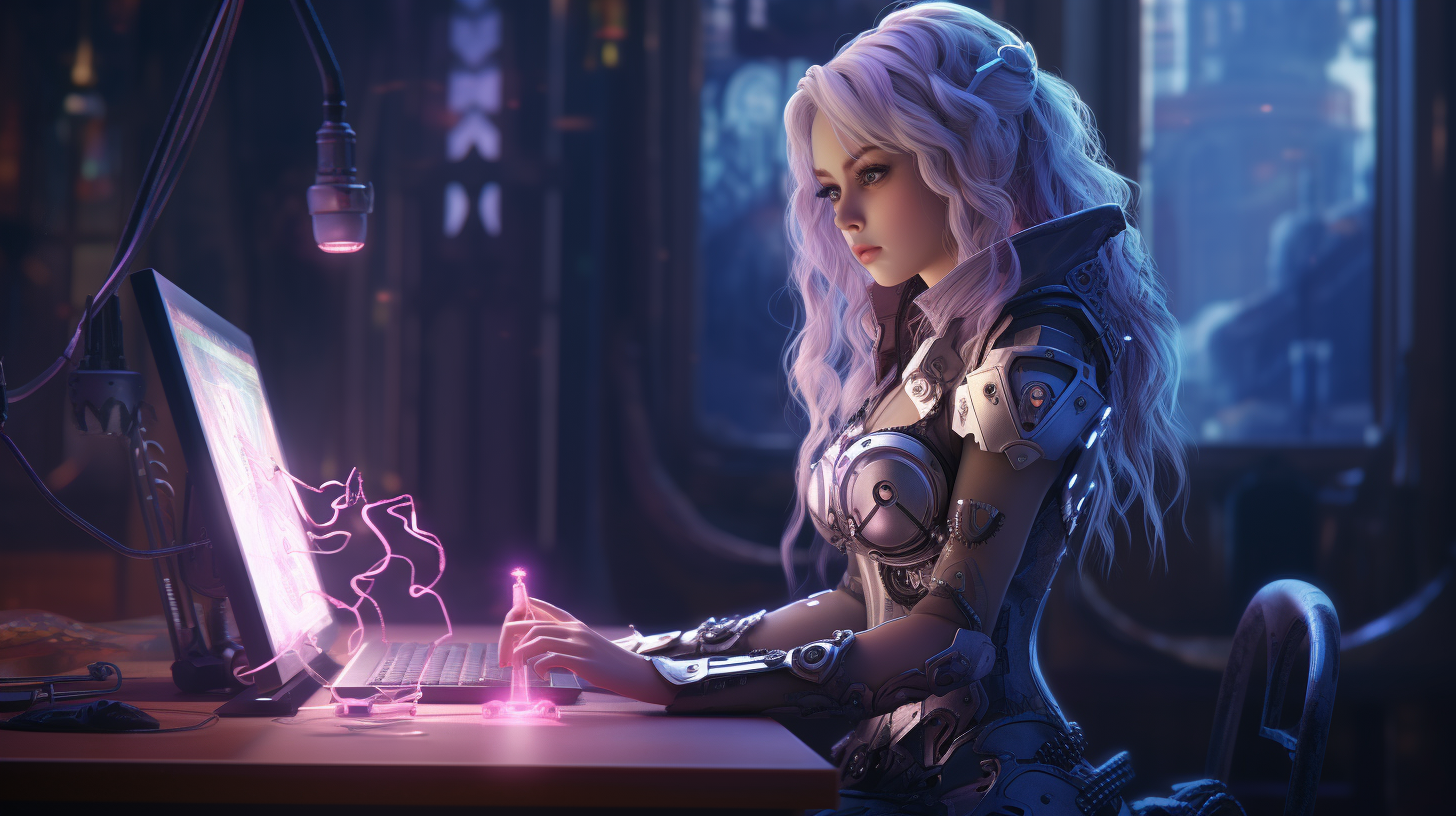
Writing Your First Python Program: A Step-by-Step Guide
To embark on your Python programming journey, the first crucial step is to install Python itself. Python is freely available and works across various operating systems, making it a versatile choice for both beginners and seasoned developers.
Visit the official Python website at python.org. Here, you will find the latest version of Python available for download. Depending on your operating system, select the appropriate installer. For Windows users, downloading the executable file is simpler. macOS users can opt for the installer or use package managers like Homebrew.
Once the installer is downloaded, you’ll want to run it. During the installation process on Windows, ensure to check the box that says Add Python to PATH. This step is important, as it allows you to run Python from the command line without additional configuration.
After following the installation prompts, you can verify the installation by opening a terminal or command prompt and typing:
python --version
Or, for some systems, you might need to use:
python3 --version
This command should return the version number of Python you just installed. If you see an error message instead, revisit the installation steps and ensure that Python is correctly installed.
For complete compatibility with various libraries and frameworks, consider installing pip, Python’s package manager, which is typically included with the Python installation. To check if pip is installed, you can use:
pip --version
With Python and pip installed, you’re now primed to explore Python programming. The next phase involves configuring your development environment, an important step that can significantly enhance your coding experience.
Setting Up Your Development Environment
Setting up your development environment is essential for effective coding in Python. A well-configured environment can streamline your workflow, making it easier to write, test, and debug your scripts. Here’s how to create an optimal setup for your Python programming journey.
First, you need a text editor or an Integrated Development Environment (IDE). Both options have their pros and cons, and the choice often boils down to personal preference. Popular text editors include Sublime Text, Atom, and Visual Studio Code, while well-known IDEs include PyCharm and Thonny. For beginners, Thonny is particularly uncomplicated to manage and comes pre-installed with Python.
To install Visual Studio Code, visit the official website at code.visualstudio.com and download the installer for your operating system. After installation, you can enhance its functionality by installing extensions. For Python, search for the “Python” extension in the Extensions view (accessed via the square icon on the sidebar or by pressing Ctrl+Shift+X). This extension provides features like IntelliSense, linting, and debugging support that are invaluable for developing Python applications.
Another critical component of your development environment is version control. Git is the most widely used version control system, so that you can track changes in your code and collaborate with others. To install Git, download it from git-scm.com. After installation, you can set it up by running the following commands in your terminal or command prompt:
git config --global user.name "Your Name" git config --global user.email "[email protected]"
With Git configured, you can create repositories and manage your project versions. Learning the basic commands, such as git init, git add, git commit, and git push, will set you on the right path to effective code management.
It’s also crucial to organize your projects effectively. Create a dedicated folder for your Python projects. Inside this folder, create subdirectories for each project. For instance:
mkdir ~/PythonProjects cd ~/PythonProjects mkdir Project1 Project2
Within each project folder, you can create virtual environments using Python’s built-in venv module. Virtual environments allow you to manage dependencies separately for each project, preventing conflicts between libraries. To create a virtual environment, navigate to your project folder and run:
python -m venv venv
To activate the virtual environment, use the following command:
# On Windows .venvScriptsactivate # On macOS/Linux source venv/bin/activate
When you’re done with your project, you can deactivate the virtual environment by simply typing:
deactivate
Now, you are ready to install any libraries your project might need using pip, ensuring they remain contained within the virtual environment. For example:
pip install requests
By following these steps to set up your development environment, you’ll lay the groundwork for efficient and effective Python programming. With the right tools at your disposal, you can focus on writing code and solving problems, rather than wrestling with configuration issues.
Understanding Python Syntax and Structure
Understanding Python syntax and structure is a fundamental aspect of becoming an effective programmer. Python is praised for its readability, which is largely due to its clean and consistent syntax. Unlike some programming languages that require extensive boilerplate code, Python allows you to express concepts in fewer lines of code, enhancing clarity and maintainability.
One of the most distinguishing features of Python is its indentation-based structure. Indentation is not just for readability; it defines the blocks of code. This means that proper indentation especially important, as it directly affects how your code is interpreted by the Python interpreter.
For instance, think the following example:
def greet(name): print("Hello, " + name + "!") # Indented block of code greet("Alice")
In this example, the print statement is indented within the function greet
. If you remove the indentation, you will encounter an IndentationError, which illustrates how Python uses indentation to group statements. This eliminates the need for braces or keywords to denote code blocks, leading to code this is often more simpler to read and understand.
Python also utilizes comments to improve code comprehension. Single-line comments are initiated with the #
symbol, while multi-line comments can be created using triple quotes. Here’s how you can use comments effectively:
# That's a single-line comment print("Hello, World!") # This prints a greeting """ This is a multi-line comment. It can span several lines. """ print("Goodbye, World!")
Next, let’s delve into variables. In Python, you don’t need to declare variable types explicitly; Python automatically infers the type based on the assigned value. For example:
name = "Alice" # String age = 30 # Integer height = 5.5 # Float is_student = True # Boolean
Here, the variables name
, age
, height
, and is_student
are created without any type declarations. This dynamic typing allows for greater flexibility, but it’s important to be mindful of the types you are using, as they can lead to runtime errors if mismanaged.
Control structures in Python, such as if
statements, for
loops, and while
loops, also adhere to the indentation rule. Here’s an example of an if
statement:
temperature = 30 if temperature > 25: print("It's a hot day!") # This block executes if the condition is true else: print("It's a cool day.")
In this snippet, the indented lines under the if
and else
clauses are executed based on the condition evaluated. This logical flow is easy to follow, enhancing the readability of your code.
Finally, Python supports functions as first-class objects. You can define functions, pass them as arguments, and even return them from other functions. Here’s a simple example demonstrating a function returning another function:
def outer_function(msg): def inner_function(): print(msg) return inner_function my_greeting = outer_function("Hello, World!") my_greeting() # This will output: Hello, World!
This example showcases the power of Python’s function handling capabilities, making it possible to create higher-order functions that can simplify complex programming tasks.
Understanding these core principles of Python syntax and structure will set a solid foundation for your programming journey. The simplicity and elegance inherent in Python’s design make it a powerful language for both beginners and experienced coders alike.
Writing Your First Python Script
Now that you have a grasp of Python’s syntax and structure, it’s time to put that knowledge to practical use by writing your first Python script. A script is simply a collection of Python commands saved in a file, and creating one is a simpler yet rewarding task. Let’s walk through how to write your first script step-by-step.
Start by creating a new file in your text editor or IDE. You can name it something like hello.py
. The .py extension indicates that this is a Python file. Open this file and type in the following code:
print("Hello, World!")
This simple command instructs Python to output the text “Hello, World!” to the console. It serves as a traditional first program in many programming tutorials, and it’s a great way to familiarize yourself with the basic structure of a Python script.
Next, save your file. Now, you’re ready to run your script. Open your terminal or command prompt, navigate to the directory where your hello.py
file is located, and execute the following command:
python hello.py
Or, if your system requires it, you might need to use:
python3 hello.py
Press Enter, and you should see the output:
Hello, World!
Congratulations! You’ve successfully written and executed your first Python script. This might seem like a small step, but it’s a significant milestone in your programming journey.
To deepen your understanding, let’s modify your script to accept user input. This will illustrate how to interact with users through your program. Update your hello.py
file as follows:
name = input("What's your name? ") print("Hello, " + name + "!")
In this revised script, the input
function prompts the user to enter their name, which is then stored in the variable name
. The program then greets the user with a personalized message. Save your changes and run the script again:
python hello.py
This time, when you execute the program, it will ask for your name. After you input it, you’ll receive a personalized greeting. For example:
What's your name? Alice Hello, Alice!
This exercise introduces fundamental concepts like variables and user input, which are essential in programming. As you progress, you will encounter more complex scenarios that build upon these foundational skills.
Keep experimenting with your scripts. Try adding more features, such as asking for additional information, performing calculations, or even writing conditional statements that alter the program’s behavior based on user input. Each modification will enhance your understanding of Python and bolster your confidence as a programmer.
Now that you’ve successfully written and executed your first Python script, you’re on your way to mastering more advanced programming techniques. The journey ahead is filled with exciting challenges and opportunities to learn, so embrace it wholeheartedly.
Running and Testing Your Program
With your first Python script under your belt, the next step is to become proficient at running and testing your programs. This process especially important to ensuring that your code works as intended and helps you identify any potential issues before deploying your application. Let’s delve into some techniques and practices that will enhance your programming workflow.
To run your Python scripts, you’ll primarily use the command line or terminal. As you’ve already done, navigating to the directory containing your script and executing it with python your_script.py
or python3 your_script.py
is the standard approach. However, many modern IDEs and text editors allow you to run scripts directly from within the application. For example, in Visual Studio Code, you can run your script by simply pressing F5 or selecting the Run option from the menu. This integration can save time and streamline the development process.
Once your script is running, you’ll want to focus on testing. Testing is an essential aspect of software development, as it helps ensure that your code behaves as expected under various conditions. Here are some fundamental testing concepts to consider:
1. Manual Testing: This involves running your code and observing the output. You may need to test various inputs to see how the script reacts. For instance, when testing the greeting example, try different names, including special characters and empty strings:
name = input("What's your name? ") print("Hello, " + name + "!")
By inputting diverse values, you can check if your program handles edge cases without errors.
2. Automated Testing: As your projects grow more complex, manual testing can become cumbersome. Automated tests allow you to write scripts that run tests for you. Python has a built-in module called unittest
that you can use to create a test suite for your code. Here’s a simple example:
import unittest def greet(name): return "Hello, " + name + "!" class TestGreeting(unittest.TestCase): def test_greet(self): self.assertEqual(greet("Alice"), "Hello, Alice!") self.assertEqual(greet("Bob"), "Hello, Bob!") if __name__ == "__main__": unittest.main()
In this snippet, we define a function, greet
, and create a test case to verify its correctness. Running this test will automatically check if the function returns the expected output for the given inputs.
3. Debugging: Occasionally, your code may not work as expected, leading to bugs. Python provides several tools to help you debug your scripts effectively. The print()
function is commonly used to output variable values or messages at different stages of your program, helping you trace the flow of execution. Additionally, Python’s built-in pdb
(Python Debugger) module allows you to step through your code line by line, inspect variables, and understand where things might be going awry. To use it, simply insert the following line where you want the debugger to pause:
import pdb; pdb.set_trace()
When you run your program, it will stop execution at this line, so that you can examine the current state of your program interactively.
4. Using Assertions: You can also include assert
statements in your code to ensure certain conditions hold true during execution. If an assertion fails, it raises an AssertionError
, which can help catch bugs early. Here’s how you can implement it:
def divide(x, y): assert y != 0, "Cannot divide by zero!" return x / y
This code will raise an error if someone attempts to divide by zero, effectively preventing a runtime error later in execution.
Mastering the art of running and testing your Python programs is vital for developing robust applications. Embrace these testing strategies and debugging techniques as you continue your journey in Python programming. Each step in this process will not only improve your usage in coding but also bolster your confidence as you tackle more complex projects.
Debugging Common Errors in Python
Debugging is an inevitable part of programming, and Python offers various mechanisms to help you identify and resolve issues in your code. Understanding common errors and how to interpret error messages can greatly ease this process. Let’s explore some of the most frequently encountered errors in Python and how to debug them effectively.
One of the most common types of errors you will encounter is the SyntaxError. This occurs when Python encounters code that it doesn’t understand, which often happens due to typos or incorrect formatting. For instance:
print("Hello, World!" # Missing closing parenthesis
When you run this code, Python will throw a SyntaxError, indicating precisely where it encountered the problem. Always read the error message carefully; it will guide you to the line and character where the error occurred.
Another frequent source of headaches is the IndentationError. Python relies on indentation to define code blocks, so inconsistent or incorrect indentation will lead to errors. For example:
def greet(name): print("Hello, " + name + "!") # Incorrect indentation
In this case, the print statement should be indented. Python will inform you of the indentation problem, highlighting the lack of proper structure in your code.
NameError is another common issue that arises when you reference a variable that hasn’t been defined. If you try to run code like this:
print(message) # 'message' has not been defined
You will receive a NameError, which indicates that Python doesn’t recognize the variable ‘message’. Ensure that you’ve defined your variables before attempting to use them.
Similarly, there’s the TypeError, which occurs when an operation or function is applied to an object of an inappropriate type. For instance:
result = "The answer is: " + 42 # Concatenating string and integer
This will raise a TypeError because you cannot concatenate a string and an integer directly. To resolve this, you need to convert the integer to a string:
result = "The answer is: " + str(42)
Beyond understanding specific errors, adopting a systematic debugging approach very important. Here are a few effective debugging strategies:
1. **Read the Error Message**: Python’s error messages are generally helpful. They tell you what went wrong and where. Use this information as your first step toward resolution.
2. **Use Print Statements**: Inserting print statements throughout your code can help you track the values of variables at different execution points. This simple technique often reveals the root cause of issues quickly.
x = 10 y = 0 print("Before division") # Debugging statement result = x / y # This will raise an error
3. **Utilize Python’s Built-in Debugger**: The Python Debugger (pdb) allows you to set breakpoints, step through code line by line, and inspect variable states interactively. This provides precise control over execution and helps you understand program flow deeply.
import pdb def divide(x, y): pdb.set_trace() # Set a breakpoint here return x / y divide(10, 0) # Running this will invoke the debugger on error
4. **Employ Assertions**: Assertions can be embedded into your code to ensure certain conditions are met. If an assertion fails, it will raise an AssertionError, which can help catch logical errors early in your program’s execution.
def calculate_area(length, width): assert length > 0 and width > 0, "Length and width must be positive!" return length * width
5. **Check External Resources**: Sometimes, the issue may stem from external libraries or APIs. Ensure that any libraries you are using are properly installed and compatible with your Python version. Reviewing documentation for specific functions can also provide insights into their expected behavior.
By incorporating these debugging techniques into your programming routine, you will become more adept at identifying and resolving issues within your code. Remember, debugging is as much an art as it is a skill, and with experience, you will develop your own intuitive methods for tackling errors effectively.