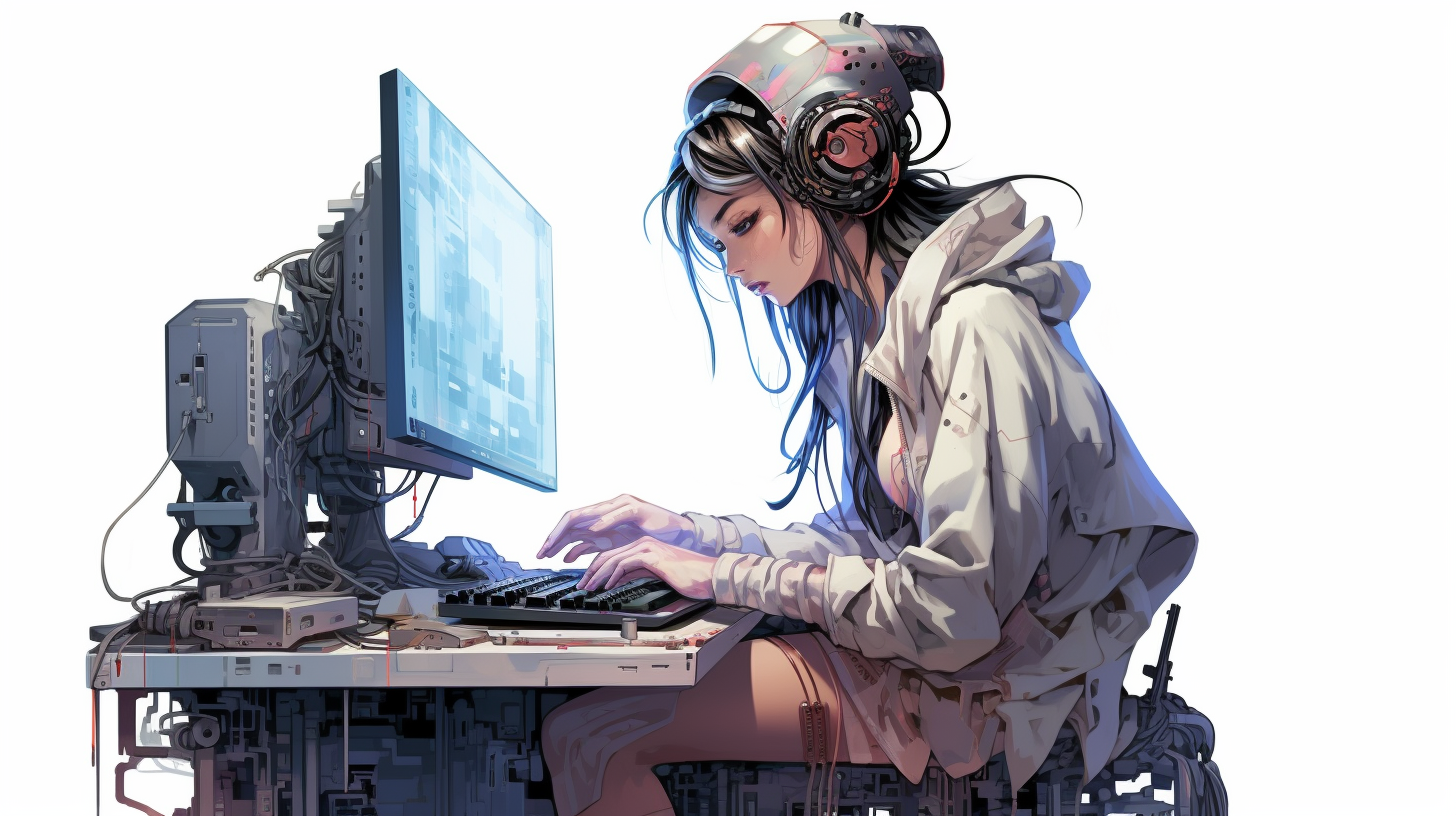
Swift and Metal
The Metal graphics framework is a powerful tool that allows developers to tap into the full potential of the GPU for rendering graphics and performing data-parallel computations. Metal is designed to provide low-level access to the GPU, which means it exposes the intricate details of the hardware that higher-level APIs usually abstract away. This access allows for more efficient rendering and computation, making Metal an ideal choice for performance-critical applications.
At its core, Metal is built around a streamlined, low-overhead architecture that facilitates high-performance graphics rendering and data processing. By minimizing CPU overhead and maximizing GPU throughput, Metal allows developers to achieve higher frame rates and richer visuals in their applications.
Metal is closely integrated with other Apple technologies, including UIKit and Core Animation, which enhances the graphics experience in iOS and macOS applications. This tight integration allows developers to efficiently manage resources across different layers of their applications, optimizing rendering performance.
One of the most significant advantages of Metal is its support for both graphics and compute operations. Developers can use Metal to render 2D and 3D graphics while also performing complex calculations at once. This dual capability is particularly beneficial in applications such as games and simulations, where rendering and physics calculations must occur simultaneously.
To get started with Metal, you’ll need to set up a Metal device, which represents the GPU. The following Swift code snippet illustrates how to create a Metal device:
import Metal // Create a Metal device guard let metalDevice = MTLCreateSystemDefaultDevice() else { fatalError("Metal is not supported on this device") }
Once you have a Metal device, you can create a Metal buffer to store data that the GPU will use during rendering or computation. Buffers are essential for transferring data between the CPU and GPU efficiently:
let bufferSize = MemoryLayout.size * 1024 let buffer = metalDevice.makeBuffer(length: bufferSize, options: [])!
Metal also utilizes command queues to manage the execution of tasks on the GPU. Command queues enable developers to submit multiple commands for execution, allowing for more efficient rendering and computation pipelines. Here’s how to create a command queue in Swift:
let commandQueue = metalDevice.makeCommandQueue()!
Understanding the Metal graphics framework especially important for any developer looking to harness the power of the GPU for high-performance applications. With its low-level access and dual capabilities for graphics and compute operations, Metal provides a robust foundation for building visually stunning and computationally intensive applications on Apple platforms.
Integrating Metal with Swift
Integrating Metal with Swift involves a seamless blend of the languages and frameworks that allow developers to leverage GPU capabilities effectively. Swift’s modern syntax and safety features make it an ideal companion for the Metal framework, streamlining the development process while maintaining performance. The integration points between Swift and Metal can be delineated into several key areas: setting up the rendering pipeline, managing resources, and submitting tasks to the GPU.
To begin with, after establishing a Metal device and a command queue, the next step is to set up a rendering pipeline. This involves creating a render pass descriptor that dictates how rendering operations will be conducted on the GPU. A render pass descriptor defines the framebuffer used for rendering and the associated colors, depth, and stencil formats. Here’s an example of how to create a render pass descriptor in Swift:
let passDescriptor = MTLRenderPassDescriptor() passDescriptor.colorAttachments[0].texture = yourTexture passDescriptor.colorAttachments[0].loadAction = .clear passDescriptor.colorAttachments[0].clearColor = MTLClearColor(red: 0.0, green: 0.0, blue: 0.0, alpha: 1.0) passDescriptor.colorAttachments[0].storeAction = .store
Once the render pass descriptor is in place, the next step is creating a Metal pipeline state object, which encapsulates the shaders used for rendering. The pipeline state is a critical component that informs the GPU how to process data using vertex and fragment shaders. Below is an example of how to create a pipeline state in Swift:
let vertexFunction = metalLibrary.makeFunction(name: "vertex_main")! let fragmentFunction = metalLibrary.makeFunction(name: "fragment_main")! let pipelineDescriptor = MTLRenderPipelineDescriptor() pipelineDescriptor.vertexFunction = vertexFunction pipelineDescriptor.fragmentFunction = fragmentFunction pipelineDescriptor.colorAttachments[0].pixelFormat = .bgra8Unorm let pipelineState = try metalDevice.makeRenderPipelineState(descriptor: pipelineDescriptor)
With the pipeline state established, you can begin to manage the resources that will be used by the GPU. This typically involves creating buffers for vertex data and any other resources needed for rendering. In Swift, creating a buffer is straightforward:
let vertices: [Float] = [ 0.0, 1.0, 0.0, -1.0, -1.0, 0.0, 1.0, -1.0, 0.0 ] let vertexBuffer = metalDevice.makeBuffer(bytes: vertices, length: MemoryLayout.size * vertices.count, options: [])
After setting up the rendering pipeline and managing resources, you’re ready to submit commands for execution. That is done using a command encoder, which encapsulates a series of commands that you want the GPU to execute. Here’s how to create and use a render command encoder:
let commandBuffer = commandQueue.makeCommandBuffer()! let renderEncoder = commandBuffer.makeRenderCommandEncoder(descriptor: passDescriptor)! renderEncoder.setRenderPipelineState(pipelineState) renderEncoder.setVertexBuffer(vertexBuffer, offset: 0, index: 0) renderEncoder.drawPrimitives(type: .triangle, vertexStart: 0, vertexCount: 3) renderEncoder.endEncoding() commandBuffer.present(yourDrawable) commandBuffer.commit()
This entire process—from device setup to command submission—is where the synergy of Swift and Metal shines. Swift’s strong type system and error handling allow for robust code this is less prone to runtime errors, while Metal’s low-level control over the GPU provides the performance needed for graphics-intensive applications. The integration of these two technologies enables developers to create applications that are not only visually stunning but also remarkably efficient.
Optimizing Performance in Swift with Metal
The optimization of performance in Swift with Metal heavily relies on understanding GPU architectures and using Metal’s features to their fullest extent. The key to achieving high-performance graphics rendering and compute operations lies in efficient resource management, minimizing CPU-GPU synchronization, and using Metal’s pipeline capabilities.
One of the first steps in optimizing performance is to minimize the overhead of state changes and resource binding. By grouping similar draw calls and batching them together, you can reduce the number of state changes the GPU needs to process. This approach can significantly enhance rendering efficiency. For example, if you are rendering a series of objects that share the same material properties, you should group their render commands together:
let commandBuffer = commandQueue.makeCommandBuffer()! let renderEncoder = commandBuffer.makeRenderCommandEncoder(descriptor: passDescriptor)! renderEncoder.setRenderPipelineState(pipelineState) // Batch draw calls for objects with the same state for object in objects { renderEncoder.setVertexBuffer(object.vertexBuffer, offset: 0, index: 0) renderEncoder.drawPrimitives(type: .triangle, vertexStart: 0, vertexCount: object.vertexCount) } renderEncoder.endEncoding() commandBuffer.present(yourDrawable) commandBuffer.commit()
Another crucial aspect of optimization is resource management. When using Metal, it’s beneficial to create buffers that are optimized for usage patterns. For instance, if certain buffers are static and do not change frequently, they should be created with the MTLResourceStorageMode.shared
option. This enables the GPU to cache these resources efficiently. Conversely, dynamic buffers that change frequently can utilize MTLResourceStorageMode.private
for quicker access:
let staticBuffer = metalDevice.makeBuffer(bytes: vertices, length: MemoryLayout.size * vertices.count, options: [.storageModeShared])! let dynamicBuffer = metalDevice.makeBuffer(length: bufferSize, options: [.storageModePrivate])!
Moreover, using Metal’s MTLCommandBuffer
effectively can lead to significant performance boosts. Command buffers are executed asynchronously, allowing the CPU to prepare and enqueue multiple command buffers while the GPU processes previous buffers. It’s vital to balance the workload between the CPU and GPU without overwhelming either side. You can utilize MTLCommandBufferCompletionHandler
to synchronize when the GPU has finished processing:
commandBuffer.addCompletedHandler { _ in // Perform any necessary updates or clean-up here }
Another optimization strategy involves reducing CPU-GPU synchronization points. Frequent synchronization can stall the GPU, leading to performance degradation. By structuring your application to perform as much work on the GPU as possible before coming back to the CPU, you can keep the GPU busy. For instance, use asynchronous compute to handle tasks like physics calculations or post-processing effects without waiting for the rendering pipeline to finish:
let computeCommandBuffer = commandQueue.makeCommandBuffer()! let computeEncoder = computeCommandBuffer.makeComputeCommandEncoder()! computeEncoder.setComputePipelineState(computePipelineState) // Set up buffers and execute compute functions here computeEncoder.dispatchThreadgroups(threadGroups, threadsPerThreadgroup: threadsPerGroup) computeEncoder.endEncoding() computeCommandBuffer.commit()
Lastly, profiling and debugging are essential to further refine performance. Using tools such as Xcode’s Metal Frame Debugger can help identify bottlenecks in rendering and compute tasks, enabling you to make data-driven decisions on optimizations. By continuously iterating on performance and using the full breadth of Metal’s capabilities, you can achieve exceptional results in your Swift applications.
Real-World Applications of Swift and Metal
When it comes to real-world applications of Swift and Metal, the combination opens up transformative possibilities across various fields, ranging from game development to scientific simulations and augmented reality. The ability to leverage the power of the GPU in conjunction with the expressive syntax and safety features of Swift creates a platform for developing highly interactive, visually engaging applications that can perform complex computations in real time.
In the gaming industry, for instance, Metal has become the go-to framework for developers aiming to push the boundaries of graphics fidelity and performance. Game developers can utilize Metal to implement advanced rendering techniques such as deferred shading, physically-based rendering, and real-time global illumination. These techniques enable the creation of stunning visual effects that can significantly enhance the player’s experience. Below is an example of how a simple game loop can be structured using Metal for rendering:
func gameLoop() { autoreleasepool { let commandBuffer = commandQueue.makeCommandBuffer()! let renderEncoder = commandBuffer.makeRenderCommandEncoder(descriptor: passDescriptor)! // Set up game objects and update their states updateGameObjects() // Draw each game object for gameObject in gameObjects { renderEncoder.setVertexBuffer(gameObject.vertexBuffer, offset: 0, index: 0) renderEncoder.drawPrimitives(type: .triangle, vertexStart: 0, vertexCount: gameObject.vertexCount) } renderEncoder.endEncoding() commandBuffer.present(yourDrawable) commandBuffer.commit() } }
Moreover, in the sphere of augmented reality (AR), the synergy between Swift and Metal has proven to be invaluable. With frameworks like ARKit, developers can create immersive environments that blend digital content with the real world. Metal handles the rendering of these 3D objects efficiently, allowing for high frame rates and responsive interactions. For example, when tracking the user’s movements and rendering virtual objects, the performance gains provided by Metal are crucial:
func renderARContent() { let commandBuffer = commandQueue.makeCommandBuffer()! let renderEncoder = commandBuffer.makeRenderCommandEncoder(descriptor: passDescriptor)! // Update the position of AR objects based on camera tracking updateARObjects() for arObject in arObjects { renderEncoder.setVertexBuffer(arObject.vertexBuffer, offset: 0, index: 0) renderEncoder.drawPrimitives(type: .triangle, vertexStart: 0, vertexCount: arObject.vertexCount) } renderEncoder.endEncoding() commandBuffer.present(yourDrawable) commandBuffer.commit() }
In scientific and data visualization applications, Metal enables the rendering of complex datasets and simulations. For example, developers can visualize large 3D datasets, perform real-time data processing, or conduct simulations that require substantial computational resources. The compute capabilities of Metal allow for efficient processing of data on the GPU, which is essential for tasks such as fluid dynamics simulations or molecular modeling:
func performComputation() { let computeCommandBuffer = commandQueue.makeCommandBuffer()! let computeEncoder = computeCommandBuffer.makeComputeCommandEncoder()! computeEncoder.setComputePipelineState(computePipelineState) computeEncoder.setBuffer(dataBuffer, offset: 0, index: 0) computeEncoder.dispatchThreadgroups(threadGroups, threadsPerThreadgroup: threadsPerGroup) computeEncoder.endEncoding() computeCommandBuffer.commit() }
The versatility of Swift and Metal in real-world applications cannot be overstated. From high-performance gaming to immersive AR experiences and complex scientific computations, developers are empowered to create applications that are not only visually stunning but also computationally efficient. By using the features of Metal alongside the modern constructs of Swift, developers can unlock new potentials that were previously constrained by the limitations of higher-level graphics APIs.