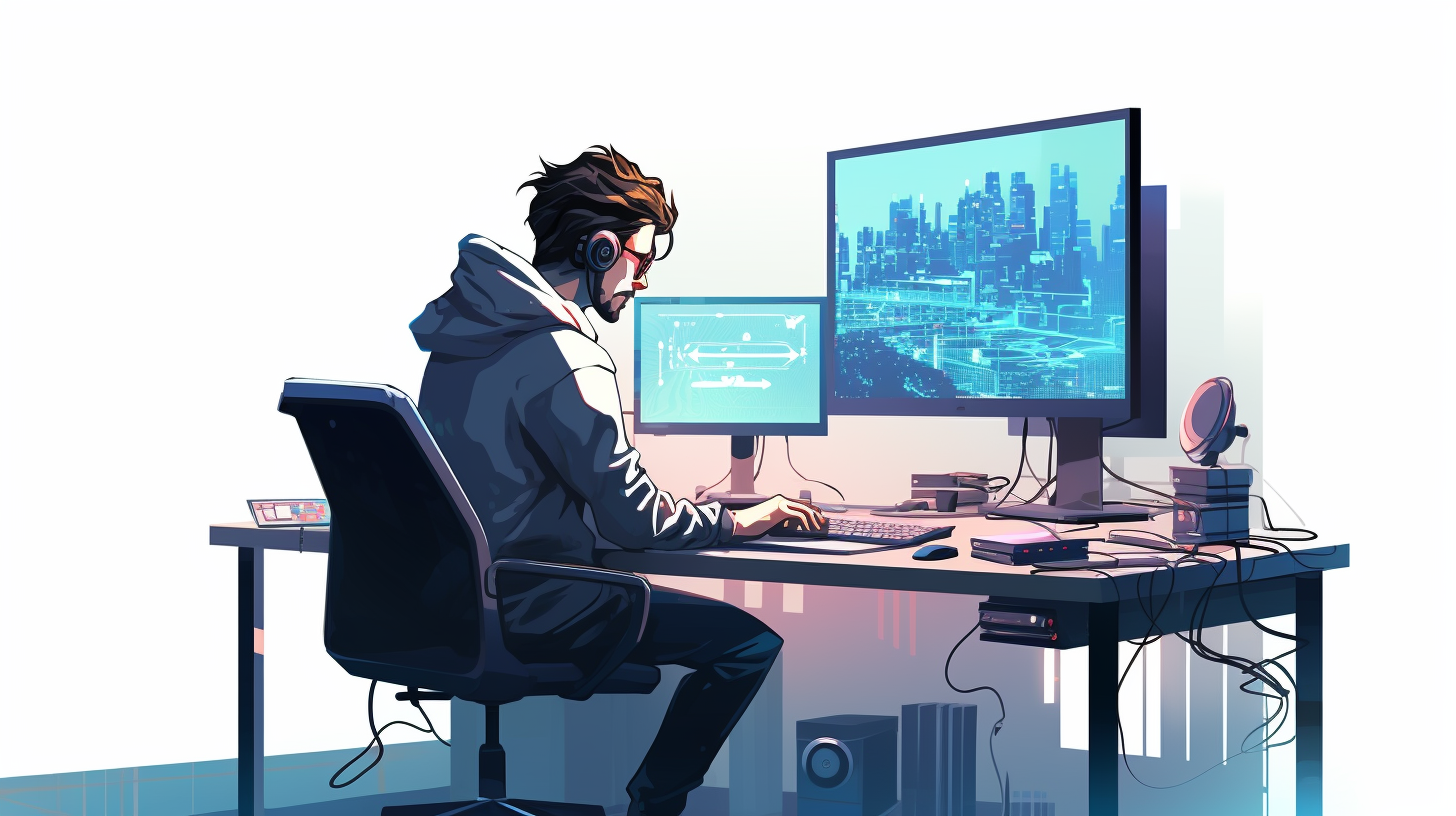
Swift Playgrounds for Learning
Swift Playgrounds is an innovative app developed by Apple that serves as an interactive learning environment for aspiring programmers, particularly those looking to delve into the Swift programming language. It effectively transforms the often daunting task of learning to code into an engaging and enjoyable experience. With its intuitive interface, Swift Playgrounds caters to beginners and experienced developers alike, bridging the gap between theory and practice.
One of the standout aspects of Swift Playgrounds is its focus on real-time feedback. As users write code, they can immediately see the results of their work, which fosters a deeper understanding of programming concepts. This immediate feedback loop is a core principle of effective learning, allowing users to experiment and iterate on their ideas without the fear of making mistakes.
Moreover, Swift Playgrounds is designed to be accessible on both iPad and Mac, providing a versatile platform for users to learn wherever they are. The app comes equipped with a high number of pre-built templates and challenges, enabling learners to tackle a wide range of programming tasks. By integrating rich visuals and interactive elements, it makes the learning process akin to playing a game, where users can solve puzzles and unlock new challenges as they progress.
Swift Playgrounds also supports a range of features that enhance the learning experience. For instance, users can import their own resources, such as images and sounds, into their playgrounds, encouraging creativity and personal expression. Additionally, the app offers a plethora of built-in lessons that cover foundational concepts, such as loops, conditionals, and functions.
Think this simple example that demonstrates how to create a basic loop in Swift:
for i in 1...5 { print("Iteration number: (i)") }
This code snippet showcases a for loop that iterates from 1 to 5, printing the current iteration number. Such examples help solidify the understanding of loops and their applications within programming.
Furthermore, the community aspect of Swift Playgrounds cannot be overstated. Learners can share their playgrounds with others, gaining insights and inspiration from peers. This collaborative environment encourages knowledge sharing and fosters a sense of belonging, which is important for sustained engagement in learning.
Swift Playgrounds represents a significant step forward within the scope of coding education. Its interactive and effortless to handle approach demystifies the learning process, making it an ideal choice for anyone looking to embark on their coding journey.
Key Features and Benefits
Additionally, Swift Playgrounds incorporates gamification elements that further enrich the learning experience. By framing coding challenges as games, users are not only motivated to complete tasks but also to improve their skills in a playful and less intimidating manner. For example, learners may encounter missions that require them to navigate a character through a maze, using coding commands to overcome obstacles. This approach not only makes learning fun but also reinforces problem-solving skills in a practical context.
Another remarkable feature of Swift Playgrounds is its ability to provide a rich set of resources and documentation right within the app. Users can access detailed descriptions, tutorials, and examples without having to leave the learning environment. This seamless integration ensures that learners can quickly reference information as they code, reducing frustration and enabling them to stay focused on their projects.
Let’s take a look at a simple example that demonstrates how to define and use a function in Swift:
func greetUser(name: String) { print("Hello, (name)!") } greetUser(name: "Alice")
In this snippet, we define a function called greetUser
that takes a String
parameter. When invoked with the argument "Alice"
, it outputs a personalized greeting. Such practical examples allow users to see how functions can encapsulate logic and be reused throughout their code, an essential concept in programming.
Moreover, Swift Playgrounds is equipped with a variety of tools that make debugging and understanding code behavior simpler. The app features a built-in console for output, enabling users to track their program’s execution and identify issues in real time. Additionally, the visual representations of code execution flow can dramatically enhance comprehension, particularly for complex algorithms.
Swift Playgrounds also embraces the principles of coding best practices, guiding users toward writing cleaner and more efficient code. The app encourages the use of comments, effective variable naming conventions, and modular programming techniques. By instilling these habits early in the learning process, Swift Playgrounds lays a solid foundation for future programming endeavors.
The key features and benefits of Swift Playgrounds position it as a powerful tool for learning to code. Its engaging design, immediate feedback, rich resources, and supportive community create a comprehensive educational experience this is both effective and enjoyable. Whether you are a novice or looking to sharpen your skills, Swift Playgrounds offers something valuable for every aspiring developer.
Getting Started with Swift Playgrounds
To get started with Swift Playgrounds, first, you need to download the app from the App Store if you’re on an iPad, or from the Mac App Store for Mac users. Once installed, launching the app presents you with a welcoming interface that invites you to explore various learning paths. The main screen displays a range of playgrounds, each tailored to different concepts and skills in Swift programming.
Upon selecting a playground, the user is greeted with a split-screen view. The left pane contains the code editor where you write your Swift code, while the right pane displays the results in real-time, whether it be text output, graphics, or animations. This immediate visual feedback is essential for understanding how your code translates into action.
Swift Playgrounds offers numerous built-in tutorials that cover a wide array of topics, from the basics of syntax to more advanced concepts such as object-oriented programming. Beginners can start with simple tasks that gradually build in complexity, allowing for a gentle introduction to programming concepts. For instance, the idea of variables can be illustrated with the following code snippet:
var greeting = "Hello, Playgrounds!" print(greeting)
This example shows how to declare a variable called `greeting` and print its value. Understanding variables is fundamental as they hold values that can change, making them a cornerstone of programming logic.
As you progress, Swift Playgrounds encourages users to experiment with code. You might be prompted to modify existing code to achieve a specific outcome, which is a fantastic way to reinforce learning. For example, if you are tasked with changing the greeting to include your name, you might update the variable as follows:
var name = "Alice" var greeting = "Hello, (name)!" print(greeting)
In this snippet, the variable `name` is introduced, showcasing string interpolation—a powerful feature that allows for dynamic string creation. This kind of hands-on learning reflects real-world programming tasks, providing context and relevance to the concepts being taught.
Another key element of getting started with Swift Playgrounds is the availability of challenges that test your skills. These challenges range from solving puzzles to creating small games, reinforcing the programming principles you have learned. Each challenge is crafted to encourage critical thinking and problem-solving skills, essential traits for any developer.
Moreover, as you explore the app, you’ll discover various resources and tools designed to facilitate your coding journey. The integrated documentation provides quick references and examples, rendering it effortless to look up unfamiliar functions or concepts without interrupting your flow. For instance, if you want to learn about arrays, you can pull up the relevant documentation and see practical examples, such as:
let fruits = ["Apple", "Banana", "Cherry"] print(fruits[0]) // Outputs "Apple"
This snippet introduces arrays, a fundamental data structure used to store collections of values. By seeing how to access elements within an array, users can grasp how to manage and manipulate data effectively.
Swift Playgrounds also incorporates the use of visual coding elements to represent concepts graphically, providing a richer understanding of the material. You can visualize algorithms and data flows, making abstract concepts much more tangible. This aspect of Swift Playgrounds is particularly beneficial for visual learners, who may struggle with purely textual explanations.
As you embark on your journey with Swift Playgrounds, remember that the key to success is consistent practice and exploration. Don’t hesitate to experiment with your code, make mistakes, and learn from them. Each session in Swift Playgrounds is a step closer to mastering the Swift programming language and developing your coding skills.
Interactive Learning Activities
Interactive learning activities form the heart of Swift Playgrounds, transforming coding education into a dynamic, engaging experience. By integrating coding challenges into game-like scenarios, Swift Playgrounds fosters a playful environment where users can explore programming concepts through hands-on experimentation. This approach not only makes learning enjoyable but also promotes critical thinking and problem-solving skills essential for any developer.
One of the most effective ways Swift Playgrounds facilitates interactive learning is through its array of puzzles and challenges. These tasks encourage learners to apply their coding knowledge in practical situations. For example, users may be required to guide a character through a series of obstacles by writing code to control its movements. This gamified approach allows learners to see the immediate consequences of their code, reinforcing their understanding of programming logic.
func moveCharacter(steps: Int) { for _ in 1...steps { // Code to move the character forward print("Character moved forward!") } } moveCharacter(steps: 3)
In the above snippet, the `moveCharacter` function illustrates a simple way to control character movement. By passing the number of steps as an argument, users can easily adapt the code to change the character’s journey. This type of interactive activity not only solidifies understanding of function definitions and loops but also encourages users to think creatively about how they can manipulate their code.
Another engaging format offered in Swift Playgrounds is the use of real-world scenarios that require learners to devise solutions through coding. For instance, users might be tasked with developing a simple game where they need to score points by catching falling objects. This not only challenges them to implement logic and conditions but also enables them to see how their programming skills can produce tangible results.
var score = 0 func catchObject(isCaught: Bool) { if isCaught { score += 1 print("Object caught! Score: (score)") } else { print("Missed the object!") } } catchObject(isCaught: true)
In this example, the function `catchObject` uses a Boolean parameter to determine if an object was successfully caught, updating the score accordingly. The clear feedback provided by print statements simulates the type of responses users can expect in a real game scenario, illustrating the effectiveness of conditional statements in action.
Furthermore, Swift Playgrounds encourages collaborative learning through its sharing capabilities. Users have the opportunity to share their completed playgrounds with others, fostering a community of learners who can offer insights and inspire each other. This element of collaboration is crucial—working together not only enhances understanding but also cultivates a supportive network that can motivate users to keep pushing their limits.
The interactive learning activities in Swift Playgrounds are meticulously designed to cater to various learning styles. Whether it’s through visual representations, auditory feedback, or kinesthetic coding practices, the app provides multiple ways for learners to grasp complex concepts. This multiplicity ensures that regardless of how a student learns best, there’s an interactive component in Swift Playgrounds that will meet their needs.
Moreover, as learners progress, they encounter increasingly complex challenges that build upon their foundational knowledge, keeping them engaged and motivated. The app cleverly scaffolds learning, introducing new concepts gradually while revisiting previously learned material. This strategy helps solidify understanding and enables users to connect the dots between various programming principles.
The interactive learning activities within Swift Playgrounds not only make coding education enjoyable but also empower users to develop their skills in a structured yet flexible manner. By encouraging exploration, collaboration, and creativity, Swift Playgrounds stands out as a transformative tool for anyone eager to learn the world of programming.
Tips for Maximizing Your Learning Experience
To maximize your learning experience with Swift Playgrounds, it is essential to cultivate a mindset geared toward exploration and experimentation. The app is designed to be a sandbox environment where you can freely test ideas, make mistakes, and learn from them. This hands-on approach is fundamental to grasping programming concepts effectively. Instead of merely following tutorials, challenge yourself to modify code snippets and predict their outcomes. For instance, try changing variable values or altering function parameters to see how it impacts the results.
In addition to experimentation, setting specific learning goals can help maintain focus and motivation. Ponder targeting particular concepts or skills you wish to master within a set timeframe. For example, you might decide to spend a week strengthening your understanding of loops. To do this, engage with various challenges related to loops each day, and document your learning progress. This structured approach transforms your coding practice into a more goal-oriented endeavor, reinforcing the discipline necessary for skill development.
Using the community features of Swift Playgrounds can significantly enhance your learning journey. By sharing your projects and playgrounds, you open yourself up to feedback and alternative solutions from fellow learners. Participating in online forums or local coding groups centered around Swift Playgrounds can also provide a wealth of knowledge. Engaging with others allows you to absorb different perspectives and techniques that you might not have considered. You can learn from their successes and challenges, enriching your own understanding and capabilities.
Moreover, take full advantage of the built-in resources within the app. Swift Playgrounds includes comprehensive documentation and tutorials that can be accessed directly while you code. Whenever you come across a new concept, don’t hesitate to look it up immediately. For example, if you’re unsure how to implement a specific data structure, pulling up the documentation can provide you not only with definitions but also practical code examples:
let numbers = [1, 2, 3, 4, 5] let doubled = numbers.map { $0 * 2 } print(doubled) // Outputs "[2, 4, 6, 8, 10]"
This snippet demonstrates how to use the `map` function to double the values in an array. Learning to leverage such built-in functionalities can save time and help you write more efficient code.
Additionally, practice is key. Dedicate consistent time each week to coding in Swift Playgrounds. Just as with any skill, regular practice will solidify your knowledge and enhance muscle memory. Think setting aside time for both guided lessons and free coding sessions where you can explore concepts that pique your interest. During these creative sessions, focus on personal projects that excite you, whether it’s a small game, an interactive story, or a simple utility app. Passion-driven projects often yield the most satisfying learning experiences.
Finally, embrace patience. Learning to code is a journey filled with ups and downs. You may encounter challenges that seem insurmountable at times, but persistence is vital. Remember that every programmer has experienced moments of confusion and frustration. Keeping a positive attitude and viewing challenges as opportunities to learn will serve you well as you navigate the intricacies of Swift programming.
By embracing experimentation, setting goals, using community resources, practicing consistently, and maintaining patience, you can maximize your learning experience with Swift Playgrounds. This innovative platform offers you not just the tools to learn coding but also the environment to thrive as a developer.