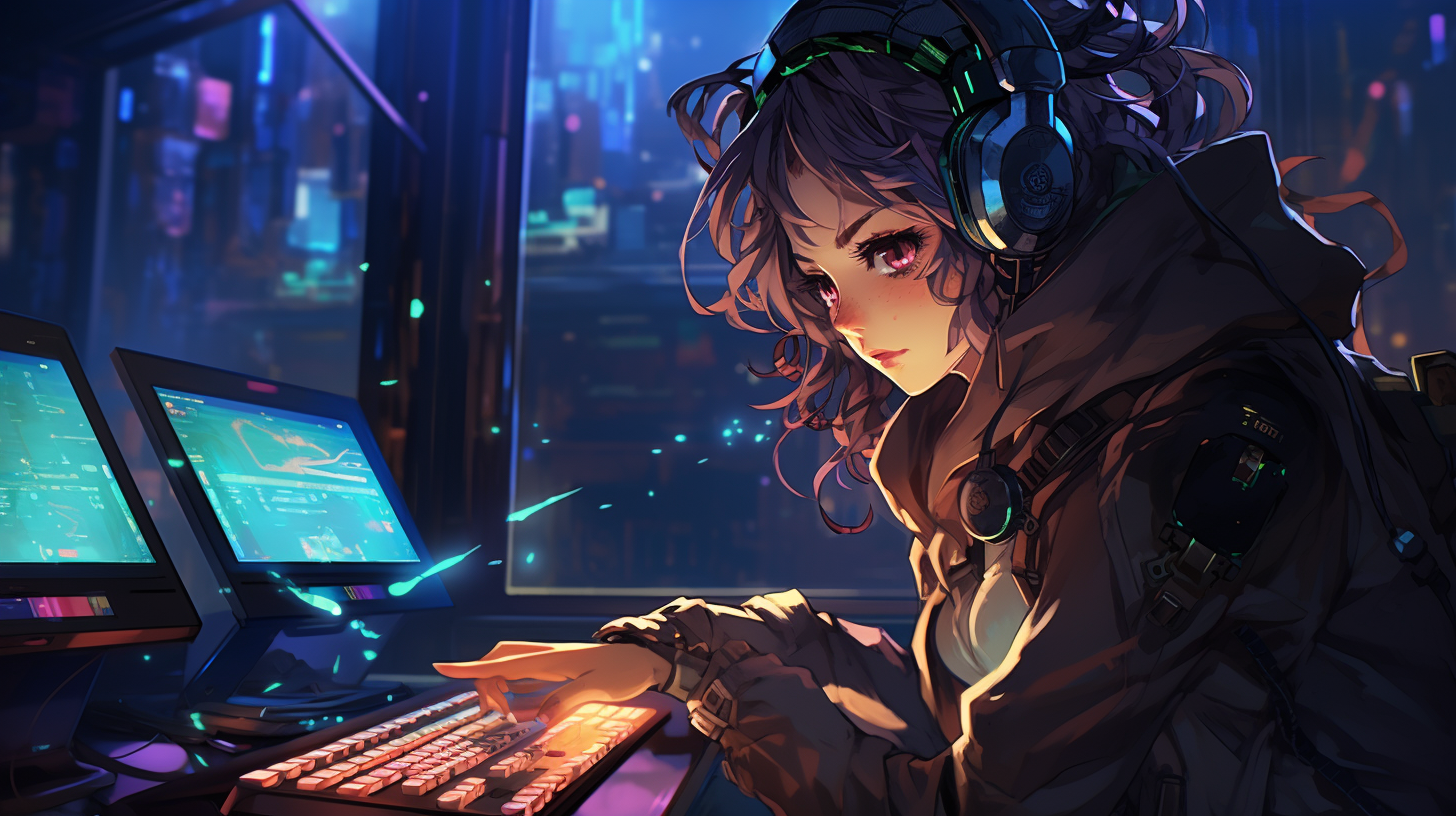
Swift and PhotoKit
The PhotoKit framework is a powerful tool for developers to manage and interact with the photo and video assets stored on a user’s device. Built on the principles of performance and efficiency, PhotoKit provides a high-level API that abstracts away many complexities involved in handling multimedia content.
At its core, PhotoKit allows for seamless integration with the user’s photo library. The framework provides a unified interface for accessing, importing, and exporting images and videos, enabling developers to build rich media applications that can leverage the vast amount of content stored on iOS devices.
One of the primary components of PhotoKit is the PHAsset
class, which represents an image or video in the library. Each asset contains metadata such as the creation date, location, and media type, allowing developers to retrieve detailed information about the content. The framework also provides various collections, such as albums and moments, to organize these assets hierarchically.
To interact with the user’s photo library, developers need to utilize the PHPhotoLibrary
class, which serves as the entry point for all operations involving photos and videos. This class provides methods for fetching assets, observing changes in the library, and performing edits.
Here’s a simple example of how to fetch all photos from the user’s library:
import Photos func fetchPhotos() { let fetchOptions = PHFetchOptions() fetchOptions.sortDescriptors = [NSSortDescriptor(key: "creationDate", ascending: false)] let allPhotos = PHAsset.fetchAssets(with: .image, options: fetchOptions) allPhotos.enumerateObjects { (asset, _, _) in print("Found photo: (asset)") } }
Understanding the PhotoKit framework also involves grasping the importance of asynchronous operations. Fetching assets can be a time-consuming task, especially with large libraries, so it’s important to handle these tasks on background threads to maintain a responsive user interface.
In addition to basic asset management, PhotoKit supports advanced features such as image and video editing, creating and managing albums, and integrating with iCloud for synchronized access across devices. This versatility makes PhotoKit an essential framework for any app that interacts with media content.
As you dive deeper into photo management, you’ll discover how to optimize the user experience by using localized resources, caching mechanisms, and the capabilities of the device. The combination of robust APIs and system-level access positions PhotoKit as a cornerstone for multimedia applications on iOS.
Setting Up Your Swift Project for PhotoKit
To set up your Swift project for using the PhotoKit framework, the first step is to ensure that your project is properly configured to access the user’s photo library. This involves several key actions, including adding the necessary permissions to your app’s Info.plist file, importing the framework, and establishing the required classes and methods to interact with PhotoKit.
Begin by including the PhotoKit framework in your project. You can do this by importing it at the top of your Swift file:
import Photos
Next, you’ll need to request permission from the user to access their photo library. This is a critical step, as users must grant permission for your app to interact with their media. You should include the appropriate keys in the Info.plist file to inform users why you require access. The keys you typically need are:
- A message explaining why you need access to the photo library.
- A message explaining why you need permission to add to the photo library.
Here is an example of how to request access to the photo library:
PHPhotoLibrary.requestAuthorization { status in switch status { case .authorized: print("Access granted to photo library.") case .denied, .restricted: print("Access denied to photo library.") case .notDetermined: print("Access not determined yet.") @unknown default: fatalError("Unknown authorization status.") } }
Once permission is granted, you can begin fetching assets from the library. Initialization of PHPhotoLibrary is simpler, but ensure you handle this on an appropriate thread to maintain efficiency. For example, if you are fetching assets right after obtaining permission, consider wrapping your fetch call in a background task:
DispatchQueue.global(qos: .userInitiated).async { let fetchOptions = PHFetchOptions() fetchOptions.sortDescriptors = [NSSortDescriptor(key: "creationDate", ascending: false)] let allPhotos = PHAsset.fetchAssets(with: .image, options: fetchOptions) allPhotos.enumerateObjects { (asset, _, _) in // Process each asset here print("Found photo: (asset)") } }
Setting up your project also requires you to handle changes in the photo library. This is important for dynamically updating your UI when new images are added or when existing ones are modified. You can achieve this by observing the photo library for changes:
let changeObserver = PHPhotoLibrary.shared().register(self)
You must conform to the PHPhotoLibraryChangeObserver protocol and implement the photoLibraryDidChange(_:) method to respond to changes:
extension YourClass: PHPhotoLibraryChangeObserver { func photoLibraryDidChange(_ changeInstance: PHChange) { // Handle changes in the photo library } }
With these configurations and setups, your Swift project will be primed for using the capabilities of PhotoKit. As you progress, you can build on this foundation to incorporate more advanced functionalities such as asset editing, creating albums, or handling Live Photos, all while ensuring a smooth and responsive user experience.
Accessing and Managing Photos in the Library
Accessing and managing photos in the library requires a solid understanding of the PhotoKit components and how to effectively interact with them. The PHAsset class is central to this process, representing individual media items within the user’s photo library. By fetching these assets, developers can retrieve not only the images and videos themselves but also their associated metadata, which is invaluable for building rich media applications.
To begin fetching assets, you will typically want to use a PHFetchOptions
object to customize your requests according to your app’s needs. These options allow you to specify sorting criteria, filtering by media type, and more. The following is an example of how to fetch all photos from the library, sorted by creation date:
import Photos func fetchPhotos() { let fetchOptions = PHFetchOptions() fetchOptions.sortDescriptors = [NSSortDescriptor(key: "creationDate", ascending: false)] let allPhotos = PHAsset.fetchAssets(with: .image, options: fetchOptions) allPhotos.enumerateObjects { (asset, _, _) in print("Found photo: (asset)") } }
Once you’ve fetched the assets, think how you’ll manage them. You might want to display these assets in a collection view, or allow users to select multiple items for sharing or editing. Remember that the user experience is paramount; loading and manipulating assets should be done asynchronously to keep your UI responsive.
To handle user interactions effectively, you can implement a delegate or closure that triggers when a user selects an asset. This way, you can retrieve and display additional information related to the asset or trigger editing functionalities. Here’s a basic example of how you can handle asset selection:
func assetSelected(asset: PHAsset) { // Load the image for display let imageManager = PHImageManager.default() let requestOptions = PHImageRequestOptions() requestOptions.isSynchronous = false requestOptions.deliveryMode = .highQualityFormat imageManager.requestImage(for: asset, targetSize: CGSize(width: 300, height: 300), contentMode: .aspectFill, options: requestOptions) { (image, _) in if let image = image { // Display the image in your UI print("Loaded image: (image)") } } }
In addition to fetching and displaying assets, you might want to manage albums and collections. Creating a new album programmatically is simpler using the PHAssetCollectionChangeRequest
class. Here’s how you can create an album and add an asset to it:
func createAlbum(albumName: String, asset: PHAsset) { var placeholder: PHObjectPlaceholder? PHPhotoLibrary.shared().performChanges({ let createAlbumRequest = PHAssetCollectionChangeRequest.creationRequestForAssetCollection(withTitle: albumName) placeholder = createAlbumRequest.placeholderForCreatedAssetCollection let assetRequest = PHAssetChangeRequest(for: asset) createAlbumRequest.addAssets([assetRequest.placeholderForCreatedAsset!]) }) { success, error in if success { print("Album created successfully.") } else if let error = error { print("Error creating album: (error.localizedDescription)") } } }
Remember that any modifications to the photo library must occur within a change block, as shown above. This ensures that your changes are batched and handled efficiently by the library.
As you work with the user’s photo library, keep in mind that user privacy is paramount. Always check and handle permissions appropriately before accessing or managing any assets. The user’s trust hinges on how you handle their data, so maintain transparency about what you’re accessing and why.
Accessing and managing photos in the library with PhotoKit provides a robust set of tools for developers. By using the capabilities offered by the PHAsset, PHPhotoLibrary, and associated classes, you can create a effortless to handle experience that allows users to interact with their media content seamlessly.
Implementing Photo Editing Features
Implementing photo editing features using the PhotoKit framework is a game changer for developers looking to improve user engagement and creativity within their applications. With PhotoKit, you can provide powerful editing capabilities directly within your app, allowing users to manipulate images in ways that suit their preferences. The editing features revolve around the use of the PHAsset class, along with PHContentEditingInput and PHContentEditingOutput, which facilitate the retrieval and storage of edited content.
To start editing photos, you first need to request the necessary editing input from a given PHAsset. This involves creating an instance of PHImageManager and using it to fetch the PHContentEditingInput. The editing input contains the original image along with metadata that describes its properties. Here’s a basic example of how to fetch the editing input for a selected asset:
func fetchEditingInput(for asset: PHAsset, completion: @escaping (PHContentEditingInput?) -> Void) { let imageManager = PHImageManager.default() let requestOptions = PHContentEditingInputRequestOptions() requestOptions.canHandleAdjustmentData = { (adjustmentData) -> Bool in return true // Handle the adjustment data if needed } imageManager.requestContentEditingInput(for: asset, options: requestOptions) { (editingInput, _) in completion(editingInput) } }
Once you have the editing input, you can present the image in your UI and allow users to apply various editing functionalities, such as cropping, filtering, or adjusting exposure. For example, you may integrate third-party libraries or use native controls to implement these features. After the user completes their edits, the next step is to save the changes back to the photo library.
To save the edited content, you need to create a PHContentEditingOutput object to hold the adjustments made to the original image. This output contains the edited image data, along with adjustment data that describes the edits. Here’s how you can save the edits:
func saveEdits(for asset: PHAsset, editingInput: PHContentEditingInput, adjustments: PHAdjustmentData) { PHPhotoLibrary.shared().performChanges({ let request = PHAssetChangeRequest(for: asset) let output = PHContentEditingOutput(contentEditingInput: editingInput) // Here, apply your edited image to the output output.fullSizeImage = UIImage(named: "editedImage")?.jpegData(compressionQuality: 1.0) output.adjustmentData = adjustments request.contentEditingOutput = output }) { success, error in if success { print("Edits saved successfully.") } else if let error = error { print("Error saving edits: (error.localizedDescription)") } } }
As you implement these editing features, keep in mind the importance of user experience. Make sure the editing process feels intuitive and responsive, providing clear feedback to users on their actions. Think providing undo/redo options, as well as previews of the original and edited images to help users make informed decisions about their edits.
Implementing photo editing features with PhotoKit not only enhances the capabilities of your app but also enriches the user experience by giving users creative control over their media. With just a few lines of code, you can enable powerful editing functionalities that empower users to personalize their photos in a seamless manner.
Using Live Photos and Memories
Using Live Photos and Memories in your Swift applications through the PhotoKit framework opens up exciting possibilities for enhancing user engagement with their media. Live Photos, introduced with iPhone 6s, capture a few seconds of video along with a still image, while Memories automatically curates collections of photos based on significant moments in a user’s life. Integrating these features into your app not only enriches the user experience but also allows for innovative ways to interact with media content.
To work with Live Photos, you need to understand how PhotoKit distinguishes them from regular photos. Live Photos are represented by PHAsset objects as well, but they come with additional properties, namely a video file alongside the still image. To determine if an asset is a Live Photo, you can check its media subtypes:
let fetchOptions = PHFetchOptions() fetchOptions.predicate = NSPredicate(format: "mediaType = %d AND mediaSubtypes = %d", PHAssetMediaType.image.rawValue, PHAssetMediaSubtype.photoLive.rawValue) let livePhotos = PHAsset.fetchAssets(with: fetchOptions) livePhotos.enumerateObjects { (asset, _, _) in if asset.mediaSubtypes.contains(.photoLive) { print("Found Live Photo: (asset)") } }
To play a Live Photo, you can use the PHLivePhotoView class, which provides a simple interface for displaying and interacting with Live Photos. Here’s how to configure a PHLivePhotoView to present a Live Photo:
import PhotosUI func displayLivePhoto(asset: PHAsset) { let livePhotoView = PHLivePhotoView(frame: CGRect(x: 0, y: 0, width: 300, height: 300)) PHLivePhoto.request(with: asset, targetSize: CGSize(width: 300, height: 300), contentMode: .aspectFill) { livePhoto, _ in if let livePhoto = livePhoto { livePhotoView.livePhoto = livePhoto livePhotoView.startPlayback(with: .full) } } }
Memories offer another dimension of media interaction, automatically curating collections based on user behavior, locations, and events. To utilize Memories, a developer can create a PHAssetCollection and utilize the associated memories. While PhotoKit does not provide direct access to the underlying algorithms that generate Memories, you can display user-created collections through PHAssetCollection objects.
Here’s how you can fetch Memories from the user’s library:
func fetchMemories() { let fetchOptions = PHFetchOptions() fetchOptions.predicate = NSPredicate(format: "mediaType = %d", PHAssetMediaType.image.rawValue) let memories = PHAssetCollection.fetchAssetCollections(with: .moment, subtype: .albumRegular, options: fetchOptions) memories.enumerateObjects { (collection, _, _) in print("Found memory: (collection.localizedTitle ?? "Unknown Title")") } }
Displaying these memories in your app can greatly enhance user interaction, allowing users to relive significant moments through photo slideshows or curated galleries. As you implement features that leverage Live Photos and Memories, think how you can create a seamless user experience that encourages exploration and interaction with these rich media types.
Handling Permissions and Privacy Settings
When developing an application that interacts with the user’s photo library using the PhotoKit framework, handling permissions and privacy settings is a fundamental step. Privacy is paramount on iOS, and as a developer, it is essential to respect user data and ensure that you obtain the necessary permissions before accessing their photos and videos.
To start, you need to request authorization from the user to access their photo library. That is done using the PHPhotoLibrary.requestAuthorization(_:) method
. It’s crucial to handle various authorization statuses that the user can grant, such as granted, denied, restricted, or not determined. The approach here is to inform the user about why your application needs access, and you can do this through the Info.plist file.
In your Info.plist, you should include the following keys:
- A string that tells the user why your app needs access to their photo library.
- A string that explains why your app needs permission to add photos to the user’s library.
Once you’ve added these keys, you can implement the request for authorization as follows:
PHPhotoLibrary.requestAuthorization { status in switch status { case .authorized: print("Access granted to photo library.") case .denied, .restricted: print("Access denied to photo library.") case .notDetermined: print("Access not determined yet.") @unknown default: fatalError("Unknown authorization status.") } }
Handling the response appropriately very important. If access is granted, you can proceed to fetch and manage assets. However, if access is denied, you should gracefully inform the user about the limitations of the app’s functionality due to their privacy settings.
Furthermore, if your app requires the ability to add photos to the user’s library, you will need to handle the NSPhotoLibraryAddUsageDescription key as well. Without this, your application will not be able to save images or videos to the user’s library, resulting in a poor user experience.
When a user denies access, consider offering an alternative flow, such as guiding them to the app settings to manually enable permissions if they wish to use the photo-related features. You can also remind users of the benefits of granting access, which might encourage them to change their minds.
As a developer, it is also essential to monitor changes in the user’s photo library. For this, you’re required to handle PHPhotoLibraryChangeObserver
. By registering for changes, you can update your UI or handle changes in the assets without requiring the user to restart the app. Here’s how you can set it up:
let changeObserver = PHPhotoLibrary.shared().register(self) extension YourClass: PHPhotoLibraryChangeObserver { func photoLibraryDidChange(_ changeInstance: PHChange) { // Handle changes in the photo library } }
Finally, ponder that user privacy is an ongoing concern. Regularly remind users of how their data is being used and provide clear options for them to manage their own privacy settings within your app. By maintaining transparency and adhering to best practices regarding permissions, you can foster trust and ensure a positive user experience.