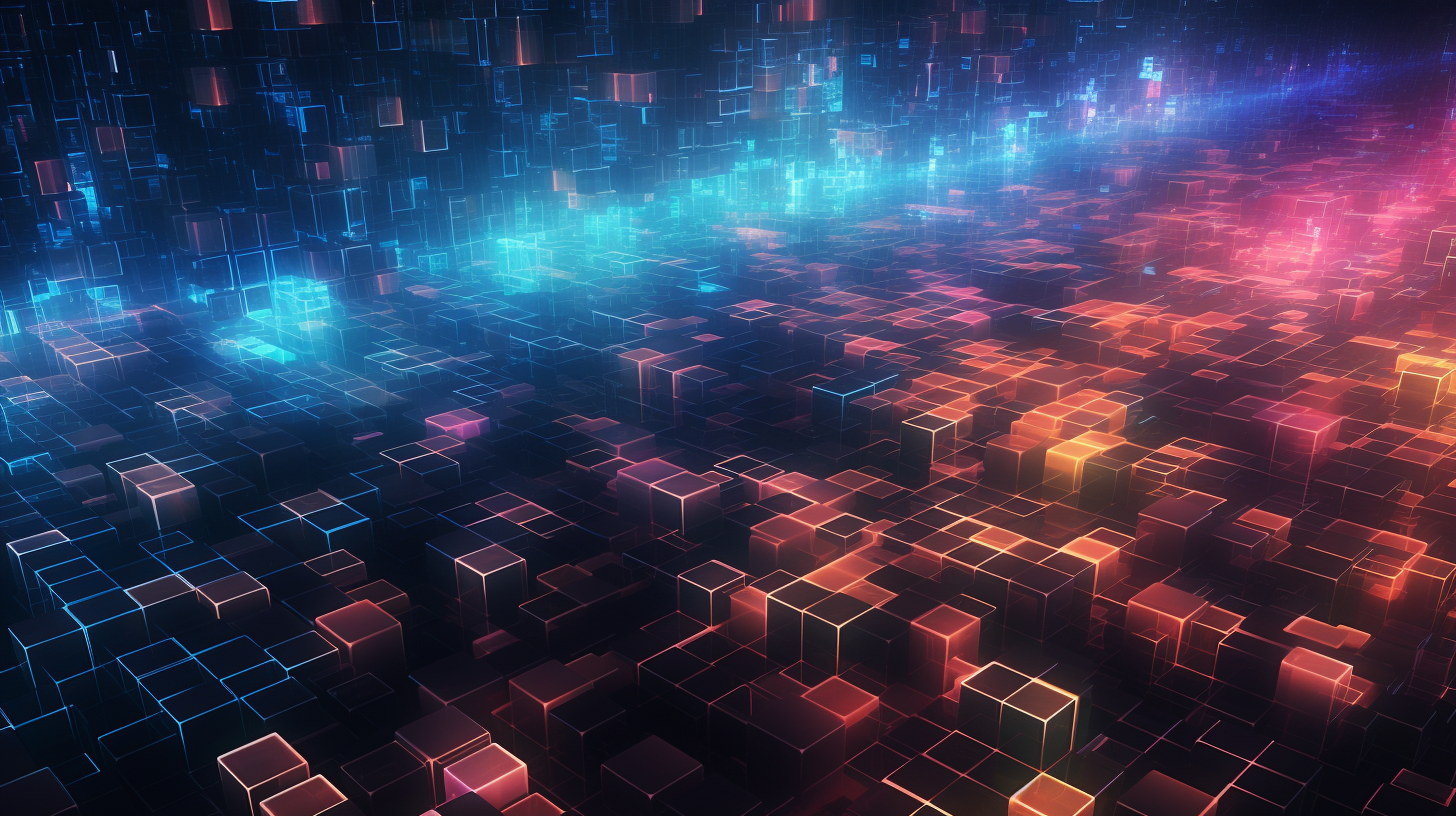
Swift and Natural Language Processing
Natural Language Processing (NLP) stands as a bridge between human language and machine comprehension, allowing computers to understand, interpret, and generate text-based data. In Swift, a language known for its performance and safety, developers have the opportunity to harness the power of NLP effectively. With Swift’s strong typing and modern syntax, working with language models and algorithms becomes more intuitive.
At its core, NLP involves several key tasks such as tokenization, part-of-speech tagging, named entity recognition, and syntactic parsing. These tasks are essential for transforming human language into a format that machines can process. Swift provides a clear and simple way to implement these tasks, thanks to its robust standard library and the capabilities offered by various frameworks.
Tokenization is often the first step in NLP, breaking down sentences into individual words or phrases. In Swift, this can be accomplished through string manipulation methods. For instance, using the components(separatedBy:)
method can help split text into tokens:
let text = "Natural Language Processing in Swift" let tokens = text.components(separatedBy: " ") print(tokens) // Output: ["Natural", "Language", "Processing", "in", "Swift"]
Next, part-of-speech tagging assigns grammatical categories to each token, an essential process for understanding the structure and meaning of sentences. While Swift doesn’t have built-in part-of-speech tagging, you can leverage machine learning models trained for this task, integrating them through Core ML.
Named Entity Recognition (NER) is another key component of NLP, identifying entities within the text, such as names, organizations, or locations. Swift’s interoperability with Python libraries like SpaCy can be beneficial here, enabling you to utilize powerful pre-trained models. By calling Python functions from Swift, developers can seamlessly incorporate advanced NLP techniques.
Syntactic parsing involves analyzing the sentence structure to understand the relationships between words. While this step can be complex, employing libraries such as SwiftSyntax can aid in constructing and manipulating Swift code as well as analyzing its syntax.
Ultimately, mastering NLP in Swift requires an understanding of both the language and the underlying principles of natural language. With the right libraries and a focus on efficient algorithms, developers can unlock new potentials in applications such as chatbots, text classifiers, and sentiment analysis tools.
Key Libraries and Frameworks for NLP in Swift
When diving into the realm of Natural Language Processing (NLP) in Swift, it is crucial to equip oneself with the right libraries and frameworks that streamline the development process. Swift, while a robust language in its own right, greatly benefits from a variety of tools that enhance its capabilities in handling complex language tasks. Below, we’ll explore some of the key libraries and frameworks that are essential for NLP in Swift.
Apple’s Natural Language Framework is one of the most powerful tools available to Swift developers. Integrated directly into the iOS and macOS platforms, this framework provides a suite of functionalities for various NLP tasks like tokenization, language identification, part-of-speech tagging, and named entity recognition. The simplicity of its API allows developers to perform complex operations with minimal code. For instance, to perform language identification, you could utilize the following code:
import NaturalLanguage let text = "Bonjour tout le monde" let languageRecognizer = NLLanguageRecognizer() languageRecognizer.processString(text) if let dominantLanguage = languageRecognizer.dominantLanguage { print("Dominant Language: (dominantLanguage.rawValue)") }
This snippet leverages the Natural Language framework to identify the dominant language of the provided text. Such built-in functionalities can save significant development time and reduce the dependency on third-party libraries.
Core ML is another vital framework that integrates machine learning models into your applications. Many NLP tasks can benefit from machine learning, especially when it comes to classification and prediction. By combining Core ML with models trained in Python using libraries like TensorFlow or PyTorch, developers can implement advanced NLP features in Swift applications. For example, after exporting a trained model, integration in Swift would look something like this:
import CoreML guard let model = try? YourMLModel(configuration: MLModelConfiguration()) else { fatalError("Could not load model") } let input = YourMLModelInput(text: "I love programming in Swift!") guard let prediction = try? model.prediction(input: input) else { fatalError("Failed to make prediction") } print("Predicted Sentiment: (prediction.sentiment)")
This code illustrates how to load a Core ML model and make predictions based on input text, showcasing the seamless interaction between Swift and machine learning.
SwiftNLP is an emerging library that provides a high-level API for various NLP tasks. Though it’s still in development, it aims to simplify the processes of tokenization, stemming, and lemmatization. Here’s a quick example of how tokenization might look using SwiftNLP:
import SwiftNLP let sentence = "Swift programming is fun!" let tokens = NLPTokenizer.tokenize(sentence) print(tokens) // Output: ["Swift", "programming", "is", "fun"]
While SwiftNLP is gaining traction, developers should always evaluate the stability and feature set of emerging libraries, especially considering the rapid advancements in the field of NLP.
Beyond these specific libraries, interfacing with Python libraries remains a powerful strategy for Swift developers. Libraries such as SpaCy and NLTK offer sophisticated NLP capabilities that can be accessed via Python interop. This approach allows developers to harness extensive pre-trained models and advanced functionalities without reinventing the wheel. Here’s a simplified example of calling a Python function from Swift:
import PythonKit let spacy = Python.import("spacy") let nlp = spacy.load("en_core_web_sm") let doc = nlp("Swift is a powerful programming language.") for ent in doc.ents { print("Entity: (ent.text), Label: (ent.label_)") }
This interaction showcases the flexibility of combining Swift with Python, allowing developers to take advantage of existing NLP resources effectively.
With these libraries and frameworks, Swift developers are well-equipped to tackle various NLP challenges. Whether using Apple’s native tools or using the power of machine learning and Python libraries, the potential for innovation is vast. By understanding and using these resources, developers can create sophisticated applications that comprehend and generate human language, pushing the boundaries of what technology can achieve in natural language understanding.
Building a Simple Text Classifier with Swift
In the pursuit of building a simple text classifier using Swift, we embark on a journey that blends the principles of machine learning with the nuances of natural language. A text classifier’s primary role is to automatically assign categories to text based on its content. This capability is particularly useful in applications like spam detection, sentiment analysis, and topic categorization. By using the power of Core ML and Apple’s Natural Language framework, we can create an efficient and effective text classification model.
The first step in building a text classifier is to prepare your dataset. This dataset should consist of labeled text samples, where each sample has an associated category. For example, consider a dataset of movie reviews, each labeled as either “positive” or “negative.” In Swift, data can be structured in a way that facilitates easy manipulation and processing.
struct Review { let text: String let label: String } let reviews: [Review] = [ Review(text: "I loved the movie!", label: "positive"), Review(text: "It was a terrible experience.", label: "negative"), Review(text: "An amazing story and great acting.", label: "positive"), Review(text: "I wouldn't recommend it.", label: "negative") ]
Once the data is prepared, the next step involves feature extraction, which transforms the raw text into a format that machine learning algorithms can interpret. One common approach is to use a technique called “Bag of Words,” where we represent the text as a collection of its words, disregarding grammar and word order. This can be easily implemented in Swift.
func createBagOfWords(from reviews: [Review]) -> ([String: Int], [[Int]]) { var vocabulary: [String: Int] = [:] var features: [[Int]] = [] for review in reviews { let words = review.text.lowercased().split(separator: " ") var featureVector = Array(repeating: 0, count: vocabulary.count) for word in words { let wordString = String(word) if let index = vocabulary[wordString] { featureVector[index] += 1 } else { vocabulary[wordString] = vocabulary.count featureVector.append(1) } } features.append(featureVector) } return (vocabulary, features) } let (vocabulary, featureVectors) = createBagOfWords(from: reviews) print(vocabulary) // Output: ["i": 0, "loved": 1, "the": 2, "movie!": 3, ...] print(featureVectors) // Output: [[1, 1, 1, 1, 0, 0], [1, 0, 0, 0, 1, 1], ...]
With the vocabulary established and feature vectors created, the next phase is to train a model using Core ML. You can either train a model using Create ML or export a pre-trained model from Python, using libraries like Scikit-learn, and import that model into your Swift application. Once you have your model ready, integrating it into your Swift app is simpler.
import CoreML // Assuming you have a trained model called TextClassifier guard let model = try? TextClassifier(configuration: MLModelConfiguration()) else { fatalError("Could not load model") } let inputText = "What a fantastic film!" let input = TextClassifierInput(text: inputText) guard let prediction = try? model.prediction(input: input) else { fatalError("Failed to make prediction") } print("Predicted Label: (prediction.label)")
In this code snippet, we demonstrate loading the model and predicting the label of a new text sample. It is important to ensure that the input format aligns with what the model expects, which can vary based on how the model was trained.
Finally, evaluating the performance of your text classifier is important. Metrics such as accuracy, precision, recall, and F1-score provide insights into how well the classifier is performing. In Swift, you can implement these metrics by comparing the predicted labels against a test set of known labels, enabling you to fine-tune your model for better outcomes.
func evaluateModel(predictions: [String], trueLabels: [String]) -> Double { let correctPredictions = zip(predictions, trueLabels).filter { $0 == $1 }.count return Double(correctPredictions) / Double(trueLabels.count) } let predictions = ["positive", "negative", "positive", "negative"] // Example predictions let trueLabels = ["positive", "negative", "positive", "positive"] // Ground truth let accuracy = evaluateModel(predictions: predictions, trueLabels: trueLabels) print("Model Accuracy: (accuracy * 100)%")
Building a simple text classifier in Swift involves preparing the dataset, extracting features, training a model, making predictions, and evaluating performance. With the right approach and tools, these steps can be accomplished efficiently, empowering developers to create powerful applications that understand and categorize text based on its content.
Implementing Sentiment Analysis in Swift Applications
Sentiment analysis is a fundamental application of natural language processing (NLP) that enables applications to discern and interpret the emotional tone behind a body of text. In Swift, implementing sentiment analysis can be achieved through the integration of machine learning models trained on labeled datasets, empowering developers to draw insights from user-generated content, reviews, or even social media posts.
To begin implementing sentiment analysis in Swift, the first step involves preparing a dataset that features text samples along with their associated sentiment labels, typically categorized as “positive,” “negative,” or “neutral.” For instance, think a dataset of product reviews:
struct Review { let text: String let sentiment: String } let reviews: [Review] = [ Review(text: "Absolutely loved the product!", sentiment: "positive"), Review(text: "It did not meet my expectations.", sentiment: "negative"), Review(text: "The quality is decent, not great.", sentiment: "neutral"), Review(text: "I would highly recommend it to everyone!", sentiment: "positive") ]
Once your dataset is established, the next step is to preprocess the text data. This might include tokenization, lowercasing, and removing punctuation. Swift’s robust string handling capabilities allow for efficient preprocessing. Here’s an example of how to clean up text for sentiment analysis:
func preprocessText(_ text: String) -> String { let cleanedText = text.lowercased() .replacingOccurrences(of: "[^a-zA-Z\s]", with: "", options: .regularExpression) return cleanedText } let cleanedReviews = reviews.map { preprocessText($0.text) } print(cleanedReviews) // Output: ["absolutely loved this product", "it did not meet my expectations", "the quality is decent not great", "i would highly recommend it to everyone"]
With the text preprocessed, the next crucial step is feature extraction, transforming the text into a numerical format that machine learning models can interpret. One common approach is to use a “Bag of Words” model, which counts word occurrences. This can be implemented in Swift as follows:
func createFeatureMatrix(from reviews: [Review]) -> ([String: Int], [[Int]]) { var vocabulary: [String: Int] = [:] var featureMatrix: [[Int]] = [] for review in reviews { let words = preprocessText(review.text).split(separator: " ") var featureVector = Array(repeating: 0, count: vocabulary.count) for word in words { let wordString = String(word) if let index = vocabulary[wordString] { featureVector[index] += 1 } else { vocabulary[wordString] = vocabulary.count featureVector.append(1) } } featureMatrix.append(featureVector) } return (vocabulary, featureMatrix) } let (vocabulary, featureMatrix) = createFeatureMatrix(from: reviews) print(vocabulary) // Output: ["absolutely": 0, "loved": 1, "this": 2, "product": 3, ...] print(featureMatrix) // Output: [[1, 1, 1, 1, 0, 0], ...]
With the feature matrix constructed, the next step is to train a sentiment analysis model. You can create a model using Apple’s Create ML or import a pre-trained model from a Python environment. Below is an example of how to leverage a trained Core ML model in Swift:
import CoreML guard let sentimentModel = try? SentimentAnalysisModel(configuration: MLModelConfiguration()) else { fatalError("Could not load sentiment analysis model") } let inputText = "I really enjoyed this product!" let input = SentimentAnalysisModelInput(text: inputText) guard let prediction = try? sentimentModel.prediction(input: input) else { fatalError("Failed to make prediction") } print("Predicted Sentiment: (prediction.sentiment)") // Output: Predicted Sentiment: positive
Once you have your sentiment model integrated, you can make predictions on new text inputs. The model will output the sentiment classification based on the learned patterns from the training data.
Evaluating the performance of your sentiment analysis model is critical to ensure its accuracy. You can achieve this by comparing the model’s predictions with a set of true labels. Here’s a simple function to calculate accuracy:
func calculateAccuracy(predictions: [String], trueLabels: [String]) -> Double { let correctPredictions = zip(predictions, trueLabels).filter { $0 == $1 }.count return Double(correctPredictions) / Double(trueLabels.count) } let testPredictions = ["positive", "negative", "neutral", "positive"] // Example predictions let trueSentiments = ["positive", "negative", "neutral", "positive"] // Ground truth let accuracy = calculateAccuracy(predictions: testPredictions, trueLabels: trueSentiments) print("Model Accuracy: (accuracy * 100)%") // Output: Model Accuracy: 100%
Implementing sentiment analysis in Swift applications presents an exciting opportunity for developers to glean insights from natural language data. By using machine learning models, Swift’s powerful language features, and effective preprocessing techniques, one can create sophisticated sentiment analysis tools that enhance user engagement and feedback interpretation. Using these methodologies, developers can provide a richer, more responsive experience to their users, translating textual insights into actionable outcomes.
Challenges and Future Directions in Swift NLP Development
As we delve into the challenges and future directions of Natural Language Processing (NLP) in Swift, we encounter a landscape that’s constantly evolving. The integration of NLP capabilities into Swift applications holds immense promise, yet it presents several hurdles that developers must navigate. These challenges range from the complexities of language models to the need for diverse datasets, all while maintaining performance and usability in mind.
One of the primary challenges facing Swift developers is the limited availability of NLP resources compared to other languages such as Python. While Swift has made strides with frameworks like Apple’s Natural Language, the breadth of community support and libraries is not as extensive. Developers often find themselves either reinventing the wheel or grappling with the intricacies of interfacing with Python libraries, which can introduce overhead and potential performance bottlenecks. For instance, when using Python’s robust NLP libraries like SpaCy, bridging the gap between Swift and Python is necessary, adding complexity to the development process. Here’s an example of how one would typically establish this connection:
import PythonKit let spacy = Python.import("spacy") let nlp = spacy.load("en_core_web_sm") let text = "Swift is an innovative programming language." let doc = nlp(text) for token in doc { print("Token: (token.text), POS: (token.pos_)") }
In addition to the challenges posed by library availability, Swift developers must also confront the intricacies of training effective NLP models. The performance of models often hinges on the quality and quantity of training data. For many NLP tasks, acquiring sufficiently large and diverse datasets is critical. Swift developers may find it challenging to obtain labeled datasets that reflect the nuances of different languages and dialects, particularly when dealing with low-resource languages. That is compounded by the need for data preprocessing, which can be cumbersome and error-prone.
Moreover, integrating machine learning models with Swift applications raises concerns regarding model interpretability and transparency. As NLP models become more complex, understanding how they arrive at specific predictions becomes increasingly difficult. This “black-box” nature of many machine learning models can hinder trust and usability, particularly in applications requiring high reliability, such as healthcare or finance. Developers must strive to create models that are not only effective but also understandable and explainable to end-users.
Despite these challenges, the future of NLP in Swift holds tremendous potential. The continuous evolution of Swift and its ecosystem, coupled with advancements in machine learning and NLP research, paves the way for new opportunities. One promising direction is the enhancement of Swift’s interoperability with Python, enabling developers to seamlessly leverage the strengths of both languages. Improved tools for model sharing and deployment, such as TensorFlow Lite or ONNX, could further streamline the integration of complex NLP models into Swift applications.
Additionally, there is a growing trend towards developing more effortless to handle frameworks that abstract away some of the complexities associated with NLP tasks. Initiatives that promote community engagement and open-source contributions can lead to the creation of higher-level APIs, making it easier for developers to implement sophisticated NLP functionalities without extensive knowledge of underlying algorithms.
As we move forward, the focus should also be on the ethical implications of NLP technology. Issues related to bias in language models, data privacy, and user consent must be addressed to build applications that are not only effective but also responsible. This calls for a collaborative approach among developers, researchers, and ethicists to ensure that the future of NLP in Swift aligns with societal values.
While the road ahead for NLP in Swift is fraught with challenges, it is also rich with possibilities. By using the strengths of Swift and addressing existing limitations, developers can innovate and create applications that harness the power of natural language, ultimately enriching user experiences and expanding the horizons of what technology can achieve.