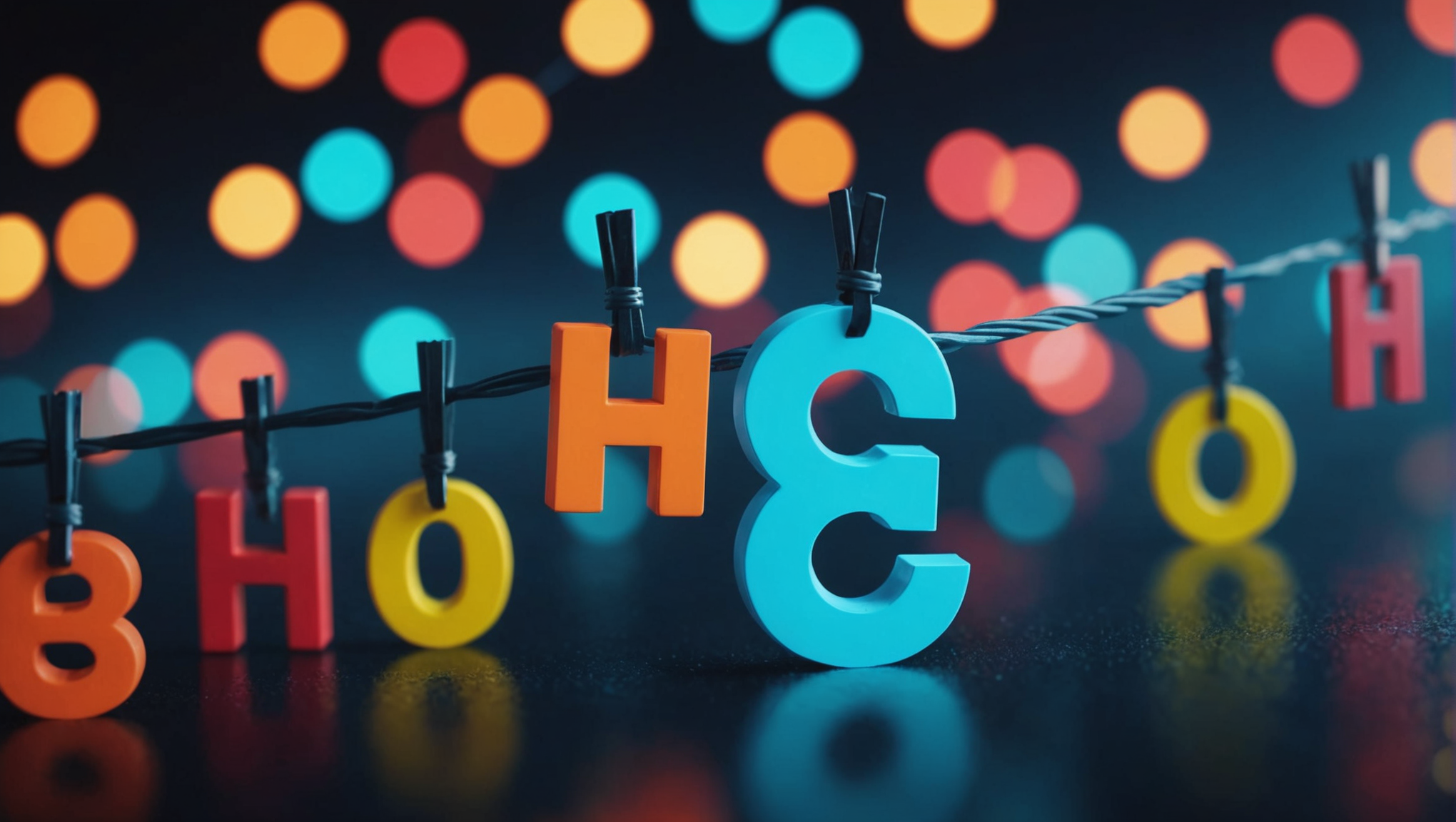
Working with Strings in Swift
In Swift, strings are a fundamental part of the language and are used extensively in various applications. Creating and initializing strings can be accomplished in several ways. Here are some common methods to initialize and create strings in Swift:
- You can create an empty string using the String initializer.
let emptyString = String()
- You can also directly assign a string literal to a variable or constant.
let greeting = "Hello, World!"
- Swift supports multi-line string literals using triple quotes.
let multiLineString = """ This is a string spanning multiple lines. """
- You can initialize a string from other data types, such as integers or doubles.
let number = 42 let stringFromNumber = String(number)
- Similar to other languages, Swift allows you to create strings using formatted strings.
let name = "Alice" let age = 30 let formattedString = String(format: "%@ is %d years old.", name, age)
- You can also create a string from an array of characters.
let charArray: [Character] = ["H", "e", "l", "l", "o"] let stringFromChars = String(charArray)
Swift provides various initializers to create strings that accommodate different needs, making string manipulation a flexible and powerful feature of the language.
String Interpolation and Formatting
String interpolation is a powerful feature in Swift that allows you to construct new strings by embedding variables and expressions directly within a string literal. This feature enhances code readability and streamlines the process of combining strings and values. To use string interpolation, you enclose the variable or expression in parentheses and prepend it with a backslash.
Here’s a simple example of string interpolation in action:
let name = "Bob" let age = 25 let greeting = "Hello, my name is (name) and I am (age) years old." print(greeting) // Output: Hello, my name is Bob and I am 25 years old.
In this example, the variables `name` and `age` are interpolated into the string `greeting`, creating a complete sentence that includes their values.
String interpolation can also include more complex expressions. For instance, you can perform calculations directly within the interpolation syntax:
let num1 = 10 let num2 = 20 let sumMessage = "The sum of (num1) and (num2) is (num1 + num2)." print(sumMessage) // Output: The sum of 10 and 20 is 30.
Swift also provides a way to format numbers and dates within interpolated strings. You can format floating-point numbers to a specific decimal point or format date objects to present them in a user-friendly manner:
let pi = 3.14159 let formattedPi = String(format: "Pi rounded to 2 decimal places is %.2f", pi) print(formattedPi) // Output: Pi rounded to 2 decimal places is 3.14 let today = Date() let dateFormatter = DateFormatter() dateFormatter.dateStyle = .full let formattedDate = "Today's date is (dateFormatter.string(from: today))." print(formattedDate)
In this example, we see how to format a floating-point number and a date, enhancing the output clarity and relevance.
Additionally, you can control text alignment and padding using various format specifiers directly within the interpolation. This feature becomes useful for presenting tables of data or aligning strings in a visually appealing format:
let name = "Alice" let score = 95 let formattedOutput = String(format: "%-10s %03d", name, score) print(formattedOutput) // Output: Alice 095
In this output, the `%-10s` specifier left-aligns the name within a field of 10 characters, and `%03d` pads the score with zeros to ensure it’s always three digits.
Using string interpolation enhances both performance and readability while constructing strings in Swift. It allows developers to effortlessly incorporate dynamic content into their string literals with minimal overhead and maximum clarity.
Common String Manipulation Methods
Swift provides a rich set of methods to manipulate strings effectively, enabling developers to perform various operations such as searching, replacing, and modifying string content. Here are some commonly used string manipulation methods in Swift:
- You can easily find the number of characters in a string using the
count
property.
let sampleString = "Hello, World!" let characterCount = sampleString.count print(characterCount) // Output: 13
- Swift allows you to compare strings using relational operators. This enables checking if strings are equal, greater than, or less than.
let string1 = "apple" let string2 = "banana" if string1 < string2 { print("(string1) is less than (string2)") // Output: apple is less than banana }
- You can extract substrings using the
prefix
,suffix
, andsubstring(with:)
methods. These methods allow you to grab portions of the string easily.
let text = "Swift Programming" let prefix = text.prefix(5) // "Swift" let suffix = text.suffix(11) // "Programming" let startIndex = text.index(text.startIndex, offsetBy: 6) let endIndex = text.index(text.endIndex, offsetBy: -5) let substring = text[startIndex..<endIndex] // "Programming"
- Swift provides methods like
append(_:)
to add content to a string orinsert(_:at:)
to insert a character at a specific position.
var mutableString = "Hello" mutableString.append(", World!") print(mutableString) // Output: Hello, World! mutableString.insert("S", at: mutableString.startIndex) print(mutableString) // Output: SHello, World!
- You can replace parts of a string using the
replacingOccurrences(of:with:)
method. That is useful for tasks like censoring inappropriate words.
let originalString = "The quick brown fox" let modifiedString = originalString.replacingOccurrences(of: "fox", with: "dog") print(modifiedString) // Output: The quick brown dog
- You can split a string into an array of substrings using the
split(separator:)
method, which is handy for parsing data.
let csvString = "apple,banana,cherry" let fruits = csvString.split(separator: ",") print(fruits) // Output: ["apple", "banana", "cherry"]
- Use the
trimmingCharacters(in:)
method to remove unwanted whitespace or specified characters from the beginning and end of a string.
let unwantedSpaces = " Hello, World! " let trimmedString = unwantedSpaces.trimmingCharacters(in: .whitespaces) print(trimmedString) // Output: "Hello, World!"
- The
contains(_:)
method allows you to check if a string contains another substring, which is useful for validation or searching.
let sentence = "The quick brown fox jumps over the lazy dog" if sentence.contains("fox") { print("The sentence contains 'fox'.") // Output: The sentence contains 'fox'. }
These methods provide a solid foundation for manipulating strings in Swift, allowing developers to handle text efficiently and intuitively, making string manipulation both powerful and simpler.
Working with Substrings
In Swift, working with substrings is an essential aspect of string manipulation that allows developers to extract and modify parts of strings efficiently. Substrings in Swift are instances of the `SubString` type, which share memory with the original string. This gives you a performance advantage since you avoid unnecessary copying of string data. Below are some key methods and techniques for working with substrings in Swift:
Creating Substrings
You can create a substring by slicing a string using range operators or through specific methods provided within the Swift Standard Library. Here are a few examples:
let fullString = "Hello, Swift Programming!" let startIndex = fullString.index(fullString.startIndex, offsetBy: 7) let endIndex = fullString.index(fullString.startIndex, offsetBy: 12) let substring = fullString[startIndex..<endIndex] // "Swift" print(substring) // Output: Swift
In the above example, we specify a start and end index to extract the substring “Swift” from the full string.
Using Prefix and Suffix
Swift provides convenient methods to obtain the prefix or suffix portions of a string. The prefix
method extracts the beginning characters, while the suffix
method retrieves characters from the end of the string.
let greeting = "Hello, World!" let prefix = greeting.prefix(5) // "Hello" let suffix = greeting.suffix(6) // "World!" print(prefix) // Output: Hello print(suffix) // Output: World!
Checking Substring Containment
You can check if a substring exists within a string using the contains
method, which returns a Boolean indicating the presence of the specified substring.
let sentence = "Swift is a powerful programming language." let containsSwift = sentence.contains("Swift") // true let containsJava = sentence.contains("Java") // false print(containsSwift) // Output: true print(containsJava) // Output: false
Converting Substrings to Strings
When you need to convert a substring back to a string, you can do so easily, as Swift allows you to create a new string from a substring. That’s often useful when you want to manipulate or store the substring independently.
let partial = sentence.prefix(10) // "Swift is a" let stringFromSubstring = String(partial) // Converts Substring to String print(stringFromSubstring) // Output: Swift is a
Indexing and Slicing Strings
Swift strings are indexed, which will allow you to navigate through them character by character. However, keep in mind that due to the way Swift handles Unicode, indexing requires careful usage of the index
method, as direct integer indexing is not supported.
let indexString = "Swift Programming" let firstCharacter = indexString[indexString.startIndex] // "S" let secondCharacterIndex = indexString.index(indexString.startIndex, offsetBy: 1) let secondCharacter = indexString[secondCharacterIndex] // "w" print(firstCharacter) // Output: S print(secondCharacter) // Output: w
Trimming Whitespace and Newlines
When working with user inputs or strings read from external sources, it’s common to encounter leading or trailing whitespace and newline characters. Swift provides the trimmingCharacters(in:)
method to remove unwanted characters.
let rawString = " Hello, Swift! n" let trimmedString = rawString.trimmingCharacters(in: .whitespacesAndNewlines) print(trimmedString) // Output: Hello, Swift!
Swift’s handling of substrings provides powerful tools to work with portions of strings efficiently and cleanly. Understanding how to create, manipulate, and convert substrings will make string handling in your Swift applications more effective.
Performance Considerations in String Handling
When dealing with strings in Swift, performance considerations are paramount, especially in resource-constrained environments such as mobile applications. Strings in Swift are more than just collections of characters; they are sophisticated data structures optimized for a multitude of operations. Understanding the performance implications of different string operations can help developers write more efficient code. Here are some key aspects to consider:
- Swift strings are value types, which means that when a string is assigned to a new variable or passed to a function, a copy is made. This copying behavior can lead to performance overhead if not managed properly, especially for large strings. However, Swift employs a performance optimization called “copy-on-write” which minimizes unnecessary copies, making it efficient in most cases.
- Swift strings are immutable by default, meaning that once a string is created, it cannot be modified. If you need to perform multiple modifications, it may be beneficial to use a mutable representation, such as constructing an array of characters first, and then converting it to a string once all modifications are done. This can provide better performance than modifying a string repeatedly.
- When you create a substring from a string, Swift generates a view into the original string rather than creating a new copy of the substring. This can lead to better performance while using memory efficiently. However, care should be taken if the original string is modified, as this could invalidate the substring.
- String indexing in Swift is not as simpler as in some other languages due to the complexity of Unicode characters. Operations involving string indices can be slower if not handled properly. Use properties like startIndex, endIndex, and methods like index(_:offsetBy:) to manage indices effectively. Avoid unnecessary index calculations to minimize performance hits.
- Counting the number of characters in a string can be computationally expensive, particularly for long strings and when using complex Unicode characters. Use the
count
property judiciously, especially in loops or performance-critical sections of your code. - Different string operations have different time complexities. For instance, appending strings is generally O(n) where n is the length of the string being appended. Conversely, string comparisons are O(n) as well, where n is the length of the shortest string. When performance is a concern, it is wise to minimize the use of costly operations in loops or frequently called functions.
- While string interpolation is a powerful feature, excessive use of it in performance-critical paths can lead to overhead due to string reconstruction. Be cautious when interpolating within tight loops or performance-sensitive code. If necessary, consider using alternative methods like
String(format:)
or building the string incrementally using an array of strings.
By taking these performance considerations into account, Swift developers can create applications that are not only functional but also efficient in handling strings, resulting in smoother user experiences and better resource utilization.