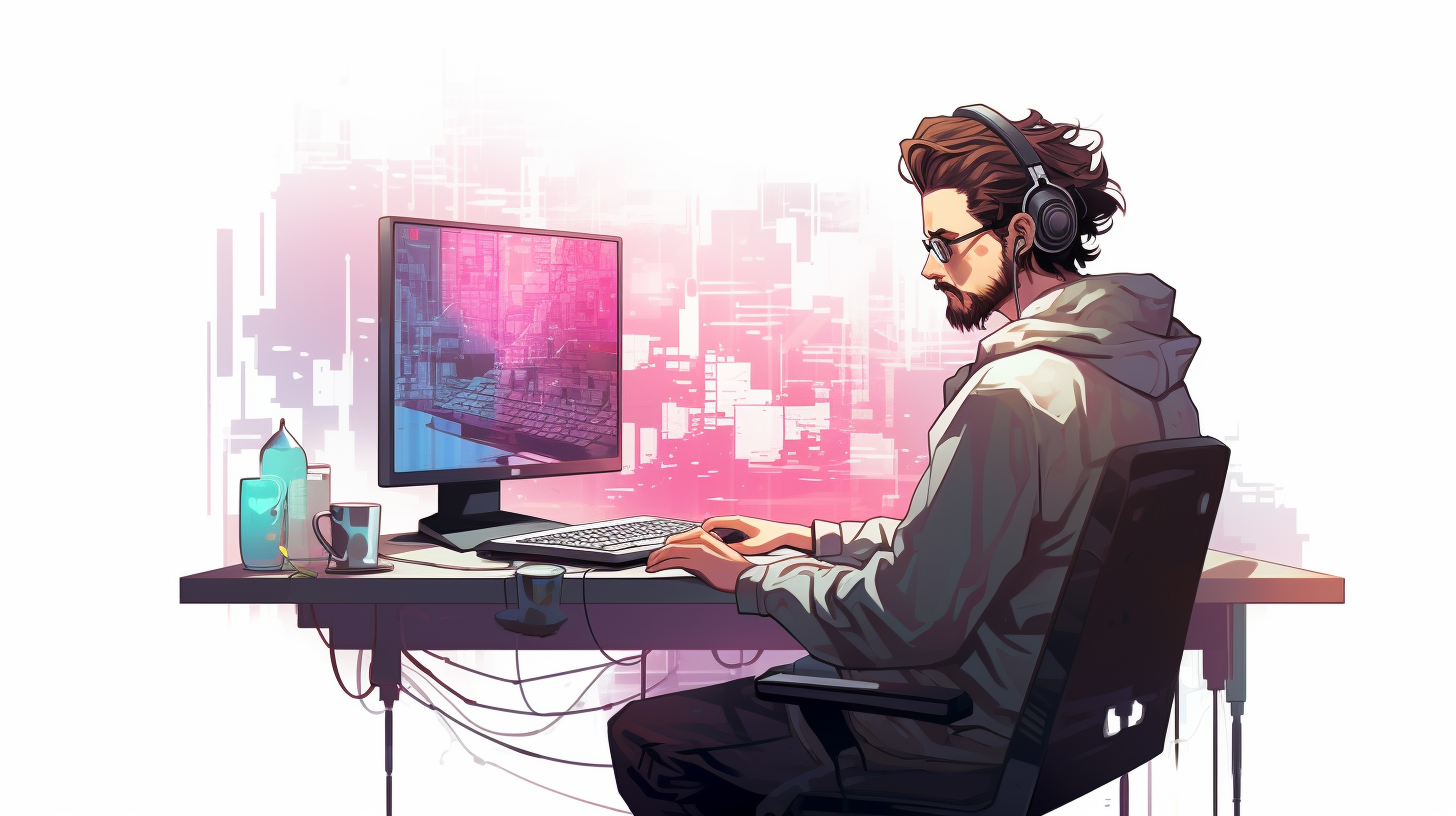
Understanding JavaScript Hoisting
JavaScript hoisting is a fundamental concept that can often lead to confusion among developers, especially those transitioning from other programming languages. At its core, hoisting is the behavior in which variable and function declarations are moved to the top of their containing scope during the compilation phase. This means that you can use variables and functions before you declare them in the code, as JavaScript effectively “hoists” these declarations to the top.
To illustrate this, think the following example:
console.log(myVar); // Output: undefined var myVar = 5; console.log(myVar); // Output: 5
In the code above, when we first attempt to log myVar
, JavaScript doesn’t throw a ReferenceError, as one might expect. Instead, it outputs undefined. This occurs because the declaration of myVar
is hoisted to the top of its scope, but the assignment of the value 5
remains in its original position. Hence, before the assignment, the variable exists, but it’s uninitialized.
It’s crucial to note that only the declarations are hoisted, not the initializations. This distinction is pivotal in understanding the unpredictable nature of hoisting, especially in larger codebases where variable scope and execution contexts become complex.
Another layer of complexity arises with function declarations. Function hoisting allows you to invoke a function before its definition appears in the code, which is a behavior distinct from how variables behave:
sayHello(); // Output: Hello, World! function sayHello() { console.log("Hello, World!"); }
In this case, the entire function declaration is hoisted, allowing the function to be called before it is defined. This capability can lead to cleaner code and a more organized structure, but it also necessitates a solid understanding of how hoisting operates to avoid confusion.
Hoisting is an integral aspect of JavaScript that allows for flexibility in how code is written. However, it is also a source of potential pitfalls if not fully understood, necessitating that developers pay careful attention to how and where they declare their variables and functions.
How Variable Hoisting Works
When diving deeper into how variable hoisting works, it is essential to understand that hoisting applies differently depending on the type of variable declaration used. In JavaScript, the two most common declarations are var, let, and const, with var being the original mechanism for declaring variables prior to the rise of ES6.
With var, hoisting occurs in a predictable manner. As we’ve seen, variable declarations are lifted to the top of their function or global scope. This means that if you declare a variable using var within a function, it will be available throughout that entire function, regardless of where it’s declared within the code block. Here’s an example illustrating this:
function hoistExample() { console.log(hoistedVar); // Output: undefined var hoistedVar = 'I am hoisted!'; console.log(hoistedVar); // Output: I am hoisted! } hoistExample();
In the example above, the variable hoistedVar is declared with var, and its declaration is hoisted to the top of the hoistExample function. This explains why the first log outputs undefined instead of throwing an error. The assignment happens later in the code, which does not affect the hoisting behavior.
On the other hand, when using let and const, the hoisting behavior is slightly different. While declarations made with let and const are also hoisted, they’re not initialized. This results in a temporal dead zone (TDZ), which is the period from the start of the block until the declaration is encountered. Any attempt to access these variables before their declaration will result in a ReferenceError:
function letConstExample() { console.log(myLet); // ReferenceError: Cannot access 'myLet' before initialization let myLet = 'I am not hoisted!'; } letConstExample();
In this case, trying to log myLet results in a ReferenceError because the variable is in the temporal dead zone until its declaration is reached in the code. The same principle applies to const, where similar behavior can be observed:
function constExample() { console.log(myConst); // ReferenceError: Cannot access 'myConst' before initialization const myConst = 'I am also not hoisted!'; } constExample();
As such, understanding these distinctions is key for developers. Using var can lead to unintended behaviors due to its hoisting characteristics, while let and const offer a more predictable environment, albeit with stricter access rules. This knowledge empowers developers to write clearer, more maintainable code, ultimately minimizing confusion when dealing with variable hoisting in JavaScript.
Function Hoisting Explained
Function hoisting, while similar in concept to variable hoisting, has its own intricacies that are essential for any JavaScript developer to grasp. When you declare a function using a function declaration, the entire function definition is hoisted to the top of its enclosing scope. This characteristic allows you to call the function before its actual position in the code, which can enhance readability and organization in certain scenarios.
To elucidate this concept, think the following JavaScript snippet:
sayHello(); // Output: Hello, World! function sayHello() { console.log("Hello, World!"); }
In this example, the call to sayHello()
occurs before the function is defined. Nonetheless, JavaScript executes this without a hitch, resulting in the expected output. This behavior stems from the fact that the entire function definition is hoisted, not merely the declaration. Thus, any call to sayHello()
can safely occur before the function’s definition without leading to an error.
This predictability of function hoisting is one of the aspects that make it different from variable hoisting. However, function expressions, which are often confused with function declarations, do not enjoy the same privilege. When a function is defined as a function expression, only the variable declaration itself is hoisted, not the assignment. This distinction can lead to unexpected behaviors if not properly understood.
Take a look at this example:
sayGoodbye(); // TypeError: sayGoodbye is not a function var sayGoodbye = function() { console.log("Goodbye, World!"); };
Here, the call to sayGoodbye()
occurs before the function expression is assigned. Because only the variable declaration is hoisted (the variable sayGoodbye
exists but is undefined at the point of the call), JavaScript throws a TypeError, indicating that it cannot invoke undefined
as a function. That’s an important lesson in understanding hoisting mechanics: function declarations and function expressions behave differently when it comes to hoisting.
Another important aspect of function hoisting relates to the use of named function expressions. In this case, the function name is also hoisted, but the behavior remains akin to that of a regular function expression:
sayFarewell(); // TypeError: sayFarewell is not a function var sayFarewell = function farewell() { console.log("Farewell, World!"); };
As observed, even with a named function expression, the function cannot be called before its definition, leading to the same TypeError. The name farewell
is hoisted, but the actual function body is not, resulting in the same limitations as before.
While function hoisting can simplify code structure by allowing function calls before their definitions, developers must remain vigilant about the nuances that differentiate function declarations from function expressions. Recognizing these distinctions is key to using hoisting effectively without falling into the common traps that can arise from misunderstandings of how JavaScript handles function declarations and expressions. The implications of these behaviors become increasingly critical as the complexity of your codebase grows, making it imperative to cultivate a firm grasp of function hoisting principles.
The Role of ‘let’ and ‘const’ in Hoisting
The introduction of block-scoped variables in JavaScript, namely let and const, brought about fundamental changes in the behavior of hoisting. Unlike var, which is hoisted in a way that allows it to be accessed (though uninitialized) throughout its function or global scope, let and const create a distinct scenario that developers must navigate. Specifically, both declarations are hoisted to the top of their block scope; however, they remain uninitialized until their actual declaration is reached within the code. This uninitialized state leads to what’s known as the temporal dead zone (TDZ).
The temporal dead zone is the period from the beginning of the block until the variable is declared. During this time, any attempt to access the variable will result in a ReferenceError. Think the following example that highlights this behavior:
function temporalDeadZoneExample() { console.log(myLet); // ReferenceError: Cannot access 'myLet' before initialization let myLet = 'I am in the TDZ!'; } temporalDeadZoneExample();
In this scenario, the attempt to log myLet before its declaration leads to a ReferenceError. This occurs because myLet exists in the TDZ until we reach its declaration, emphasizing a critical distinction between var and the newer let and const declarations.
Moreover, const behaves similarly in terms of hoisting and the TDZ. Any attempt to access a const variable before it’s initialized will also yield a ReferenceError. Here’s an illustrative example:
function constTDZExample() { console.log(myConst); // ReferenceError: Cannot access 'myConst' before initialization const myConst = 'I am also in the TDZ!'; } constTDZExample();
In both cases, the principles of hoisting still apply; however, the introduction of let and const has shifted the dynamics significantly. While var allows for more flexible access to variables, it can also lead to ambiguous code behavior due to its hoisting mechanics. In contrast, let and const impose stricter rules that enhance the predictability of the code, although they require a deeper understanding of scope and initialization.
Another vital aspect of let and const is their block-scoping. Variables declared with these keywords are limited to the block in which they are declared, which is a significant departure from var, where the scope is function-level or global. This behavior can be demonstrated as follows:
function blockScopeExample() { if (true) { let blockScopedVar = 'I am block-scoped!'; console.log(blockScopedVar); // Output: I am block-scoped! } console.log(blockScopedVar); // ReferenceError: blockScopedVar is not defined } blockScopeExample();
In this example, blockScopedVar is accessible only within the if block. Outside of that block, attempting to log the variable results in a ReferenceError, highlighting how let and const enhance the encapsulation of variables within their defined scopes.
In summary, the introduction of let and const in JavaScript has redefined the landscape of hoisting by introducing the temporal dead zone and block scoping. This evolution empowers developers to write clearer, more modular code while minimizing the risks associated with variable declarations. As you continue to work with JavaScript, embracing these concepts will lead to better practices and a more profound understanding of how hoisting operates in modern JavaScript development.
Common Hoisting Pitfalls
When it comes to hoisting, developers often encounter a myriad of pitfalls that can lead to unexpected behavior in their JavaScript code. These pitfalls arise from a misunderstanding of how hoisting interacts with variable declarations, especially when using var, let, and const, as well as function declarations and expressions. Recognizing these common mistakes is essential for writing robust code and avoiding frustrating debugging sessions.
One prevalent pitfall involves the use of var. Since variable declarations using var are hoisted, it is easy to assume that you can reference a variable anywhere in its enclosing scope, regardless of where it is declared. This can lead to scenarios where the variable is accessed before its initialization, resulting in undefined values:
console.log(myVar); // Output: undefined var myVar = 'I am defined!'; console.log(myVar); // Output: I am defined!
In this case, the initial console log returns undefined, which can be misleading for developers who expect that accessing a variable before its declaration would throw an error. Instead, understanding that the declaration has been hoisted (but not the initialization) clarifies why this occurs.
Another common source of confusion stems from function expressions. Unlike function declarations, which are fully hoisted, function expressions only hoist the variable name and leave it uninitialized until the assignment occurs. This discrepancy can lead to TypeErrors if an attempt is made to invoke a function expression before it has been defined:
sayGoodbye(); // TypeError: sayGoodbye is not a function var sayGoodbye = function() { console.log("Goodbye, World!"); };
In this example, the call to sayGoodbye() results in a TypeError because the variable sayGoodbye is hoisted but not initialized to a function until after the call. Understanding this distinction is important for avoiding such pitfalls in your code.
Furthermore, the introduction of let and const has created additional complexities. Both declarations are hoisted, but they remain uninitialized until their definition is reached. This leads to the well-known temporal dead zone (TDZ), where accessing a variable before its declaration results in a ReferenceError:
function letTDZExample() { console.log(myLet); // ReferenceError: Cannot access 'myLet' before initialization let myLet = 'I exist!'; } letTDZExample();
Such errors can be particularly jarring for developers used to var’s more lenient behavior. The strictness imposed by let and const can be a double-edged sword; while it enhances code clarity and predictability, it also requires that developers remain vigilant about variable scope and initialization.
Moreover, developers often encounter scoping issues when using let and const. Unlike var, which is function-scoped or globally scoped, let and const are block-scoped. This can lead to confusion when attempting to access variables outside of their defined blocks:
function blockScopePitfall() { if (true) { let blockScopedVar = 'I am only visible here!'; } console.log(blockScopedVar); // ReferenceError: blockScopedVar is not defined } blockScopePitfall();
In this scenario, blockScopedVar is inaccessible outside of the if block, leading to a ReferenceError when trying to log it. Understanding this block-level scope is essential for avoiding similar pitfalls.
Hoisting in JavaScript presents several common pitfalls that can trip up even seasoned developers. By being aware of the nuances surrounding variable and function hoisting, as well as the specific behaviors of var, let, and const, developers can write more predictable and maintainable code, reducing the likelihood of encountering these frustrating issues in their projects.
Practical Examples and Use Cases
Practical examples are invaluable for grasping the subtleties of JavaScript hoisting. They provide a tangible way to see how hoisting operates in real-world scenarios. Let’s delve into some illustrative cases that highlight both typical behaviors and the potential pitfalls associated with variable and function hoisting.
One of the most simpler instances of hoisting involves variable declarations. Ponder the following example:
console.log(aVar); // Output: undefined var aVar = 10; console.log(aVar); // Output: 10
Here, the variable aVar is declared using var, which is hoisted to the top of its scope. Consequently, when the first console.log statement is executed, it does not throw an error but instead returns undefined, as the initialization (assignment of 10) occurs after the log statement. This behavior can be perplexing for developers who might expect an error, leading to confusion about the variable’s state before its actual assignment.
An additional example demonstrates how this can be misinterpreted, particularly within a function:
function hoistingTest() { console.log(testVar); // Output: undefined var testVar = 'Hoisted!'; } hoistingTest();
Similar to the previous example, the declaration of testVar is hoisted to the top of hoistingTest(), leading to the same undefined output. Understanding this pattern is critical for writing clear and effective JavaScript.
Now, let’s explore function hoisting further through practical usage. Function declarations can be called before their defined location in the code due to hoisting:
greet(); // Output: Hello! function greet() { console.log("Hello!"); }
This function can be invoked before its definition without any errors. However, contrast this with a function expression example:
farewell(); // TypeError: farewell is not a function var farewell = function() { console.log("Goodbye!"); };
In this case, the call to farewell() results in a TypeError because the variable farewell is declared but not initialized as a function at the point of invocation. This illustrates the crucial difference between function declarations and expressions regarding hoisting.
As we transition to the topic of let and const, let’s ponder their behavior in practical terms. Using let introduces a temporal dead zone, which can cause unexpected behaviors:
function letExample() { console.log(myLet); // ReferenceError: Cannot access 'myLet' before initialization let myLet = 'I am let!'; } letExample();
As shown, trying to access myLet before its declaration results in a ReferenceError. This reinforces the necessity for developers to be attentive to the scope and initialization timing of variables declared with let.
Another practical example illustrates the scoping rules of let and const:
function blockScopeExample() { if (true) { let blockScoped = 'I exist inside the block!'; console.log(blockScoped); // Output: I exist inside the block! } console.log(blockScoped); // ReferenceError: blockScoped is not defined } blockScopeExample();
Here, blockScoped is confined to the if block, and any attempt to access it outside that block results in a ReferenceError. This behavior underscores the importance of understanding the block-scoping feature introduced with let and const, which aids in preventing variable collisions and enhances code maintainability.
Through these practical examples, we can see how the rules of hoisting operate not just in theory but also in practice. Understanding these nuances empowers developers to write cleaner, more predictable JavaScript, effectively minimizing confusion and errors that may arise from improper handling of variable and function declarations.