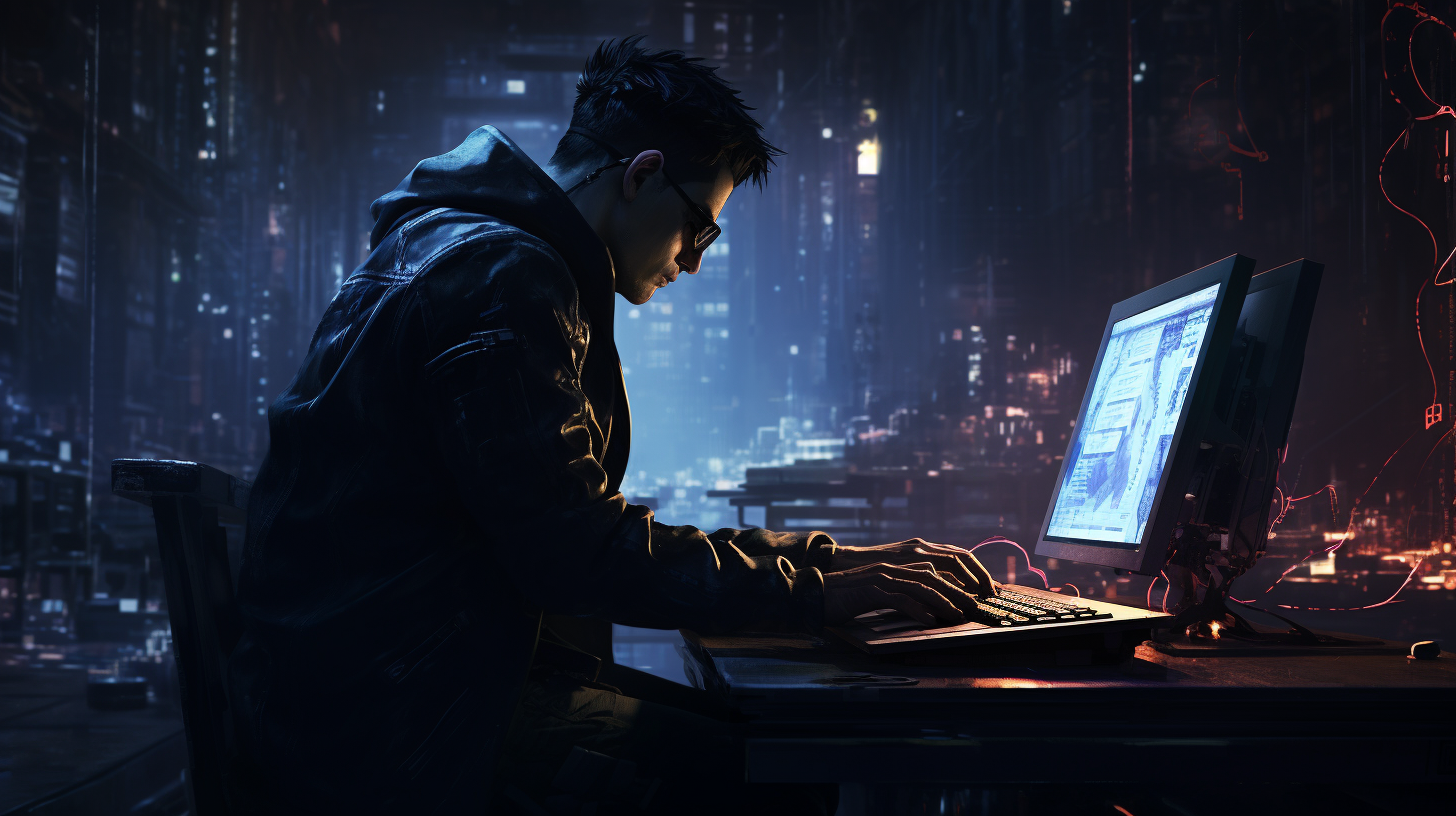
Swift Collections: Dictionaries
Dictionaries in Swift are an essential and versatile collection type that allows you to store and manage data as key-value pairs. They provide a way to associate unique keys with corresponding values, enabling efficient data retrieval and manipulation. The power of dictionaries lies in their ability to offer fast access to values by their keys, making them a preferred choice for many scenarios where lookups are frequent.
A dictionary in Swift is defined using the syntax [KeyType: ValueType]
, where KeyType
is the type of the keys and ValueType
is the type of the values. Swift dictionaries are unordered, which means that the items do not have a defined order, and the same key can only exist once in a dictionary.
One of the key aspects of dictionaries is that they’re type-safe. This means that both the keys and values must be of a specific type, leading to safer and more predictable code. Additionally, Swift dictionaries are mutable by default, meaning you can add, remove, and change the values associated with keys after the dictionary has been created.
To illustrate the concept further, consider the following code example that demonstrates how to declare a dictionary, initialize it with values, and access its contents:
var capitals: [String: String] = [ "France": "Paris", "Italy": "Rome", "Germany": "Berlin" ] // Accessing a value using its key if let capitalOfItaly = capitals["Italy"] { print("The capital of Italy is (capitalOfItaly).") }
In this example, the capitals
dictionary maps country names (as keys) to their respective capital cities (as values). The dictionary is initialized with three entries. Accessing a value is done by using the subscript syntax with the key, which returns an optional value. If the key is not found, the result will be nil
.
Understanding dictionaries is fundamental for using their capabilities in various programming tasks. They serve as a powerful tool for managing collections of related data, enabling you to write more organized and efficient Swift code.
Creating and Initializing Dictionaries
Creating and initializing dictionaries in Swift can be done in several simpler ways, allowing for flexibility depending on the specific use case. You can initialize an empty dictionary, create a dictionary with a literal, or use a dictionary type with a specified type declaration.
To create an empty dictionary, you can use the following syntax:
var emptyDictionary: [String: Int] = [:]
This declaration creates an empty dictionary called emptyDictionary
that is intended to hold String
keys and Int
values. You can also use the Dictionary()
initializer:
var anotherEmptyDictionary = Dictionary()
Both methods achieve the same result of initializing an empty dictionary, but the choice between them often comes down to personal preference or code readability.
Another common way to create and initialize a dictionary is by using a dictionary literal. This method is efficient and allows you to populate the dictionary with key-value pairs upon initialization. Here’s an example:
var fruits: [String: String] = [ "apple": "A sweet red or green fruit.", "banana": "A long yellow fruit.", "cherry": "A small round fruit, often red." ]
In this case, the dictionary fruits
is initialized with three entries, each mapping a fruit name to a brief description. This approach is concise and illustrates the key-value relationship in a clear manner.
Swift also supports using a tuple to initialize a dictionary. This approach is handy when you want to create a dictionary from a sequence of key-value pairs, such as arrays of tuples:
let fruitArray = [("apple", "A sweet red or green fruit."), ("banana", "A long yellow fruit.")] var fruitDictionary = Dictionary(uniqueKeysWithValues: fruitArray)
In this example, the fruitArray
contains tuples of fruit names and their descriptions. The Dictionary(uniqueKeysWithValues:)
initializer creates a dictionary directly from the array of tuples.
These various methods of creating and initializing dictionaries provide flexibility and efficiency, enabling developers to choose the approach that best suits their coding style and requirements. Understanding how to effectively create and initialize dictionaries very important for using their power in Swift programming.
Accessing and Modifying Dictionary Values
Accessing and modifying values in dictionaries is an important skill that enhances your ability to manage data effectively in Swift. Given that dictionaries use unique keys to store values, accessing an individual value is simpler and intuitive. You can gain access to a value by using the key in a subscript operation. If the key exists in the dictionary, you will receive the corresponding value; if not, the result will be nil, as shown in the previous example.
For instance, think a scenario where you want to retrieve the capital of a country:
if let capitalOfFrance = capitals["France"] { print("The capital of France is (capitalOfFrance).") } else { print("Country not found.") }
In this snippet, we safely unwrap the optional value returned by the dictionary. The use of optional binding (if let) ensures that we handle the case where the key might not exist without crashing our program.
Modifying dictionary values is equally simpler. You can update the value for a specific key by simply assigning a new value to that key. If the key already exists, the associated value will be replaced. Here’s an example:
capitals["Italy"] = "Roma" // Update the capital of Italy print(capitals["Italy"]!) // Output: Roma
In this code, we replaced the capital of Italy from “Rome” to “Roma”. Note the use of the force unwrap operator (!) to access the new value directly since we know the key exists.
Additionally, if you want to add a new key-value pair to the dictionary, the same subscript syntax can be utilized. Here’s how you can add a new entry:
capitals["Spain"] = "Madrid" // Add the capital of Spain
To remove a key-value pair from the dictionary, you can use the removeValue(forKey:) method:
capitals.removeValue(forKey: "Germany") // Remove Germany from the dictionary
This method returns the value that was removed, which will allow you to handle it as needed. If the key does not exist, the method returns nil.
When working with dictionaries, it’s important to think the implications of these operations. Modifying a dictionary is a constant-time operation, which makes it highly efficient for real-time data manipulation. However, remember that since dictionaries are unordered collections, the sequence in which keys are stored or returned is not guaranteed.
Swift provides a robust mechanism for accessing and modifying dictionary values. By using subscripting and dedicated methods, you can efficiently manage the data stored in dictionaries, making it an indispensable tool in your programming arsenal.
Common Dictionary Operations and Use Cases
When working with dictionaries in Swift, you often encounter situations that require common operations like iteration, filtering, and transforming the key-value pairs. These operations are not only vital for data manipulation but also enhance the usability of dictionaries in various programming scenarios.
One of the most fundamental operations is iterating over the key-value pairs in a dictionary. You can achieve this conveniently using a for-in loop, which allows you to access each key-value pair in the dictionary. Here’s an example demonstrating how to iterate through the ‘capitals’ dictionary:
for (country, capital) in capitals { print("The capital of (country) is (capital).") }
In this code snippet, the loop traverses each entry in the ‘capitals’ dictionary, unpacking the country and capital into separate variables. This approach provides a simpler method to access both the keys and values concurrently.
Another common operation is filtering the dictionary. Swift’s higher-order functions like filter
can help you create a new dictionary based on specific criteria. For instance, if you want to filter out capitals that are shorter than 6 characters, you could do the following:
let filteredCapitals = capitals.filter { $0.value.count < 6 }
This code uses the filter
method to generate a new dictionary named filteredCapitals
that includes only those entries where the length of the capital’s name is less than 6 characters. The closure $0.value.count < 6
serves as the filtering condition.
Transforming a dictionary into another collection type is also a prevalent use case. You can utilize the map
function to create an array or another dictionary based on the existing key-value pairs. Here’s how you can create an array of capital names from the ‘capitals’ dictionary:
let capitalNames = capitals.map { $0.value }
In this example, map
iterates through each entry in the dictionary, and the closure $0.value
extracts the capital name. The result is stored in an array called capitalNames
.
Another essential operation is checking for the existence of a key within the dictionary. You can use the contains
method in combination with the keys
property to determine if a specific key is present:
if capitals.keys.contains("France") { print("France is in the dictionary.") }
This snippet verifies whether the key “France” exists in the ‘capitals’ dictionary, allowing you to handle the data appropriately based on the result.
Swift dictionaries also allow you to merge two dictionaries seamlessly. The merge
method can combine the contents of two dictionaries, specifying how to handle duplicate keys. Here’s an example:
var moreCapitals: [String: String] = ["Spain": "Madrid", "Portugal": "Lisbon"] capitals.merge(moreCapitals) { (current, _) in current }
In this code, the merge
method combines moreCapitals
into the capitals
dictionary. The closure { (current, _) in current }
dictates that if a duplicate key exists, the current value is preserved, and the new value is discarded.
These common operations showcase the versatility and power of dictionaries in Swift. By using iteration, filtering, transformation, existence checking, and merging, you can manipulate data effectively, making dictionaries an invaluable asset in your programming toolkit.