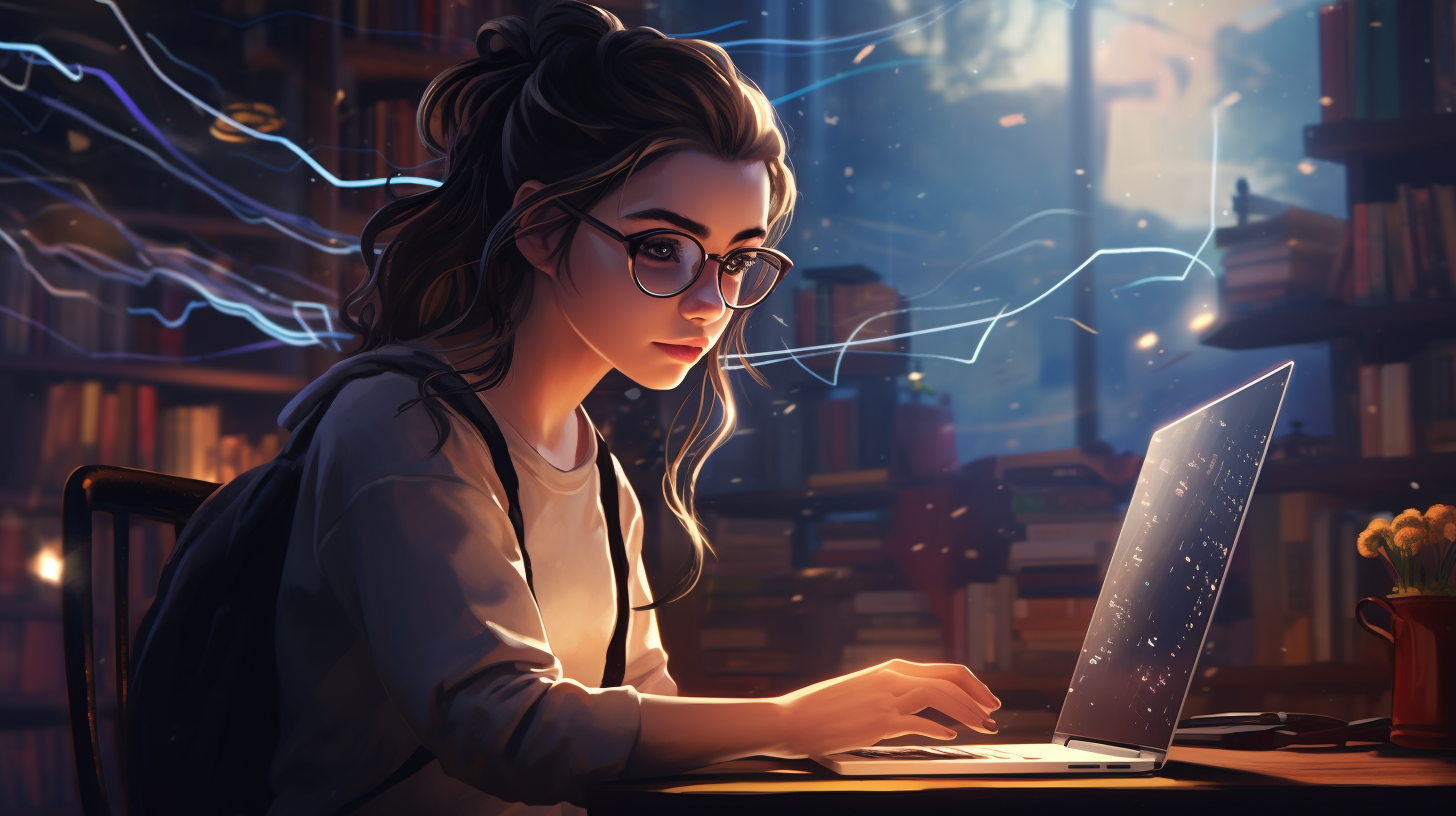
Swift and WKWebView
WKWebView is a powerful component introduced by Apple as part of the WebKit framework, designed to render web content directly within your iOS or macOS applications. It replaces the older UIWebView and comes with a host of improvements in performance and security. Understanding the functionality of WKWebView especially important for developers looking to create rich web-based experiences within their apps.
At its core, WKWebView provides a seamless way to load and display web pages. Unlike UIWebView, which had its share of performance bottlenecks, WKWebView is built on a modern architecture that uses a multi-process model. This ensures that the main application remains responsive while the web content is rendered in a separate process. This separation not only enhances performance but also improves security by sandboxing the web content away from your app’s main process.
WKWebView supports a wide variety of web technologies, including HTML5, CSS3, and JavaScript, making it suitable for rendering complex web applications. It also supports features like JavaScript execution, DOM manipulation, and the ability to communicate back and forth between your Swift code and the web content.
To create an instance of WKWebView, you start with the following code snippet:
import WebKit class ViewController: UIViewController { var webView: WKWebView! override func viewDidLoad() { super.viewDidLoad() // Initialize and configure the WKWebView webView = WKWebView(frame: self.view.bounds) webView.autoresizingMask = [.flexibleWidth, .flexibleHeight] // Load a URL let url = URL(string: "https://www.example.com")! let request = URLRequest(url: url) webView.load(request) // Add WKWebView to the view hierarchy self.view.addSubview(webView) } }
In the code above, we create a simple UIViewController subclass that initializes a WKWebView and loads a specified URL. The `autoresizingMask` property allows the web view to adapt to changes in its parent view’s size, which is essential for responsive layouts.
Additionally, WKWebView provides a robust set of delegates that allow developers to handle various events in the web view lifecycle, such as navigation actions, loading states, and errors. By conforming to the WKNavigationDelegate protocol, you can intercept and respond to these events effectively.
WKWebView is an advanced web rendering engine that enhances the way we integrate web content into our applications. Its improved performance, security features, and easy integration make it an indispensable tool for modern iOS and macOS development.
Implementing WKWebView in Your Project
To implement WKWebView effectively in your project, you can follow a structured approach that encompasses initializing the web view, configuring it for various use cases, and managing its lifecycle. Here, we’ll dive deeper into the necessary steps and considerations to ensure a smooth integration.
First, make sure you import the WebKit framework. That is essential as WKWebView resides within this framework. The setup begins in your view controller, where you can instantiate the WKWebView. It’s also critical to set the navigation delegate to manage different web view events effectively.
import UIKit import WebKit class ViewController: UIViewController, WKNavigationDelegate { var webView: WKWebView! override func viewDidLoad() { super.viewDidLoad() // Initialize and configure the WKWebView webView = WKWebView(frame: self.view.bounds) webView.autoresizingMask = [.flexibleWidth, .flexibleHeight] webView.navigationDelegate = self // Set the navigation delegate // Load a URL let url = URL(string: "https://www.example.com")! let request = URLRequest(url: url) webView.load(request) // Add WKWebView to the view hierarchy self.view.addSubview(webView) } // WKNavigationDelegate methods func webView(_ webView: WKWebView, didFinish navigation: WKNavigation!) { print("Finished loading") } func webView(_ webView: WKWebView, didFail navigation: WKNavigation!, withError error: Error) { print("Failed to load with error: (error.localizedDescription)") } }
In this example, we not only create a WKWebView instance but also set up the navigation delegate to respond to events. The methods `webView(_:didFinish:)` and `webView(_:didFail:withError:)` are implemented to provide feedback on the loading state of the web content.
When dealing with links in your web view, you might want to control how navigation occurs, especially to ensure that links open within the WKWebView rather than in Safari. You can do this by implementing the `webView(_:decidePolicyFor:decisionHandler:)` method from the WKNavigationDelegate protocol:
func webView(_ webView: WKWebView, decidePolicyFor navigationAction: WKNavigationAction, decisionHandler: @escaping (WKNavigationActionPolicy) -> Void) { if let url = navigationAction.request.url, url.scheme == "https" { // Allow the navigation decisionHandler(.allow) } else { // Block the navigation decisionHandler(.cancel) } }
This method allows you to inspect the navigation action and decide whether to allow or cancel it based on specific criteria. In this case, we are permitting only HTTPS links to be loaded within the WKWebView.
Additionally, you can monitor the loading progress of your content by using the `estimatedProgress` property of WKWebView. You might want to display a loading indicator to improve user experience:
override func viewDidLoad() { super.viewDidLoad() webView.addObserver(self, forKeyPath: "estimatedProgress", options: .new, context: nil) } override func observeValue(forKeyPath keyPath: String?, of object: Any?, change: [NSKeyValueChangeKey : Any]?, context: UnsafeMutableRawPointer?) { if keyPath == "estimatedProgress" { print("Loading progress: (webView.estimatedProgress)") } } deinit { webView.removeObserver(self, forKeyPath: "estimatedProgress") }
By observing the `estimatedProgress`, you can react to changes, such as updating a UIProgressView or a loading spinner, providing users with a clear indication of the web content’s loading status.
With these fundamental implementation steps, you can successfully integrate WKWebView into your iOS or macOS applications. The configuration and delegation patterns not only enhance performance but also enrich user interaction, allowing for a more dynamic app experience.
Handling Navigation and User Interactions
Handling navigation and user interactions in WKWebView is a pivotal aspect that allows you to create a responsive and interactive web experience within your application. By using the power of the WKNavigationDelegate protocol, you can gain granular control over the navigation flow and user interactions, so that you can tailor the web content experience to fit your app’s design and functionality.
To effectively manage navigation, it’s essential to implement several key delegate methods. These methods give you insight into the lifecycle of web content loading, enabling you to respond appropriately based on user actions or the state of the content being loaded. For example, when a user taps a link, you might want to intercept that action to either allow or prevent navigation based on certain conditions.
The following code shows how to implement this navigation control:
func webView(_ webView: WKWebView, decidePolicyFor navigationAction: WKNavigationAction, decisionHandler: @escaping (WKNavigationActionPolicy) -> Void) { if let url = navigationAction.request.url { // Here you can inspect the URL and decide whether to allow the navigation if url.host == "www.example.com" { decisionHandler(.allow) // Allow navigation to this site } else { decisionHandler(.cancel) // Prevent navigation to other sites } } else { decisionHandler(.cancel) // Cancel if URL is nil } }
In this method, we check the host of the URL being navigated to. If it matches a specific domain, we allow the navigation; otherwise, we cancel it. This approach can help maintain user focus within your application and ensure that they don’t accidentally navigate away to unwanted sites.
Moreover, you can handle user interactions with embedded JavaScript by using message handlers. This allows communication between the web content and your Swift code, which can be particularly useful for responding to events triggered by user actions. To do this, you need to set up a message handler:
class ViewController: UIViewController, WKScriptMessageHandler { var webView: WKWebView! override func viewDidLoad() { super.viewDidLoad() let contentController = WKUserContentController() contentController.add(self, name: "appHandler") let config = WKWebViewConfiguration() config.userContentController = contentController webView = WKWebView(frame: self.view.bounds, configuration: config) webView.autoresizingMask = [.flexibleWidth, .flexibleHeight] self.view.addSubview(webView) // Load your HTML content that includes a reference to this handler let htmlString = """ """ webView.loadHTMLString(htmlString, baseURL: nil) } func userContentController(_ userContentController: WKUserContentController, didReceive message: WKScriptMessage) { if message.name == "appHandler" { print("Received message: (message.body)") // Handle the message from JavaScript } } }
In this example, we create a button in our HTML content that, when clicked, sends a message back to the native Swift code. The `userContentController(_:didReceive:)` method handles these messages, which will allow you to execute specific code in response to user interactions occurring in the web content.
Another important consideration involves the back and forward navigation. You can implement basic back and forward functionality by using the web view’s built-in navigation methods:
@IBAction func goBack(_ sender: Any) { if webView.canGoBack { webView.goBack() } } @IBAction func goForward(_ sender: Any) { if webView.canGoForward { webView.goForward() } }
These methods check if the web view can navigate back or forward in the history stack and perform the navigation if possible. By providing buttons or gestures for these actions, you enhance the web browsing experience within your app, making it feel more like a native application.
Lastly, handling user interactions also extends to managing errors and loading states. Implementing the `webView(_:didFail:withError:)` method allows you to provide feedback to users when navigation fails, ensuring they understand what went wrong and possibly offering them options to retry:
func webView(_ webView: WKWebView, didFail navigation: WKNavigation!, withError error: Error) { // Notify the user of the error let alert = UIAlertController(title: "Error", message: error.localizedDescription, preferredStyle: .alert) alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil)) present(alert, animated: true, completion: nil) }
This method presents an alert to the user when an error occurs, providing a clear and effortless to handle way to handle navigation issues. Understanding and implementing these various aspects of navigation and user interactions will significantly enhance the functionality and user experience of your app when using WKWebView.
Best Practices for WKWebView Performance and Security
When it comes to optimizing the performance and security of your WKWebView implementation, there are several best practices that developers should adhere to. These practices not only improve the overall user experience but also help safeguard your application from potential vulnerabilities associated with web content. Here’s a detailed look at how to ensure your WKWebView is both efficient and secure.
1. Use a WKWebViewConfiguration
Always create a customized WKWebViewConfiguration
instance when initializing your WKWebView. This allows you to set various options such as enabling JavaScript, managing content controllers, and more. A well-configured web view can lead to improved performance and a more controlled environment for your web content.
let config = WKWebViewConfiguration() config.preferences.javaScriptEnabled = true webView = WKWebView(frame: self.view.bounds, configuration: config)
By explicitly configuring JavaScript preferences, you ensure that only necessary features are enabled, minimizing unnecessary resource consumption.
2. Implement Content Security Policies
To protect against cross-site scripting (XSS) attacks, you can implement Content Security Policies (CSP) within your web content. This involves sending a CSP header from your server that restricts resources the browser is allowed to load. For instance, you could specify that scripts can only be loaded from your domain.
let htmlString = """ <html> <head> <meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self'"> </head> <body> <h1>Hello, Secure World!</h1> </body> </html> """ webView.loadHTMLString(htmlString, baseURL: nil)
By doing this, you minimize exposure to potentially malicious content from third-party sources.
3. Limit Network Access
Control network access by using a custom WKURLSchemeHandler
for specific URL schemes. This allows you to intercept requests and ensure that only validated requests are sent out. You can implement domain whitelisting to restrict navigation to a predefined list of URLs.
class MyURLSchemeHandler: NSObject, WKURLSchemeHandler { func handle(_ request: WKURLSchemeTask) { // Validate request and manage loading } } let config = WKWebViewConfiguration() config.setURLSchemeHandler(MyURLSchemeHandler(), forURLScheme: "myscheme")
This approach not only enhances security but also provides a pathway for implementing advanced networking features.
4. Use Safe JavaScript Practices
When working with JavaScript, ensure that it does not execute untrusted code. Use message passing cautiously and validate any data sent between the web content and your native code. This very important to prevent injection attacks that can manipulate your application’s behavior.
func userContentController(_ userContentController: WKUserContentController, didReceive message: WKScriptMessage) { guard let safeData = message.body as? String else { return } // Process safeData after validation }
By validating incoming data, you can avoid executing potentially harmful scripts that could exploit your app.
5. Monitor and Limit Memory Usage
WKWebView can consume considerable memory, especially when displaying complex web pages or running JavaScript-heavy applications. Monitor memory usage and clear caches when necessary. You can also use WKWebsiteDataStore
to manage caching and reduce the footprint of your web view.
let dataStore = WKWebsiteDataStore.default() dataStore.removeData(ofTypes: [.memoryCache], modifiedSince: Date(timeIntervalSinceNow: -86400)) { print("Cache cleared.") }
Regularly clearing cache helps to manage memory and improve performance, particularly in apps that frequently load web content.
6. Handle Errors Gracefully
Implement error handling for network requests and web view actions. Utilize the webView(_:didFail:withError:)
method to provide feedback to users when something goes wrong. This ensures that users are not left confused and allows you to log errors for troubleshooting.
func webView(_ webView: WKWebView, didFail navigation: WKNavigation!, withError error: Error) { let alert = UIAlertController(title: "Error", message: error.localizedDescription, preferredStyle: .alert) alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil)) present(alert, animated: true, completion: nil) }
This practice not only boosts user trust but also provides essential insights into issues that may arise during web content loading.
7. Enable App Transport Security (ATS)
Ensure that App Transport Security (ATS) is configured correctly in your app’s Info.plist file. ATS requires that all network connections use HTTPS, which provides encryption and security for data in transit. That’s critical in today’s environment, where data breaches are increasingly common.
By adhering to these best practices, you can significantly enhance the performance and security of your WKWebView implementation. A well-structured approach not only safeguards your application but also ensures a smooth and responsive user experience, contributing to the overall success of your app.