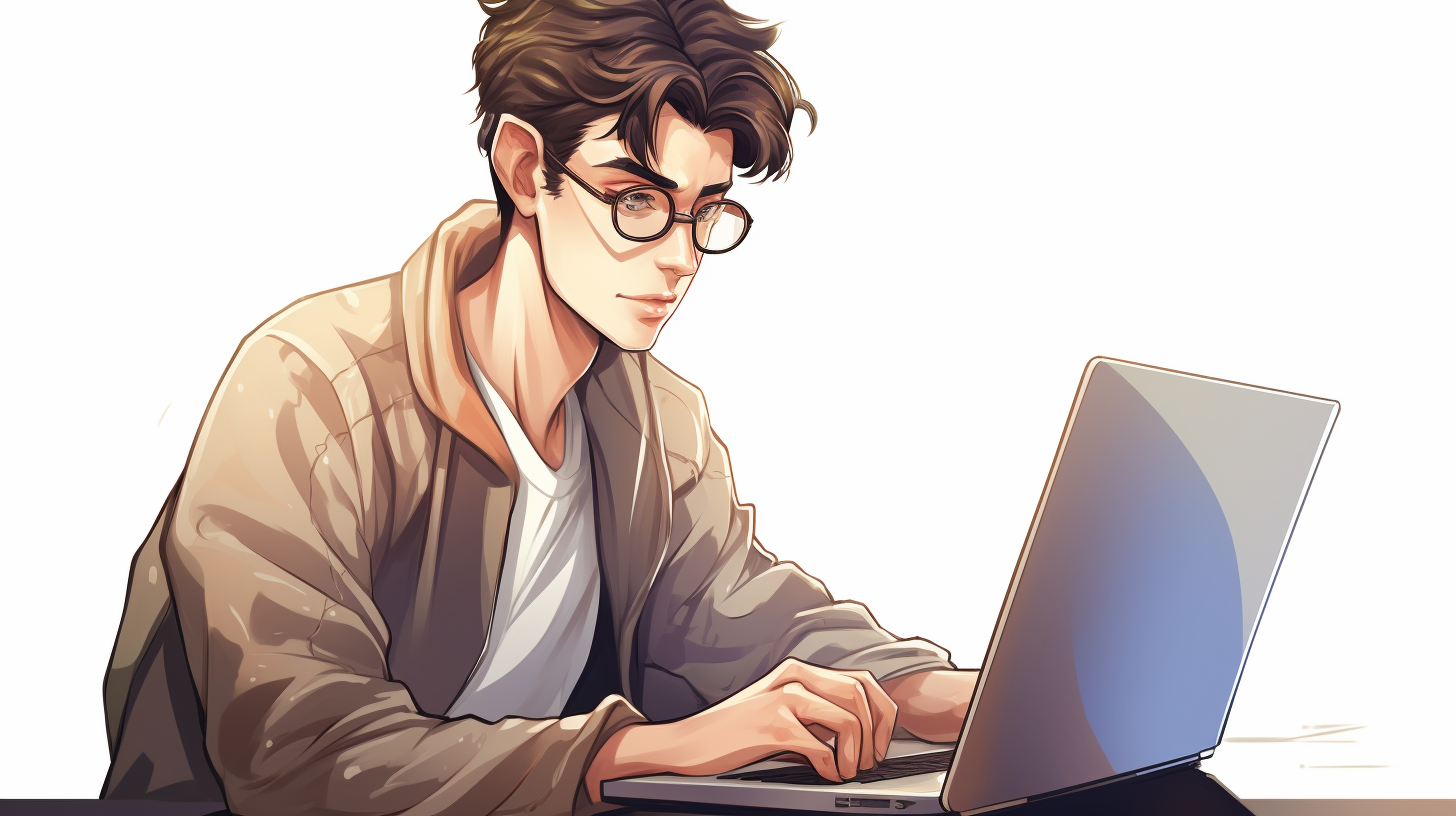
Using SQL for Duplicate Data Removal
Duplicate data in databases is a pervasive challenge that can lead to inefficiencies, inaccurate reporting, and increased storage costs. Understanding what constitutes duplicate data is essential for anyone working with relational databases. At its core, duplicate data occurs when identical records or entries exist within the same table or across multiple tables within a database.
To illustrate, think a table of customers where two entries exist for the same individual. This can happen when different data entry points lack validation checks, leading to the same customer being recorded multiple times, often with slight variations in the data such as name spelling or address details. The implications of such duplicates can severely distort analytics, reporting, and operational processes.
Identifying duplicate data can be simpler if the dataset is small, but as the volume of records increases, the task becomes more complex. SQL provides powerful tools for querying and managing data, including techniques to identify and handle duplicates effectively.
Generally, duplicate records can arise from several factors:
- Manual entry mistakes can lead to identical records being created inadvertently.
- When integrating data from different sources, duplicates may arise if the same entity is represented in both datasets.
- During data migrations, incomplete or improper handling can lead to duplicated entries.
- Automatic data import routines that lack primary key constraints can inadvertently introduce duplicates.
To better understand this concept, let’s look at a practical example involving a customers table. Think the following representation of duplicate data:
CREATE TABLE customers ( id INT PRIMARY KEY, name VARCHAR(100), email VARCHAR(100) ); INSERT INTO customers (id, name, email) VALUES (1, 'Neil Hamilton', '[email protected]'), (2, 'Jane Smith', '[email protected]'), (3, 'Nick Johnson', '[email protected]'); -- Duplicate entry
In this example, the entry for ‘Alex Stein’ exists twice, creating ambiguity in any operations targeting customer records. Understanding the nature and causes of duplicate data is the first step toward effective management and resolution, setting the stage for subsequent techniques that can be employed to identify and eliminate these redundancies.
Common Causes of Duplicate Data
When discussing the common causes of duplicate data, it is important to recognize that they can stem from various operational practices and human behaviors. Let’s delve into some of the key contributors that lead to the unfortunate proliferation of duplicate records in databases.
User error is perhaps the most simpler cause of duplicate data. When individuals manually input data, the chances of mistakes—such as misspellings, variations in naming conventions, or inconsistencies in formatting—can significantly increase the likelihood of duplicates. For instance, two entries for the same person may exist as “Alex Stein” and “Jon Doe” due to a typographical error during the data entry process. This can create challenges for querying and reporting, as the database treats these entries as distinct records.
Merging databases is another common scenario where duplicates arise. Organizations often combine data from various departments, systems, or external sources to create a unified dataset. If proper deduplication processes are not followed, multiple entries for the same entity can be retained in the merged dataset. This situation may occur, for example, if both the sales and support databases contain records for the same customer without a reconciliation process in place.
System migrations can also lead to duplicate records. When transitioning data from one system to another, improper handling of unique constraints or failure to enforce primary key integrity can result in duplicates. For instance, if records are dumped into a new database without adequate checks, you might end up with multiple entries for the same user if their data was split across different tables in the original system.
Data import processes are often automated, which can introduce duplicates if not carefully controlled. When importing external data, especially from sources that do not enforce primary key constraints, it is easy to unintentionally create duplicate entries. For example, an automated import script that pulls customer data from an external source could lead to the same customer being added multiple times if the script doesn’t check for existing records.
To show how these factors can manifest in a relational database, ponder the following example:
CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT, order_date DATE ); INSERT INTO orders (order_id, customer_id, order_date) VALUES (1, 1, '2023-01-01'), (2, 2, '2023-01-02'), (3, 1, '2023-01-01'), -- Duplicate order for the same customer (4, 3, '2023-01-03');
In this orders table, the same order has been recorded twice for ‘customer_id 1’, both on ‘2023-01-01’. This not only leads to inflated sales figures but can also create confusion in order fulfillment processes.
Understanding these common causes is critical for database administrators and data analysts alike, as it allows them to proactively implement measures to prevent duplicates from occurring in the first place, thereby ensuring more accurate data management and reporting.
Techniques for Identifying Duplicates
Identifying duplicates in a database especially important for maintaining data integrity and ensuring accurate reporting. SQL provides several techniques to help pinpoint duplicate entries. The goal is to filter through the records and isolate those that are identical based on specified criteria. Below are some effective methods to uncover duplicates.
One of the most simpler techniques involves using the GROUP BY clause combined with the HAVING clause. This allows you to group records based on the fields that define duplication and count how many times each group appears. For instance, in the customers table, you might want to find duplicates based on the name and email fields:
SELECT name, email, COUNT(*) as count FROM customers GROUP BY name, email HAVING COUNT(*) > 1;
This query groups the records by both the name and email columns and counts how many times each combination appears in the table. The HAVING COUNT(*) > 1 clause filters the results to show only those combinations that appear more than once, effectively identifying duplicates.
Another approach is to use the ROW_NUMBER() window function. This method is particularly useful when you want to retain one version of a record while marking all duplicates. By assigning a unique sequential integer to each row within a partition of a result set, you can easily filter out duplicates:
WITH RankedCustomers AS ( SELECT *, ROW_NUMBER() OVER (PARTITION BY name, email ORDER BY id) as row_num FROM customers ) SELECT * FROM RankedCustomers WHERE row_num > 1;
Here, the ROW_NUMBER() function is used to assign a unique number to each record within groups of duplicates (defined by the PARTITION BY clause). The result is that you can easily select records where row_num is greater than 1, giving you all duplicates except for the first occurrence.
For cases where the database structure involves multiple tables, you might need to join tables to identify duplicates across them. Let’s ponder a scenario where we want to find duplicate customer orders based on the customer_id:
SELECT o.customer_id, COUNT(*) as order_count FROM orders o JOIN customers c ON o.customer_id = c.id GROUP BY o.customer_id HAVING COUNT(*) > 1;
This query joins the orders and customers tables to count how many orders each customer has placed. The filter applied through the HAVING clause reveals any customers who have multiple orders.
Using these techniques, database administrators and analysts can effectively identify duplicate data, laying the groundwork for subsequent actions to clean and maintain the database. The precision of SQL empowers users to conduct thorough investigations into their data, ensuring that they can pinpoint discrepancies with minimal hassle.
Methods for Safely Removing Duplicates
Removing duplicates from a database is a sensitive operation that requires careful planning and execution to prevent unintended data loss. The goal is not only to eliminate redundant records but also to ensure that the integrity of the remaining data is maintained. Several methods can be employed to safely remove duplicates, and the choice of method often depends on the specific requirements of the database and the nature of the duplicates.
One of the safest methods involves using a CTE (Common Table Expression) along with the ROW_NUMBER() function, which allows you to identify and retain a single instance of a duplicate record while marking others for deletion. Let’s revisit the customers table example to illustrate this approach:
WITH RankedCustomers AS ( SELECT *, ROW_NUMBER() OVER (PARTITION BY name, email ORDER BY id) as row_num FROM customers ) DELETE FROM RankedCustomers WHERE row_num > 1;
In this query, we create a CTE named RankedCustomers that assigns a unique integer to each record within groups of duplicates, partitioned by the name and email fields. After identifying the duplicates, we then delete all records where the row number is greater than 1, effectively keeping only the first occurrence of each duplicate entry. This method is efficient and minimizes the risk of data loss since it allows you to explicitly specify which records to retain.
Another method involves using a temporary table to hold the unique records before removing the duplicates. This approach can serve as a backup, ensuring you have a copy of the original data should anything go awry during the deletion process. Here’s how you can implement this method:
CREATE TABLE temp_customers AS SELECT DISTINCT * FROM customers; TRUNCATE TABLE customers; INSERT INTO customers SELECT * FROM temp_customers;
In this case, we create a temporary table called temp_customers that contains only distinct records from the original customers table. After truncating the original table to remove all entries, we then insert the unique records back into the customers table. This method guarantees that only unique records remain, while also providing the flexibility to revert to the original data if necessary.
For scenarios where you need to remove duplicates based on specific criteria across multiple tables, an approach involving a DELETE statement with a JOIN can be effective. Think the following example where you want to remove duplicate orders for the same customer:
DELETE o1 FROM orders o1 INNER JOIN orders o2 ON o1.customer_id = o2.customer_id AND o1.order_id > o2.order_id;
In this query, we join the orders table to itself, comparing records with the same customer_id. The condition o1.order_id > o2.order_id ensures that we only delete the later entry for any duplicates. This method is particularly useful in maintaining relational integrity while eliminating redundancies.
It’s essential to always back up your data before performing deletion operations. This practice not only protects your data against accidental loss but also provides a safety net for recovery. Additionally, running these operations within a transaction can further safeguard against errors:
BEGIN TRANSACTION; -- Your deletion logic here COMMIT;
With these methods, database administrators can safely navigate the delicate task of removing duplicates, ensuring that the remaining data is both accurate and useful for business operations. Each method has its advantages, and understanding the context of your data will guide you in selecting the most appropriate approach for your specific needs.