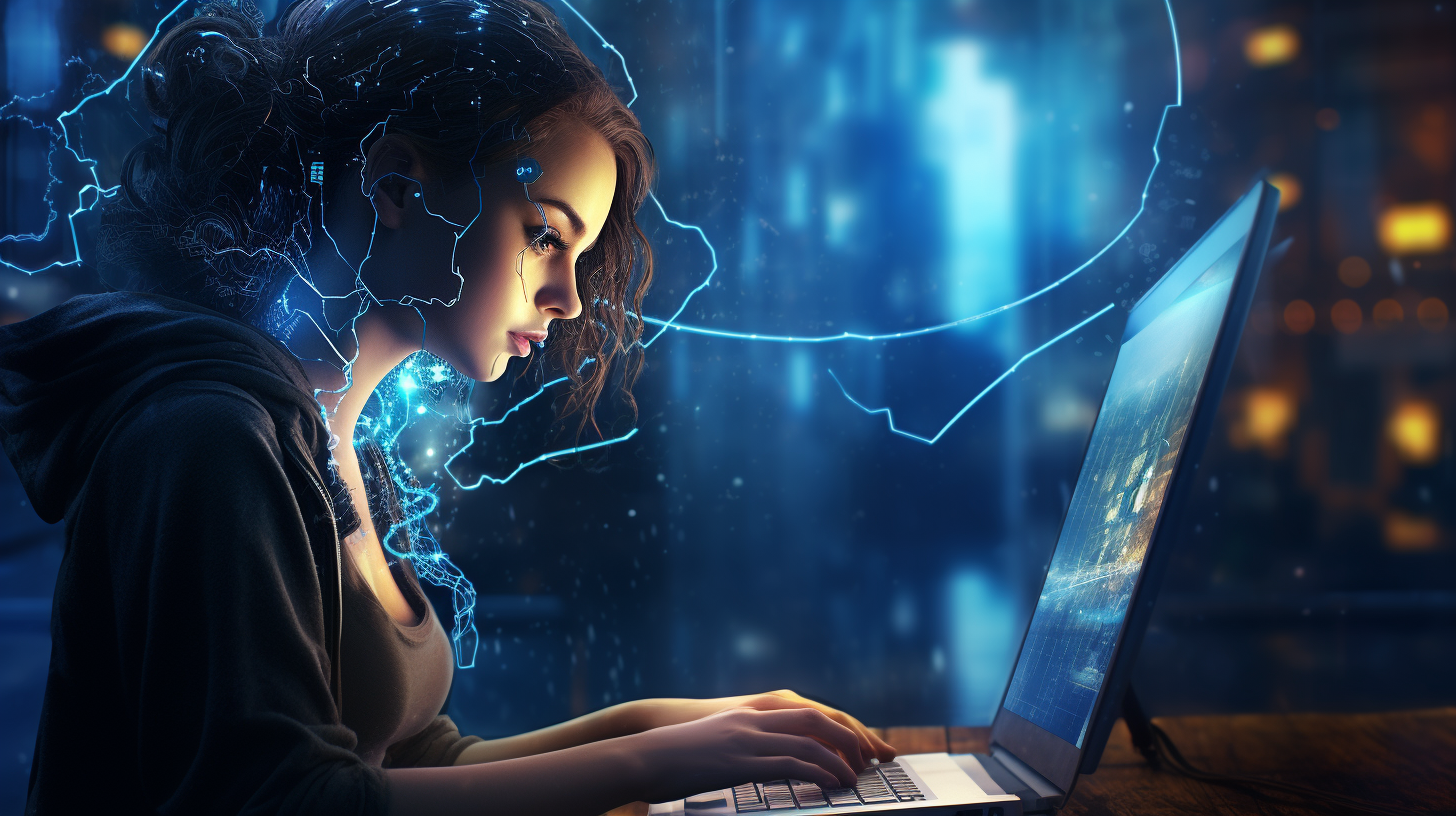
Understanding Python’s ‘self’ and ‘init’
In Python, the concept of ‘self’ especially important for defining the behavior and properties of class instances. It serves as a reference to the instance of the class itself, allowing access to the attributes and methods defined within the class. When you define a method in a class, the first parameter is conventionally named ‘self’, although you could technically name it anything. However, adhering to this convention is important for consistency and readability.
Using ‘self’ in class methods is essential for distinguishing between instance variables and local variables. Instance variables are those that belong to the object created from the class, and ‘self’ allows you to set and retrieve those variables. Here’s an example:
class Dog: def __init__(self, name, age): self.name = name # Instance variable self.age = age # Instance variable def bark(self): print(f"{self.name} says Woof!") my_dog = Dog("Buddy", 3) my_dog.bark() # Output: Buddy says Woof!
In the __init__
method, ‘self’ is used not only to bind the parameters name
and age
to the instance of the class but also to provide a way to access those attributes in other methods, like bark
in this case.
Another important aspect of ‘self’ is that it allows for a clear and consistent interface when interacting with objects. When you create multiple instances of the same class, each instance retains its own separate state, thanks to ‘self’. This reinforces the concept of encapsulation in object-oriented programming, as each object’s data is protected within its own namespace.
Here’s an example that illustrates this point:
class Cat: def __init__(self, name): self.name = name def meow(self): print(f"{self.name} says Meow!") cat1 = Cat("Whiskers") cat2 = Cat("Felix") cat1.meow() # Output: Whiskers says Meow! cat2.meow() # Output: Felix says Meow!
In this example, even though both cat1
and cat2
are instances of the Cat
class, they contain different data, and the ‘self’ reference allows each instance to maintain its own state. That is the essence of object-oriented programming in Python—managing related data and behaviors in a cohesive manner.
The Purpose and Functionality of ‘__init__’ Method
The __init__ method in Python serves as the initializer or constructor for class instances. This method is automatically called when a new object of the class is created, and it is the ideal place to set up initial values for instance variables. Without the __init__ method, the class would not have a built-in way to set these variables upon instantiation, leaving objects in an undefined state. This concept is fundamental to maintaining the integrity and usability of an object, allowing developers to ensure that an object is always created with a valid state.
When you define an __init__ method, you typically include parameters that allow you to pass values as arguments during object creation. The first parameter must always be ‘self’, which refers to the instance being created. This parameter is followed by any additional parameters you wish to include for initializing instance variables.
class Car: def __init__(self, make, model, year): self.make = make # Instance variable for car's make self.model = model # Instance variable for car's model self.year = year # Instance variable for car's year def display_info(self): print(f"{self.year} {self.make} {self.model}") my_car = Car("Toyota", "Corolla", 2020) my_car.display_info() # Output: 2020 Toyota Corolla
In the Car class above, the __init__ method takes three parameters—make, model, and year—along with ‘self’. These parameters are then assigned to instance variables that can be accessed by other methods within the class, like display_info. This encapsulation of data ensures that each Car instance holds its unique information, thereby promoting modularity and reusability in your code.
Furthermore, the __init__ method can also be designed to include default values for parameters. This flexibility allows you to create instances with varying levels of detail without the need for multiple constructors, which is a challenge in languages that do not support method overloading. You can provide default values directly in the parameter list.
class Bicycle: def __init__(self, brand, type="Mountain"): self.brand = brand # Instance variable for bicycle's brand self.type = type # Instance variable for bicycle's type def display_info(self): print(f"{self.brand} {self.type} Bicycle") my_bike = Bicycle("Trek") # Type defaults to "Mountain" my_bike.display_info() # Output: Trek Mountain Bicycle my_road_bike = Bicycle("Giant", "Road") # Specify type my_road_bike.display_info() # Output: Giant Road Bicycle
In the Bicycle class, the type parameter has a default value of “Mountain”. When creating an instance of Bicycle, if the type is not specified, it defaults to “Mountain”, demonstrating the flexibility that __init__ provides. This capability to set default parameters can significantly reduce the complexity of your class design, allowing for more concise code without sacrificing functionality.
Additionally, the __init__ method can also include logic to validate parameters before assigning them to instance variables. This can be particularly useful in ensuring that objects are always created with valid data, thereby helping to prevent potential runtime errors down the line.
class Person: def __init__(self, name, age): if age < 0: raise ValueError("Age cannot be negative") self.name = name self.age = age def display_info(self): print(f"{self.name} is {self.age} years old.") try: person1 = Person("Alice", 30) person1.display_info() # Output: Alice is 30 years old. person2 = Person("Bob", -5) # This will raise an error except ValueError as e: print(e) # Output: Age cannot be negative
In the Person class, an exception is raised if an invalid age is provided. This validation step within the __init__ method enforces rules on object instantiation, enhancing the robustness of your application by catching errors early in the execution flow. This demonstrates the powerful role that the __init__ method plays in not only creating objects but also ensuring their proper configuration and integrity from the outset.
How ‘self’ Enhances Object-Oriented Programming
When we delve into the mechanics of how ‘self’ enhances object-oriented programming (OOP) in Python, it’s vital to recognize that ‘self’ is not just a mere convention but a foundational element that facilitates the very essence of OOP. By employing ‘self’, Python allows for a seamless interaction with instance attributes and methods, thus promoting a clear structure in our programming paradigms.
The beauty of ‘self’ lies in its ability to differentiate between instance variables and local variables within class methods. This distinction is important, as it allows for the encapsulation of data, where each object maintains its own state without interfering with others. Ponder the following class definition, which illustrates this point:
class Library: def __init__(self, name): self.name = name self.books = [] # Initialize an empty list of books def add_book(self, book_title): self.books.append(book_title) # Use 'self' to access instance variable print(f'Added {book_title} to {self.name}.') def show_books(self): print(f'Books in {self.name}: {", ".join(self.books)}') city_library = Library("City Library") city_library.add_book("1984") # Output: Added 1984 to City Library. city_library.add_book("To Kill a Mockingbird") # Output: Added To Kill a Mockingbird to City Library. city_library.show_books() # Output: Books in City Library: 1984, To Kill a Mockingbird
In this example, the Library
class encapsulates its own list of books. The use of ‘self’ in the methods add_book
and show_books
ensures that each instance of Library
can independently manage its book collection. This independent state management is a core principle of OOP, effectively separating concerns and reducing potential errors.
Furthermore, the ‘self’ reference promotes readability and clarity in the code. It explicitly indicates that a variable belongs to an instance of the class, thereby making it easier for developers to understand the flow of data within their programs. This clarity becomes especially important when classes grow in size and complexity. Consider the following enhancement of our initial example, where we include error handling to maintain integrity:
class EnhancedLibrary: def __init__(self, name): self.name = name self.books = [] def add_book(self, book_title): if book_title in self.books: print(f'{book_title} already exists in the library.') else: self.books.append(book_title) print(f'Added {book_title} to {self.name}.') def show_books(self): if not self.books: print(f'{self.name} has no books.') else: print(f'Books in {self.name}: {", ".join(self.books)}') my_library = EnhancedLibrary("My Local Library") my_library.add_book("1984") # Output: Added 1984 to My Local Library. my_library.add_book("1984") # Output: 1984 already exists in the library. my_library.show_books() # Output: Books in My Local Library: 1984
The EnhancedLibrary
class not only manages a collection of books but also ensures that duplicate entries are avoided, reinforcing the idea that ‘self’ is an enabler for both functionality and data integrity. By allowing each instance to maintain its own state, ‘self’ fosters a programming environment where objects are not just data holders but active entities that can enforce rules and behaviors.
The role of ‘self’ in Python is integral to the principles of object-oriented programming. It allows for the encapsulation of data, clarity of code, and the validation of state, all of which contribute to building robust, maintainable, and scalable applications. As we continue to explore the nuances of Python’s OOP capabilities, the importance of ‘self’ as a reference to instance state will become increasingly apparent, underlining its pivotal role in the art of programming.
Common Mistakes and Best Practices with ‘self’ and ‘__init__’
When working with ‘self’ and the ‘__init__’ method in Python, it is easy to encounter pitfalls that can hinder the clarity and functionality of your classes. One common mistake is failing to use ‘self’ consistently, particularly when trying to access instance variables. Forgetting to prefix instance variables with ‘self’ will lead to Python treating them as local variables, which can result in unexpected behavior or errors. For example, think the following class:
class Person: def __init__(self, name, age): name = name # Incorrect: This does not bind to self.name age = age # Incorrect: This does not bind to self.age def display_info(self): print(f"{self.name} is {self.age} years old.") # Raises AttributeError try: person1 = Person("Alice", 30) person1.display_info() except AttributeError as e: print(e) # Output: 'Person' object has no attribute 'name'
The above code will raise an AttributeError because the instance variables ‘name’ and ‘age’ were never assigned to ‘self’. To fix this, you need to ensure that all instance variables are correctly prefixed with ‘self’:
class Person: def __init__(self, name, age): self.name = name # Correctly bind to self.name self.age = age # Correctly bind to self.age def display_info(self): print(f"{self.name} is {self.age} years old.") person1 = Person("Alice", 30) person1.display_info() # Output: Alice is 30 years old.
Another frequent oversight is in the management of mutable default arguments in the ‘__init__’ method. Using mutable types like lists or dictionaries as default values can lead to shared state among instances, which is often unintended. Consider this example:
class Inventory: def __init__(self, items=[]): # Mutable default argument self.items = items inventory1 = Inventory() inventory1.items.append("Sword") inventory2 = Inventory() print(inventory2.items) # Output: ['Sword'] - Not what was expected!
In this case, both inventory1 and inventory2 share the same list instance. The recommended approach is to use ‘None’ as a default value and create a new list inside the function if needed:
class Inventory: def __init__(self, items=None): # Use None as the default value if items is None: items = [] # Create a new list self.items = items inventory1 = Inventory() inventory1.items.append("Sword") inventory2 = Inventory() print(inventory2.items) # Output: [] - Correct behavior
Attention to such details can greatly enhance the reliability of your code. Additionally, it’s important to be mindful of the visibility of instance variables. Python uses a convention of prefixing instance variables with an underscore to indicate that they are intended for internal use. While this is not enforced, it signals to other developers that they should avoid accessing these variables directly. Here’s how you might implement this:
class BankAccount: def __init__(self, account_number, balance=0): self._account_number = account_number # Indicate that's internal self._balance = balance def deposit(self, amount): self._balance += amount def get_balance(self): return self._balance account = BankAccount("12345678") account.deposit(100) print(account.get_balance()) # Output: 100
By following these best practices, such as ensuring proper use of ‘self’, avoiding mutable default arguments, and using naming conventions for variable visibility, you can write clearer, more maintainable, and robust Python code. Remember, the clarity and integrity of your class design ultimately lead to more reliable software solutions.