ML. Before diving into the coding specifics, it is essential to grasp some key concepts:
Data Representation: Machine learning models require data in a structured format. In Swift, you typically handle datasets using arrays, dictionaries, or custom data structures. It is vital to prepare your data correctly, as the quality of your dataset directly affects the performance of your model.
Features and Labels: In supervised learning, data points consist of features (input variables) and labels (output variables). For example, in a model predicting house prices, the features could be the size of the house, location, and number of rooms, while the label is the price itself. Understanding how to engineer these features is critical in the development of effective ML models.
Algorithms: Various algorithms are employed for different tasks within machine learning, such as regression, classification, and clustering. Swift provides a clean syntax to implement these algorithms, whether through built-in libraries or custom implementations. Each algorithm has its strengths and weaknesses, and selecting the appropriate one depends on your specific problem.
Training and Testing: Machine learning models require training on a dataset and then testing to evaluate performance. In Swift, splitting your dataset into training and testing sets can be achieved using simple array manipulations. This process ensures that your model can generalize well to unseen data.
Evaluation Metrics: Once a model is trained, it’s vital to evaluate its performance using metrics like accuracy, precision, recall, and F1-score. These metrics help in understanding how well the model is performing and guide further tuning and adjustments. Swift provides excellent tools to perform these calculations efficiently.
Let’s look at a simple example where we create a basic linear regression model using Swift. This will illustrate some of the foundational concepts in action:
import Foundation struct LinearRegression { var slope: Double = 0.0 var intercept: Double = 0.0 mutating func train(data: [(x: Double, y: Double)]) { let n = Double(data.count) let sumX = data.reduce(0) { $0 + $1.x } let sumY = data.reduce(0) { $0 + $1.y } let sumXY = data.reduce(0) { $0 + $1.x * $1.y } let sumX2 = data.reduce(0) { $0 + $1.x * $1.x } slope = (n * sumXY - sumX * sumY) / (n * sumX2 - sumX * sumX) intercept = (sumY - slope * sumX) / n } func predict(x: Double) -> Double { return slope * x + intercept } } var model = LinearRegression() let trainingData: [(Double, Double)] = [(1, 2), (2, 3), (3, 5), (4, 6)] model.train(data: trainingData) let prediction = model.predict(x: 5) print("Predicted value for x=5: (prediction)")
In this code, we define a simple linear regression model and implement a training method that calculates the slope and intercept using ordinary least squares. After training the model with a small dataset, we can predict the output for a new input.
By grasping these fundamental concepts and employing Swift’s expressive syntax, developers can begin using the power of machine learning effectively. As we delve deeper into Core ML and other advanced techniques, these principles will serve as the bedrock for more sophisticated applications.
Core ML: Integrating Machine Learning into iOS Apps
Core ML stands as Apple’s premier framework designed to seamlessly integrate machine learning models into iOS applications. This framework allows developers to leverage pre-trained models across various domains, including image analysis, natural language processing, and sound classification, while maintaining optimal performance and operational efficiency on iOS devices.
One of the standout features of Core ML is its ability to work with multiple model types, such as TensorFlow, PyTorch, and Scikit-learn, which can be converted into Core ML format using tools like `coremltools`. This aspect simplifies the process of using state-of-the-art models without needing to reimplement them from scratch in Swift.
To integrate a machine learning model into an iOS app using Core ML, you typically follow a simpler workflow:
import CoreML // Load the model guard let model = try? MyModel(configuration: MLModelConfiguration()) else { fatalError("Could not load model") } // Prepare your input data let input = MyModelInput(feature1: 5.0, feature2: "example") // Make a prediction guard let output = try? model.prediction(input: input) else { fatalError("Prediction failed") } // Use the output print("Predicted value: (output.predictedLabel)")
In this example, we see how to load a pre-trained model, prepare input data, and make predictions using Core ML. The `MyModel` class is auto-generated from the `.mlmodel` file, which contains the machine learning model. The `MyModelInput` class represents the structure of the input accepted by the model, while the `output` contains the prediction results.
Core ML also facilitates the management of model versions and updates. Developers can easily switch models without significant adjustments to their codebase, allowing for continuous improvement and experimentation. Moreover, Core ML’s optimizations ensure that your application runs efficiently, using the device’s CPU and GPU resources effectively.
Another critical feature of Core ML is its support for on-device training with the new Model Update feature. Developers can implement a mechanism to adapt models based on user interactions and feedback, enhancing personalization while preserving user privacy. That’s particularly useful in applications where user data can improve service quality, such as recommendation systems.
For example, let’s ponder enhancing a recommendation system directly on the user’s device:
// Load an initial model let initialModel = try? MyRecommendationModel(configuration: MLModelConfiguration()) // Suppose we collect user interaction data let userInteractionData: [UserInteraction] = fetchUserInteractions() // Update the model with new data let updatedModel = try? initialModel.update(with: userInteractionData)
This piece of code demonstrates how we can collect user interaction data and update an existing Core ML model to reflect changes in user preferences. This functionality not only enhances user experience but also aligns with best practices in privacy, as data remains on the device.
Using Core ML effectively requires understanding the input and output formats of your models, as well as how to preprocess data to match those formats. Swift’s type safety and expressive syntax make data handling intuitive, allowing developers to focus on the core logic of their applications rather than boilerplate code.
Core ML provides a powerful, efficient, and user-friendly way to integrate machine learning capabilities into your iOS applications. By using its rich features, developers can create responsive and intelligent apps that learn and adapt to user needs while maintaining high performance across a range of devices.
Building and Training Models with Swift
In building and training models with Swift, developers can create custom algorithms tailored to specific tasks or utilize existing libraries to simplify the process. Unlike traditional programming paradigms, where logic is defined explicitly, machine learning focuses on feeding data into models, allowing them to discover patterns and relationships on their own. This approach requires careful data preparation, model selection, and training processes to ensure the model learns effectively.
To illustrate the process of building a model from scratch, let’s ponder implementing a simple decision tree classifier. Decision trees are interpretable and simpler models that make decisions based on feature values. Below is a simplified version of how you might implement this in Swift:
import Foundation class DecisionTree { var featureIndex: Int? var threshold: Double? var left: DecisionTree? var right: DecisionTree? var prediction: Double? func train(data: [[Double]], labels: [Double]) { // Base case: if all labels are the same, return a leaf node if Set(labels).count == 1 { prediction = labels[0] return } // Find the best split guard let (bestFeature, bestThreshold) = findBestSplit(data: data, labels: labels) else { prediction = labels.reduce(0, +) / Double(labels.count) return } featureIndex = bestFeature threshold = bestThreshold // Split the data let (leftData, leftLabels, rightData, rightLabels) = split(data: data, labels: labels, feature: bestFeature, threshold: bestThreshold) // Recursively train the left and right branches left = DecisionTree() left?.train(data: leftData, labels: leftLabels) right = DecisionTree() right?.train(data: rightData, labels: rightLabels) } func predict(sample: [Double]) -> Double { if let prediction = prediction { return prediction } let featureValue = sample[featureIndex!] if featureValue (Int, Double)? { // Implement method to find best feature and threshold for splitting data return nil // Placeholder for demonstration } private func split(data: [[Double]], labels: [Double], feature: Int, threshold: Double) -> ([[Double]], [Double], [[Double]], [Double]) { var leftData: [[Double]] = [] var leftLabels: [Double] = [] var rightData: [[Double]] = [] var rightLabels: [Double] = [] for (i, row) in data.enumerated() { if row[feature] <= threshold { leftData.append(row) leftLabels.append(labels[i]) } else { rightData.append(row) rightLabels.append(labels[i]) } } return (leftData, leftLabels, rightData, rightLabels) } } let trainingData: [[Double]] = [[2.0, 3.0], [1.0, 1.0], [4.0, 5.0], [6.0, 7.0]] let labels: [Double] = [0.0, 0.0, 1.0, 1.0] let tree = DecisionTree() tree.train(data: trainingData, labels: labels) let prediction = tree.predict(sample: [5.0, 6.0]) print("Predicted class: (prediction)")
This example demonstrates the key components of a decision tree classifier. We start by defining the `DecisionTree` class, which includes methods for training the model and making predictions. The `train` method recursively splits the dataset based on the best feature and threshold until it arrives at a leaf node, which holds the predicted label. The `predict` method traverses the tree based on the input features to make a classification.
When working with machine learning in Swift, using frameworks such as Create ML can significantly simplify the process of model training. Create ML provides a easy to use interface for training various types of models without delving deeply into the underlying algorithmic complexities. Using Swift’s seamless integration with Create ML allows developers to prototype and iterate quickly, leading to more efficient development cycles.
For instance, using Create ML to train an image classifier can be as simple as loading a dataset and calling a method to initiate training:
import CreateML // Assuming images are organized in folders named after their labels let trainingData = try MLDataTable(contentsOf: URL(fileURLWithPath: "path/to/images")) // Train the model let model = try MLImageClassifier(trainingData: trainingData) // Save the model try model.write(to: URL(fileURLWithPath: "path/to/save/MyImageClassifier.mlmodel"))
This snippet shows how simpler it’s to create an image classifier using Create ML. The framework handles the underlying complexities of feature extraction and model training, enabling you to focus on the higher-level aspects of your application. With Swift’s powerful capabilities and frameworks like Create ML, building and training custom models becomes a streamlined and efficient process.
Advanced Techniques: Custom ML Models and Frameworks
import Foundation class DecisionTree { var featureIndex: Int? var threshold: Double? var left: DecisionTree? var right: DecisionTree? var prediction: Double? func train(data: [[Double]], labels: [Double]) { // Base case: if all labels are the same, return a leaf node if Set(labels).count == 1 { prediction = labels[0] return } // Find the best split guard let (bestFeature, bestThreshold) = findBestSplit(data: data, labels: labels) else { prediction = labels.reduce(0, +) / Double(labels.count) return } featureIndex = bestFeature threshold = bestThreshold // Split the data let (leftData, leftLabels, rightData, rightLabels) = split(data: data, labels: labels, feature: bestFeature, threshold: bestThreshold) // Recursively train the left and right branches left = DecisionTree() left?.train(data: leftData, labels: leftLabels) right = DecisionTree() right?.train(data: rightData, labels: rightLabels) } func predict(sample: [Double]) -> Double { if let prediction = prediction { return prediction } let featureValue = sample[featureIndex!] if featureValue < threshold! { return left?.predict(sample: sample) ?? 0.0 } else { return right?.predict(sample: sample) ?? 0.0 } } private func findBestSplit(data: [[Double]], labels: [Double]) -> (Int, Double)? { // This method should implement the logic to find the best feature and threshold for splitting data return nil // Placeholder for demonstration } private func split(data: [[Double]], labels: [Double], feature: Int, threshold: Double) -> ([[Double]], [Double], [[Double]], [Double]) { var leftData: [[Double]] = [] var leftLabels: [Double] = [] var rightData: [[Double]] = [] var rightLabels: [Double] = [] for (i, row) in data.enumerated() { if row[feature] < threshold { leftData.append(row) leftLabels.append(labels[i]) } else { rightData.append(row) rightLabels.append(labels[i]) } } return (leftData, leftLabels, rightData, rightLabels) } } let trainingData: [[Double]] = [[2.5, 2.0], [1.0, 1.5], [3.5, 3.0]] let trainingLabels: [Double] = [0, 0, 1] var decisionTree = DecisionTree() decisionTree.train(data: trainingData, labels: trainingLabels) let sample = [2.0, 2.5] let prediction = decisionTree.predict(sample: sample) print("Predicted class for sample (sample): (prediction)")
In this implementation of a simple decision tree, we observe how the model is constructed and leveraged for predictions. The `train` method recursively builds the tree by finding the best feature and threshold to split the data based on the given labels. The `predict` method traverses the tree to yield a class prediction for new samples.
However, as simpler as this example is, building custom ML models in Swift is only the beginning. It opens doors to implementing advanced techniques such as ensemble learning, where multiple models are combined to enhance predictions, or using neural networks through libraries like Swift for TensorFlow, which allows for dynamic computation graphs and gradient-based learning directly in Swift.
With Swift’s capabilities and the powerful frameworks at hand, developers can build sophisticated models that leverage the latest research in machine learning. The flexibility to create custom algorithms tailored to specific needs is a significant advantage, particularly for unique applications that may not fit standard machine learning paradigms. This customization allows for experimentation and iteration, facilitating the development of cutting-edge solutions in various domains from healthcare to finance.
As we explore custom machine learning models and frameworks further, it becomes evident that Swift is not just about syntax or performance; it is about enabling developers to push the boundaries of what’s possible in machine learning, using a rich ecosystem of tools and libraries to create intelligent applications that learn and adapt in real-time.