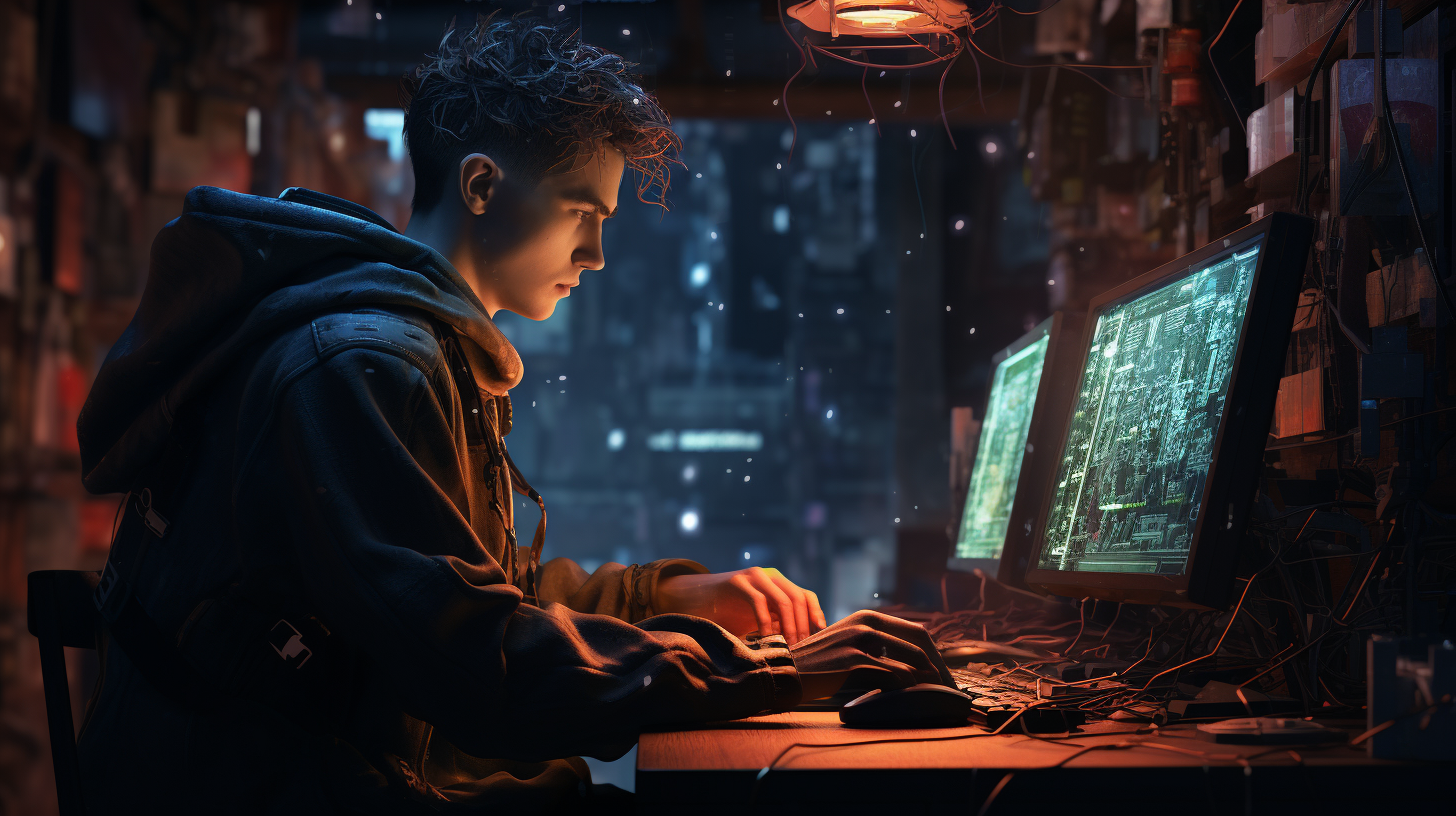
Using SQL Views for Simplified Data Representation
SQL views are virtual tables that provide a way to simplify complex queries and represent data in a more user-friendly format. They’re defined by a SQL query that pulls data from one or more underlying tables. Unlike physical tables, views do not store the data themselves; instead, they provide a dynamic representation of the data, which is updated in real-time as the underlying data changes.
One of the primary purposes of using SQL views is to encapsulate complex logic or data transformations that might otherwise clutter your main queries. By creating a view, you can abstract intricate joins, calculations, or filtering logic, making it easier for users to work with the data without needing to understand the underlying complexities.
Another significant aspect of views is that they enhance data security. By providing a view that only exposes certain columns or rows of data, you can restrict access to sensitive information while still allowing users to interact with the data they need. This idea of data abstraction is a cornerstone of good database design.
In addition to improving security and simplifying access, views also promote reuse of SQL code. Once a view is created, it can be referenced in multiple queries, thereby reducing redundancy and ensuring consistency across your data retrieval processes.
For instance, think a scenario where you have a table named Employee, which contains sensitive information such as salaries. You can create a view that excludes the salary column:
CREATE VIEW EmployeeView AS SELECT EmployeeID, FirstName, LastName, Department FROM Employee;
By using EmployeeView, users can access employee data without compromising sensitive salary information. This not only simplifies the data access process but also reinforces data governance practices.
SQL views serve multiple purposes: they simplify data representation, enhance security, and promote code reuse. Understanding these fundamental aspects of views is essential for using their full potential in your SQL database implementations.
Benefits of Using Views for Data Simplification
One of the most compelling benefits of using SQL views for data simplification is their ability to present a cohesive picture of data that’s often spread across multiple tables. This capability is particularly useful in environments where data normalization has led to an intricate web of related tables. By creating a view that consolidates relevant data, users can access a unified dataset without needing to understand the complexity of the underlying structure.
Views can also be optimized to deliver specific datasets tailored to the needs of varying user groups. For example, a sales team may need a view that highlights key performance indicators, whereas the finance team may require a more detailed view for analytical purposes. By defining different views for different roles, you can streamline workflows and minimize the risk of errors that occur when users attempt to write their own complex queries.
Another benefit of views is their ability to encapsulate frequently used queries, which leads to both efficiency and consistency. Consider the scenario where a company frequently queries sales data that requires several joins and aggregations. Instead of rewriting the same complex SQL each time, one can define a view:
CREATE VIEW SalesSummary AS SELECT s.SaleID, s.SaleDate, c.CustomerName, SUM(si.Quantity * si.UnitPrice) AS TotalSale FROM Sales s JOIN SalesItems si ON s.SaleID = si.SaleID JOIN Customers c ON s.CustomerID = c.CustomerID GROUP BY s.SaleID, s.SaleDate, c.CustomerName;
This view, SalesSummary
, allows users to retrieve summarized sales data with a simple SELECT
query:
SELECT * FROM SalesSummary;
Such a simplification not only reduces the likelihood of errors but also enhances performance by caching the execution plan and improving readability. Users can focus on interpreting the results rather than grappling with the intricacies of the SQL syntax used to produce them.
Additionally, views can play an important role in data transformation. When dealing with data that requires consistent formatting or conversion, views can handle these transformations seamlessly. For example, if your database architecture includes a date stored in different formats across tables, you can create a view that unifies this representation:
CREATE VIEW UnifiedDateView AS SELECT EmployeeID, CAST(HireDate AS DATE) AS UniformHireDate FROM Employee;
This view ensures that whenever the hire date is accessed, it’s returned in a consistent format, thus reducing the potential for discrepancies in reporting and analysis.
The benefits of using SQL views for data simplification are manifold. They not only enable a more manageable and intuitive interaction with complex datasets but also boost productivity by allowing users to focus on analysis rather than data retrieval intricacies. As organizations continue to grapple with increasingly complex data landscapes, using views will remain a fundamental strategy for enhancing clarity and efficiency in data management.
Creating and Managing SQL Views
Creating SQL views is a simpler process that requires a solid understanding of the underlying data. To begin with, the CREATE VIEW statement is used to define a new view, and it typically includes a SELECT query that specifies which columns and rows from the underlying tables should be included in the view. The syntax for creating a view is as follows:
CREATE VIEW view_name AS SELECT column1, column2, ... FROM table_name WHERE condition;
When creating a view, it’s important to consider the data structure and how the view will be used. You can create views that join multiple tables, incorporate aggregations, or filter data to meet specific needs. For example, if you want to create a view that combines data from an Orders table and a Customers table, you might write:
CREATE VIEW CustomerOrders AS SELECT c.CustomerID, c.CustomerName, o.OrderID, o.OrderDate FROM Customers c JOIN Orders o ON c.CustomerID = o.CustomerID;
This view, CustomerOrders, enables users to easily access customer and order information without writing complex joins each time.
Managing SQL views involves various operations such as modifying, dropping, or updating them. To modify an existing view, you can use the CREATE OR REPLACE VIEW syntax, which allows you to redefine the view without having to drop it first. For instance:
CREATE OR REPLACE VIEW CustomerOrders AS SELECT c.CustomerID, c.CustomerName, o.OrderID, o.OrderDate, o.TotalAmount FROM Customers c JOIN Orders o ON c.CustomerID = o.CustomerID;
In this case, the updated view now includes a new column, TotalAmount, providing additional information to users.
To delete a view, the DROP VIEW statement is utilized. When you no longer need a view, it’s important to drop it to maintain database cleanliness:
DROP VIEW CustomerOrders;
Views can also be leveraged for data security and access control. By exposing only specific columns or rows, you can create views that restrict access to sensitive data. When defining a view, always think the principle of least privilege: only provide access to the data necessary for users to perform their tasks.
Furthermore, views can become outdated if the underlying data structure changes, such as when columns are added or removed from the base tables. Therefore, it’s crucial to regularly review and update views to ensure they remain relevant and functional. This is where the ALTER VIEW statement can come in handy, allowing for adjustments without needing to drop and recreate the view.
Creating and managing SQL views is an essential skill for database administrators and developers. By encapsulating complex queries and enhancing security, views facilitate smoother data interaction and improve overall efficiency in data retrieval processes.
Performance Considerations When Using Views
When considering the performance implications of SQL views, it’s crucial to recognize that while views abstract complexity and enhance usability, they can also introduce overhead depending on their design and usage. The performance of views largely depends on how they’re structured and how the underlying data is queried.
One of the key factors affecting view performance is whether the view is created from a simple query or a complex one involving multiple joins and aggregations. Simple views that pull data directly from a single table generally perform well. However, as the complexity of the view increases, with multiple joins and calculations, the database engine may need to execute the underlying SELECT statement each time the view is queried, which could lead to slower performance.
CREATE VIEW ComplexView AS SELECT a.Column1, b.Column2, SUM(c.Column3) AS Total FROM TableA a JOIN TableB b ON a.ID = b.AID JOIN TableC c ON b.ID = c.BID GROUP BY a.Column1, b.Column2;
In such cases, it’s important to monitor query execution plans to ensure optimal performance. The execution plan will reveal how the database engine processes the query, so that you can identify any bottlenecks or inefficient operations. Indexing the underlying tables properly can significantly enhance the performance of views, especially when they involve joins. Creating indexes on the columns used in JOIN and WHERE clauses can lead to faster data retrieval, as the database can locate the relevant rows more efficiently.
Another consideration is the use of materialized views, which store the query result physically. Unlike standard views, materialized views cache the results of the underlying query and can be refreshed periodically. They are particularly useful for complex queries that involve aggregations, as they eliminate the need to recompute results with every access. However, one must balance the benefits of speed against the overhead of maintaining the materialized view, especially in systems where the underlying data changes frequently.
CREATE MATERIALIZED VIEW SalesSummaryMaterialized AS SELECT s.SaleID, s.SaleDate, SUM(si.Quantity * si.UnitPrice) AS TotalSale FROM Sales s JOIN SalesItems si ON s.SaleID = si.SaleID GROUP BY s.SaleID, s.SaleDate;
When using views in transactional environments, be mindful not to create unnecessary views that could lead to complications in data consistency. If the base tables are frequently updated, the view may reflect stale data unless properly refreshed. Always ponder the read vs. write patterns of your application and design views that align with those patterns.
Another performance consideration relates to how views handle filtering and sorting. When you apply ORDER BY or WHERE clauses in a view, the database may not utilize indexes effectively. It’s often more efficient to apply these clauses at the query level rather than within the view definition itself, especially for large datasets. This optimizes the performance by allowing the database engine to leverage its indexing capabilities directly on the data.
SELECT * FROM ComplexView WHERE Total > 1000 ORDER BY SaleDate;
While SQL views provide a powerful abstraction for managing complexity in data retrieval, careful attention must be paid to their design and usage to ensure they do not become performance bottlenecks. Regular analysis of query performance, proper indexing, and considering materialized views for complex aggregations can help maintain system efficiency while using the advantages that views offer.
Common Use Cases for SQL Views
SQL views are not merely tools for abstraction; they are also powerful constructs that can significantly enhance the usability and organization of data within a relational database. Their versatility allows them to be utilized in various scenarios, adapting to the diverse needs of different user groups, applications, or analytical tasks. Here are some common use cases where SQL views shine.
One simpler use case for views is encapsulating complex joins. In many databases, data is normalized across multiple tables, which can make direct queries cumbersome. For example, suppose we have an Orders table and a Customers table. Users frequently need to retrieve a combined view of this data for reporting purposes. Instead of writing repetitive SQL joins, you can create a view that simplifies this task:
CREATE VIEW OrderDetails AS SELECT o.OrderID, o.OrderDate, c.CustomerName, c.Email FROM Orders o JOIN Customers c ON o.CustomerID = c.CustomerID;
This view, OrderDetails, allows users to access all necessary order and customer information with a simple query: SELECT * FROM OrderDetails;
. That is particularly useful in reporting applications where ease of access is paramount.
Another common scenario is data abstraction for security purposes. Organizations often need to comply with stringent data protection regulations, and using views to restrict access to sensitive data is a best practice. For instance, ponder a table containing employee records, including sensitive information like salaries. You can create a view that excludes the salary column:
CREATE VIEW EmployeePublicInfo AS SELECT EmployeeID, FirstName, LastName, Department FROM Employees;
In doing so, you ensure that users can still access necessary employee information without exposing sensitive data, thus enhancing your database’s security posture.
Views are also invaluable for data transformation. A typical use case involves formatting or deriving new calculated fields. For instance, if you need to display product prices in a standardized currency, you might create a view that applies the necessary conversion:
CREATE VIEW ProductPrices AS SELECT ProductID, ProductName, Price * 1.1 AS PriceWithTax FROM Products;
This view provides a direct way to access product prices that include tax without altering the original data structure, keeping the database clean while ensuring consistent presentation of pricing information.
Moreover, views can serve as a foundation for implementing role-based access within applications. For example, different departments may require tailored views based on their specific data access needs. A sales team might need a view that highlights sales metrics and performance indicators:
CREATE VIEW SalesMetrics AS SELECT SalesRepID, SUM(SaleAmount) AS TotalSales, COUNT(OrderID) AS TotalOrders FROM Sales GROUP BY SalesRepID;
By creating specialized views like SalesMetrics, you empower teams with the data they require without overwhelming them with unnecessary information, thereby enhancing productivity and focus.
In analytical environments, views can simplify complex aggregations or calculations, making data readily accessible for reports or dashboards. For example, if your analytics team needs a monthly sales summary, you might create a view that aggregates sales data by month:
CREATE VIEW MonthlySalesSummary AS SELECT YEAR(SaleDate) AS SaleYear, MONTH(SaleDate) AS SaleMonth, SUM(SaleAmount) AS TotalSales FROM Sales GROUP BY YEAR(SaleDate), MONTH(SaleDate);
This view not only simplifies reporting for the analytics team but also ensures that they consistently retrieve aggregated data formatted for their specific reporting needs.
Another notable use case for views lies in providing a consolidated interface for reporting tools. Many business intelligence (BI) tools rely on views to generate dashboards and reports. By creating well-structured views, you can streamline the interaction between your database and BI tools, enhancing performance and usability.
SQL views serve a multitude of purposes across different scenarios—from encapsulating complex joins and securing sensitive information to transforming data into a more usable format. They empower users by simplifying data access and providing customized datasets tailored to various roles and needs, thereby promoting efficiency and clarity in data management.
Best Practices for Designing Effective Views
When designing effective SQL views, several best practices can help ensure that the views are not only useful but also performant and maintainable. Understanding how users interact with the data and the underlying structure of the database is essential for creating views that serve their intended purpose without introducing unnecessary complexity.
1. Keep it Simple: The primary goal of a view is to simplify access to data. Therefore, avoid making views overly complex. Limit the number of joins and aggregations; strive for clarity. If a view is too complicated, it may confuse users rather than help them. For example, a simple view can be created to provide customer details and their latest order:
CREATE VIEW LatestOrders AS SELECT c.CustomerID, c.CustomerName, o.OrderID, o.OrderDate FROM Customers c LEFT JOIN Orders o ON c.CustomerID = o.CustomerID WHERE o.OrderDate = (SELECT MAX(OrderDate) FROM Orders WHERE CustomerID = c.CustomerID);
2. Use Descriptive Naming: The name of the view should clearly indicate its purpose and the data it encapsulates. This practice enhances readability and aids maintenance. Instead of generic names like ‘View1’, use descriptive names like ‘CustomerOrderSummary’. It helps users to understand the purpose of the view at a glance.
3. Limit the Number of Columns: Only include columns that are necessary for your view. Including excess columns can lead to confusion and may impact performance. If users need additional data, think creating separate views tailored to different use cases. For instance, an employee view that only shows essential details might look like this:
CREATE VIEW EmployeeBasicInfo AS SELECT EmployeeID, FirstName, LastName, Department FROM Employees;
4. Filter Data Appropriately: Use WHERE clauses to filter data within views. This ensures users see only the relevant data, which can improve performance and usability. For example, if you want to provide a view of active customers, you might write:
CREATE VIEW ActiveCustomers AS SELECT CustomerID, CustomerName FROM Customers WHERE IsActive = 1;
5. Ponder Performance Impact: Analyze the performance of your views, especially if they involve complex joins or aggregations. Use indexing on underlying tables to optimize access patterns. If performance becomes an issue, consider materialized views for heavy computations or aggregations. They can significantly enhance query response times:
CREATE MATERIALIZED VIEW MonthlySales AS SELECT MONTH(SaleDate) AS SaleMonth, SUM(SaleAmount) AS TotalSales FROM Sales GROUP BY MONTH(SaleDate);
6. Regularly Review and Update Views: As the underlying data structure evolves, it’s crucial to review existing views to ensure they remain accurate and relevant. Periodic maintenance can help avoid issues caused by changes in the schema, such as missing columns or altered data types. Use the ALTER VIEW statement as needed:
ALTER VIEW EmployeeBasicInfo AS SELECT EmployeeID, FirstName, LastName, Department, HireDate FROM Employees;
7. Implement Security Constraints: Be mindful of the security implications of your views. Follow the principle of least privilege by ensuring that views expose only necessary data to users. Create views that mask sensitive information and restrict access to certain columns or rows. For example:
CREATE VIEW PublicEmployeeInfo AS SELECT EmployeeID, FirstName, LastName, Department FROM Employees WHERE IsPublic = 1;
By adhering to these best practices, you can design SQL views that are not only powerful tools for simplifying data representation but also efficient and secure. A thoughtful approach to view design ensures that they add real value to the database system and improve overall data management.