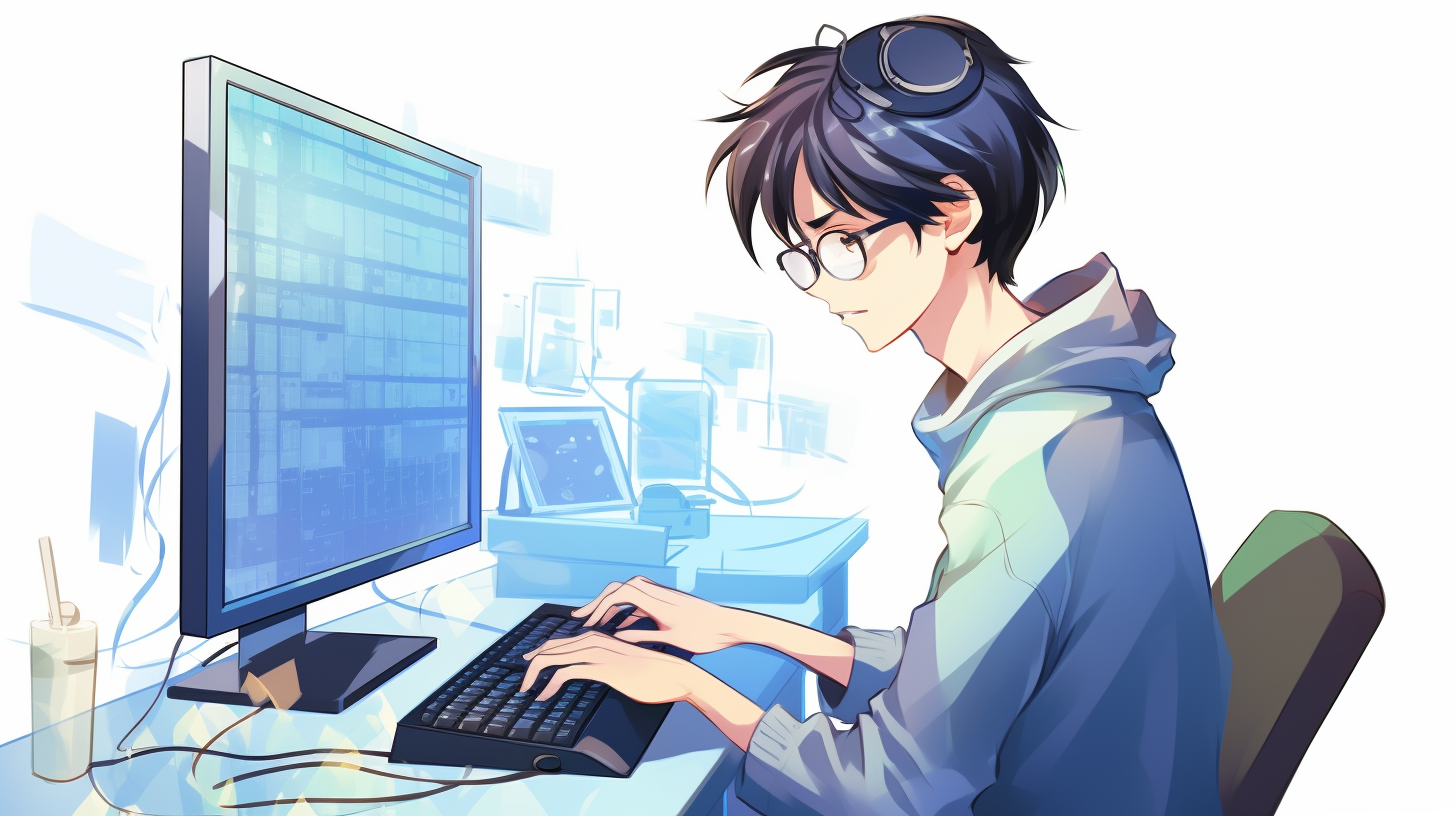
Swift Syntax Fundamentals
In Swift, understanding variables and constants is fundamental to managing data effectively. Variables are used to store values that can change over time, while constants are used for values that remain fixed throughout the lifetime of the program. This differentiation is important in ensuring that your code behaves as expected.
To declare a variable in Swift, you use the var
keyword, followed by the variable name and its initial value. For example:
var age = 30
In this snippet, age
is a variable initialized with the value of 30. You can change the value of age
later in the code:
age = 31
On the other hand, if you want to create a constant, you use the let
keyword. Constants are immutable, meaning that once you set their value, it cannot be changed. Here’s how you declare a constant:
let pi = 3.14159
Attempting to change the value of pi
later in the code would result in a compile-time error, reinforcing the integrity of your constants.
Swift also employs type inference, which means that the compiler can automatically deduce the type of a variable or constant based on its initial value. However, you can explicitly specify a type if needed:
var score: Int = 100
In this example, score
is declared as an integer. If you try to assign a non-integer value to it, the compiler will raise an error, helping to catch bugs early in the development process.
Additionally, Swift supports compound types such as arrays and dictionaries, which are also declared with var
or let
. For instance, creating an array of integers can be accomplished as follows:
var primeNumbers: [Int] = [2, 3, 5, 7, 11]
In this scenario, primeNumbers
is mutable, allowing additions and modifications, whereas a constant array would restrict such changes.
Moreover, Swift’s optionals provide a powerful way to handle the absence of a value. By declaring a variable or constant as optional using a question mark (?
), you indicate that it might hold a value or be nil
:
var middleName: String? = nil
In this example, middleName
can either be a String
or nil
, providing flexibility in data handling.
Mastering the use of variables and constants in Swift is an essential skill. Properly using these constructs not only enhances the clarity of your code but also improves performance and reduces the likelihood of bugs.
Control Flow Statements
Control flow statements in Swift are essential for directing the execution of code based on specific conditions. They enable developers to implement logic that can change the path of execution, making their applications dynamic and responsive to different situations.
Swift offers several control flow constructs, including if statements, switch statements, and looping constructs such as for, while, and repeat-while loops. Understanding these constructs allows developers to create more complex and functional applications.
The basic structure of an if statement allows you to execute code conditionally. Here’s a simple example:
let temperature = 30 if temperature > 25 { print("It's a hot day!") } else { print("The weather is pleasant.") }
In this code snippet, the output will be “It’s a hot day!” if the temperature exceeds 25 degrees. The else clause provides an alternative action if the condition is not met.
Swift’s switch statement is particularly powerful, allowing for a more expressive way to handle multiple conditions without the verbosity of multiple if statements. Here’s an example:
let day = "Saturday" switch day { case "Monday": print("Start of the work week.") case "Saturday", "Sunday": print("It's the weekend!") default: print("It's a weekday.") }
In this example, the switch statement evaluates the value of day and matches it against different cases. The ability to group cases together, like Saturday and Sunday, enhances code readability and organization.
For repeating tasks, Swift provides several looping constructs. The for-in loop is commonly used for iterating over arrays or ranges. Think the following example:
let numbers = [1, 2, 3, 4, 5] for number in numbers { print("Number: (number)") }
This loop will print each number in the array, demonstrating the concise syntax of Swift for iterating collections.
The while loop continues executing as long as a specified condition remains true. Here’s an example:
var count = 5 while count > 0 { print("Countdown: (count)") count -= 1 }
This will print a countdown from 5 to 1. The loop terminates when the condition count > 0 is no longer satisfied.
Another looping construct, the repeat-while loop, guarantees that the code block will execute at least once before checking the condition:
var number = 0 repeat { print("Number: (number)") number += 1 } while number < 3
In this scenario, the loop will print the numbers 0, 1, and 2, showcasing its behavior of executing the loop body before evaluating the condition.
Combining these control flow statements allows for building robust and versatile applications. Whether making decisions based on user input or executing repetitive tasks, mastering control flow in Swift is vital for any developer aiming to write efficient and effective code.
Functions and Closures
Functions and closures are central to Swift programming, providing a powerful mechanism for organizing code and enhancing its reusability. A function in Swift is defined using the `func` keyword, followed by the function name, parameters, and the return type. Functions can take zero or more parameters, and they may return a value or be void.
Here’s a basic example of a function that takes two integers as parameters and returns their sum:
func add(a: Int, b: Int) -> Int { return a + b }
In this example, the `add` function accepts two integers, `a` and `b`, and returns their sum. To call this function, you’d write:
let result = add(a: 5, b: 3) // result is 8
Functions can also have default parameter values, which is a convenient way to provide a default behavior without requiring the caller to specify every parameter. Here’s an example:
func greet(name: String, greeting: String = "Hello") { print("(greeting), (name)!") } greet(name: "Alice") // prints "Hello, Alice!" greet(name: "Bob", greeting: "Hi") // prints "Hi, Bob!"
Closures, on the other hand, are self-contained blocks of functionality that can be passed around and used in your code. They can capture and store references to variables and constants from the context in which they’re defined. A closure can take parameters and return values just like a function. Here’s how you define a simple closure that squares a number:
let square: (Int) -> Int = { number in return number * number } let result = square(4) // result is 16
Closures are particularly useful in scenarios where you need to pass functionality as a parameter to functions, such as sorting or filtering collections. For example, using a closure to sort an array of integers in descending order can be done as follows:
let numbers = [5, 2, 9, 1, 5, 6] let sortedNumbers = numbers.sorted { $0 > $1 } // sortedNumbers is [9, 6, 5, 5, 2, 1]
Swift’s closure syntax is compact and expressive. The shorthand argument names `$0`, `$1`, etc., are particularly convenient, allowing for concise definitions without needing to specify parameters explicitly when the context is clear.
Additionally, closures can capture and store references to variables and constants from their surrounding context, a feature known as “capturing values.” This behavior can lead to useful patterns, such as retaining state or creating custom behavior in asynchronous programming. Here’s an example demonstrating capturing:
func makeIncrementer(incrementAmount: Int) -> () -> Int { var total = 0 let incrementer: () -> Int = { total += incrementAmount return total } return incrementer } let incrementByTwo = makeIncrementer(incrementAmount: 2) print(incrementByTwo()) // prints 2 print(incrementByTwo()) // prints 4
In this example, the `makeIncrementer` function returns a closure that increments a total variable by the specified amount. Each call to `incrementByTwo` retains the state of `total`, demonstrating the power of closures in capturing and maintaining state across multiple calls.
Mastering functions and closures is essential for writing clean, modular Swift code. They not only enhance code organization and readability but also enable powerful programming paradigms, such as functional programming approaches. Taking advantage of these features will significantly improve your ability to create efficient and maintainable applications.
Object-Oriented Programming Concepts
Object-oriented programming (OOP) is a paradigm that allows developers to model real-world entities using classes and objects, promoting code reuse and modularity. In Swift, everything revolves around the concepts of classes, structures, and protocols, providing a robust framework for building applications.
A class in Swift is a blueprint for creating objects, encapsulating data and behavior. You define a class using the class
keyword, followed by the class name and a set of properties and methods. Here’s a simple example of a class that represents a car:
class Car { var make: String var model: String var year: Int init(make: String, model: String, year: Int) { self.make = make self.model = model self.year = year } func displayInfo() { print("Car: (year) (make) (model)") } }
In this example, the Car
class has three properties: make
, model
, and year
. The initializer init
is used to set these properties when creating a new instance of the class. The displayInfo
method provides functionality to print the car’s details.
To create an object from the Car
class, you simply instantiate it:
let myCar = Car(make: "Toyota", model: "Corolla", year: 2020) myCar.displayInfo() // Output: Car: 2020 Toyota Corolla
In addition to classes, Swift also provides structures, which are similar to classes but have some important differences. Structures are value types, meaning that when you assign or pass them, a copy is made. This can lead to different behavior compared to classes, which are reference types. Here’s an example of a structure representing a point:
struct Point { var x: Int var y: Int }
Using the Point
structure, you can create instances the same way as with classes:
var pointA = Point(x: 10, y: 20) var pointB = pointA // pointB is a copy of pointA pointB.x = 30 print(pointA.x) // Output: 10 print(pointB.x) // Output: 30
Here, modifying pointB
does not affect pointA
, illustrating the value type behavior of structures.
Protocols in Swift define a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality. Protocols are a fundamental part of Swift’s design, allowing for flexible and decoupled code structures. You can define a protocol using the protocol
keyword:
protocol Drivable { var speed: Int { get } func accelerate() }
Any class or struct that conforms to the Drivable
protocol must provide an implementation for the speed
property and the accelerate
method. Here’s how you might implement this in a class:
class Bike: Drivable { var speed: Int = 0 func accelerate() { speed += 10 print("Accelerating to (speed) km/h") } }
Now you can create a Bike
object and use its methods defined by the Drivable
protocol:
let myBike = Bike() myBike.accelerate() // Output: Accelerating to 10 km/h
Swift’s combination of classes, structures, and protocols empowers developers to build complex systems in a clear and concise manner. By using these object-oriented principles, you can create reusable components, enhance code maintainability, and facilitate collaboration within teams. Understanding and mastering these fundamental concepts of OOP in Swift very important for developing robust applications.