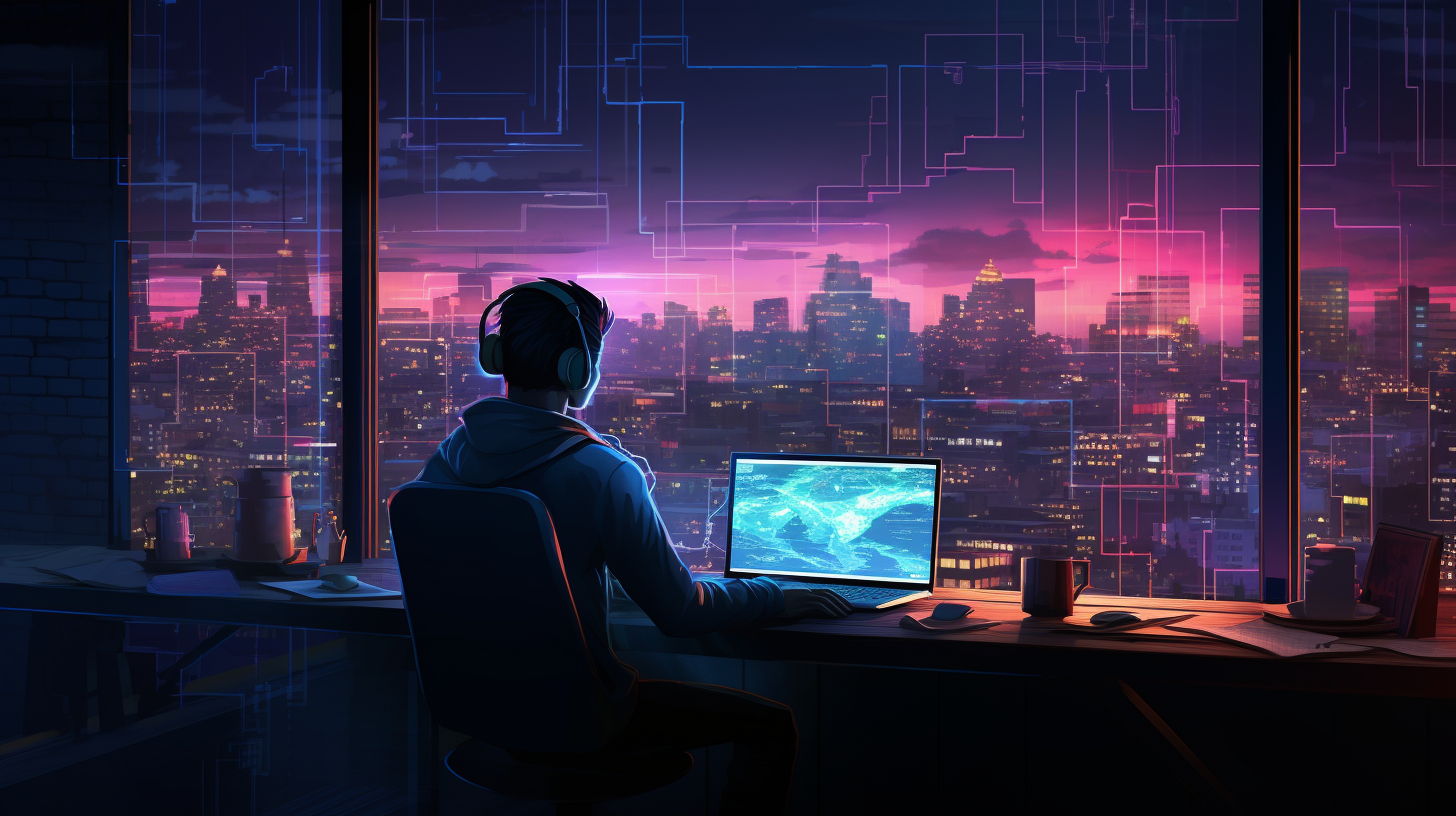
Understanding Java Interfaces
Java interfaces represent a fundamental building block of the Java programming language, allowing developers to define contracts that classes can adhere to. An interface is essentially a collection of abstract methods that a class can implement, enabling a form of polymorphism that enhances code flexibility and reusability.
At its core, an interface defines a set of methods without providing any implementation. This means that any class that implements the interface must provide concrete implementations for all of its methods. This characteristic allows for a clear separation between the definition of behaviors (what methods should be available) and their implementations (how those methods execute).
Unlike classes, interfaces can extend multiple other interfaces, allowing for a form of multiple inheritance. This is particularly useful for defining a common set of behaviors that can be shared across different class hierarchies. When a class implements an interface, it’s promising to fulfill the contract defined by that interface, leading to better code organization and enhanced maintainability.
Another significant aspect of Java interfaces is their ability to support polymorphism. When an object is referenced by an interface type, any class that implements that interface can be used interchangeably. This means that the same interface reference can point to objects of different classes, invoking the methods defined in the interface without needing to know the specific types of those objects.
Java also allows interfaces to contain constants. These constants are implicitly public, static, and final. This means that any constant defined within an interface can be accessed directly via the interface name, promoting a clean and organized way to manage constant values associated with a set of behaviors.
To show how interfaces work, ponder the following example:
// Define an interface public interface Drawable { void draw(); // abstract method } // Implement the interface in a class public class Circle implements Drawable { @Override public void draw() { System.out.println("Drawing a Circle"); } } // Another implementation of the same interface public class Rectangle implements Drawable { @Override public void draw() { System.out.println("Drawing a Rectangle"); } } // Using the interface to invoke methods public class Main { public static void main(String[] args) { Drawable circle = new Circle(); Drawable rectangle = new Rectangle(); circle.draw(); // Outputs: Drawing a Circle rectangle.draw(); // Outputs: Drawing a Rectangle } }
In this example, we define an interface called Drawable with an abstract method draw(). The classes Circle and Rectangle implement this interface, each providing its own version of the draw() method. When we create instances of these classes and reference them using the Drawable interface, we can call the draw() method polymorphically, demonstrating the power and flexibility of interfaces.
Overall, interfaces in Java provide a robust mechanism for defining contracts and promoting reusable code while supporting polymorphism and multiple inheritance of types. Their utility in defining clear APIs and facilitating cleaner code architecture cannot be overstated.
Declaring and Implementing Interfaces
Declaring an interface in Java is simpler. An interface is created using the interface
keyword, followed by its name, and it can contain method signatures, constants, and nested types. The method signatures in an interface are implicitly public and abstract, meaning they do not contain a body and must be implemented by any class that claims to implement the interface.
Let’s delve into a more detailed example of how to declare and implement an interface:
// Define an interface public interface Animal { void makeSound(); // abstract method void eat(); // another abstract method }
In this snippet, we define an interface named Animal
with two abstract methods: makeSound
and eat
. Now, any class that implements this interface must provide implementations for these methods.
Next, let’s see how we can implement this interface in different classes:
// Implementing the Animal interface in the Dog class public class Dog implements Animal { @Override public void makeSound() { System.out.println("Bark"); } @Override public void eat() { System.out.println("Dog is eating"); } } // Implementing the Animal interface in the Cat class public class Cat implements Animal { @Override public void makeSound() { System.out.println("Meow"); } @Override public void eat() { System.out.println("Cat is eating"); } }
Here, we have two classes, Dog
and Cat
, both implementing the Animal
interface. Each class provides its own version of the makeSound
and eat
methods. This illustrates how interfaces allow for different implementations of the same behavior.
Now, let’s see how we can use these implementations polymorphically:
// Using the Animal interface to demonstrate polymorphism public class Main { public static void main(String[] args) { Animal myDog = new Dog(); Animal myCat = new Cat(); myDog.makeSound(); // Outputs: Bark myDog.eat(); // Outputs: Dog is eating myCat.makeSound(); // Outputs: Meow myCat.eat(); // Outputs: Cat is eating } }
In the Main
class, we create instances of Dog
and Cat
using the Animal
interface type. This allows us to call the makeSound
and eat
methods on both objects without needing to know their specific types. The output clearly shows the unique behaviors of each animal, demonstrating the polymorphic nature of interfaces in Java.
Moreover, interfaces can also include constants, which can be useful for defining fixed values related to the behavior defined in the interface. For instance:
public interface Vehicle { int MAX_SPEED = 120; // constant void accelerate(); }
In the interface Vehicle
, we declare a constant MAX_SPEED
. This constant is implicitly public, static, and final, and can be accessed as Vehicle.MAX_SPEED
from any implementation.
In implementing classes, we can leverage these constants, further enriching the capabilities of the interface. In summary, declaring and implementing interfaces in Java not only facilitates a clean, organized structure for code but also significantly enhances the flexibility and maintainability of applications.
Default and Static Methods in Interfaces
With the introduction of Java 8, interfaces gained the ability to include default and static methods, significantly enhancing their functionality and versatility. This evolution reflects a broader trend toward allowing interfaces to contain behavior, thereby reducing the need for boilerplate code in implementing classes while promoting code reuse.
Default Methods
A default method is a method defined in an interface that provides a default implementation. This allows developers to add new methods to existing interfaces without breaking the implementing classes. Default methods are prefixed with the default
keyword.
This capability is particularly useful in scenarios where you have a library of interfaces, and you want to improve them over time without forcing all implementing classes to adopt the changes immediately. Let’s take a look at an example:
// Define an interface with a default method public interface Vehicle { void accelerate(); default void honk() { System.out.println("Honking the horn!"); } } // Implementing the Vehicle interface in a Car class public class Car implements Vehicle { @Override public void accelerate() { System.out.println("Car is accelerating"); } } // Using the default method in the main application public class Main { public static void main(String[] args) { Vehicle myCar = new Car(); myCar.accelerate(); // Outputs: Car is accelerating myCar.honk(); // Outputs: Honking the horn! } }
In this example, the Vehicle
interface defines a default method honk
. The Car
class implements the Vehicle
interface but does not override honk
, allowing it to use the default implementation. This feature provides flexibility and allows for smoother evolution of interfaces.
Static Methods
Static methods can also be added to interfaces. Like static methods in classes, these methods belong to the interface itself, not to any instance of the implementing classes. Static methods are defined using the static
keyword and can be useful for utility functions that are relevant to the interface but do not depend on instance-specific data. Here’s how you can define and use a static method in an interface:
// Define an interface with a static method public interface Calculator { static int add(int a, int b) { return a + b; } } // Using the static method in the main application public class Main { public static void main(String[] args) { int sum = Calculator.add(5, 10); // Call the static method from the interface System.out.println("The sum is: " + sum); // Outputs: The sum is: 15 } }
In this case, the Calculator
interface has a static method add
that allows you to perform addition without the need to instantiate any class. That is an elegant way to provide utility methods that can be accessed directly through the interface, promoting a clean and organized coding structure.
The addition of default and static methods in interfaces has revolutionized how we interact with them in Java, allowing for greater flexibility and reducing the need to create abstract base classes solely for method implementations. This balances the need for abstraction with the practicality of easily extensible design, making for a more robust and maintainable codebase.
Functional Interfaces and Lambda Expressions
Functional interfaces are a special category of interfaces in Java that have exactly one abstract method. This characteristic makes them ideal for use with lambda expressions, a feature introduced in Java 8 that allows for a more concise and expressive coding style. The concept of functional interfaces aligns closely with the principles of functional programming, enabling developers to treat behavior as a first-class citizen. By using lambda expressions, you can provide a clear and succinct way to implement these interfaces without the boilerplate code associated with traditional anonymous classes.
To define a functional interface, you annotate it with the @FunctionalInterface annotation, although that’s not mandatory. However, using this annotation helps convey the intent of the interface and ensures that it adheres to the functional interface contract. If you inadvertently add a second abstract method, the compiler will generate an error, enforcing the constraint of having a single abstract method.
Here’s an example of a functional interface:
// Define a functional interface @FunctionalInterface public interface Operation { int apply(int a, int b); // single abstract method }
In this snippet, we define a functional interface called Operation with a single method apply that takes two integers and returns an integer. This interface can be implemented using a lambda expression, providing a clean and readable way to express behavior.
Let’s look at how we can use this functional interface with a lambda expression:
// Using the functional interface with a lambda expression public class Main { public static void main(String[] args) { // Implementing Operation using a lambda expression Operation addition = (a, b) -> a + b; Operation subtraction = (a, b) -> a - b; System.out.println("Addition: " + addition.apply(5, 3)); // Outputs: Addition: 8 System.out.println("Subtraction: " + subtraction.apply(5, 3)); // Outputs: Subtraction: 2 } }
In this example, we create two instances of Operation: one for addition and another for subtraction. Instead of creating a separate class or an anonymous class for each implementation, we use lambda expressions to define the behavior succinctly. This not only reduces the amount of code but also improves readability, making it easier to understand the logic at a glance.
Functional interfaces are widely used in the Java Collections Framework, particularly with methods that accept behavior as an argument, such as forEach, map, and filter. For instance:
import java.util.Arrays; import java.util.List; public class Main { public static void main(String[] args) { List names = Arrays.asList("Alice", "Bob", "Charlie"); // Using a lambda expression with forEach names.forEach(name -> System.out.println("Hello, " + name + "!")); } }
Here, we utilize the forEach method of the List interface, passing in a lambda expression to define the action to be performed on each element in the list. This further highlights the power of functional interfaces in Java, as they facilitate a more declarative style of programming.
Functional interfaces play a pivotal role in Java by enabling the use of lambda expressions, thus enhancing the expressiveness and conciseness of the code. By adopting functional programming principles, Java developers can create more flexible and maintainable applications, using the benefits of behavior as a first-class entity.
Common Use Cases for Interfaces
When exploring the common use cases for interfaces in Java, it’s important to recognize their versatility in various design patterns and architectural styles. One of the most significant roles of interfaces is to define a clear contract for classes, allowing disparate implementations to be utilized interchangeably. This capability is particularly beneficial in scenarios requiring abstraction, such as in plugin architectures, where new functionalities can be added with minimal impact on existing code.
One prominent use case for interfaces is in the implementation of the Strategy Pattern. This design pattern allows an algorithm’s behavior to be selected at runtime. By defining a family of algorithms in an interface, you can create concrete implementations that vary the behavior without modifying the context in which they’re used. Here’s an example:
// Define the Strategy interface public interface Strategy { int execute(int a, int b); } // Concrete implementation of addition public class AddOperation implements Strategy { @Override public int execute(int a, int b) { return a + b; } } // Concrete implementation of subtraction public class SubtractOperation implements Strategy { @Override public int execute(int a, int b) { return a - b; } } // Context that uses the Strategy public class Context { private Strategy strategy; public Context(Strategy strategy) { this.strategy = strategy; } public int executeStrategy(int a, int b) { return strategy.execute(a, b); } } // Using the Strategy Pattern public class Main { public static void main(String[] args) { Context context = new Context(new AddOperation()); System.out.println("10 + 5 = " + context.executeStrategy(10, 5)); // Outputs: 10 + 5 = 15 context = new Context(new SubtractOperation()); System.out.println("10 - 5 = " + context.executeStrategy(10, 5)); // Outputs: 10 - 5 = 5 } }
In this example, the Strategy interface defines a method for executing an operation. The AddOperation and SubtractOperation classes implement this interface, providing specific algorithms. The Context class utilizes the Strategy interface, allowing it to dynamically change the behavior based on the strategy implementation passed to it.
Interfaces also play an important role in defining callback mechanisms, especially in asynchronous programming. When implementing user interface components or handling events, interfaces can be used to define callbacks that will be executed when a certain event occurs. This approach promotes loose coupling between components, making your code more modular and easier to maintain.
// Define a ClickListener interface for event handling public interface ClickListener { void onClick(); } // Button class that uses ClickListener public class Button { private ClickListener listener; public void setClickListener(ClickListener listener) { this.listener = listener; } public void click() { if (listener != null) { listener.onClick(); // Invoke the callback } } } // Using the Button with a ClickListener public class Main { public static void main(String[] args) { Button button = new Button(); button.setClickListener(() -> System.out.println("Button clicked!")); // Lambda expression for the listener button.click(); // Outputs: Button clicked! } }
Here, the ClickListener interface defines a callback method onClick(). The Button class contains a method to set a listener and invokes the callback when the button is clicked. By allowing any class to implement the ClickListener interface, you can easily define different behaviors for the button click while keeping the Button class agnostic of the specific implementations.
Another critical use case for interfaces is when defining Data Access Objects (DAOs). Interfaces can abstract away the implementation details of data persistence, allowing for different data sources (like databases or web services) to be swapped in and out seamlessly. This promotes the Single Responsibility Principle by separating the data access logic from the business logic of your application.
// Define the User DAO interface public interface UserDAO { void addUser(User user); User getUser(int id); } // SQL implementation of the UserDAO public class SQLUserDAO implements UserDAO { @Override public void addUser(User user) { // Code to add user to SQL database } @Override public User getUser(int id) { // Code to retrieve user from SQL database return null; // Placeholder return } } // Using the UserDAO public class Main { public static void main(String[] args) { UserDAO userDAO = new SQLUserDAO(); User user = new User("Luke Douglas"); userDAO.addUser(user); } }
In this example, the UserDAO interface defines the methods for user data operations. The SQLUserDAO class implements the interface, providing the actual data access logic. This abstraction allows you to easily create other implementations, such as a NoSQLUserDAO, without altering the code that depends on the UserDAO interface.
Interfaces in Java are not just a means of achieving abstraction; they are pivotal in implementing flexible and scalable designs across various contexts. By allowing different implementations to conform to a defined contract, interfaces empower developers to craft applications that are easier to manage and extend in the long run.
Best Practices for Designing Interfaces
When designing interfaces in Java, adhering to best practices especially important for ensuring that the interfaces serve their intended purpose effectively and enhance the overall architecture of your code. A well-designed interface can lead to maintainable, extensible, and robust systems. Here are some key best practices to ponder when creating interfaces:
1. Keep Interfaces Focused
Interfaces should be narrowly focused on a specific behavior or responsibility. That’s often referred to as the Interface Segregation Principle. Rather than creating a large interface with many methods, break it down into smaller, more cohesive interfaces. This ensures that implementing classes only need to be concerned with the methods that are relevant to them, reducing unnecessary complexity.
// Interface for a printer public interface Printer { void print(); } // Interface for a scanner public interface Scanner { void scan(); } // Combined interface public interface MultifunctionDevice extends Printer, Scanner { void fax(); }
In this example, we have separate interfaces for printing and scanning. The MultifunctionDevice
interface extends both but only when a class needs to implement both functionalities. This keeps each interface minimal and focused.
2. Favor Composition Over Inheritance
Instead of relying solely on inheritance from a base class, think using interfaces to define behaviors that can be composed. This allows for greater flexibility and reduces tight coupling between classes. By using interfaces, you can change implementations dynamically at runtime, which is a significant advantage over traditional class inheritance.
// An interface representing a behavior public interface Flyable { void fly(); } // A class implementing the Flyable interface public class Bird implements Flyable { @Override public void fly() { System.out.println("Bird is flying."); } } // A class that can incorporate the Flyable behavior public class Airplane implements Flyable { @Override public void fly() { System.out.println("Airplane is flying."); } }
In this case, both Bird
and Airplane
implement the Flyable
interface. This allows you to work with instances of Flyable
without needing to know the specific type of flying object.
3. Avoid Exposing Implementation Details
Interfaces should define the behavior without exposing any implementation details. This encapsulation allows developers to change the underlying implementations without affecting the clients that rely on the interface. Stick to method signatures and avoid including implementation logic within the interface.
// The interface should not include implementation details public interface Database { void connect(); void disconnect(); } // The implementation handles the details public class MySQLDatabase implements Database { @Override public void connect() { // Specific connection logic } @Override public void disconnect() { // Specific disconnection logic } }
Here, the Database
interface does not reveal how the connection is established. The client code interacts with the interface without needing to know the specifics of the implementation.
4. Use Descriptive Names
Choosing the right names for interfaces is essential for clarity and understanding. Interface names should convey the purpose of the interface clearly. Use suffixes like able
, ible
, or or
to indicate behavior when appropriate, such as Runnable
or Serializable
.
// An interface for a data processor public interface DataProcessor { void process(Data data); }
The name DataProcessor
clearly indicates the purpose of the interface, making it easier for other developers to understand its functionality at a glance.
5. Document Your Interfaces
Documentation is vital in promoting understanding and ease of use for interfaces. Use Javadoc comments to describe the purpose of the interface and the expected behavior of its methods. This practice helps other developers understand how to use the interface correctly and what to expect from its implementations.
// Javadoc comment for the interface /** * This interface defines the operations for a generic stack data structure. */ public interface Stack { void push(T item); T pop(); boolean isEmpty(); }
By documenting the Stack
interface, you provide clear guidance on its intended use, which facilitates better implementation and usage across your codebase.
6. Ponder Default Methods Carefully
With the introduction of default methods in Java 8, you have the option to provide default implementations in interfaces. While this feature can reduce boilerplate code, it should be used judiciously. Default methods can lead to ambiguity if multiple interfaces are implemented that contain default methods with the same signature. Always prioritize clarity and maintainability over convenience.
// An interface with a default method public interface Vehicle { void start(); default void honk() { System.out.println("Vehicle honk!"); } } // A class that implements the Vehicle interface public class Car implements Vehicle { @Override public void start() { System.out.println("Car starting."); } }
In this case, the Car
class can utilize the default behavior of the honk
method. However, if a more specific implementation is required, the class can override it. Use default methods to add functionality without breaking existing implementations.
Designing interfaces with intention and following best practices leads to cleaner, more maintainable code. By focusing on clarity, keeping interfaces narrow, using composition, and documenting thoroughly, you foster an environment conducive to high-quality software development.