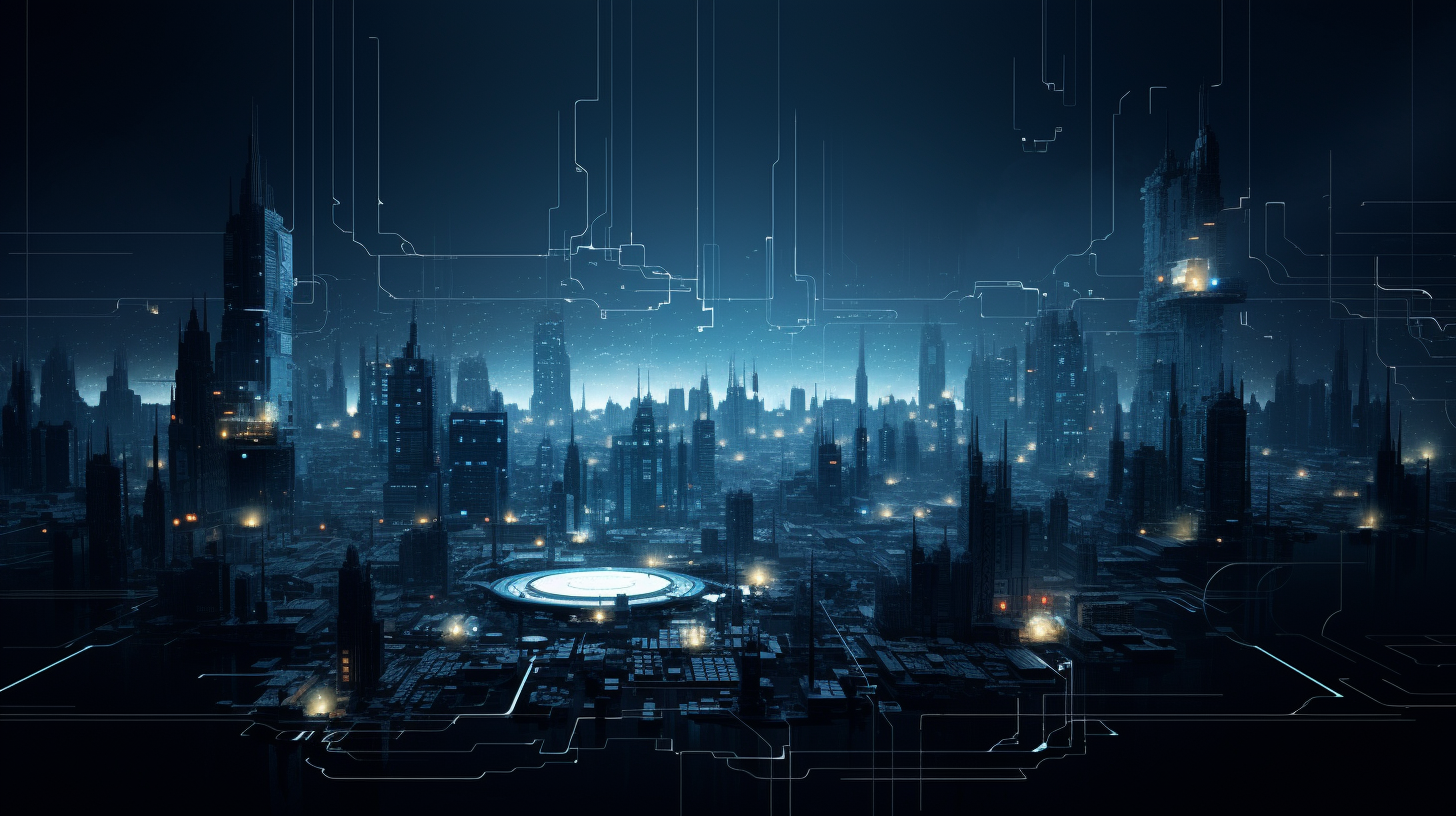
Understanding Python Variables and Data Types
In Python, a variable acts as a symbolic name for a value and is a fundamental concept that helps in storing and manipulating data. Unlike some other programming languages, Python does not require explicit declaration of variable types; instead, it dynamically infers the type from the value assigned to the variable at runtime. This dynamic typing allows for flexibility, but it also necessitates understanding how Python manages memory and types behind the scenes.
When you create a variable in Python, you’re essentially creating a reference to a value stored in memory. Here’s a simple example:
# Assigning an integer value to a variable x = 10 # Assigning a string value to another variable name = "Alice"
In the above code, the variable x
references the integer 10
, while name
references the string "Alice"
. The power of variables lies in their ability to refer to different values over time. You can easily change the reference of a variable by assigning it a new value:
# Reassigning x to a new value x = 20 # Now name refers to a different string name = "Bob"
It’s crucial to understand that when you reassign a variable, the previous value it referenced is not destroyed immediately; it might remain in memory until Python’s garbage collector decides to reclaim that space. This characteristic highlights the importance of memory management in Python, especially when dealing with larger datasets or more complex applications.
Additionally, variables in Python are case-sensitive. This means that myVariable
, MyVariable
, and MYVARIABLE
are considered distinct identifiers:
myVariable = 5 MyVariable = 10 MYVARIABLE = 15
Each of these variables could hold a different value without conflict due to their unique names. This aspect can lead to bugs if not handled carefully, particularly in larger codebases where similar names may exist.
A thorough understanding of how variables work in Python is vital for effective programming. The seamless nature of variable creation and manipulation fosters a dynamic environment that is both powerful and daunting. Embracing these concepts will pave the way for deeper exploration of data handling in Python.
Exploring Python Data Types
Exploring the landscape of Python data types reveals a rich and diverse toolkit that developers can wield to manage and manipulate data effectively. Python, being a dynamically typed language, supports a variety of built-in data types, which can be broadly categorized into several key groups: numeric types, sequences, mappings, sets, and boolean values.
The numeric types in Python include integers and floating-point numbers. Integers are whole numbers, while floating-point numbers are represented with a decimal point, allowing for fractional values. Here’s a simpler illustration:
# Integer and floating-point examples a = 5 # An integer b = 3.14 # A floating-point number
Additionally, Python offers complex numbers, which are expressed with a real and an imaginary part. This can be especially useful in fields such as engineering and scientific computing:
# Complex number example c = 2 + 3j # 2 is the real part, 3 is the imaginary part
Moving beyond numbers, sequences in Python—such as lists, tuples, and strings—allow for the storage and manipulation of ordered collections of items. Lists are mutable, meaning you can change their contents after creation:
# List example fruits = ['apple', 'banana', 'cherry'] fruits[1] = 'blueberry' # Changing 'banana' to 'blueberry'
On the other hand, tuples are immutable. Once a tuple is created, its contents cannot be altered:
# Tuple example coordinates = (10.0, 20.0) # A tuple holding x and y coordinates
Strings, a fundamental data type for textual data, can be manipulated using various methods. They’re also immutable, which means any operation that seems to modify a string actually creates a new string:
# String manipulation greeting = "Hello" greeting = greeting + ", World!" # Creating a new string by concatenation
In addition to sequences, Python provides mappings, with dictionaries being the primary example. Dictionaries store key-value pairs, enabling efficient data retrieval:
# Dictionary example student = {'name': 'Alice', 'age': 22, 'major': 'Computer Science'}
Sets represent an unordered collection of unique items, and they offer operations such as union, intersection, and difference, which can be particularly useful for handling collections of data:
# Set example unique_numbers = {1, 2, 3, 1, 2} # This will only store {1, 2, 3}
Lastly, the boolean data type, which can take on the values True or False, plays a critical role in control flow and conditionals throughout Python programming. It allows you to make decisions based on conditions:
# Boolean example is_valid = True if is_valid: print("Valid data!")
Understanding these various data types not only enhances your ability to write effective Python code but also contributes to your capability to solve complex problems with elegance and efficiency. Each type has its own set of properties and methods, allowing for a wide range of operations that can be performed on data.
Type Conversion and Casting
Type conversion and casting are important concepts in Python programming that allow developers to manipulate and transform data types to meet specific needs. In Python, type conversion refers to the process of converting a value from one data type to another. That is particularly useful when performing operations that require operands to be of the same type. Python provides several built-in functions to handle common type conversions.
One of the most frequently used functions is int()
, which converts a value to an integer. If the value is a floating-point number, int()
truncates the decimal part. If the input is a string that represents a number, it will be converted to an integer as long as it’s a valid representation. Here’s how you can use it:
# Converting a float to an integer num_float = 3.99 num_int = int(num_float) # num_int will be 3 # Converting a string to an integer num_str = "42" num_int_from_str = int(num_str) # num_int_from_str will be 42
Similarly, the float()
function converts a value to a floating-point number. It works with integers, strings, and other numeric types:
# Converting an integer to a float num_int = 5 num_float = float(num_int) # num_float will be 5.0 # Converting a string to a float num_str = "3.14" num_float_from_str = float(num_str) # num_float_from_str will be 3.14
The str()
function can be used for converting values to strings. That’s particularly useful when you need to concatenate numbers with other strings:
# Converting an integer to a string num_int = 10 num_str = str(num_int) # num_str will be '10' # Concatenating strings greeting = "The number is " + num_str # 'The number is 10'
Type casting is slightly different from type conversion. It typically refers to the explicit conversion of one data type to another through the use of a casting operator or function. In Python, this often involves the aforementioned built-in functions. It very important to note that while converting, one must always ensure that the data being converted is compatible with the target type; otherwise, Python will raise an error.
In practice, type conversion and casting can help you avoid type-related errors when performing calculations or operations. For instance, if you attempt to add a string to a number directly, Python will throw a TypeError. However, by explicitly converting types, you can achieve the desired results without encountering such issues:
# Error example # result = "The result is: " + 10 # This will raise TypeError # Correcting the error using type conversion result = "The result is: " + str(10) # This will work: 'The result is: 10'
Moreover, Python also supports implicit type conversion, where the interpreter automatically converts one data type to another when it sees fit. For example, when performing arithmetic operations involving both integers and floats, Python automatically converts integers to floats to prevent loss of precision:
# Implicit type conversion int_value = 5 float_value = 2.5 result = int_value + float_value # result will be 7.5 (float)
Understanding type conversion and casting is essential for effective Python programming. It enhances your ability to manipulate data types as required, ensuring that your code runs smoothly without type-related errors. By mastering these concepts, you can leverage Python’s flexibility to handle a variety of data types in a seamless and efficient manner.
Mutable vs Immutable Data Types
The distinction between mutable and immutable data types is one of the cornerstones of understanding Python’s behavior when managing variable states and memory. In Python, mutable data types allow for modification of their contents without changing their identity, while immutable data types do not permit such alterations post-creation.
Mutable data types include lists, dictionaries, and sets. For instance, when you modify a list, you’re not creating a new list; rather, you’re changing the contents of the existing list in place:
# Mutable list example numbers = [1, 2, 3] numbers[1] = 4 # Changing the second element print(numbers) # Output: [1, 4, 3]
In this example, the list numbers
retains its identity even though its contents have changed. That is an important feature when passing lists to functions; they can be altered without needing to return a new list.
On the other hand, immutable data types, such as tuples, strings, and frozensets, cannot be altered once they are defined. Any operation that appears to modify an immutable type actually results in the creation of a new object:
# Immutable string example greeting = "Hello" greeting = greeting + ", World!" # Creates a new string print(greeting) # Output: "Hello, World!"
Here, the original string greeting
remains unchanged in memory, while the operation results in the creation of a new string. This behavior can lead to subtle bugs if a programmer mistakenly believes that an immutable variable has been modified in place.
Understanding the implications of mutability very important, particularly in the context of function arguments. When passing a mutable object to a function, changes made to that object can affect the original object outside the function. Conversely, passing an immutable object will not yield any side effects on the original object:
def modify_list(lst): lst.append(4) # Modifying the original list def modify_string(s): s += " World!" # This does not affect the original string my_list = [1, 2, 3] my_string = "Hello" modify_list(my_list) modify_string(my_string) print(my_list) # Output: [1, 2, 3, 4] print(my_string) # Output: "Hello"
In this example, my_list
is modified by modify_list
, while my_string
remains unchanged when passed to modify_string
. This distinction is essential for developers to grasp in order to avoid unintended side effects and bugs in their code.
Memory management also plays a key role in understanding mutability. Mutable objects can lead to challenges in memory management, especially in large applications where the identity of objects is not easily tracked. Immutable objects, while safer in this regard, can lead to higher memory usage since they create a new instance upon modification.
Ultimately, knowing when to use mutable versus immutable data types can enhance both the performance and reliability of your Python code. By using the strengths of each, developers can write cleaner, more efficient, and more predictable programs.
Best Practices for Variable Naming and Usage
When it comes to variable naming in Python, certain conventions and best practices can significantly enhance code readability and maintainability. Choosing meaningful names for your variables is important, as it provides context and clarity, allowing both you and others to understand your code better. A well-named variable can convey its purpose at a glance, while a poorly named variable can lead to confusion and misunderstandings.
Descriptive Names
One of the fundamental principles of naming variables is to use descriptive names that reflect the data they hold. For instance, instead of using generic names like x
or temp
, opt for names that clearly describe the variable’s content or purpose. If a variable holds a student’s age, a name like student_age
is far more informative than simply age
.
student_age = 20 # Descriptive variable name
Using descriptive names not only aids in understanding the code but also assists in debugging and maintaining it, especially as codebases grow in size and complexity.
Follow Python Naming Conventions
Python has several naming conventions that developers are encouraged to follow. The most notable conventions are:
- Use snake_case for variable names, where words are separated by underscores (e.g.,
user_name
,total_price
). - Use lowercase letters for variable names, reserving uppercase letters for class names (e.g.,
CalculateTotal
). - Avoid using single character variable names except in specific contexts, such as loop counters (e.g.,
for i in range(10)
).
By adhering to these conventions, you can ensure consistency and improve the overall readability of your code.
Avoid Reserved Words
Python has a set of reserved words that cannot be used as variable names, including class
, def
, if
, else
, and many others. Attempting to use these keywords as variable names will result in a syntax error. To prevent such issues, always refer to the list of reserved words in Python and ensure your variable names are unique and descriptive.
# Incorrect usage of reserved word # def = 5 # This will raise a SyntaxError # Correct usage my_variable = 5 # That is valid
Use Consistent and Predictable Naming
Consistency in naming is essential. If you decide to use a particular naming scheme (e.g., camelCase
or snake_case
), stick with it throughout your codebase. This consistency helps maintain a uniform style, making it easier for others (and yourself) to read and navigate your code.
Limit Scope and Use Contextual Naming
When naming variables, ponder their scope. For instance, if a variable is being used within a small function, a short name might be appropriate. However, if the variable is global or part of a larger module, a more descriptive name is warranted. Additionally, use context to inform your naming decisions. In a financial application, variables like amount_due
or payment_status
can provide immediate context, reducing the cognitive load on anyone reading your code.
def calculate_total_price(prices): total_price = sum(prices) # Local variable with context-appropriate name return total_price
Commenting and Documentation
Even with the best variable names, there may be cases where additional context is beneficial. Use comments and docstrings to provide further clarification on the purpose of a variable, especially if the naming alone does not convey all necessary information. This practice not only aids you in the short term but also helps others who may work with your code in the future.
# Calculate the total price of items in the cart total_price = sum(prices) # Total price based on the list of prices
By applying these best practices for variable naming and usage, you can greatly enhance the clarity, maintainability, and overall quality of your Python code. Remember, the goal is to write code that is not only functional but also understandable, allowing for efficient collaboration and future development.