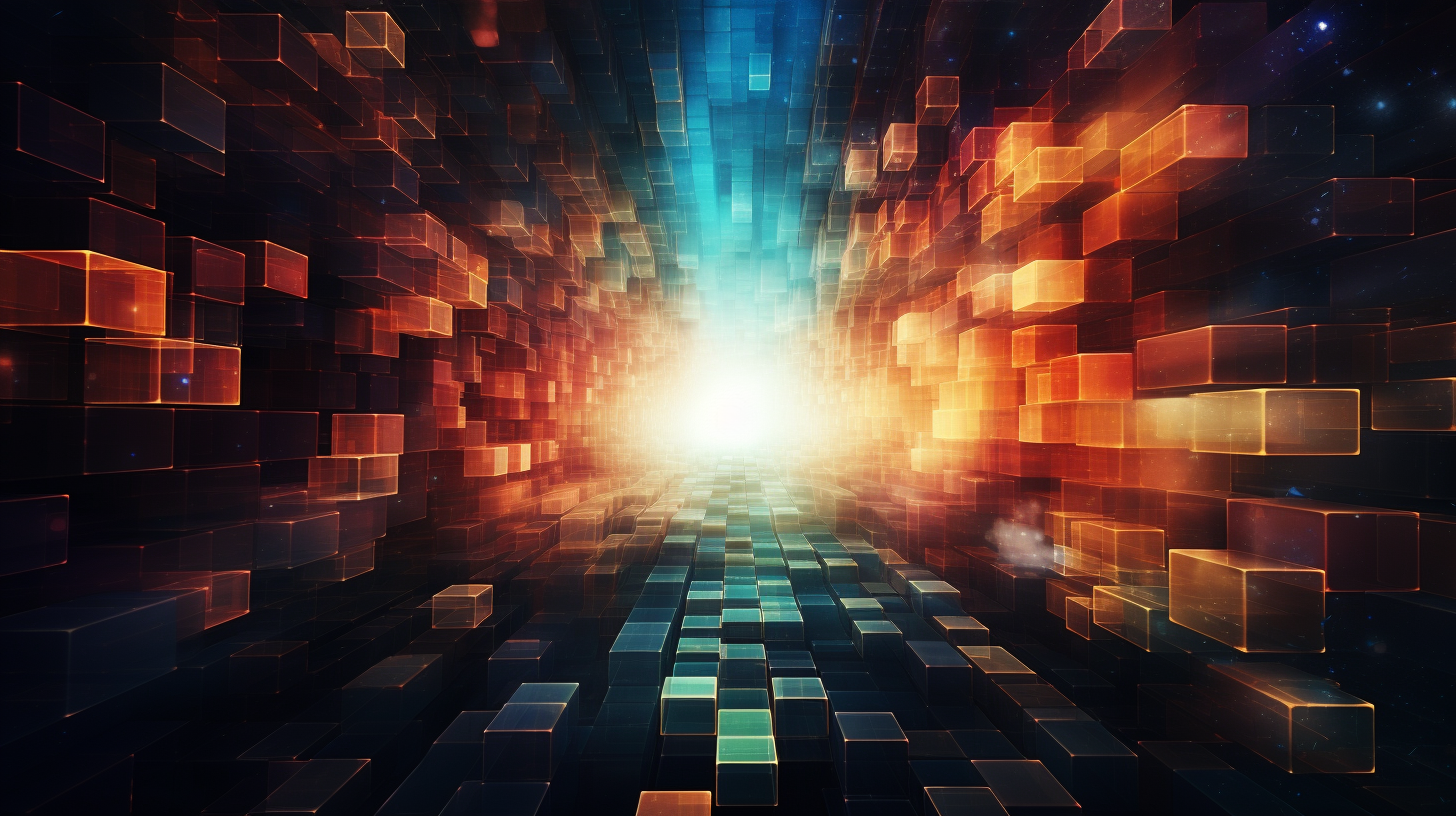
Swift for watchOS Development
To effectively develop applications for watchOS, it’s imperative to grasp the underlying architecture that differentiates it from other Apple platforms. WatchOS runs on a unique operating system designed specifically for the Apple Watch, focusing on performance, battery life, and user interaction. The architecture is structured around a hierarchy of components that work cohesively to deliver a seamless experience.
At the core of watchOS is the WatchKit framework, which allows developers to create watchOS apps that can interact with the paired iPhone. This interaction very important because many watchOS apps are lightweight and rely on the iPhone for heavy processing tasks.
WatchOS applications operate in two primary modes: WatchKit App and WatchKit Extension. The WatchKit App is the user interface that runs on the Apple Watch, while the WatchKit Extension runs on the paired iPhone. This separation ensures that the Apple Watch remains responsive and uses minimal resources.
One of the key features of this architecture is the use of Interface Controllers, which manage the app’s user interface. These controllers are lightweight and designed to handle the limited screen space and interaction paradigms of the watch.
Communication between the WatchKit App and the WatchKit Extension is facilitated through a defined communication protocol. This allows data to be sent back and forth efficiently, minimizing lag and maintaining a fluid user experience. Developers can leverage the WCSession
class to send messages, transfer files, and manage application context between the watch and the phone.
import WatchConnectivity class SessionManager: NSObject, WCSessionDelegate { static let shared = SessionManager() private override init() { super.init() setupSession() } private func setupSession() { if WCSession.isSupported() { let session = WCSession.default session.delegate = self session.activate() } } func session(_ session: WCSession, didReceiveMessage message: [String : Any]) { // Handle incoming messages from iPhone print("Received message: (message)") } }
In addition to WatchKit, watchOS also integrates deeply with various frameworks like HealthKit for health-related applications and Core Motion for tracking activity data. This allows developers to create rich, interactive, and health-focused experiences that leverage the unique capabilities of the Apple Watch.
Another aspect to ponder is the App Lifecycle. WatchOS apps have a distinct lifecycle compared to iOS applications. They can transition between active and inactive states, responding to user interactions or notifications. This lifecycle management very important for optimizing performance and ensuring that the app responds quickly to user inputs.
class InterfaceController: WKInterfaceController { override func awake(withContext context: Any?) { super.awake(withContext: context) // Perform initialization } override func willActivate() { // The view is about to be visible to the user } override func didDeactivate() { // The view is no longer visible } }
Understanding this architecture is fundamental to building efficient and responsive watchOS applications. Emphasizing the interaction between the WatchKit App and the WatchKit Extension, alongside the integration with other frameworks, lays the groundwork for any dev to create compelling experiences on the Apple Watch.
Setting Up Your Development Environment
Before diving into the actual coding of your watchOS application, it’s essential to set up your development environment correctly. This preparation ensures that you can leverage all the tools and frameworks available for watchOS development. The first step is to install Xcode, Apple’s integrated development environment (IDE), which provides all the necessary tools for building, testing, and deploying watchOS applications.
To get started, download the latest version of Xcode from the Mac App Store. Once installed, Xcode will also provide you with the iOS SDK, which is integral for developing applications that communicate with your Apple Watch. Make sure to check for updates regularly, as Apple frequently releases new features and bug fixes that enhance both Xcode and the watchOS SDK.
Once you have Xcode up and running, you’ll need to create a watchOS project. Open Xcode and select “Create a new Xcode project.” In the template chooser, navigate to the ‘watchOS’ section and select the ‘App’ template. This template sets up a basic structure that includes both a WatchKit App and a WatchKit Extension, which will allow you to manage the user interface and business logic separately.
// That is a sample code snippet for creating a new watchOS project in Xcode. let project = Project(name: "MyWatchApp") project.addTarget("MyWatchApp WatchKit App") project.addTarget("MyWatchApp WatchKit Extension")
After setting up the project, ensure that you have the appropriate provisioning profiles and certificates configured. You’ll need an Apple Developer account to deploy your app to a physical device, which is essential for testing the performance and responsiveness of your application. Go to the Apple Developer website to enroll and manage your certificates.
Next, you should familiarize yourself with Xcode’s Interface Builder. This tool allows you to design your app’s user interface visually. You can drag and drop elements such as buttons, labels, and images into your WatchKit App’s storyboard. Keep in mind the limited screen real estate of the Apple Watch; design with simplicity and clarity in mind.
// Sample code for creating a button in your WatchKit App's interface. @IBOutlet weak var myButton: WKInterfaceButton! @IBAction func buttonTapped() { // Handle button tap action print("Button was tapped!") }
Testing your watchOS application is equally important. Xcode offers an excellent simulator for watchOS, which allows you to see how your app will perform on a virtual Apple Watch. However, using an actual device very important for a definitive experience, as it reveals nuances in performance and user interaction that simulators may not capture. Connect a physical Apple Watch via the paired iPhone to your development environment and select it as the run destination in Xcode.
Finally, be sure to leverage the extensive documentation provided by Apple. The watchOS Developer Documentation is a treasure trove of information, including sample projects, guidelines for best practices, and insights into optimizing your app for performance and user experience. Referencing these resources throughout your development process will help you navigate the complexities of watchOS and make informed decisions.
By setting up your development environment properly, you lay the groundwork for creating high-quality watchOS applications that can deliver robust functionality while providing an excellent user experience. With the right tools at your disposal, you’re ready to embark on the journey of watchOS app development.
Creating Your First watchOS App
Creating your first watchOS app is an exciting venture that allows you to explore the unique capabilities of the Apple Watch platform. Once you have your development environment set up, the next step is to craft a functional and engaging application that serves a specific purpose. In this section, we will walk through the essential steps to create a basic watchOS app, using Xcode and the WatchKit framework.
To kick things off, let’s start by creating a new watchOS project in Xcode. Open Xcode and select “Create a new Xcode project.” Choose the “App” template under the watchOS section. This template will automatically configure the necessary components for you, including the WatchKit App and the WatchKit Extension.
// This code snippet showcases how to define a new watchOS project setup let project = Project(name: "MyFirstWatchApp") project.addTarget("MyFirstWatchApp WatchKit App") project.addTarget("MyFirstWatchApp WatchKit Extension")
After the project is created, you’ll be greeted by a series of files that organize your code and resources. The main components you’ll work with include the Interface.storyboard file for designing your user interface and the InterfaceController.swift file for handling user interactions.
Next, let’s focus on designing the user interface. Open the Interface.storyboard file, where you can drag and drop interface elements onto your watch app screen. For our example, let’s add a label to display a greeting and a button that, when tapped, changes the label text.
To add a label and button, drag a WKInterfaceLabel and a WKInterfaceButton from the object library into your interface. After placing the elements, create IBOutlet and IBAction connections to your InterfaceController.swift file.
@IBOutlet weak var greetingLabel: WKInterfaceLabel! @IBAction func greetButtonTapped() { greetingLabel.setText("Hello, watchOS!") }
In the above code, the greetingLabel IBOutlet allows us to reference the label in our code. The greetButtonTapped action is triggered when the user taps the button, updating the label’s text accordingly.
After designing the interface and writing the necessary code, it’s crucial to test your app. Xcode’s watchOS simulator provides a simpler way to run your app on a virtual Apple Watch. However, testing on a real device is recommended for evaluating performance and user experience more accurately.
To run your app, select your watchOS target in Xcode and click the run button. Once the simulator launches, you’ll be able to see your interface, interact with the button, and observe the label update dynamically. This process will give you an initial feel for how your app performs on the Apple Watch.
Remember, as you develop your app, ponder the limitations of the watchOS platform. Design your interface for quick interactions and focus on providing immediate feedback. Given the watch’s small screen and limited processing power, simplicity is key.
After achieving a working version of your first watchOS app, you may wish to improve its functionality by integrating additional features, such as notifications, watch complications, or health data from HealthKit. The groundwork you’ve laid by creating this initial app will serve as a solid foundation as you expand your knowledge and skills in watchOS development.
Designing User Interfaces for watchOS
Designing user interfaces for watchOS requires a distinct approach, emphasizing clarity and efficiency due to the limited screen real estate of the Apple Watch. Unlike traditional iOS applications that can utilize more complex layouts and interactions, watchOS apps must prioritize simplicity and user engagement.
The primary building blocks for a watchOS user interface are found in the WatchKit framework, which provides a set of interface objects that can be easily integrated into your application. These objects include labels, buttons, sliders, and pickers, all designed to be lightweight and optimized for a smaller screen.
When designing an interface, start by considering the context in which your app will be used. Users interact with their watches in quick, glanceable moments. Therefore, every screen should communicate its purpose immediately. Use clear text and intuitive visuals to guide users effectively. Avoid cluttering the interface with too many elements; instead, focus on the most important actions and information.
Moreover, the use of gestures especially important in watchOS. Taps and swipes are the primary methods of interaction, so your design should accommodate these gestures seamlessly. For example, creating a simple menu with buttons that users can tap to navigate or trigger actions is an effective way to enhance usability.
To illustrate the design process, let’s create a basic user interface for a fitness tracking app. This app will display the user’s step count and allow them to track their daily goal. We will utilize a WKInterfaceLabel for displaying the step count and a WKInterfaceButton to reset the steps.
@IBOutlet weak var stepCountLabel: WKInterfaceLabel! @IBOutlet weak var resetButton: WKInterfaceButton! var stepCount: Int = 0 @IBAction func resetButtonTapped() { stepCount = 0 updateStepCountLabel() } func updateStepCountLabel() { stepCountLabel.setText("(stepCount) steps") }
In this snippet, we have an IBOutlet for the step count label and a reset button. The resetButtonTapped action resets the step count to zero and updates the label accordingly. This interaction is simpler, allowing users to quickly reset their steps without navigating through multiple screens.
Another important concept in designing watchOS interfaces is the use of context and notifications. By integrating these elements, you can create a more engaging experience. For instance, you can utilize WKUserNotificationInterfaceController to display notifications directly within your app’s interface. This allows users to respond to notifications without having to switch contexts, enhancing the overall usability.
class NotificationController: WKUserNotificationInterfaceController { override func didReceive(_ notification: UNNotification) { // Handle the notification and update the interface // Display relevant information to the user } }
As you design your user interface, always test it rigorously. Use the watchOS simulator to iterate through different layouts and interactions. However, nothing beats real-world testing on a physical Apple Watch to uncover nuances in performance and user experience. Pay attention to how users interact with your app and be open to making adjustments based on their feedback.
Designing user interfaces for watchOS is an exercise in simplicity and effectiveness. By focusing on the essential features, using intuitive interactions, and continuously testing your designs, you can create compelling watchOS applications that meet users’ needs while providing a delightful experience.
Using HealthKit and Fitness Data
Using HealthKit and fitness data is a cornerstone of many watchOS applications, allowing developers to create powerful health-focused experiences that benefit from the unique capabilities of the Apple Watch. HealthKit provides a central repository for health and fitness data, enabling apps to access and share information such as step count, heart rate, and sleep patterns. Understanding how to utilize this framework effectively can drastically enhance the functionality of your applications.
To begin, it is essential to import the HealthKit framework into your project. This can be done by adding the following line to your Swift file:
import HealthKit
Before you can access any health data, you need to check if HealthKit is available on the device. This can be done using the following function:
func isHealthDataAvailable() -> Bool { return HKHealthStore.isHealthDataAvailable() }
Next, you will need to request permission to access specific types of health data. This involves defining which data types you wish to read and/or write, and then prompting the user for access. Here’s how you can set this up:
let healthStore = HKHealthStore() func requestAuthorization() { let stepsCount = HKQuantityType.quantityType(forIdentifier: .stepCount)! let typesToShare: Set = [stepsCount] let typesToRead: Set = [stepsCount] healthStore.requestAuthorization(toShare: typesToShare, read: typesToRead) { (success, error) in if success { print("Authorization granted") } else { print("Authorization denied: (error?.localizedDescription ?? "Unknown error")") } } }
Once you have the necessary permissions, you can start reading data from HealthKit. For instance, if you want to retrieve the total step count over a specific period, you can create a query like this:
func fetchStepCount(completion: @escaping (Double) -> Void) { let stepsCount = HKQuantityType.quantityType(forIdentifier: .stepCount)! let calendar = Calendar.current let startDate = calendar.startOfDay(for: Date()) let endDate = Date() let predicate = HKQuery.predicateForSamples(withStart: startDate, end: endDate, options: .strictEndDate) let query = HKSampleQuery(sampleType: stepsCount, predicate: predicate, limit: HKObjectQueryNoLimit, sortDescriptors: nil) { (query, results, error) in guard let results = results as? [HKQuantitySample] else { print("Error fetching step count: (error?.localizedDescription ?? "Unknown error")") completion(0) return } let totalSteps = results.reduce(0) { $0 + $1.quantity.doubleValue(for: HKUnit.count()) } completion(totalSteps) } healthStore.execute(query) }
The `fetchStepCount` function constructs a sample query to retrieve step count data from HealthKit. It defines a time range from the start of the current day to the present, checks for any results, and sums the step counts to provide the total. This function can be invoked as needed, such as when the user opens your app or taps a button.
Integrating this data into your watchOS application can enhance user engagement. For instance, ponder updating the user interface to display the current step count or alerting the user when they reach their daily goal. Here’s a simple example of how you might update your interface:
@IBOutlet weak var stepCountLabel: WKInterfaceLabel! func updateStepCountUI() { fetchStepCount { [weak self] totalSteps in DispatchQueue.main.async { self?.stepCountLabel.setText("(totalSteps) steps") } } }
In this snippet, the `updateStepCountUI` function fetches the step count and updates the label on the main thread. This ensures that the user interface remains responsive and accurately reflects the current data.
HealthKit also supports writing data back to the store, allowing your app to log activities such as workouts. This is done in a similar way: define the quantity type, create a sample, and save it to HealthKit. Here’s how you can log a workout session:
func logWorkout(duration: TimeInterval, calories: Double) { let workout = HKWorkout(activityType: .walking, start: Date(), end: Date().addingTimeInterval(duration)) healthStore.save(workout) { (success, error) in if success { print("Workout saved successfully.") } else { print("Error saving workout: (error?.localizedDescription ?? "Unknown error")") } } }
In this example, we create a new workout instance and save it to HealthKit. Note that you’ll want to ensure the user has granted permissions for writing workout data as well.
By using HealthKit, you can provide users with valuable insights into their health and fitness data, creating a more engaging and useful application. With careful handling of permissions, efficient data retrieval, and thoughtful integration into your user interface, your watchOS app can become an indispensable tool for fitness tracking and health management.
Optimizing Performance for watchOS Devices
Optimizing performance for watchOS devices is a critical aspect of developing applications that provide a great user experience. Given the resource constraints of the Apple Watch, it is essential to leverage best practices to ensure that your app runs smoothly while preserving battery life. The strategies to enhance performance can be categorized into several key areas: efficient rendering of user interfaces, minimizing resource usage, and ensuring that data is accessed intelligently.
One of the first considerations in performance optimization is the design of your user interface. The watchOS interface must be responsive, with minimal lag between user input and app output. Using lightweight components and limiting the number of elements displayed on the screen at any given time can significantly improve rendering performance. For instance, using WKInterfaceGroup to manage multiple interface elements can reduce the rendering burden on the watchOS system.
@IBOutlet weak var myGroup: WKInterfaceGroup! func configureGroup() { myGroup.setBackgroundColor(.clear) // Add other configuration for group elements here }
When designing layouts, opt for simpler designs that are visually appealing yet efficient. Avoid using complex gradients and shadows, as these can add unnecessary processing overhead. Instead, focus on using flat colors and minimalistic designs that convey the necessary information without excessive visual weight.
Another crucial area for performance optimization is the handling of data. When interacting with data sources, be mindful of how often you fetch and update data. For example, if your app displays health data, you should implement a mechanism to periodically fetch updates rather than doing so continuously. This can be achieved using timers or by listening for notifications from HealthKit. For instance, you might set up a timer that fetches the latest step count every few minutes:
var dataUpdateTimer: Timer? func startDataUpdateTimer() { dataUpdateTimer = Timer.scheduledTimer(withTimeInterval: 300, repeats: true) { [weak self] _ in self?.fetchStepCount() } }
Using asynchronous operations is also pivotal in maintaining a responsive user interface. When accessing data that may take time to retrieve, such as HealthKit data, you should perform these operations in the background and only update the UI on the main thread once the data is ready. This ensures that your app’s interface remains fluid, without lagging due to data fetching processes.
func fetchStepCount() { // Perform data fetching on a background thread DispatchQueue.global().async { // Your data fetching logic here let totalSteps = ... // Fetch steps DispatchQueue.main.async { self.updateStepCountUI(totalSteps: totalSteps) } } }
Memory management plays a significant role in performance optimization as well. Always be aware of the objects you create and ensure they’re released when no longer needed. That is particularly important with watchOS, where memory resources are limited. Use instruments in Xcode to monitor your app’s memory usage and identify any potential leaks or unnecessary allocations.
Moreover, ponder employing caching strategies for data that doesn’t change frequently. For example, if your app retrieves data from an API or database, store this data in memory or use a local database to reduce the need for repeated network calls. This not only speeds up operations but also conserves battery life by minimizing network activity.
let cache = NSCache() func fetchData(key: String) -> NSNumber? { if let cachedValue = cache.object(forKey: key as NSString) { return cachedValue } // Fetch from data source and cache the value let fetchedValue = ... cache.setObject(fetchedValue, forKey: key as NSString) return fetchedValue }
Lastly, always test your app extensively on actual devices. The watchOS simulator may not accurately reflect performance characteristics, especially regarding battery consumption and resource management. Use profiling tools to assess the performance of your app in real-time and make necessary adjustments based on your findings.
By implementing these optimization strategies, you can create watchOS applications that are not only functional but also efficient and responsive. This attention to performance details will enhance the overall user experience, making your app a joy to use on one of the most intimate devices in the Apple ecosystem.