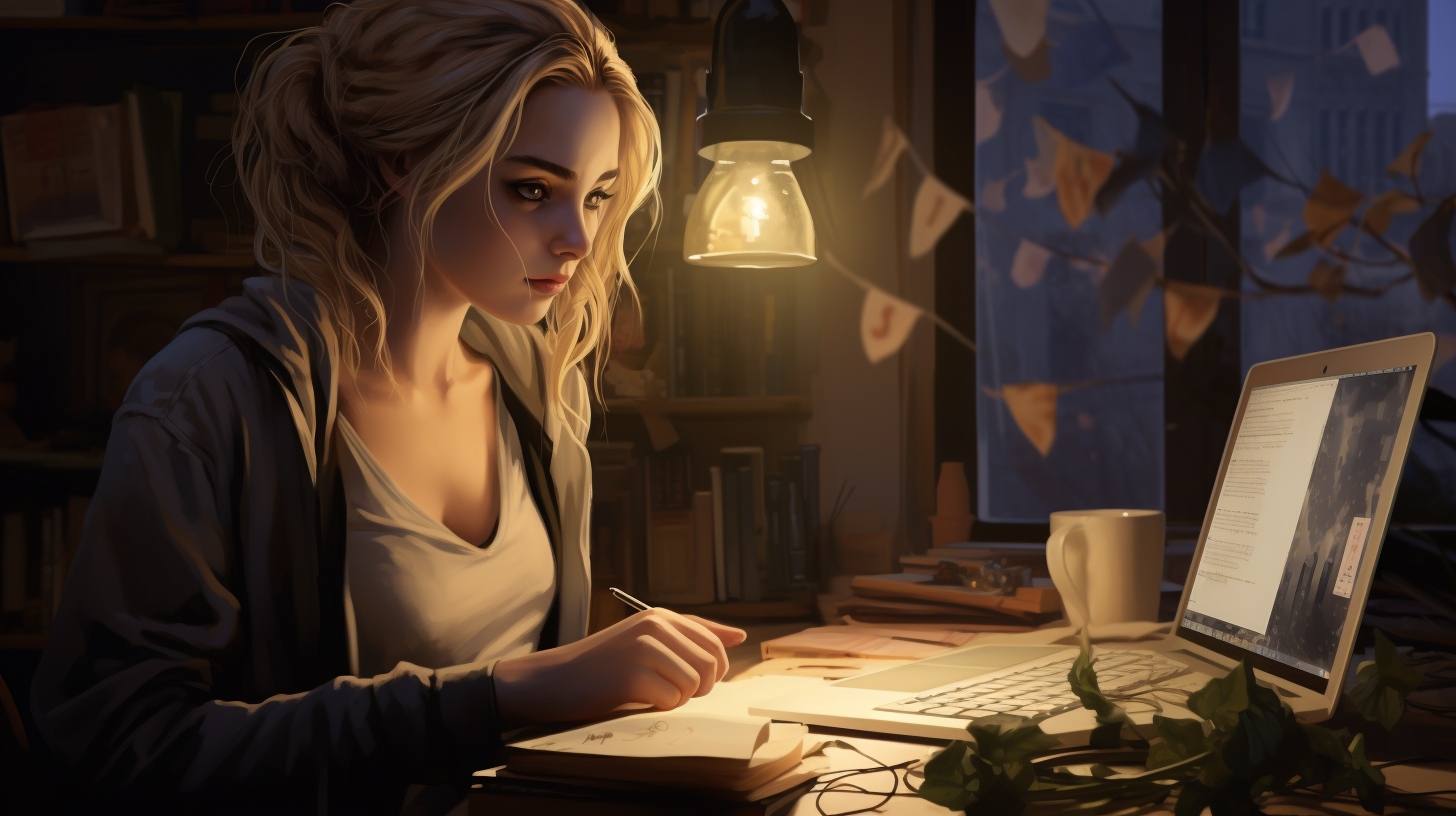
Understanding Python Inheritance and Polymorphism
Inheritance is a fundamental concept in object-oriented programming, allowing a class to inherit the properties and methods of another class. In Python, this mechanism promotes code reusability and establishes a hierarchical relationship between classes. The class this is inherited from is called the base class (or parent class), while the class that inherits is referred to as the derived class (or child class).
To illustrate inheritance, think the following simple example where we have a base class called Animal
and a derived class called Dog
. The Dog
class will inherit the properties of the Animal
class.
class Animal: def __init__(self, name): self.name = name def speak(self): return "Some sound" class Dog(Animal): def speak(self): return "Woof!" my_dog = Dog("Buddy") print(my_dog.name) # Output: Buddy print(my_dog.speak()) # Output: Woof!
Here, the Dog
class inherits the __init__
method from the Animal
class, allowing us to create an instance of Dog
with a name. The speak
method is overridden in the Dog
class to provide a specific implementation.
In Python, inheritance is achieved by passing the base class as an argument to the derived class. That’s done within parentheses following the derived class name, as shown in the Dog
class definition. When a method is called on an instance of the derived class, Python first checks if the method is implemented in the derived class. If it’s not found there, it looks in the base class.
This structure allows for a clean and organized codebase, improving maintainability. As applications grow in complexity, using inheritance ensures that shared behavior is centralized, reducing redundancy and potential errors.
Additionally, inheritance can introduce the idea of polymorphism. This occurs when different classes implement the same method in different ways, allowing for a common interface while enabling varied behaviors. This versatility is key in designing systems that are both flexible and scalable.
Types of Inheritance: Single, Multiple, and Multilevel
In Python, we can categorize inheritance into several types, each serving specific design purposes and architectural needs. The primary types of inheritance include single, multiple, and multilevel inheritance. Understanding these types very important for writing efficient and maintainable code.
Single Inheritance is the simplest form of inheritance where a derived class inherits from a single base class. This simpler relationship makes it easy to follow the hierarchy. Here’s an example:
class Vehicle: def start_engine(self): return "Engine started" class Car(Vehicle): def drive(self): return "Car is driving" my_car = Car() print(my_car.start_engine()) # Output: Engine started print(my_car.drive()) # Output: Car is driving
In this example, the Car
class inherits from the Vehicle
class. The Car
class can use the start_engine
method defined in the Vehicle
class, illustrating the single inheritance concept.
Multiple Inheritance occurs when a class can inherit from more than one base class. While this feature increases flexibility and code reuse, it can also introduce complexity, especially concerning method resolution order (MRO). Python uses the C3 linearization algorithm to determine the order in which classes are checked for methods. Here’s an example:
class Engine: def start(self): return "Engine starting" class MusicSystem: def play_music(self): return "Playing music" class Car(Engine, MusicSystem): def drive(self): return "Car is driving" my_car = Car() print(my_car.start()) # Output: Engine starting print(my_car.play_music()) # Output: Playing music print(my_car.drive()) # Output: Car is driving
In this example, Car
inherits from both Engine
and MusicSystem
. This allows the Car
class to access methods from both parent classes, demonstrating the power of multiple inheritance.
Multilevel Inheritance is a scenario where a class is derived from another derived class. This forms a chain of inheritance, allowing the derived class to inherit from its parent class as well as its grandparent class. Here’s a quick example:
class Vehicle: def start_engine(self): return "Engine started" class Car(Vehicle): def drive(self): return "Car is driving" class SportsCar(Car): def race(self): return "Sports car is racing" my_sports_car = SportsCar() print(my_sports_car.start_engine()) # Output: Engine started print(my_sports_car.drive()) # Output: Car is driving print(my_sports_car.race()) # Output: Sports car is racing
Here, the SportsCar
class inherits from the Car
class, which in turn inherits from the Vehicle
class. This allows SportsCar
to access methods from both Car
and Vehicle
, showcasing how multilevel inheritance can create more specialized classes.
Each inheritance type has its own advantages and trade-offs. Single inheritance is simple and simpler, while multiple inheritance provides flexibility at the cost of potential complications in method resolution. Multilevel inheritance can create a rich hierarchy but may also lead to complexities if not designed carefully. Understanding how to effectively utilize these inheritance types is essential for any Python programmer aiming to design robust and scalable applications.
The Role of Method Overriding in Polymorphism
Method overriding is a powerful feature in object-oriented programming that plays a pivotal role in achieving polymorphism. In Python, it allows a derived class to provide a specific implementation of a method this is already defined in its base class. This capability is essential in scenarios where the behavior of a method needs to be altered to fit the context of the derived class. By overriding methods, we maintain a consistent interface while allowing for the diversity of behavior among different classes.
To illustrate method overriding, let’s consider an example involving a base class called Shape and two derived classes: Circle and Rectangle. Each class will implement the method area
, which calculates the area of the shape in its own way:
class Shape: def area(self): raise NotImplementedError("This method should be overridden by subclasses") class Circle(Shape): def __init__(self, radius): self.radius = radius def area(self): return 3.14 * (self.radius ** 2) class Rectangle(Shape): def __init__(self, width, height): self.width = width self.height = height def area(self): return self.width * self.height my_circle = Circle(5) my_rectangle = Rectangle(4, 6) print(my_circle.area()) # Output: 78.5 print(my_rectangle.area()) # Output: 24
In this example, the Shape
class contains a method area
, which is intended to be overridden in derived classes. The Circle
class overrides this method to provide its specific calculation for the area of a circle, while the Rectangle
class implements its version for calculating the area of a rectangle.
When we call the area
method on instances of these classes, Python utilizes the method defined in the respective derived class. This demonstrates polymorphism as the same method name area
is used, but it behaves differently depending on the object type invoking it.
Moreover, method overriding is particularly useful in frameworks and libraries where base classes define default behavior, and derived classes can modify or extend that behavior without altering the base class. This promotes a clean separation of concerns, making the codebase more maintainable and scalable. For instance, if we wanted to add a new shape, say Triangle
, we only need to implement the area
method specific to triangles without changing the existing structure:
class Triangle(Shape): def __init__(self, base, height): self.base = base self.height = height def area(self): return 0.5 * self.base * self.height my_triangle = Triangle(4, 5) print(my_triangle.area()) # Output: 10.0
This encapsulation of behavior through method overriding enhances code flexibility, allowing developers to introduce new functionalities seamlessly. In summary, method overriding is an integral aspect of implementing polymorphism in Python, enabling classes to provide specific behaviors while adhering to a common interface.
Implementing Interfaces and Abstract Classes
In Python, the concepts of interfaces and abstract classes are utilized to define a blueprint for other classes, ensuring that they adhere to particular structure and behavior. While Python does not have formal interfaces like some other languages, it provides abstract base classes (ABCs) in the ‘abc’ module that allow you to achieve similar functionality. An abstract class can contain abstract methods, which are methods that are declared but contain no implementation. Derived classes are required to implement these methods, ensuring that the essential behaviors are present.
To illustrate this, let’s define an abstract class called Shape that specifies an interface for calculating the area of different shapes:
from abc import ABC, abstractmethod class Shape(ABC): @abstractmethod def area(self): pass @abstractmethod def perimeter(self): pass
Here, the Shape class inherits from ABC and defines two abstract methods: area and perimeter. Any derived class must implement these methods to be instantiated. Let’s create two classes, Circle and Rectangle, which will inherit from Shape and provide concrete implementations:
import math class Circle(Shape): def __init__(self, radius): self.radius = radius def area(self): return math.pi * (self.radius ** 2) def perimeter(self): return 2 * math.pi * self.radius class Rectangle(Shape): def __init__(self, width, height): self.width = width self.height = height def area(self): return self.width * self.height def perimeter(self): return 2 * (self.width + self.height)
In this example, both Circle and Rectangle provide implementations for the area and perimeter methods specified in the Shape abstract class. This enforces a consistent interface while allowing each shape to define its unique behavior.
Let’s instantiate these classes and see how they behave:
my_circle = Circle(5) my_rectangle = Rectangle(4, 6) print(f"Circle Area: {my_circle.area()}") # Output: Circle Area: 78.53981633974483 print(f"Circle Perimeter: {my_circle.perimeter()}") # Output: Circle Perimeter: 31.41592653589793 print(f"Rectangle Area: {my_rectangle.area()}") # Output: Rectangle Area: 24 print(f"Rectangle Perimeter: {my_rectangle.perimeter()}") # Output: Rectangle Perimeter: 20
Through this use of abstract classes, we ensure that all derived classes adhere to the same interface, allowing for polymorphic behavior where a method can accept objects of different shapes without needing to know their specific types. This leads to more maintainable and flexible code, as you can add new shapes by simply implementing the required methods without modifying existing code.
Implementing interfaces and abstract classes in Python enables developers to define clear contracts for behavior, fostering a structured approach to class design that’s conducive to polymorphism and code reusability. This is especially powerful in larger systems where the complexity can be managed through well-defined interactions between diverse components.
Practical Examples of Inheritance and Polymorphism in Python
Practical examples of inheritance and polymorphism in Python demonstrate the power and flexibility of these concepts in real-world scenarios. To solidify our understanding, let’s ponder a case involving an e-commerce platform where different types of products share common characteristics but also possess unique attributes and behaviors.
We can start with a base class called Product, which will define the general attributes and methods applicable to all products. Then, we will derive specific product classes such as Book and ElectronicDevice that extend the functionality of the base class.
class Product: def __init__(self, name, price): self.name = name self.price = price def get_info(self): return f"Product: {self.name}, Price: ${self.price:.2f}" class Book(Product): def __init__(self, name, price, author): super().__init__(name, price) self.author = author def get_info(self): return f"{super().get_info()}, Author: {self.author}" class ElectronicDevice(Product): def __init__(self, name, price, brand): super().__init__(name, price) self.brand = brand def get_info(self): return f"{super().get_info()}, Brand: {self.brand}" my_book = Book("1984", 15.99, "George Orwell") my_device = ElectronicDevice("Smartphone", 699.99, "TechBrand") print(my_book.get_info()) # Output: Product: 1984, Price: $15.99, Author: George Orwell print(my_device.get_info()) # Output: Product: Smartphone, Price: $699.99, Brand: TechBrand
In this example, the Product class serves as a base class providing a common get_info method to retrieve product details. The derived classes Book and ElectronicDevice inherit from Product and provide their specific implementations of the get_info method, thereby demonstrating polymorphism. When we create instances of these classes and call the get_info method, Python dynamically binds the method call to the appropriate implementation based on the instance type.
Next, let’s explore a more complex scenario involving discount policies where we can further illustrate inheritance and polymorphism. We can create a base class called Discount and derive specific discount types, such as PercentageDiscount and FixedAmountDiscount.
class Discount: def apply_discount(self, price): raise NotImplementedError("This method should be overridden by subclasses") class PercentageDiscount(Discount): def __init__(self, percentage): self.percentage = percentage def apply_discount(self, price): discount_amount = price * (self.percentage / 100) return price - discount_amount class FixedAmountDiscount(Discount): def __init__(self, amount): self.amount = amount def apply_discount(self, price): return price - self.amount # Example usage original_price = 100.00 percentage_discount = PercentageDiscount(10) # 10% discount fixed_discount = FixedAmountDiscount(15) # $15 discount print(f"Original Price: ${original_price:.2f}") print(f"Price after Percentage Discount: ${percentage_discount.apply_discount(original_price):.2f}") # Output: $90.00 print(f"Price after Fixed Amount Discount: ${fixed_discount.apply_discount(original_price):.2f}") # Output: $85.00
In this case, the Discount class defines a method apply_discount, which is meant to be overridden by its subclasses. The PercentageDiscount and FixedAmountDiscount classes provide their specific implementations of how to apply a discount to a price. When we instantiate these classes and call the apply_discount method, the correct behavior is executed based on the type of discount applied.
Through these examples, we see how inheritance and polymorphism not only promote code reusability but also facilitate the creation of systems that can easily adapt to changes. By defining a clear hierarchy and allowing specific behaviors to be implemented in derived classes, we can craft flexible and maintainable applications that grow alongside our needs.