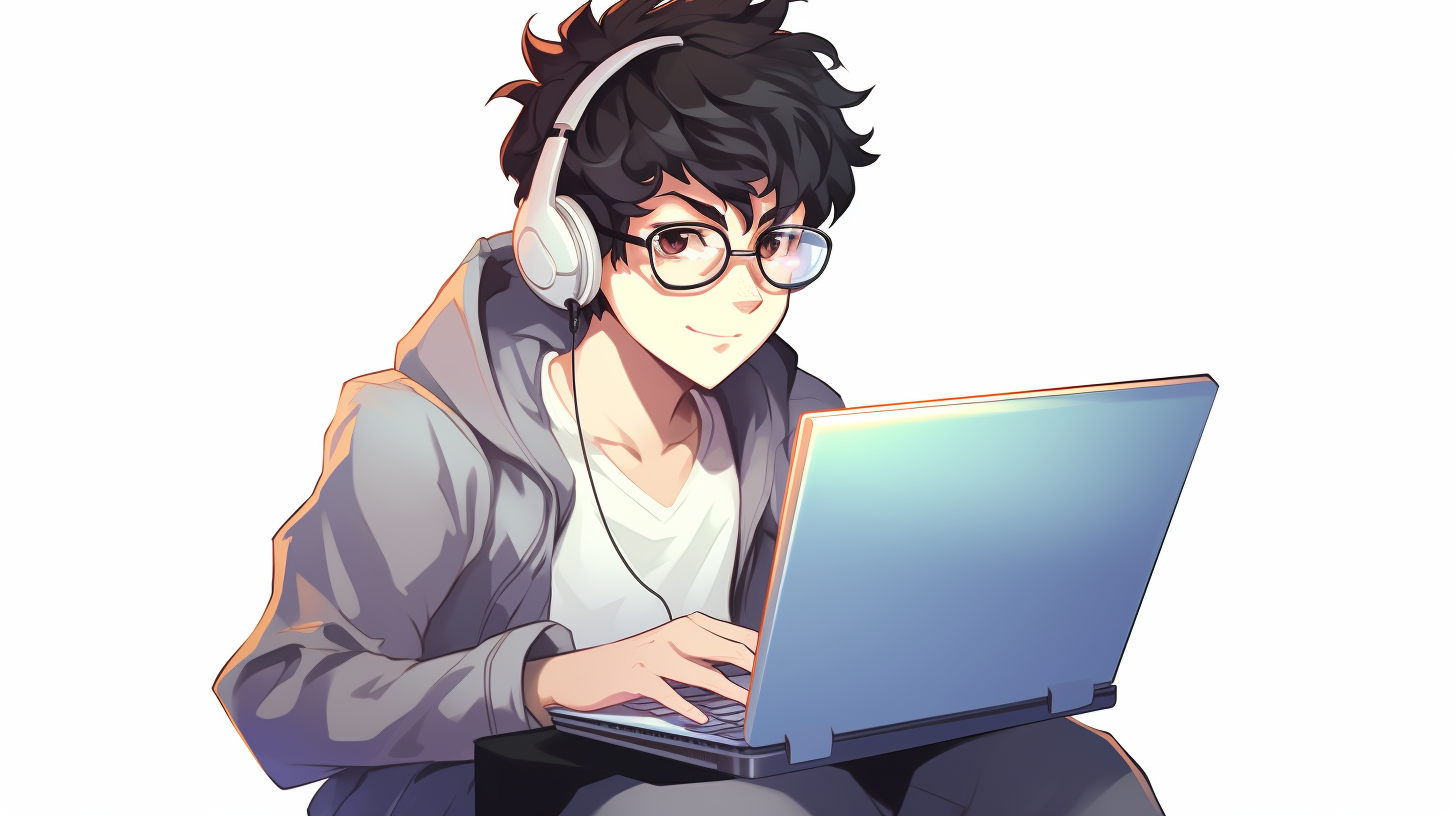
Working with Python Tuples and Sets
In Python, a tuple is an immutable sequence type, which means that once a tuple is created, its contents cannot be altered. This immutability makes tuples a reliable choice for storing data that should not change throughout the life of your program. The syntax for defining a tuple is straightforward: you simply enclose your items within parentheses, separating them with commas.
For instance, think the following example where we create a tuple containing a series of integers:
my_tuple = (1, 2, 3, 4, 5)
Tuples can also store heterogeneous data, meaning that they can contain elements of different types. Here’s an example where we combine integers, strings, and a float:
mixed_tuple = (1, "Hello", 3.14, True)
To access elements in a tuple, you can use indexing, which is similar to lists. Remember that Python uses zero-based indexing, so the first element is accessed with index zero:
print(mixed_tuple[1]) # Output: Hello
Attempting to modify an element within a tuple will result in a TypeError, which underscores the idea of immutability:
mixed_tuple[1] = "World" # Raises TypeError
One of the advantages of using tuples is that they can serve as keys in dictionaries, unlike lists. This is possible due to their immutability, which ensures that their hash value remains constant. This can be particularly useful when you want to create a mapping from compound keys.
Tuples also support various operations such as concatenation and repetition. For example, you can concatenate two tuples simply using the + operator:
new_tuple = my_tuple + mixed_tuple
And you can repeat a tuple using the * operator:
repeated_tuple = my_tuple * 2
This flexibility combined with their immutable nature makes tuples a fundamental and powerful feature in Python programming, suitable for a wide array of applications from data integrity to performance optimization.
Creating and Accessing Tuples
To create a tuple in Python, you don’t have to do much beyond the simple syntax of parentheses and commas that we have seen. However, there are a few nuances worth exploring. For instance, if you want to create a tuple with a single element, you must include a trailing comma. That’s to avoid confusion with regular parentheses used for grouping expressions. Here’s how you can define a single-element tuple:
single_element_tuple = (42,)
Without the comma, Python interprets it as just an integer enclosed in parentheses, resulting in a different data type:
not_a_tuple = (42) # This is just an integer!
When it comes to accessing elements in a tuple, you can use both positive and negative indexing. Positive indexing begins at zero, while negative indexing counts backward from the end of the tuple. For example, if we have the following tuple:
my_data = (10, 20, 30, 40, 50)
You can access elements like this:
first_item = my_data[0] # Accessing the first item: 10 last_item = my_data[-1] # Accessing the last item: 50
As you can see, negative indexing can be quite handy when you need to retrieve elements from the end of a tuple without calculating the exact index. This feature enhances flexibility and can lead to cleaner, more readable code.
Another important aspect of tuples is slicing, which allows you to access a portion of the tuple by specifying a start and an end index. The syntax for slicing is similar to that of lists:
sliced_tuple = my_data[1:4] # This retrieves items at indices 1, 2, and 3 # Result: (20, 30, 40)
Here, slicing is inclusive of the starting index but exclusive of the ending index. You can also omit the starting or ending index to slice from the beginning or to the end of the tuple, respectively:
start_slice = my_data[:3] # Results in (10, 20, 30) end_slice = my_data[2:] # Results in (30, 40, 50)
With this capability, tuples can be quite powerful in managing collections of data while ensuring that their contents remain unchanged. Tuples can also be unpacked, enabling you to assign each element of a tuple to a separate variable in a single assignment statement:
a, b, c = my_data # This assigns 10 to a, 20 to b, and 30 to c
This feature is particularly useful when working with functions that return multiple values bundled in a tuple. Thus, creating and accessing tuples not only provides an immutable data structure but also offers flexibility and convenience in accessing and manipulating data.
Tuple Methods and Immutability
When you ponder tuple methods, it’s essential to understand that the immutability of tuples does limit the operations you can perform directly on them. However, tuples do come with a couple of built-in methods that allow you to interact with their contents while respecting their immutable nature. The two primary methods available for tuples are count and index.
The count method returns the number of times a specified value appears in the tuple. This method is simpler and can be very useful for checking the frequency of an item. Here’s an example:
my_tuple = (1, 2, 3, 1, 4, 1, 5) count_of_ones = my_tuple.count(1) # Returns 3 print(count_of_ones) # Output: 3
In this snippet, we define a tuple containing several integers, including the number 1, which appears three times. The count method tallies those occurrences for us.
On the other hand, the index method is used to find the first index at which a specified value is found in the tuple. If the value is not present, it raises a ValueError. Here’s how it works:
my_tuple = (1, 2, 3, 4, 5) index_of_three = my_tuple.index(3) # Returns 2 print(index_of_three) # Output: 2
In this case, the index method finds the number 3 within the tuple and returns its position, which is 2. It is worth noting that if you search for a value that does not exist in the tuple, you will encounter an error:
my_tuple.index(6) # Raises ValueError
The immutability of tuples has its advantages—most notably, it guarantees that the contents remain unchanged, which very important when you want to maintain the integrity of your data. This property ensures that tuples can be used as keys in dictionaries or elements in sets, since their hash value won’t change over time.
However, this immutability also means that if you need to modify the contents of a tuple, you must create a new tuple instead. This can be accomplished through concatenation or slicing, as previously mentioned. For example:
original_tuple = (1, 2, 3) modified_tuple = original_tuple + (4, 5) # New tuple created print(modified_tuple) # Output: (1, 2, 3, 4, 5)
Here, we concatenate a new tuple containing 4 and 5 to the original tuple, resulting in a new tuple rather than modifying the original. This is a critical behavior to keep in mind when working with tuples in Python.
While tuples may seem limited due to their immutable nature, the available methods provide essential functionality for counting occurrences and locating indices. Understanding these methods, along with the underlying principles of immutability, allows you to leverage tuples effectively in your coding endeavors.
Exploring Python Sets
Python sets are a built-in data type that allows you to store collections of unique elements. Unlike lists or tuples, sets are unordered, meaning that the items do not have a defined order, and they cannot contain duplicate elements. This makes sets particularly useful when you want to ensure that your collection contains only distinct items. The syntax for creating a set is simple: you use curly braces or the set()
constructor. Here’s an example of creating a set:
my_set = {1, 2, 3, 4, 5}
Alternatively, you can create an empty set using the set()
function:
empty_set = set()
One of the key characteristics of sets is that they automatically eliminate any duplicate values. If you attempt to add an item that already exists in the set, it will simply be ignored. Think the following example:
my_set = {1, 2, 3, 3, 4} print(my_set) # Output: {1, 2, 3, 4}
Here, the duplicate value 3
was ignored, demonstrating the uniqueness constraint of sets.
Accessing elements in a set is not possible through indexing, as sets are unordered. However, you can iterate over the elements of a set using a loop. For instance:
for item in my_set: print(item)
This will print each item in the set, albeit in an arbitrary order.
Another interesting aspect of sets is that they support mathematical set operations such as union, intersection, and difference. This can make data manipulation both powerful and intuitive. To illustrate these operations, let’s look at a couple of sets:
set_a = {1, 2, 3, 4} set_b = {3, 4, 5, 6}
The union of two sets combines all unique elements from both sets:
union_set = set_a | set_b # or set_a.union(set_b) print(union_set) # Output: {1, 2, 3, 4, 5, 6}
The intersection of two sets retrieves only the elements that are common to both:
intersection_set = set_a & set_b # or set_a.intersection(set_b) print(intersection_set) # Output: {3, 4}
Lastly, the difference operation shows the elements that are in one set but not the other:
difference_set = set_a - set_b # or set_a.difference(set_b) print(difference_set) # Output: {1, 2}
In this case, difference_set
contains the elements from set_a
that are not in set_b
.
Sets also provide a variety of methods, such as add()
for adding elements, remove()
for deleting specific items, and discard()
, which behaves similarly to remove()
but does not raise an error if the item is not found. For example:
my_set.add(6) print(my_set) # Output: {1, 2, 3, 4, 6} my_set.remove(1) print(my_set) # Output: {2, 3, 4, 6} my_set.discard(10) # Does not raise an error
Sets are useful in numerous scenarios, particularly when you need to ensure the uniqueness of elements or perform mathematical operations on collections. The combination of these features makes sets a versatile and powerful data type in Python, ideal for handling diverse datasets.
Set Operations and Methods
When working with sets in Python, understanding the various operations and methods very important for effective data manipulation. As mentioned previously, sets inherently maintain unique elements, and this distinctive property is leveraged through several built-in methods that facilitate mathematical and logical operations.
To begin with, one of the fundamental operations is the union, which merges two or more sets. The union operation results in a new set containing all unique elements from the involved sets. You can perform a union operation using the pipe operator (|) or the union() method. Ponder the following example:
set_a = {1, 2, 3} set_b = {2, 3, 4} union_set = set_a | set_b # Using the pipe operator print(union_set) # Output: {1, 2, 3, 4}
Here, the union_set successfully combines elements from both set_a and set_b, ensuring that duplicates are removed.
Next, the intersection operation allows you to retrieve only those elements that exist in both sets. This can be accomplished using the ampersand operator (&) or the intersection() method. For example:
set_a = {1, 2, 3, 4} set_b = {3, 4, 5} intersection_set = set_a & set_b # Using the ampersand operator print(intersection_set) # Output: {3, 4}
The result captures only the elements that are common to both sets.
The difference operation, conversely, identifies elements that are present in one set but absent in another. This operation can be executed using the minus operator (-) or the difference() method. Here’s how it works:
difference_set = set_a - set_b # Using the minus operator print(difference_set) # Output: {1, 2}
In this case, difference_set includes elements from set_a that do not appear in set_b, providing clarity on unique members of a set.
Additionally, sets support symmetric difference operations. This operation retrieves elements that are in either of the sets but not in their intersection. You can achieve this using the caret operator (^) or the symmetric_difference() method:
symmetric_difference_set = set_a ^ set_b # Using the caret operator print(symmetric_difference_set) # Output: {1, 2, 5}
Sets also come with several methods that enhance their utility. The add() method allows you to insert new unique elements into a set, while remove() and discard() methods allow for the deletion of elements. The remove() method raises a KeyError if the element does not exist, while discard() simply ignores it:
my_set = {1, 2, 3} my_set.add(4) print(my_set) # Output: {1, 2, 3, 4} my_set.remove(2) print(my_set) # Output: {1, 3, 4} my_set.discard(5) # Does not raise an error
Moreover, the pop() method allows you to remove and return an arbitrary element from the set, which can be useful when the specific element being removed isn’t critical:
removed_element = my_set.pop() print(removed_element) # Output: (an arbitrary element from the set) print(my_set) # Output: {remaining elements}
Ultimately, the combination of these operations and methods makes sets a formidable choice for handling collections of data where uniqueness is essential. Whether you are performing complex mathematical operations or simply needing to manage distinct items, sets provide the tools necessary for efficient and effective data manipulation.
Use Cases for Tuples and Sets
When it comes to practical applications, tuples and sets each serve distinct yet complementary purposes in Python programming. Understanding the use cases for these data structures can enhance your coding efficiency and help you choose the right tool for the right job.
Starting with tuples, their immutability allows them to act as a stable point of reference. This makes them ideal for scenarios where data integrity is paramount. For instance, tuples can be used to hold configuration values that shouldn’t change throughout the execution of a program. Imagine a function that requires a fixed set of parameters; by passing them as a tuple, you can ensure that they remain unchanged:
def configure_system(settings): # settings is a tuple with fixed parameters print(f"Configuring system with: {settings}") config = ("192.168.1.1", 8080, "max_connections=100") configure_system(config) # Output: Configuring system with: ('192.168.1.1', 8080, 'max_connections=100')
Furthermore, tuples shine in scenarios involving multiple return values. When a function needs to return more than one piece of information, using a tuple can be an elegant solution:
def get_user_info(user_id): # Simulate fetching user data return (user_id, "Luke Douglas", 25) # Returns a tuple user_info = get_user_info(1) print(user_info) # Output: (1, 'Frank McKinnon', 25)
In this context, the tuple serves as a convenient way to package and return multiple values without needing a more complex structure.
Shifting gears to sets, their utility becomes apparent when dealing with collections of unique items. One of the most common use cases is when you need to eliminate duplicates from a dataset. For instance, if you have a list of user IDs that may contain duplicates, converting it to a set will automatically filter out any repeated entries:
user_ids = [1, 2, 2, 3, 4, 4, 5] unique_user_ids = set(user_ids) print(unique_user_ids) # Output: {1, 2, 3, 4, 5}
This capability is invaluable in data processing tasks where ensuring the uniqueness of entries is important.
Sets also excel in scenarios requiring mathematical set operations. For instance, let’s say you have two lists representing students enrolled in different courses. By converting these to sets, you can easily determine which students are enrolled in both courses (intersection), those who are only in one course (difference), and those who are in either course but not both (symmetric difference). Here’s how this can be achieved:
course_a = {"Alice", "Bob", "Charlie"} course_b = {"Bob", "Dave", "Eve"} # Intersection common_students = course_a & course_b print(common_students) # Output: {'Bob'} # Difference only_in_a = course_a - course_b print(only_in_a) # Output: {'Alice', 'Charlie'} # Symmetric Difference either_course = course_a ^ course_b print(either_course) # Output: {'Alice', 'Charlie', 'Dave', 'Eve'}
With these operations, sets provide a powerful way to analyze relationships between datasets, making them an essential part of any programmer’s toolkit.
Tuples and sets are not merely basic data structures; they’re versatile tools that, when used correctly, can greatly enhance data integrity and manipulation in Python applications. By knowing when and how to deploy these structures, you can write cleaner, more efficient, and more effective code.