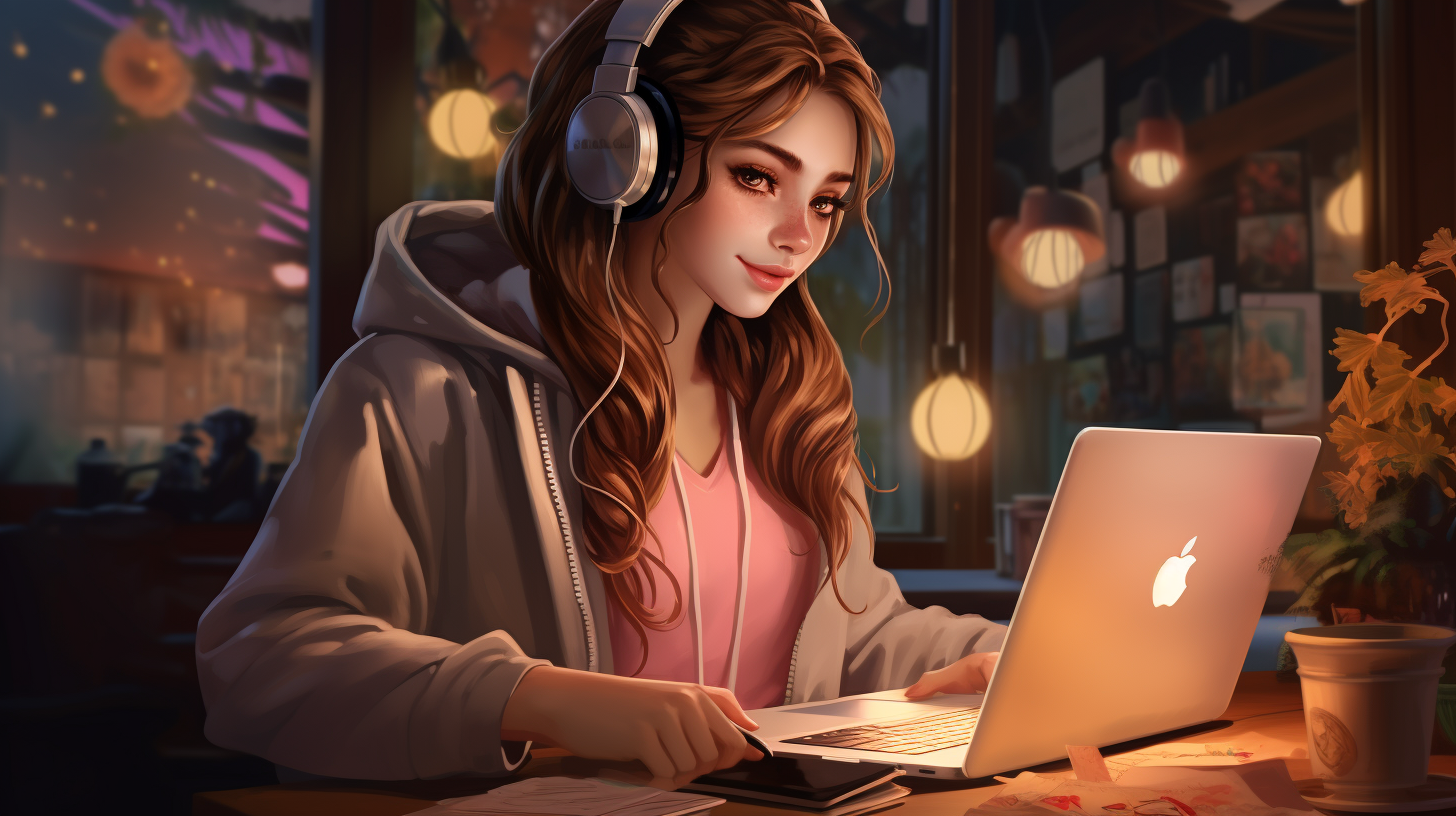
Using Bash for Image Processing
Image processing has evolved into a cornerstone of modern digital media applications, yet many developers overlook the power of command-line interfaces. Bash, often considered a mere shell for executing commands, harbors the potential to perform robust image processing tasks efficiently. Using Bash for image manipulation allows for automation and scripting, transforming tedious manual processes into streamlined workflows.
At its core, image processing in Bash hinges on the ability to interact with external tools that execute the heavy lifting. The command line provides a level of flexibility that GUI-based applications often lack. By calling upon a suite of dedicated tools, you can leverage Bash to manipulate images in various ways: resizing, converting formats, compressing, filtering, and even performing complex transformations.
The beauty of using Bash lies in its capability to handle images in bulk through scripting. This is particularly advantageous when dealing with large datasets, such as when processing photographs for a web application or preparing training datasets for machine learning algorithms. With a few simple commands, you can apply transformations to hundreds or thousands of images, saving both time and effort.
To get started, you might want to familiarize yourself with some of the popular command-line tools available for image processing. Tools like ImageMagick and GraphicsMagick provide a powerful set of features that can be accessed from within Bash scripts. These libraries not only support a wide range of image formats but also offer extensive capabilities for transformation and analysis.
For example, if you want to convert an image from PNG to JPEG format using ImageMagick, you can do so with a simple command:
convert input.png output.jpg
This command illustrates the simpler nature of image manipulation in Bash. Beyond simple conversions, you can apply filters, resize images, and even create montages with ease.
The potential of Bash in image processing is vast and largely untapped. By embracing the command line, you not only gain efficiency but also the ability to script complex workflows that can save time and reduce errors in your image processing tasks.
Essential Tools and Libraries
When delving into the essential tools and libraries for image processing in Bash, the first name that comes to mind is ImageMagick. This powerful suite of command-line tools is designed specifically for image manipulation and supports a staggering array of formats, from common ones like JPEG and PNG to more obscure types. With ImageMagick, you can perform a multitude of tasks ranging from basic operations to advanced transformations.
Another noteworthy contender is GraphicsMagick, which originated as a fork of ImageMagick. It’s optimized for performance and is particularly well-suited for batch processing large numbers of images. While it retains much of the functionality of ImageMagick, users often find GraphicsMagick to be faster and more memory-efficient, making it an attractive choice for those working with high volumes of image data.
In addition to these two giants, other tools like ExifTool can be invaluable for handling metadata in image files. ExifTool allows you to read and write metadata, which can be crucial for organizing and managing large photo collections. It seamlessly integrates with Bash, allowing you to automate tasks related to metadata extraction and modification.
For users interested in more specialized tasks, FFmpeg is a powerful tool for video and audio manipulation that also includes functionalities for image processing. With FFmpeg, you can extract frames from videos, convert image sequences to video, and perform a variety of transformations on individual image files.
Here’s a simple command using ImageMagick to resize an image while maintaining its aspect ratio:
convert input.jpg -resize 800x600 output.jpg
If you need to create a thumbnail from an image, you might use the following command:
convert input.jpg -thumbnail 150x150 thumbnail.jpg
For batch processing, suppose you want to convert all PNG files in a directory to JPEG. You can achieve this succinctly with a loop in Bash:
for file in *.png; do convert "$file" "${file%.png}.jpg" done
This snippet illustrates the efficiency of Bash combined with ImageMagick: iterate through all PNG files and convert them to JPEG format without manual intervention. The use of string manipulation ensures that the new file retains the original name, only changing the extension.
By using these tools, you empower your image processing capabilities in Bash, allowing for unprecedented efficiency and control over your digital assets. The essential libraries available not only simplify the syntax of complex tasks but also make it feasible to automate processes that would otherwise consume significant time and effort.
Basic Image Manipulations
Basic image manipulations are foundational to using the full potential of Bash for image processing. Once you have your essential tools installed, you can start performing a variety of simpler, yet powerful operations. These manipulations can range from simple format conversions to more complex transformations that adjust the appearance or quality of the images.
One of the most common tasks is resizing images. This is particularly useful for preparing images for web use, where file size and dimensions can significantly impact load times and user experience. Using ImageMagick, you can resize an image while maintaining its aspect ratio with the following command:
convert input.jpg -resize 800x600 output.jpg
In this command, the dimensions specified ensure that the output image will fit within a box of 800×600 pixels, but it will not exceed those dimensions, thus preserving the original aspect ratio.
Another common manipulation is cropping, which allows you to focus on a specific part of an image. This can be particularly useful for creating highlights or removing unwanted sections. The following command illustrates how to crop an image:
convert input.jpg -crop 200x200+50+50 output.jpg
In this example, the image is cropped to a 200×200 pixel area starting from the coordinates (50,50). This allows you to precisely select which part of the original image you wish to keep.
Applying filters and effects can also add creative flair to your images. For instance, if you want to apply a blur effect, you can use:
convert input.jpg -blur 0x8 output.jpg
This command applies a Gaussian blur with a radius of 0 and a sigma of 8, softening the image dramatically.
Additionally, adjusting color properties can enhance your images or prepare them for specific applications. To convert an image to grayscale, you can use:
convert input.jpg -colorspace Gray output.jpg
This command transforms the original image into shades of gray, which can be useful for printing or for stylistic effects.
Moreover, manipulating image quality is another vital aspect of image processing. You can compress JPEG images to reduce file size with the following command:
convert input.jpg -quality 75 output.jpg
Here, the quality parameter adjusts the compression rate, lowering the quality to 75% of the original. That is a trade-off between image fidelity and file size, which very important for web applications where bandwidth may be a concern.
For batch operations, you might want to apply these manipulations to multiple images simultaneously. Using a loop, you could resize all images in a directory to a specific size:
for file in *.jpg; do convert "$file" -resize 800x600 "resized_$file" done
In this loop, each JPEG file in the current directory is resized and saved with a “resized_” prefix, ensuring the original files remain unchanged. This approach highlights the power of Bash scripting in automating repetitive tasks.
By mastering these basic image manipulations in Bash, you can streamline your workflows and enhance your productivity in image processing tasks. The command line becomes a powerful ally, allowing you to perform complex operations with a few concise commands, ensuring you spend less time on mundane tasks and more time on creative endeavors.
Automating Batch Processing
Automating batch processing in image manipulation with Bash opens the door to a realm of efficiency that can be critically beneficial, especially when dealing with large collections of images. Instead of manually editing each file, Bash allows you to script a series of operations that can be executed on multiple files concurrently. This not only saves time but also minimizes the risk of human error that can occur during repetitive tasks.
One key advantage of using Bash for batch processing is its ability to handle loops and conditionals. This means you can easily create scripts that can adapt to different situations and requirements. For instance, if you need to convert all images in a directory from one format to another, you can write a concise script that processes each file in a loop. That is particularly useful when working with formats that may not be compatible with your intended use.
for file in *.png; do convert "$file" "${file%.png}.jpg" done
This loop takes all PNG files in the current directory and converts them to JPEG format. The use of parameter expansion “${file%.png}.jpg” ensures that the new file retains the original name while changing its extension, allowing for easy identification and organization.
Batch processing can also extend to more intricate tasks, such as resizing multiple images. Ponder a scenario where you need to resize all images to a specific dimension for a web project. You can accomplish this with a simple script as follows:
for image in *.jpg; do convert "$image" -resize 800x600 "resized_$image" done
In this example, every JPG image in the current directory is resized to fit within a box of 800×600 pixels, and each resized image is prefixed with “resized_”. This not only automates the resizing process but also preserves the original images, which very important for maintaining a backup.
Another powerful feature of batch processing in Bash is the ability to incorporate conditionals to manage file types or other properties. Suppose you only want to apply a specific transformation to images larger than a certain size. You can leverage the `identify` command from ImageMagick, which provides information about an image, to check the file size before processing it:
for img in *; do if [ $(identify -format "%[fx:width*height]" "$img") -gt 1000000 ]; then convert "$img" -resize 800x600 "resized_$img" fi done
This script checks if the width multiplied by the height of each image exceeds 1,000,000 pixels before resizing it. This level of control ensures that you’re only processing images that meet your criteria, optimizing both performance and output quality.
Furthermore, you can chain multiple operations within a single batch process to ensure that each image undergoes a series of transformations in one go. For example, you could resize, convert formats, and apply a filter all in one loop:
for img in *.png; do convert "$img" -resize 800x600 -blur 0x8 "${img%.png}.jpg" done
This command not only resizes the image but also applies a blur effect before converting it to JPEG format. The combination of these operations demonstrates the power of Bash scripting in streamlining complex workflows.
Finally, it is essential to ponder error handling when automating batch processes. Incorporating checks can prevent your script from failing silently. For instance, you can add a simple check to ensure that the file is indeed an image before attempting to process it:
for img in *; do if [[ "$img" == *.png || "$img" == *.jpg ]]; then convert "$img" -resize 800x600 "${img%.png}.jpg" fi done
This script ensures that only files with the specified extensions are processed, enhancing reliability and reducing the chances of runtime errors.
By embracing the automation capabilities of Bash for batch processing in image manipulation, you can significantly enhance your productivity. The ability to handle multiple images with a few lines of code not only makes your workflow more efficient but also empowers you to focus on more creative aspects of your projects, leaving the grunt work to the power of automation.
Advanced Techniques: Scripting Complex Tasks
When it comes to scripting complex tasks in image processing with Bash, the potential is truly remarkable. By using the power of command-line tools and the flexibility of Bash scripting, you can create intricate workflows that can manipulate images in ways that are often far beyond the capabilities of traditional GUI applications. This section delves into advanced techniques that allow you to automate and optimize your image processing tasks through sophisticated scripting.
One of the cornerstones of scripting complex tasks is the ability to combine multiple commands and process images based on various criteria. For instance, you can create a script that not only converts image formats but also applies a series of transformations based on user-defined parameters. Here’s a conceptual example where we convert images, resize them, and apply a watermark, all within a single script:
#!/bin/bash # Define watermark image WATERMARK="watermark.png" # Loop through all images in the current directory for img in *.jpg; do # Define output file name output="processed_${img%.jpg}.png" # Convert, resize, and add watermark convert "$img" -resize 800x600 -gravity southeast -composite "$WATERMARK" "$output" done
In this script, we use the `convert` command to not only resize the image but also apply a watermark positioned at the southeast corner. The flexibility of Bash enables us to easily modify parameters or outputs, allowing for a highly customizable image processing workflow.
Another powerful technique is to utilize functions within your Bash scripts. This modular approach allows you to define reusable pieces of code that can be called multiple times with different arguments. For example, a function to process images could look like this:
process_image() { local img="$1" local output="$2" convert "$img" -resize 800x600 -colorspace Gray "$output" } # Loop through images and call the function for img in *.jpg; do process_image "$img" "grayscale_${img%.jpg}.png" done
Here, we define a function called `process_image`, which takes an input image and an output filename as arguments. This design allows for cleaner and more maintainable code, as the image processing logic is encapsulated within the function. By calling this function in a loop, we can easily apply the same processing to a wide array of images.
Incorporating error handling into your scripts is also crucial when dealing with complex tasks. By checking for the existence of files or validating image types prior to processing, you can make your scripts more robust. For instance, you could include checks to ensure that a file is an image before attempting to process it:
for img in *; do if [[ "$img" == *.jpg || "$img" == *.png ]]; then process_image "$img" "processed_${img}" else echo "Skipping non-image file: $img" fi done
This example demonstrates how to filter files effectively, ensuring that only valid image files are passed to your processing function. Such checks help prevent runtime errors and ensure that your workflow proceeds smoothly.
Moreover, you can enhance your scripts by incorporating user input for parameter customization. For example, you might want to allow users to specify the resize dimensions or the watermark image at runtime:
read -p "Enter desired width: " WIDTH read -p "Enter desired height: " HEIGHT read -p "Enter watermark image path: " WATERMARK for img in *.jpg; do output="watermarked_${img%.jpg}.png" convert "$img" -resize "${WIDTH}x${HEIGHT}" -gravity southeast -composite "$WATERMARK" "$output" done
In this scenario, user input allows for greater flexibility, catering to specific project needs without requiring modifications to the script itself. Bash’s ability to read user input dynamically enhances the interactivity and usability of your image processing scripts.
As you explore further into advanced techniques, think integrating additional tools like ExifTool for metadata handling or FFmpeg for video frame extraction. By combining these tools with your Bash scripts, you can create comprehensive solutions that address a wide range of image processing requirements. The possibilities are vast, limited only by your imagination and the power of scripting.
Employing these advanced scripting techniques, you can not only automate repetitive tasks but also create sophisticated image processing workflows that are tailored to your specific needs. The command line becomes a potent instrument, allowing for efficiency and precision in manipulating images, all while empowering you to tackle complex tasks with elegance and ease.
Case Studies and Practical Examples
Within the scope of image processing, practical examples serve as an important bridge between theory and real-world application. Understanding how to apply Bash scripting to solve tangible problems can significantly enhance your proficiency and deepen your appreciation for the command line’s capabilities. Below, we explore several case studies that illustrate the power of Bash in various image processing scenarios.
One common challenge faced by photographers and web developers alike is the need to prepare images for online use. Ponder a scenario where you have a directory filled with high-resolution images that need to be resized and optimized for web display. Using Bash and ImageMagick, you can automate this task effectively. For instance, you might write a script to resize all images to a maximum width of 800 pixels while maintaining their aspect ratios:
for img in *.jpg; do convert "$img" -resize 800x800 "$img" done
This script iterates over each JPG file in the directory, applying the resize operation directly to the original files. The result is a collection of web-ready images, drastically reducing file sizes without compromising on quality.
Another practical example arises when dealing with the need to batch watermark a set of images. Watermarking is vital for photographers wishing to protect their intellectual property. Here’s how you might automate the process of adding a watermark to each image in a directory:
WATERMARK="watermark.png" for img in *.jpg; do convert "$img" -gravity southeast -composite "$WATERMARK" "watermarked_$img" done
In this script, each image has a watermark applied to the southeast corner, and the new files are prefixed with “watermarked_”. The gravity option ensures the watermark is consistently positioned, offering a professional look to your images.
Furthermore, let’s ponder a case where you need to organize images based on their dimensions. If you receive a batch of images with varying sizes and want to sort them into folders based on their width, you can achieve this with a simple Bash script:
mkdir -p small medium large for img in *.jpg; do WIDTH=$(identify -format "%w" "$img") if [ "$WIDTH" -lt 500 ]; then mv "$img" small/ elif [ "$WIDTH" -lt 1000 ]; then mv "$img" medium/ else mv "$img" large/ fi done
This example uses the `identify` command to extract the width of each image and moves the files into corresponding directories based on their dimensions. Such organization not only helps manage large libraries but makes it easier to find images suitable for specific applications.
Additionally, when preparing images for machine learning datasets, you may require images to be standardized not only in size but also in format. Consider the following script that converts all images in a directory to PNG format and resizes them in one pass:
for img in *.jpg; do convert "$img" -resize 256x256 "${img%.jpg}.png" done
This script ensures that each JPEG image is converted into PNG format while being resized to a uniform 256×256 pixels, which is often a requirement for many machine learning models.
In the sphere of video processing, FFmpeg can also be integrated for tasks such as extracting frames from a video file. If you want to capture every tenth frame from a video, the following script demonstrates how to achieve this:
ffmpeg -i video.mp4 -vf "select=not(mod(n,10))" -vsync vfr frame_%04d.png
This command extracts every tenth frame from “video.mp4” and saves it as a PNG file, illustrating the synergy between video and image processing capabilities within the Bash environment.
These case studies exemplify just a few of the many scenarios where Bash scripting can simplify and automate image processing tasks. The approach not only enhances efficiency but also empowers users to handle complex operations with ease, reinforcing the command line’s invaluable role in modern digital workflows.