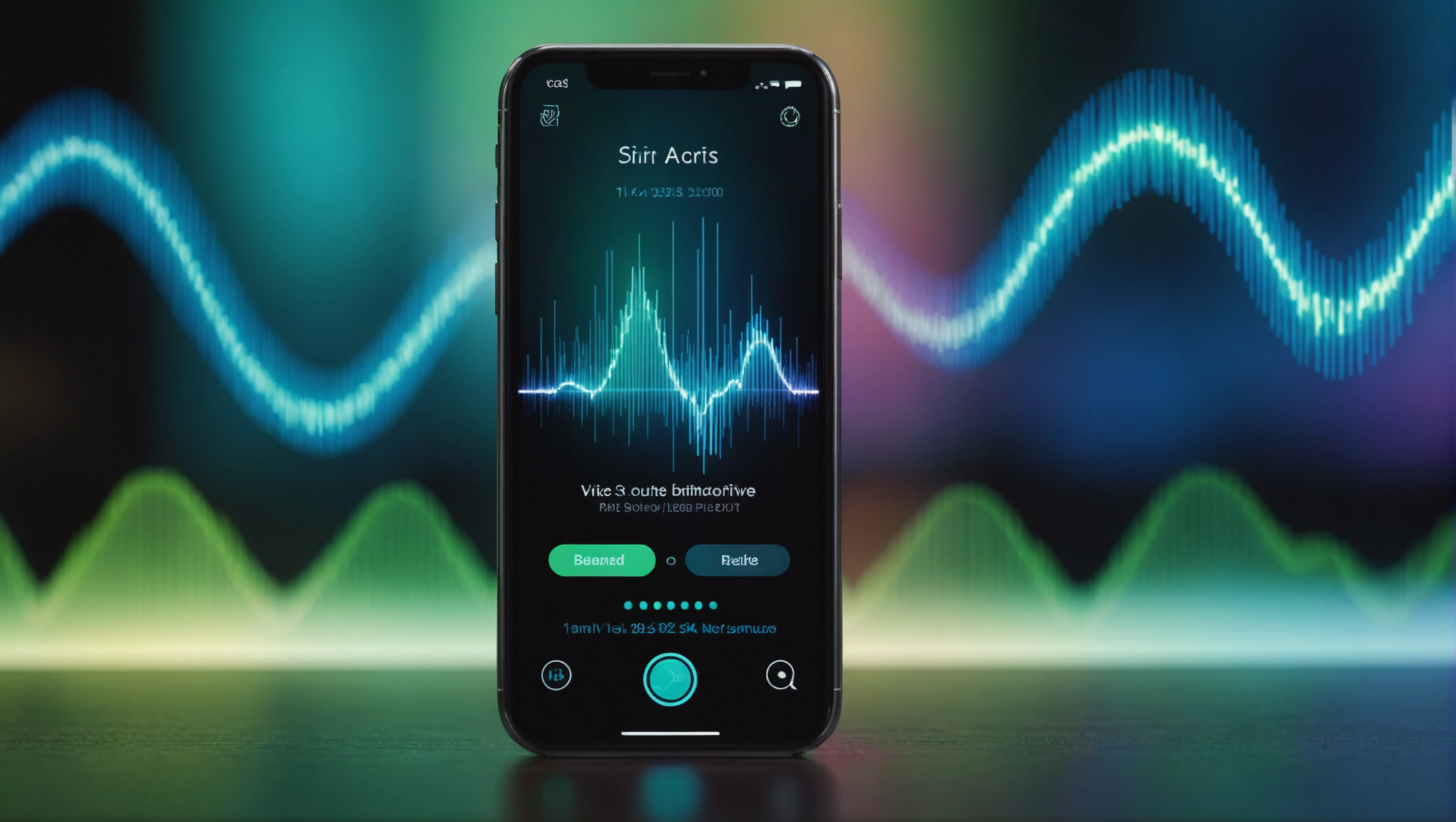
Swift and SiriKit
SiriKit is Apple’s framework that allows developers to integrate their apps with Siri, enabling users to interact with their apps through voice commands. This powerful feature enhances user engagement and provides a hands-free experience this is increasingly becoming a standard expectation in the mobile app ecosystem. At its core, SiriKit is designed to facilitate specific types of interactions, which are categorized into a number of domains, each with its own set of intents.
The primary components of SiriKit include:
- These are the specific actions that Siri can perform on behalf of the user. For example, intents might include sending a message, playing a song, or booking a ride. Each intent is defined by a set of parameters that describe the necessary information to complete the action.
- Developers define intents in an
.intentdefinition
file that specifies the parameters, options, and possible responses associated with each intent. This file acts as a bridge between your app and Siri, so that you can customize the user experience. - This is a separate target within your project that contains the logic for handling intents. When Siri receives an intent, it calls the appropriate method in your intents extension to execute the desired action, allowing your app to respond to user requests.
- For certain intents, Siri can display a custom user interface. This enables a richer interaction experience, particularly for intents that require user confirmation or additional information.
- That is implemented in the intents extension, where you define how your app processes the intents it receives. Handling includes validating input, performing the requested action, and providing a response back to Siri.
By understanding these components, developers can effectively harness the capabilities of SiriKit to create a seamless voice-driven experience within their applications. Using the right intents and ensuring that they’re well-defined in the intent definition file will significantly impact how users interact with Siri and, ultimately, the success of your app.
// Example of defining a custom intent in Swift import Intents class YourCustomIntentHandler: INExtension, YourCustomIntentHandling { func handle(intent: YourCustomIntent, completion: @escaping (YourCustomIntentResponse) -> Void) { // Handle the intent let response = YourCustomIntentResponse(code: .success, userActivity: nil) completion(response) } }
Integrating SiriKit into Your Swift Application
Integrating SiriKit into your Swift application is a multifaceted process that revolves around setting up the necessary components to enable seamless interactions between your app and Siri. The first step in this integration is to ensure that your app’s capabilities and entitlements are properly configured in Xcode.
To start, you must enable the Siri capability in your app’s target settings. This allows your app to utilize SiriKit’s functionalities. Go to your project settings, select your app target, navigate to the “Signing & Capabilities” tab, and add the “Siri” capability. This step is important as it grants your app the necessary permissions to respond to Siri requests.
Once the capability is enabled, the next step is to create an intents extension. This extension acts as the brain of your Siri integration, handling the intents that your app supports. In Xcode, you can add a new target to your project by selecting “Intents Extension”. This target contains the code that will process the requests made by Siri.
import Intents class IntentHandler: INExtension { override func handler(for intent: INIntent) -> Any? { // Return the handler for the specified intent if intent is YourCustomIntent { return YourCustomIntentHandler() } return nil } }
In the example above, the `IntentHandler` class overrides the `handler(for:)` method to return the appropriate handler for a given intent. This method very important for routing incoming intents to the proper handler, enabling Siri to execute the required functionality in your app.
After setting up the extension, the next task is to define your custom intents. That is achieved through the .intentdefinition file, where you specify the intents, their parameters, and possible responses. Xcode provides a graphical interface to facilitate this definition process, so that you can add intents and configure their attributes with ease.
Here is a brief example of how to define a custom intent:
import Intents class YourCustomIntentHandler: INExtension, YourCustomIntentHandling { func handle(intent: YourCustomIntent, completion: @escaping (YourCustomIntentResponse) -> Void) { // Process the intent and create a response let response = YourCustomIntentResponse.success(result: "Action was successful") completion(response) } }
This snippet showcases how to implement a basic intent handler. The `handle(intent:completion:)` method is where you perform the core functionality of your app in response to the user’s request. You will typically validate the input parameters here, execute the corresponding logic, and finally, construct a response to send back to Siri.
Another critical part of the integration process is ensuring that your app can be launched via Siri. You can enhance the user’s experience by providing a clear and simple voice command that triggers the desired functionality. To do this, you can use the `NSUserActivity` to create activities that inform Siri about what your app can do.
let activity = NSUserActivity(activityType: "com.yourcompany.yourapp.customAction") activity.title = "Custom Action" activity.userInfo = ["key": "value"] self.userActivity = activity self.userActivity?.becomeCurrent()
In this example, a `NSUserActivity` is created and marked as current, which allows Siri to recognize when the action is triggered. This capability enhances the app’s visibility to Siri and allows users to invoke it through voice commands.
As you proceed to implement these components, remember that thorough testing is key to ensuring a smooth integration. Using Xcode’s testing tools can help you simulate Siri interactions, allowing you to refine your intent handling logic before deploying your app.
Defining Custom Intents for Your App
Defining custom intents for your app within SiriKit is an important step in creating a voice-driven user experience that feels intuitive and seamless. Intents allow you to specify actions that your app can perform when requested by the user through Siri. The process begins with creating an .intentdefinition file, where you can outline the various intents your application will support.
Within this file, you can define each intent’s parameters and responses. Parameters are the data points required to fulfill the intent, while responses dictate how Siri communicates the outcome back to the user. To illustrate this, let’s ponder an example of a custom intent for sending a message.
import Intents // Define the custom intent in your .intentdefinition file // Parameters: recipient, message class SendMessageIntentHandler: INExtension, SendMessageIntentHandling { func handle(intent: SendMessageIntent, completion: @escaping (SendMessageIntentResponse) -> Void) { guard let recipient = intent.recipient, let message = intent.message else { completion(SendMessageIntentResponse(code: .failure, userActivity: nil)) return } // Assume sendMessage is a method that handles message sending logic let success = sendMessage(to: recipient, message: message) if success { completion(SendMessageIntentResponse(code: .success, userActivity: nil)) } else { completion(SendMessageIntentResponse(code: .failure, userActivity: nil)) } } private func sendMessage(to recipient: String, message: String) -> Bool { // Logic to send message print("Sending message: '(message)' to (recipient)") return true // Simulate a successful send } }
The `SendMessageIntentHandler` class implements the `SendMessageIntentHandling` protocol, which mandates the `handle(intent:completion:)` method. This method is where the core functionality of the intent is executed. The guard statement ensures that both the recipient and message parameters are present before proceeding with the sending logic. If either parameter is missing, a failure response is returned.
In addition to handling intents, defining custom intents also involves considering the interactions that could occur outside your app. Implementing voice shortcuts can enhance user engagement by allowing Siri to suggest relevant actions based on the user’s context or past behavior. To achieve this, you can create relevant `NSUserActivity` instances that encapsulate your app’s activities, making them easily accessible to Siri.
let activity = NSUserActivity(activityType: "com.yourcompany.yourapp.sendMessage") activity.title = "Send Message" activity.userInfo = ["recipient": "Nick Johnson", "message": "Hello!"] activity.isEligibleForPrediction = true self.userActivity = activity self.userActivity?.becomeCurrent()
In this example, the `NSUserActivity` is created with a specific type corresponding to sending a message. The `isEligibleForPrediction` property is set to true, allowing Siri to suggest this activity in relevant contexts, enhancing the app’s discoverability. By defining clear and actionable intents, you empower users to interact with your app using natural language, making your application feel more integrated into the iOS ecosystem.
As you work on defining your custom intents, take advantage of the graphical interface provided by Xcode to manage your .intentdefinition file. This interface allows you to visually organize intents, set parameters, and define responses, making it easier to visualize the intent structure and how they interact with your app’s functionality.
Ultimately, the thoughtful design of custom intents and their parameters can significantly influence how users experience your app through Siri. By crafting intuitive and effortless to handle interactions, you can ensure that your app stands out in a voice-first world.
Handling User Interactions with Siri
Handling user interactions with Siri is a pivotal aspect of integrating SiriKit into your Swift application. Once you’ve set up your intents and defined how they will be processed, the focus shifts to how your app responds to user requests in a meaningful and effective manner. The goal is to create a dialogue between your app and Siri that feels natural and intuitive for users, allowing them to complete tasks effortlessly.
When Siri receives a request, it triggers the intent handler you have defined in your intents extension. This is where you implement the logic for processing user input and executing the desired actions. Among the key considerations in handling user interactions are validating input, managing the workflow of your app’s functionalities, and returning appropriate responses to Siri.
First, let’s examine how to validate user input. It’s essential to ensure that the parameters provided by the user meet the criteria necessary for your app to perform the action successfully. For example, if you are handling a booking intent that requires a date and a location, you should check that these parameters are not only present but also valid.
func handle(intent: BookRideIntent, completion: @escaping (BookRideIntentResponse) -> Void) { guard let dateTime = intent.dateTime, let location = intent.location else { completion(BookRideIntentResponse(code: .failure, userActivity: nil)) return } // Proceed with booking the ride let success = bookRide(at: location, on: dateTime) if success { completion(BookRideIntentResponse.success(rideDetails: "Ride booked successfully!")) } else { completion(BookRideIntentResponse(code: .failure, userActivity: nil)) } }
In the code above, the `handle(intent:completion:)` method first checks whether the required parameters are present. If they are missing, a failure response is returned, preventing any further processing until valid data is provided. This validation step very important to ensure your app doesn’t attempt to perform actions based on incomplete or erroneous information.
Next, ponder the workflow of your app in response to the user’s request. That is where you implement the core functionality that fulfills the intent. You might need to call other methods, interact with your app’s backend, or update the user interface based on the outcome of the request. For instance, if the user requests to send a message, your app should handle the logic for message delivery and then provide a response back to Siri.
func handle(intent: SendMessageIntent, completion: @escaping (SendMessageIntentResponse) -> Void) { guard let recipient = intent.recipient, let message = intent.message else { completion(SendMessageIntentResponse(code: .failure, userActivity: nil)) return } let success = sendMessage(to: recipient, message: message) if success { completion(SendMessageIntentResponse(code: .success, userActivity: nil)) } else { completion(SendMessageIntentResponse(code: .failure, userActivity: nil)) } }
The `sendMessage` function encapsulates the logic for the message-sending process. Depending on its success or failure, the appropriate response is returned to Siri, which then communicates that outcome to the user. This ensures that users are informed about their actions, reinforcing a sense of control and awareness.
Another critical aspect of handling user interactions is the design of the response itself. Siri can communicate back to the user in various ways, including verbal feedback and visual updates. Crafting responses that are clear and informative enhances the user experience. For example, you can provide detailed confirmation or feedback messages that help users understand what happened as a result of their request.
func handle(intent: CheckOrderStatusIntent, completion: @escaping (CheckOrderStatusIntentResponse) -> Void) { // Assume getOrderStatus is a method that retrieves the status of an order let orderStatus = getOrderStatus(for: intent.orderID) if let status = orderStatus { completion(CheckOrderStatusIntentResponse.success(status: status)) } else { completion(CheckOrderStatusIntentResponse(code: .failure, userActivity: nil)) } }
In this example, the `CheckOrderStatusIntent` is processed by fetching the order status based on the user’s request. The user receives an update on their order, which reinforces the utility of your app and the effectiveness of Siri as an interface.
It’s also essential to think the context in which users interact with your application. Using `NSUserActivity` can allow Siri to suggest relevant actions based on previous user behavior. For instance, if a user frequently sends messages to a certain contact, Siri can suggest that action without needing the user to explicitly ask for it. This predictive functionality can significantly enhance user engagement.
let activity = NSUserActivity(activityType: "com.yourcompany.yourapp.sendMessage") activity.title = "Send Message to Nick Johnson" activity.userInfo = ["recipient": "Alex Stein", "message": "Hey!"] activity.isEligibleForPrediction = true self.userActivity = activity self.userActivity?.becomeCurrent()
By making your app’s functionalities easily accessible to Siri through well-defined intents and responsive handling logic, you create a powerful interaction model that emphasizes convenience and efficiency. The way your app manages user interactions with Siri can greatly influence the overall user experience, making it imperative to pay attention to every detail in the implementation.
Best Practices for Designing Siri Experiences
Designing great Siri experiences requires a thoughtful approach that combines user-centric design principles with the technical capabilities of SiriKit. Here are some best practices that can help you create intuitive, engaging, and effective interactions with Siri in your Swift application.
1. Keep User Intent in Mind
Understanding the user’s intent is paramount when designing Siri interactions. Ensure that your intents align closely with user expectations and common phrases they might use. Conduct user research to identify how potential users would naturally phrase their requests. This insight helps you capture the essence of user queries, making your app more accessible through voice commands.
For example, if users are likely to say, “Send a message to John,” your intent should accommodate variations of that phrase. Using synonyms and alternative phrasings in your intent definitions can enhance user satisfaction.
struct SendMessageIntent: INIntent { @NSManaged var recipient: String? @NSManaged var message: String? // Add additional parameters and variations as needed }
2. Provide Clear and concise Responses
When responding to user requests, clarity is key. Users should receive clear confirmation of actions taken or results obtained. Use simple language in your responses and avoid technical jargon. Here’s an example of how you might provide user feedback after sending a message:
func handle(intent: SendMessageIntent, completion: @escaping (SendMessageIntentResponse) -> Void) { // Assume message sending logic is contained in sendMessage method let success = sendMessage(to: intent.recipient, message: intent.message) if success { let response = SendMessageIntentResponse.success(result: "Your message has been sent.") completion(response) } else { let response = SendMessageIntentResponse.failure(error: "Failed to send your message.") completion(response) } }
3. Utilize Contextual Awareness
Leverage contextual data to imropve the relevance of your Siri interactions. For instance, if your app deals with calendar events, recognize the context in which a user makes a request. If a user asks, “What’s on my agenda today?” ensure that you’re providing relevant information based on the current date and user preferences.
func handle(intent: CheckAgendaIntent, completion: @escaping (CheckAgendaIntentResponse) -> Void) { let todayEvents = fetchEventsForDate(Date()) if !todayEvents.isEmpty { let response = CheckAgendaIntentResponse.success(events: todayEvents) completion(response) } else { let response = CheckAgendaIntentResponse.noEvents(message: "You have no events scheduled for today.") completion(response) } }
4. Design for Errors and Edge Cases
No interaction is perfect, and users may encounter issues. Anticipate potential errors by designing your intents and response logic to handle edge cases gracefully. Provide informative error messages that guide the user on what went wrong and how to rectify it.
func handle(intent: BookRideIntent, completion: @escaping (BookRideIntentResponse) -> Void) { guard let location = intent.location else { let response = BookRideIntentResponse.failure(error: "Please specify a pickup location.") completion(response) return } // Proceed with booking logic... }
5. Test with Real Users
Conduct usability testing with real users to gather feedback on how they interact with Siri through your app. Testing helps you identify friction points in the user experience and areas where users may struggle to get the results they expect. Use this feedback to refine your intents and improve the overall interaction quality.
6. Leverage Siri Shortcuts
Integrate Siri Shortcuts to enhance discoverability and ease of use. By allowing users to create personalized voice commands for specific actions, you empower them to interact with your app in a way that feels natural. Make it easy for users to set up these shortcuts, and provide clear instructions on how to do so.
func suggestShortcut() { let shortcut = INShortcut(intent: SendMessageIntent()) let shortcutViewController = INUIAddVoiceShortcutViewController(shortcut: shortcut) present(shortcutViewController, animated: true, completion: nil) }
By adhering to these best practices, you can create a Siri experience that not only meets user expectations but also exceeds them. The integration of voice interactions can significantly elevate your app’s usability, making it a more integral part of the user’s daily life.
Debugging and Testing SiriKit Implementations
Debugging and testing SiriKit implementations can be a nuanced endeavor, primarily due to the interactive and voice-driven nature of Siri. Ensuring that your app responds correctly to user intents requires a robust approach to testing and debugging. Here, we will explore effective strategies and techniques to streamline this process, helping you deliver a polished experience for your users.
The first step in debugging SiriKit interactions is to leverage Xcode’s excellent debugging tools. Start by placing breakpoints in your intent handler methods to observe the flow of execution when Siri invokes your intents. This allows you to inspect the parameters being passed and verify that your logic is executing correctly. For example:
func handle(intent: SendMessageIntent, completion: @escaping (SendMessageIntentResponse) -> Void) { // Set a breakpoint here to validate input parameters guard let recipient = intent.recipient, let message = intent.message else { let response = SendMessageIntentResponse(code: .failure, userActivity: nil) completion(response) return } // Proceed with sending the message let success = sendMessage(to: recipient, message: message) let response = success ? SendMessageIntentResponse.success(result: "Message sent.") : SendMessageIntentResponse.failure(error: "Message sending failed.") completion(response) }
This method helps you catch potential issues early, such as missing parameters or unexpected values, which can lead to failures when Siri attempts to process user requests.
Another critical aspect of testing is to utilize the Xcode UI Testing framework. You can create UI tests that simulate Siri interactions, which will allow you to verify that your app responds appropriately to various user inputs. For example, you can write tests that simulate sending a message through Siri:
func testSendMessageIntent() { let intent = SendMessageIntent() intent.recipient = "Alex Stein" intent.message = "Hello, John!" let expectation = self.expectation(description: "Handle SendMessageIntent") let handler = SendMessageIntentHandler() handler.handle(intent: intent) { response in XCTAssertEqual(response.code, .success) expectation.fulfill() } waitForExpectations(timeout: 5, handler: nil) }
By automating the testing of intents, you can ensure that your app behaves as expected across different scenarios, significantly reducing the likelihood of bugs slipping through to production.
In addition to unit and UI testing, think employing logging to capture detailed information about the interactions between your app and Siri. This can be invaluable when diagnosing issues that arise in production. Utilize NSLog or a logging framework to capture relevant details:
func handle(intent: SendMessageIntent, completion: @escaping (SendMessageIntentResponse) -> Void) { // Log the intent parameters for debugging purposes NSLog("Received SendMessageIntent with recipient: (String(describing: intent.recipient)), message: (String(describing: intent.message))") // Existing handling code... }
Logging provides visibility into the behavior of your intent handlers, which will allow you to track how parameters are processed and where potential issues might arise.
Another effective debugging strategy is to test your intents in different scenarios and edge cases. Think how users may invoke your intents and the various ways they might phrase their requests. Use Siri’s voice feedback to guide your testing process. By trying out different voice commands, you can identify how well Siri understands the user’s intent and whether your app handles the requests as expected. For instance, if you have an intent that expects a date, test how Siri responds when a user says, “Book a ride tomorrow” versus “Can you get me a ride for next Friday?”
Finally, keep in mind that real-world testing with users can provide insights that automated tests cannot. Conduct beta testing to gather feedback from actual users interacting with your app via Siri, and use their input to identify pain points or confusing interactions. This could include localized testing to understand how different accents and pronunciations affect Siri’s ability to understand user commands.
By applying these strategies—leveraging Xcode debugging tools, writing automated tests, using logging, testing varied user interactions, and engaging real users—you can effectively debug and test your SiriKit implementations, ensuring a seamless and satisfying user experience.