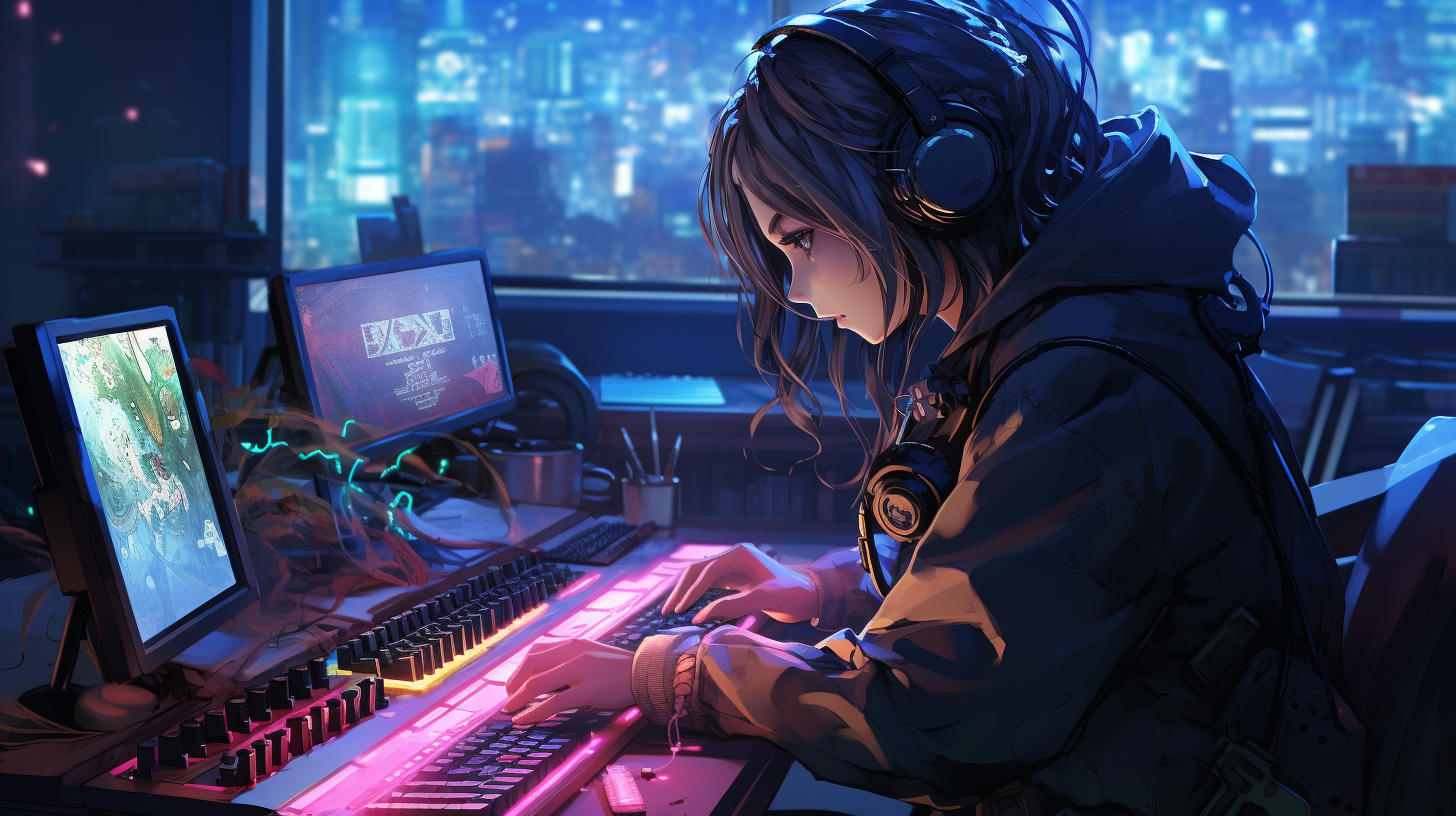
Understanding Python Lists and List Operations
Python lists are among the most versatile data structures available in the Python programming language, serving as the foundation for managing and organizing collections of items. Lists can hold an ordered collection of items, which means that the elements maintain their sequence, allowing for easy access and manipulation. They can contain various data types, including integers, strings, other lists, and even objects.
Dynamic Nature: One of the key attributes of Python lists is their dynamic nature. Unlike arrays in many other programming languages, Python lists can grow and shrink in size as needed. This allows developers to add, remove, or modify elements without having to specify the size of the list upfront. This flexibility very important for many applications where the amount of data being processed can vary significantly.
Syntax and Basics: A Python list is defined by enclosing its elements in square brackets, separated by commas. Here’s a simple example:
my_list = [1, 2, 3, "Hello", 5.6]
In this example, my_list
contains integers, a string, and a float. The ability to mix data types within a single list is one of the features that make Python lists particularly powerful.
Zero-Based Indexing: Python lists utilize zero-based indexing, meaning that the first element of the list is accessed with index 0. This indexing system allows for simpler retrieval of elements:
first_element = my_list[0] # Accessing the first element
Accessing elements out of bounds will raise an IndexError
, which is a common error that developers need to manage when working with lists.
List Mutability: Lists in Python are mutable, meaning that their contents can be changed after creation. You can modify an existing list by assigning a new value to an index or using methods like append()
and remove()
:
my_list[1] = 42 # Change the second element my_list.append("New Item") # Add a new item at the end my_list.remove(3) # Remove the item '3' from the list
This mutability allows for dynamic data handling, enabling developers to craft algorithms that efficiently manipulate collections of data.
Nesting Lists: Lists can also contain other lists, allowing for the creation of multi-dimensional structures. This capability is often used in applications requiring matrices or tables:
nested_list = [[1, 2, 3], ["A", "B", "C"], [True, False]]
To access elements in nested lists, you can chain indices:
first_inner_element = nested_list[0][1] # Accessing '2'
Understanding these fundamental characteristics of Python lists will provide the foundation you need to leverage their power effectively in your programming endeavors. The richness of list operations and the flexibility they offer can lead to elegant solutions to complex problems when used correctly.
Creating and Initializing Lists
Creating and initializing lists in Python is a simpler process, yet it offers a wealth of flexibility and power. There are several ways to create lists, each suited for different scenarios depending on the needs of your application.
The simplest way to create a list is by enclosing a comma-separated sequence of elements in square brackets. That is known as list literal syntax. Here’s an example of creating a list with various data types:
my_list = [10, "Python", 3.14, True]
In this example, my_list
contains an integer, a string, a float, and a boolean value. This illustrates the versatility of lists in holding heterogeneous data types.
Another common method to create lists is by using the list()
constructor. That’s particularly useful when you want to convert an iterable (like a string or a tuple) into a list. For instance:
my_string = "hello" my_list_from_string = list(my_string) # Converts string to list of characters
After executing this code, my_list_from_string
will contain: ['h', 'e', 'l', 'l', 'o']
. This showcases how the list()
constructor can break down data into its elemental components.
Python also supports list comprehensions, a concise way to create lists based on existing lists or iterables. This feature is not only syntactically pleasing but also enhances performance due to its underlying optimizations. Here’s an example that generates a list of squares for the numbers from 0 to 9:
squares = [x**2 for x in range(10)]
After this code runs, squares
will yield: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
. This shows how list comprehensions can be utilized to create lists dynamically in a clean and readable manner.
In scenarios where you need to initialize a list with default values, you can use the multiplication operator. This approach is efficient for creating lists of a fixed size filled with the same element. For example:
default_list = [0] * 5 # Creates a list of five zeros: [0, 0, 0, 0, 0]
Be cautious, however, when initializing lists this way, especially with mutable objects like lists themselves. Doing something like this:
nested_list = [[]] * 3 # Creates a list of three references to the same list
This results in nested_list
containing three references to the same inner list. Modifying one of them will affect all three:
nested_list[0].append(1) print(nested_list) # Output: [[1], [1], [1]]
To create a true nested list where each inner list is independent, you should use a loop or a list comprehension:
independent_nested_list = [[] for _ in range(3)] # Creates three independent lists
In summary, the ability to create and initialize lists using various methods empowers Python programmers to handle a diverse array of data manipulation tasks efficiently. Whether you’re using list literals, the list()
constructor, list comprehensions, or initializing with default values, Python lists provide a robust framework for managing collections of items in an elegant manner.
Common List Operations and Methods
When working with Python lists, understanding the various operations and methods available to manipulate them is important for efficient programming. Lists come equipped with a plethora of built-in methods that facilitate a wide range of operations, from adding and removing elements to sorting and searching. By mastering these methods, developers can handle data collections more adeptly and write cleaner, more efficient code.
One of the most commonly used methods is append(), which adds an element to the end of a list. This operation is simpler and can be performed as follows:
my_list = [1, 2, 3] my_list.append(4) # Now my_list becomes [1, 2, 3, 4]
For cases where you need to insert an element at a specific position, the insert() method is available. It takes two arguments: the index at which to insert the element and the element itself:
my_list.insert(1, 'new') # my_list now is [1, 'new', 2, 3, 4]
Removing elements from a list can be accomplished using the remove() method, which removes the first occurrence of a specified value:
my_list.remove(2) # my_list becomes [1, 'new', 3, 4]
If you need to delete an item by its index, del is your go-to option:
del my_list[0] # my_list is now ['new', 3, 4]
Another method, pop(), not only removes an element but also returns it, which can be handy if you need to manipulate the removed value:
last_item = my_list.pop() # Removes and returns 4, my_list is now ['new', 3]
For scenarios where you need to check if an item exists in a list, the in keyword provides a simple and readable approach:
if 3 in my_list: print("3 is in the list") # This will print the message
Sorting and reversing lists can be executed easily with the sort() and reverse() methods, respectively. Here’s how you can sort a list:
my_numbers = [4, 1, 3, 2] my_numbers.sort() # my_numbers is now [1, 2, 3, 4]
To reverse the elements in a list, use:
my_numbers.reverse() # my_numbers becomes [4, 3, 2, 1]
Lastly, when it comes to finding the length of a list, the built-in len() function is invaluable:
length_of_list = len(my_list) # This will store the length of my_list
The rich set of operations and methods available for Python lists allows developers to perform a variety of tasks efficiently. Understanding how to manipulate lists through these operations not only enhances code clarity but also optimizes performance, ensuring that applications can handle data collections seamlessly.
List Slicing and Indexing Techniques
List slicing and indexing are two fundamental techniques that allow developers to access and manipulate the elements of a list with precision and ease. By using Python’s powerful slicing capabilities, you can retrieve portions of a list or individual elements without the need for complex loops or conditional statements.
At the core of Python list slicing is the syntax list[start:end:step]
. This allows you to define a sub-list based on the start index, the end index (exclusive), and an optional step that determines the stride of indices taken from the original list. For instance, consider the following example:
my_list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] sliced_list = my_list[2:7] # This will yield [2, 3, 4, 5, 6]
In this case, sliced_list
contains the elements from index 2 to index 6. Remember that the end index is not included in the slice, which is a common source of confusion for new Python developers.
Moreover, omitting the start or end indices allows you to slice from the beginning or to the end of the list. For example:
beginning_slice = my_list[:5] # Yields [0, 1, 2, 3, 4] end_slice = my_list[5:] # Yields [5, 6, 7, 8, 9]
When it comes to the step parameter, it can be particularly useful for retrieving every nth element from the list. Here’s an example:
step_slice = my_list[::2] # Yields [0, 2, 4, 6, 8]
This slice takes every second element from the list, starting from index 0. If you want to reverse the list, you can use a step of -1:
reversed_list = my_list[::-1] # Yields [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
In addition to slicing, Python lists also support indexing, which allows you to access individual elements directly by their position in the list. As previously mentioned, indexing starts from zero, which means you can easily retrieve the first element with:
first_element = my_list[0] # Yields 0
To access elements from the end of the list, you can use negative indexing. For instance, the last item can be retrieved with:
last_element = my_list[-1] # Yields 9
This indexing mechanism is particularly useful for working with dynamic lists where lengths may vary, allowing for robust access patterns without explicit length checks.
When manipulating lists with slicing and indexing, it’s essential to be aware of the boundaries of the list. Attempting to slice or index outside of the valid range will raise an IndexError. An example of this would be:
out_of_bounds = my_list[10] # Raises IndexError
By mastering these slicing and indexing techniques, you can effectively handle and manipulate Python lists in a clear and efficient manner, unlocking the true power of Python’s list capabilities in your programming toolkit.
Best Practices for Working with Lists in Python
When it comes to working with Python lists, adopting best practices can significantly enhance code readability, maintainability, and performance. This section aims to highlight some essential best practices for managing Python lists effectively.
1. Use List Comprehensions: One of the most powerful features in Python is list comprehension, which allows you to create lists concisely and efficiently. Instead of using a loop to build a list, you can apply a transformation in a single line. For instance, rather than writing:
squares = [] for i in range(10): squares.append(i ** 2)
You can achieve the same result with a list comprehension:
squares = [i ** 2 for i in range(10)]
This not only reduces lines of code but also improves performance by minimizing overhead.
2. Avoid Modifying Lists While Iterating: Modifying a list while iterating through it can lead to unexpected behavior. Instead, consider creating a new list that contains the desired modifications or use list comprehensions. For example, if you want to remove all even numbers from a list:
original_list = [1, 2, 3, 4, 5, 6] filtered_list = [num for num in original_list if num % 2 != 0]
This approach keeps your original list intact and avoids the pitfalls of modifying a list during iteration.
3. Use Built-in Functions for Common Tasks: Python provides a rich set of built-in functions that can help simplify list operations. For instance, if you need to find the maximum or minimum element in a list, use max()
and min()
instead of iterating through the list manually:
numbers = [3, 1, 4, 1, 5, 9] max_value = max(numbers) # Returns 9 min_value = min(numbers) # Returns 1
These functions are optimized and make your code cleaner and easier to understand.
4. Be Cautious with Mutable Objects: When working with lists that contain mutable objects (like other lists or dictionaries), be mindful of how Python handles references. If you create a list of lists using multiplication, such as:
nested_list = [[]] * 3
All three inner lists will reference the same object. Therefore, modifying one will affect all. Instead, use a list comprehension:
independent_nested_list = [[] for _ in range(3)]
By creating independent inner lists, you will avoid unintended side effects.
5. Use Appropriate Data Structures: While lists are versatile, they may not always be the most efficient choice depending on the use case. If you need to frequently add or remove elements from both ends, consider using a deque
from the collections
module. For example:
from collections import deque my_deque = deque([1, 2, 3]) my_deque.appendleft(0) # Adds 0 to the front
This will provide better performance for operations on the ends compared to lists.
6. Keep Lists Homogeneous When Possible: While Python allows heterogeneous lists, keeping lists homogeneous (all elements of the same type) can lead to more predictable behavior and improve performance. For example, if you know that a list will always contain integers, stick to that type to avoid unnecessary type checks and conversions during operations.
By implementing these best practices, you can write Python code that’s not only efficient and effective but also clean and maintainable. Mastering list best practices allows you to utilize Python’s powerful list capabilities fully, leading to more elegant and robust solutions in your programming tasks.