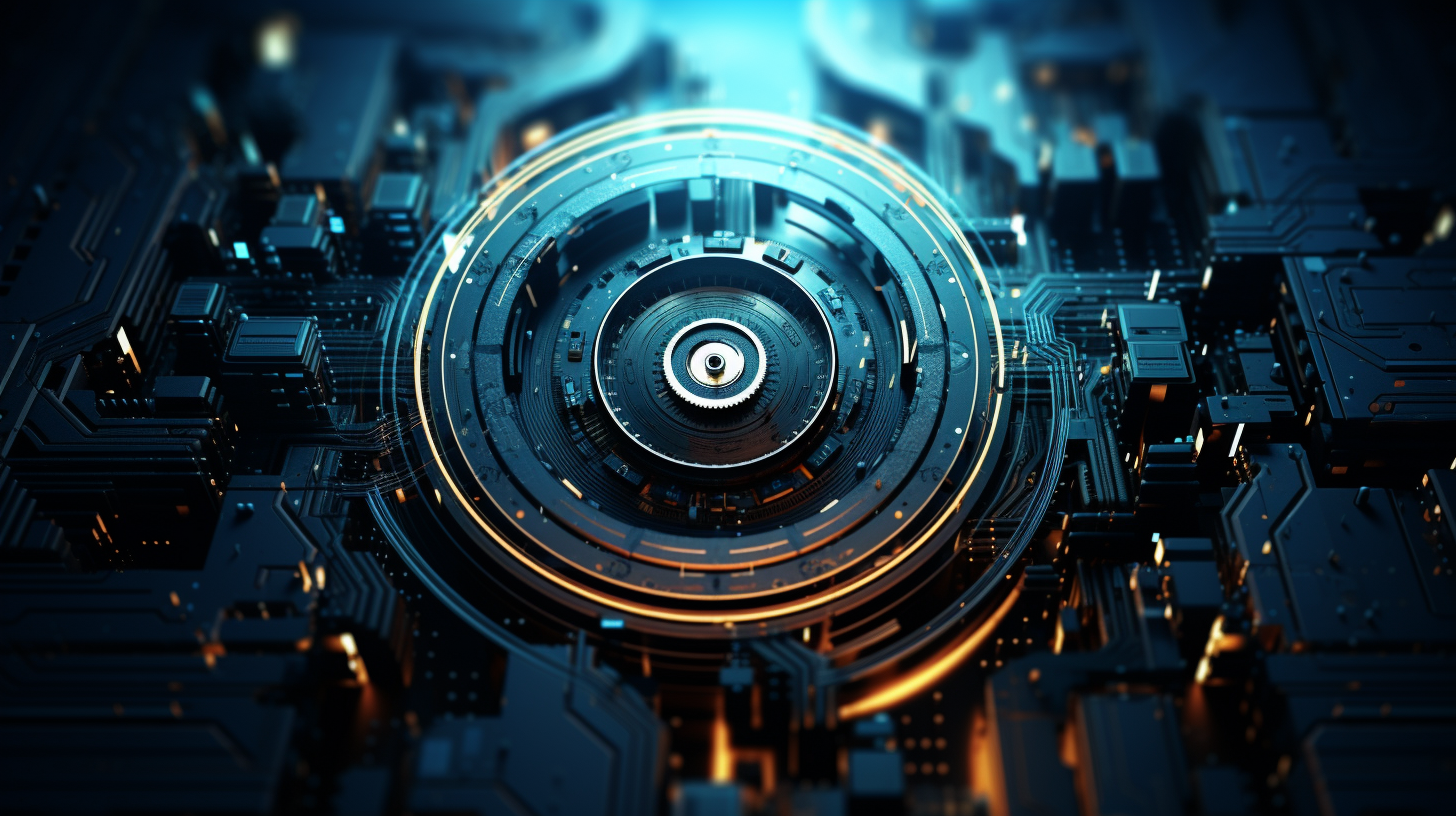
Using Bash in IoT Devices
Bash, or the Bourne Again SHell, serves as a powerful tool for developers working with Internet of Things (IoT) devices. Its lightweight nature and flexibility make it an ideal choice for various tasks that one might encounter in an IoT environment. When you ponder about the array of devices connected in an IoT landscape—from sensors to smart appliances—Bash emerges as a linchpin that facilitates communication, configuration, and management.
One of the most significant roles of Bash in IoT development is its ability to streamline workflows. Developers can write scripts to automate repetitive tasks, reducing the time spent on manual configurations and updates. This automation is critical in IoT, where devices often operate in remote locations with limited human intervention.
Moreover, Bash provides a simpler interface to interact with hardware and software components of IoT devices. Through native commands and scripting capabilities, developers can access system resources, manage device states, and handle data streams with ease. That’s particularly useful when dealing with a high number of devices, as Bash scripts can be deployed to manage multiple units concurrently.
For instance, monitoring the status of various sensors can be efficiently achieved using Bash. You can write a simple script that periodically checks the operational status of connected devices and logs the results:
#!/bin/bash for device in /dev/sensor*; do if [ -e "$device" ]; then echo "$device is operational" >> /var/log/iot_device_status.log else echo "$device is not responding" >> /var/log/iot_device_status.log fi done
In addition, the role of Bash extends to data handling and preprocessing. When IoT devices generate data, it often needs to be filtered, formatted, or transformed before analysis or storage. Bash excels in this area, allowing developers to pipe data between commands and employ various text processing tools like awk
, sed
, and grep
directly from the command line or within scripts.
Here’s a simple example of using Bash to filter out specific readings from a data log:
cat /var/log/iot_data.log | grep "temperature" | awk '{print $2, $3}'
Furthermore, the ability to interface with other programming languages and utilities makes Bash a versatile option in the IoT toolkit. Whether it’s invoking Python scripts for more complex data processing or using curl to send data to a cloud service, Bash acts as a bridge between various functionalities.
Bash’s role in IoT development cannot be overstated. Its scripting capabilities, ease of use, and ability to integrate with other tools and languages make it an invaluable asset for managing the complexities of IoT systems, allowing developers to focus on innovation rather than on the minutiae of device management.
Setting Up a Bash Environment on IoT Devices
Setting up a Bash environment on IoT devices is a critical first step in using the power of the shell for development and management tasks. Given the diversity of IoT devices, from Raspberry Pi to Arduino boards, the setup process can vary. However, the essence remains the same: ensuring that Bash is properly installed and configured to meet the needs of your specific hardware.
Most Linux-based IoT devices come with Bash pre-installed, but it’s always a good practice to verify the installation and ensure you’re using the latest version for optimal performance. To check if Bash is installed and to determine the version, you can run the following command:
bash --version
If you find that Bash is not installed, or if you wish to upgrade, you can typically install it using the device’s package manager. For instance, on a Debian-based system (like Raspbian for Raspberry Pi), you can execute:
sudo apt-get update sudo apt-get install bash
Once you have confirmed the installation, the next step involves configuring your Bash environment. This includes setting up the environment variables, customizing the prompt, and creating an efficient directory structure for your scripts. Environment variables can be set in the `.bashrc` file located in your home directory. For example, you might want to set a custom path for your scripts:
echo 'export PATH=$PATH:~/my_scripts' >> ~/.bashrc source ~/.bashrc
Customizing your prompt can also enhance your productivity, making it easier to identify your command line context. This can be done by modifying the `PS1` variable in your `.bashrc`. A simple tweak could be to add the current directory to your prompt:
export PS1="u@h:w$ "
Another important aspect of setting up Bash on IoT devices is ensuring that your scripts have the necessary permissions to execute. That’s important for automating tasks and running scripts without permission issues. After creating a script, you can make it executable with the following command:
chmod +x my_script.sh
Beyond basic setup, you might also want to establish a project structure to keep your scripts organized. A simpler approach could be to create directories for different functionalities such as logging, monitoring, and configuration:
mkdir -p ~/iot_project/{logs,monitoring,configuration}
By establishing a structured environment for your Bash scripts, you can significantly improve the efficiency and maintainability of your IoT applications. Setting up Bash correctly not only empowers developers to utilize powerful command-line capabilities but also lays the groundwork for scalable and automated IoT solutions.
Common Bash Commands for IoT Applications
In the context of IoT, the command line can often feel like a hidden gem, and mastering common Bash commands is akin to unlocking an entire toolbox filled with utilities that can streamline operations. Each command is a building block in the architecture of IoT management, offering functionality this is both simple to use and incredibly powerful when combined.
To start, one of the most fundamental commands you’ll encounter is ls, which lists files and directories. This command is essential for navigating the filesystem of your IoT device, enabling you to quickly assess the contents of directories and locate scripts or logs you may need to interact with.
ls -l /path/to/directory
The cd command is equally crucial, so that you can change directories. For instance, if you’re working within your project directory, you can efficiently move around the filesystem to access different components:
cd ~/iot_project/logs
Another highly useful command is cat, which can be employed to display the contents of files directly in the terminal. That is particularly handy for reviewing configuration files or log outputs:
cat /var/log/iot_device_status.log
When managing multiple devices or sensors, the ping command becomes invaluable for checking connectivity. With its ability to test the reachability of a host on an IP network, it can confirm whether your devices are online:
ping -c 4 192.168.1.100
In scenarios where you need to monitor resource usage, commands like top and htop provide a dynamic real-time view of system processes, CPU usage, and memory consumption. That’s essential for ensuring that your IoT devices are operating efficiently:
top
To manage files, the cp, mv, and rm commands are indispensable. They allow you to copy, move, and remove files and directories, respectively. For instance, to back up a configuration file, you might use:
cp /etc/my_config.conf ~/backup/my_config.conf
Moreover, the ability to manipulate text is important in IoT development. Commands like grep, awk, and sed empower you to filter and process data streams effectively. For example, to extract specific entries from a log file, you can use:
grep "error" /var/log/iot_data.log
When it comes to network operations, the curl command shines by which will allow you to send and receive data from networked resources. That’s particularly useful for API interactions, such as posting sensor data to a cloud service:
curl -X POST -d "temperature=22.5" http://api.example.com/sensors
Lastly, the power of Bash scripting comes into play when you need to automate these commands. By encapsulating a series of commands into a single script, you can execute complex workflows with a simple call. For instance, the following script checks the status of your sensors and logs the results:
#!/bin/bash
for sensor in /dev/sensor*; do
if [ -e "$sensor" ]; then
echo "$(date): $sensor is operational" >> /var/log/sensor_status.log
else
echo "$(date): $sensor is not responding" >> /var/log/sensor_status.log
fi
done
By mastering such common Bash commands, IoT developers can create efficient, reliable systems that perform their required tasks seamlessly. The simplicity of these commands belies their power, enabling developers to weave together complex operational tapestries from simpler threads.
Automating Tasks with Bash Scripts in IoT
Bash scripts serve as the backbone of automation in IoT environments, allowing developers to create robust solutions that can perform tasks without manual intervention. From monitoring devices to updating configurations, the ability to automate processes very important in a landscape where efficiency and reliability are paramount. The beauty of Bash lies in its simplicity, enabling developers to write scripts that can execute a series of commands with minimal fuss. Ponder, for example, a scenario in which you need to routinely update the firmware of multiple IoT devices. Instead of manually logging into each device, you can write a script that automates the update process.
#!/bin/bash # Script to update firmware on multiple IoT devices devices=("192.168.1.101" "192.168.1.102" "192.168.1.103") firmware_path="/path/to/firmware.bin" for device in "${devices[@]}"; do echo "Updating firmware on $device..." ssh user@$device "sudo cp $firmware_path /usr/local/bin/firmware && sudo systemctl restart iot_service" echo "Firmware updated on $device." done
This script demonstrates how you can loop through an array of device IP addresses, connect to each device via SSH, and execute commands to copy the firmware file and restart the respective service. The automation not only saves time but also reduces the risk of human error, ensuring that each device receives the correct update.
Another powerful use of Bash scripting is in data collection and analysis. In an IoT setting, devices churn out vast amounts of data that need to be collected, processed, and stored for analysis. A Bash script can automate the data retrieval process, constantly polling devices for data and logging the results.
#!/bin/bash # Script to collect data from IoT sensors log_file="/var/log/iot_sensor_data.log" while true; do for sensor in /dev/sensor*; do if [ -e "$sensor" ]; then data=$(cat $sensor) echo "$(date) - Sensor $sensor: $data" >> $log_file fi done sleep 60 # Wait for 60 seconds before the next collection done
This script continuously collects data from connected sensors, logs it with a timestamp into a designated file, and pauses for a minute before the next collection cycle. Such automation allows for continuous monitoring without requiring constant oversight.
Moreover, the use of cron jobs in conjunction with Bash scripts can elevate automation to even greater heights. By scheduling scripts to run at specific intervals, you can ensure that critical tasks occur without fail. For instance, if you want to automate the data collection script above to run every hour, you can add a cron job like this:
0 * * * * /path/to/your_script.sh
This line in your crontab ensures the script runs at the start of every hour, so that you can maintain a constant flow of data into your logs.
Incorporating error handling into your Bash scripts further enhances their reliability. By checking the exit status of commands and implementing conditional logic, you can ensure that your automation scripts behave intelligently, responding to failures gracefully and logging errors for further analysis.
#!/bin/bash # Script to safely update firmware with error handling devices=("192.168.1.101" "192.168.1.102") firmware_path="/path/to/firmware.bin" for device in "${devices[@]}"; do echo "Updating firmware on $device..." if ssh user@$device "sudo cp $firmware_path /usr/local/bin/firmware"; then echo "Firmware updated on $device." ssh user@$device "sudo systemctl restart iot_service" else echo "Failed to update firmware on $device. Check the connection or the firmware path." >> /var/log/firmware_update_errors.log fi done
In this example, the script checks if the firmware update command was successful before attempting to restart the service. If it fails, an error message is logged, making troubleshooting more manageable.
Embracing the power of Bash scripts in IoT has the potential to significantly enhance operational efficiency. They allow developers to automate intricate workflows, reducing manual oversight and the potential for errors while promoting a more streamlined and effective management of IoT resources. With every script, you not only save time but also gain the ability to harness the full power of your devices, ultimately driving innovation in the ever-evolving IoT landscape.
Managing IoT Device Resources with Bash
Bash provides a fine-grained control over the management of resources in IoT devices, enabling developers to efficiently allocate, monitor, and optimize the use of hardware and software resources. One of the primary tasks in managing resources is keeping an eye on system performance metrics, such as CPU load, memory usage, and disk space. These metrics help developers make informed decisions about resource allocation and identify potential bottlenecks.
To gather system metrics, you can utilize commands like top
or vmstat
. The top
command provides a real-time view of active processes, displaying CPU and memory usage, which is essential when you’re running multiple applications on an IoT device. For instance, you can run:
top
Similarly, vmstat
can be used to report on memory utilization and system performance at intervals. You might execute:
vmstat 5
This command will provide updates every 5 seconds, so that you can monitor the system’s performance over time.
Managing disk space is equally crucial, particularly when dealing with data-intensive IoT applications. The df
command offers insights into disk space usage across the mounted filesystems. To view the disk usage in a human-readable format, you can issue:
df -h
To further drill down into disk usage, the du
command helps identify how much space specific directories and files are occupying. For example, to find out the space used by your project directory, you could run:
du -sh ~/iot_project
Another important aspect of resource management in IoT is handling background processes and ensuring that they don’t consume excessive resources. Using commands like kill
or pkill
, you can terminate processes that are misbehaving or consuming too many resources. For instance, to kill a process by its ID, you would use:
kill 1234
If you want to kill all instances of a particular program, you can utilize:
pkill my_program
Moreover, Bash makes it easy to script the management of resources. For instance, you can write a script that checks for high memory usage and alerts you when certain thresholds are crossed. Here is a simple example:
#!/bin/bash # Check memory usage and alert if it exceeds 80% threshold=80 memory_usage=$(free | awk '/Mem/{printf("%.0f"), $3/$2*100}') if [ "$memory_usage" -gt "$threshold" ]; then echo "Warning: Memory usage is at $memory_usage%!" | mail -s "Memory Alert" [email protected] fi
This script leverages the free
command to calculate memory usage, comparing it against a predefined threshold and sending an email if usage is too high. Such proactive monitoring helps in maintaining optimal performance in IoT systems.
In addition to monitoring, resource management also involves configuring and tuning the system for optimal performance. This tuning can include adjusting kernel parameters, configuring services to start on boot, and optimizing application performance. The sysctl
command can be used to modify kernel parameters at runtime. For example, to adjust the maximum number of open files, you could use:
sudo sysctl -w fs.file-max=100000
By incorporating these practices into your Bash workflows, you can enhance the manageability of IoT device resources, ultimately resulting in a more efficient and stable environment for your applications. The synergy of Bash scripting with system commands fosters a robust framework for resource management, paving the way for innovative IoT solutions.
Security Considerations for Bash in IoT Systems
Bash, with its powerful scripting capabilities, plays a pivotal role in addressing security considerations for IoT systems. As IoT devices proliferate and become increasingly interconnected, the potential attack surface expands, necessitating a strong focus on security measures. The lightweight and versatile nature of Bash can be harnessed to implement effective security practices while managing IoT devices.
One of the first steps in securing your Bash environment on IoT devices is to ensure that user permissions are properly configured. Limiting access to sensitive files and directories helps mitigate risks. By setting appropriate ownership and permission levels, you can control who can execute scripts and access important configurations. For instance, using the chmod command, you can make a script executable only by the owner:
chmod 700 my_secure_script.sh
This command ensures that only the script’s owner can read, write, or execute it, minimizing the chances of unauthorized access.
Another critical aspect of security is the management of sensitive information, such as API keys or configuration settings. Instead of hardcoding these values into scripts, consider using environment variables or encrypted files. You can set an environment variable in your Bash session as follows:
export API_KEY="your_api_key_here"
In your scripts, you can then reference this variable, keeping sensitive information out of your codebase. Moreover, for added security, you may want to explore tools like GnuPG for encrypting sensitive files:
gpg -c my_sensitive_config.conf
This command prompts you for a passphrase to encrypt the configuration file, making it accessible only to those with the correct passphrase.
Network security is equally important in the context of IoT devices. Ensuring that communications between devices and servers are secure can be accomplished by using SSH for secure connections. For SSH access, generate an SSH key pair and deploy the public key on your IoT devices:
ssh-keygen -t rsa -b 2048
Then, copy the public key to the IoT device:
ssh-copy-id user@iot_device
This method allows for secure, password-less authentication, reducing the risk of interception and unauthorized access.
Regular updates and patch management are fundamental to maintaining a secure IoT environment. Using a Bash script to automate the update process can ensure that your devices are always running the latest software versions:
#!/bin/bash # Update script for IoT devices echo "Updating package lists..." sudo apt-get update echo "Upgrading installed packages..." sudo apt-get upgrade -y echo "Cleaning up unnecessary packages..." sudo apt-get autoremove -y
This script checks for available updates and applies them, helping to close any vulnerabilities that could be exploited by attackers.
Finally, logging and monitoring activities on your IoT devices is essential for detecting unauthorized access or anomalous behavior. Using Bash scripts to create logs can help maintain an audit trail of operations. For example, you can log SSH login attempts:
#!/bin/bash # Log SSH attempts echo "$(date): SSH login attempt from $SSH_CLIENT" >> /var/log/ssh_attempts.log
By regularly reviewing these logs, you can quickly identify suspicious activities and respond accordingly.
Using Bash for security considerations in IoT environments involves a multifaceted approach. By managing permissions, securing sensitive information, using SSH for safe communications, automating updates, and maintaining detailed logs, developers can significantly enhance the security posture of their IoT devices. As IoT continues to evolve, implementing robust security practices becomes not just an option, but a necessity for safeguarding critical systems and data.